Preparing for Coding Interviews While Working Full-Time: A Comprehensive Guide
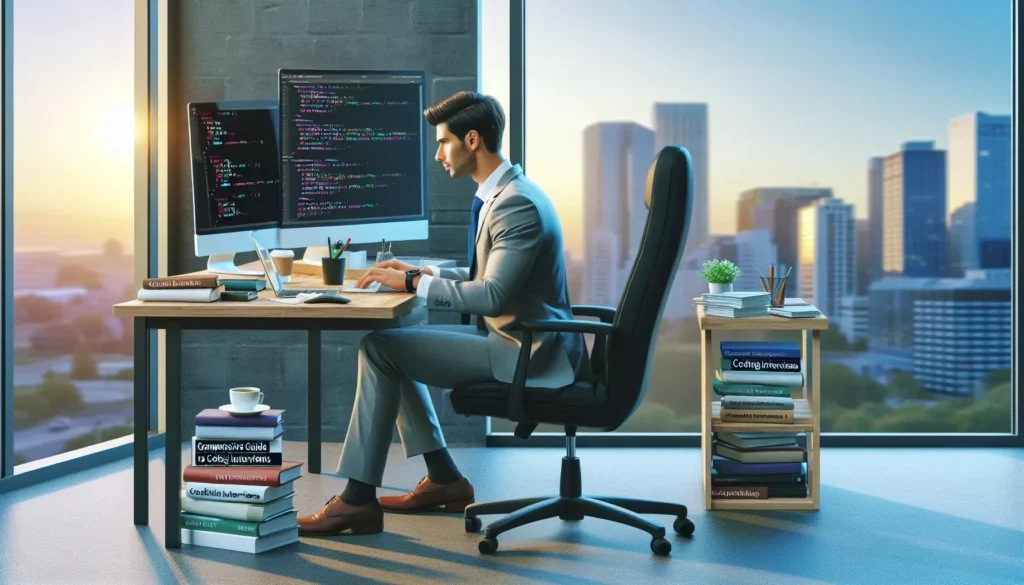
Balancing a full-time job while preparing for coding interviews can be a daunting task. However, with the right strategies and mindset, it’s entirely possible to excel in both areas. This comprehensive guide will walk you through effective methods to prepare for technical interviews, manage your time efficiently, and maintain a healthy work-life balance. Whether you’re aiming for a position at a FAANG company (Facebook, Amazon, Apple, Netflix, Google) or any other tech giant, these tips will help you navigate the challenging journey of interview preparation while juggling your current job responsibilities.
1. Understanding the Importance of Preparation
Before diving into the strategies, it’s crucial to understand why thorough preparation is essential for coding interviews, especially when targeting top tech companies:
- High competition: FAANG and similar companies receive thousands of applications for each position.
- Rigorous interview process: These companies are known for their challenging technical interviews that test not just coding skills but also problem-solving abilities and algorithmic thinking.
- Comprehensive evaluation: Interviews often cover a wide range of topics, from data structures and algorithms to system design and behavioral questions.
- Career growth: Succeeding in these interviews can lead to significant career advancement and learning opportunities.
2. Creating a Structured Study Plan
The key to effective preparation while working full-time is having a well-structured study plan. Here’s how to create one:
2.1. Assess Your Current Skills
Start by evaluating your strengths and weaknesses. Take mock tests or solve problems on platforms like LeetCode or HackerRank to identify areas that need improvement.
2.2. Set Realistic Goals
Break down your preparation into smaller, achievable goals. For example:
- Master one data structure or algorithm concept per week
- Solve 5-10 coding problems weekly
- Complete one system design question every two weeks
2.3. Create a Timeline
Depending on your target interview date, create a timeline that covers all necessary topics. Be sure to allocate more time to your weak areas.
2.4. Use a Study Planner
Utilize tools like Trello, Notion, or even a simple spreadsheet to track your progress and organize your study materials.
3. Maximizing Your Study Time
When working full-time, every minute counts. Here are strategies to make the most of your limited study time:
3.1. Utilize Commute Time
If you commute to work, use this time productively. Listen to coding podcasts, review flashcards, or read tech blogs.
3.2. Wake Up Earlier
Consider waking up 1-2 hours earlier than usual to study when your mind is fresh. Consistency is key here.
3.3. Lunch Break Learning
Use part of your lunch break to solve a coding problem or review a concept.
3.4. Weekend Deep Dives
Dedicate larger chunks of time during weekends for in-depth learning and practice.
3.5. Pomodoro Technique
Use the Pomodoro Technique (25 minutes of focused work followed by a 5-minute break) to maintain concentration during study sessions.
4. Focusing on High-Impact Study Areas
To make your preparation more efficient, focus on high-impact areas that are frequently tested in coding interviews:
4.1. Data Structures and Algorithms
Master the following key data structures and algorithms:
- Arrays and Strings
- Linked Lists
- Trees and Graphs
- Stacks and Queues
- Hash Tables
- Sorting and Searching Algorithms
- Dynamic Programming
- Recursion and Backtracking
4.2. Problem-Solving Techniques
Practice various problem-solving approaches:
- Two-pointer technique
- Sliding window
- Divide and conquer
- Greedy algorithms
- Binary search variations
4.3. System Design
For senior roles, system design is crucial. Study:
- Scalability principles
- Database design
- Caching strategies
- Load balancing
- Microservices architecture
4.4. Programming Language Proficiency
Deepen your knowledge of your preferred programming language. Know its intricacies, best practices, and common pitfalls.
5. Leveraging Online Resources
Take advantage of the wealth of online resources available for interview preparation:
5.1. Coding Platforms
- LeetCode: Offers a vast collection of coding problems with difficulty levels and company tags.
- HackerRank: Provides coding challenges and contests to improve your skills.
- CodeSignal: Offers coding assessments and interview practice.
5.2. Online Courses
- Coursera: Offers courses on algorithms and data structures from top universities.
- Udemy: Provides targeted courses for coding interview preparation.
- AlgoCademy: Offers interactive coding tutorials and AI-powered assistance for interview preparation.
5.3. Books
Some essential books for interview preparation include:
- “Cracking the Coding Interview” by Gayle Laakmann McDowell
- “Elements of Programming Interviews” by Adnan Aziz, Tsung-Hsien Lee, and Amit Prakash
- “System Design Interview” by Alex Xu
5.4. YouTube Channels
Follow coding interview preparation channels like:
- Back To Back SWE
- Tech Dose
- Clement Mihailescu
6. Practicing Mock Interviews
Practicing mock interviews is crucial for building confidence and improving your performance. Here’s how to incorporate them into your preparation:
6.1. Online Platforms
Use platforms like Pramp or InterviewBit that offer peer-to-peer mock interviews.
6.2. Study Groups
Form or join a study group with colleagues or friends who are also preparing for interviews. Conduct mock interviews for each other.
6.3. Professional Interview Coaching
Consider investing in professional interview coaching services for personalized feedback and strategies.
6.4. Record Yourself
Record your mock interview sessions to review your performance, body language, and communication skills.
7. Maintaining Work-Life Balance
While preparing intensively, it’s crucial to maintain a healthy work-life balance to avoid burnout:
7.1. Set Boundaries
Clearly define your study hours and stick to them. Avoid letting interview preparation encroach on your work responsibilities or personal time.
7.2. Take Regular Breaks
Incorporate short breaks and leisure activities into your schedule to recharge.
7.3. Stay Physically Active
Regular exercise can boost your energy levels and cognitive function, making your study sessions more productive.
7.4. Practice Mindfulness
Incorporate mindfulness or meditation techniques to manage stress and improve focus.
8. Leveraging Your Current Job for Preparation
Your current job can be a valuable asset in your interview preparation:
8.1. Apply Learned Concepts
Look for opportunities to apply the concepts you’re learning in your current work projects.
8.2. Seek Challenging Tasks
Volunteer for projects that will stretch your skills and align with areas you’re focusing on for interviews.
8.3. Learn from Colleagues
Engage in technical discussions with coworkers and learn from their experiences.
9. Handling Coding Challenges
Many companies include coding challenges as part of their interview process. Here’s how to approach them:
9.1. Time Management
Practice solving problems within time constraints. Most coding challenges have a time limit.
9.2. Read Instructions Carefully
Pay close attention to the problem statement and requirements. Misunderstanding the question is a common pitfall.
9.3. Test Your Code
Always test your code with various inputs, including edge cases, before submitting.
9.4. Optimize Your Solution
After solving the problem, look for ways to optimize your solution in terms of time and space complexity.
10. Mastering the Art of Problem-Solving
Developing strong problem-solving skills is crucial for coding interviews. Here’s a step-by-step approach:
10.1. Understand the Problem
Read the problem statement carefully and ask clarifying questions if needed.
10.2. Plan Your Approach
Before coding, outline your approach. Consider different algorithms and data structures that could be applicable.
10.3. Write Pseudocode
Jot down the high-level steps of your solution in pseudocode.
10.4. Implement the Solution
Translate your pseudocode into actual code. Start with a brute force solution if needed, then optimize.
10.5. Test and Debug
Test your solution with various inputs, including edge cases. Debug any issues you encounter.
10.6. Analyze Time and Space Complexity
Be prepared to discuss the time and space complexity of your solution.
11. Tackling System Design Questions
For senior roles, system design questions are crucial. Here’s how to approach them:
11.1. Clarify Requirements
Start by asking questions to understand the scope and constraints of the system.
11.2. Estimate Scale
Determine the scale of the system (e.g., number of users, data volume) to guide your design decisions.
11.3. Design High-Level Architecture
Sketch out the main components of the system and how they interact.
11.4. Deep Dive into Components
Choose key components to elaborate on, discussing trade-offs and potential optimizations.
11.5. Address Bottlenecks
Identify potential bottlenecks in your design and propose solutions to address them.
12. Behavioral Interview Preparation
Don’t neglect preparing for behavioral questions. They’re an important part of the interview process:
12.1. Use the STAR Method
Structure your responses using the Situation, Task, Action, Result (STAR) method.
12.2. Prepare Stories
Have a set of go-to stories that highlight your skills and experiences.
12.3. Practice Common Questions
Prepare for common questions like “Tell me about a time you faced a challenge at work” or “How do you handle disagreements with team members?”
13. The Week Before the Interview
As your interview approaches, focus on these final preparation steps:
13.1. Review Your Notes
Go through your study materials and review key concepts.
13.2. Solve Practice Problems
Continue solving problems, but focus on reinforcing concepts rather than learning new ones.
13.3. Rest and Relax
Ensure you’re well-rested. Avoid cramming the night before the interview.
13.4. Prepare Logistically
If it’s an online interview, test your setup. For in-person interviews, plan your route and arrival time.
14. During the Interview
On the day of the interview, keep these tips in mind:
14.1. Stay Calm
Take deep breaths and remember that nervousness is normal.
14.2. Think Aloud
Verbalize your thought process as you solve problems. Interviewers want to understand how you think.
14.3. Ask for Clarification
Don’t hesitate to ask questions if you’re unsure about something.
14.4. Manage Your Time
Keep an eye on the time and pace yourself accordingly.
15. Post-Interview Reflection
After the interview, take some time to reflect:
15.1. Review Your Performance
Make notes on what went well and areas for improvement.
15.2. Follow Up
Send a thank-you email to your interviewers, reiterating your interest in the position.
15.3. Continue Learning
Regardless of the outcome, continue improving your skills and preparing for future opportunities.
Conclusion
Preparing for coding interviews while working full-time is challenging, but with dedication, smart strategies, and effective time management, it’s entirely achievable. Remember that the journey of preparation is also a valuable learning experience that will enhance your skills and make you a better developer, regardless of the interview outcome.
By following this comprehensive guide, you’ll be well-equipped to balance your current job responsibilities with your interview preparation. Stay focused, maintain a consistent study routine, and leverage the wealth of resources available to you. With persistence and the right approach, you’ll be well on your way to acing your coding interviews and taking the next step in your tech career.
Good luck with your preparation, and may your hard work lead you to success in your future interviews!