Understanding Testing and Debugging in Coding Interviews: Essential Skills for Success
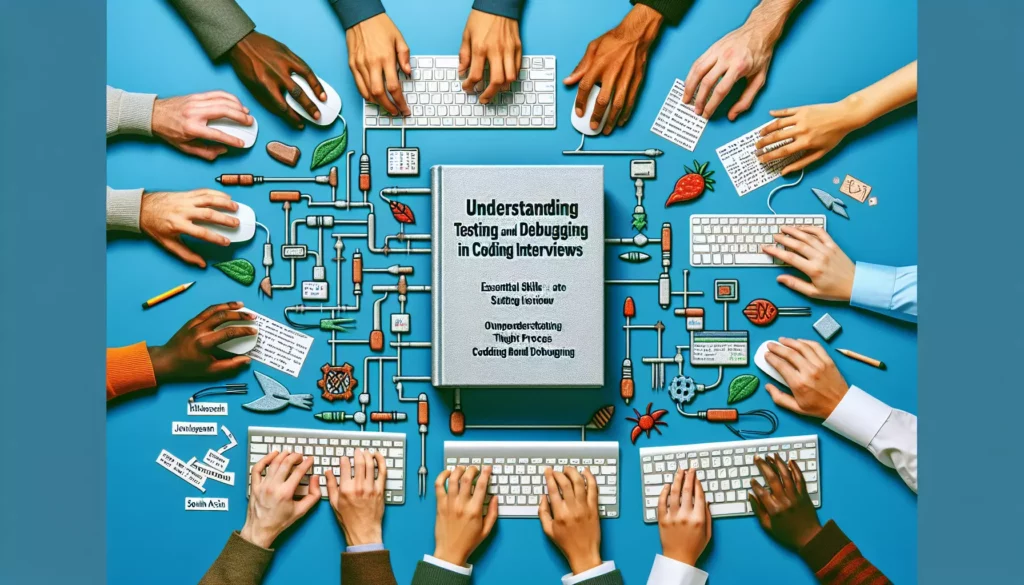
In the competitive world of tech recruitment, coding interviews have become a crucial step in the hiring process, especially for positions at major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, and Google). While mastering algorithms and data structures is undoubtedly important, many candidates overlook two equally critical skills: testing and debugging. These skills not only demonstrate your ability to write functional code but also showcase your problem-solving prowess and attention to detail. In this comprehensive guide, we’ll explore the importance of testing and debugging in coding interviews and provide strategies to help you excel in these areas.
The Importance of Testing and Debugging in Coding Interviews
Before diving into specific techniques, it’s crucial to understand why testing and debugging are so valued in coding interviews:
- Quality Assurance: Employers want to hire developers who can write reliable, bug-free code. Demonstrating your ability to test and debug effectively shows that you prioritize code quality.
- Problem-Solving Skills: Testing and debugging require analytical thinking and methodical problem-solving, which are highly sought-after skills in the tech industry.
- Efficiency: Developers who can quickly identify and fix issues save time and resources, making them valuable assets to any team.
- Communication: Explaining your testing and debugging process showcases your ability to articulate technical concepts clearly, an essential skill for collaborative work environments.
Mastering Testing in Coding Interviews
Testing is the process of verifying that your code works as intended. In a coding interview, you’ll often be expected to demonstrate your testing skills. Here are some key strategies to help you excel:
1. Start with Edge Cases
Always begin by considering edge cases – these are extreme or unusual inputs that might cause your code to fail. Common edge cases include:
- Empty inputs (e.g., empty strings, arrays, or lists)
- Very large or very small numbers
- Negative numbers (if applicable)
- Null or undefined values
- Inputs with special characters or whitespace
By addressing these cases first, you demonstrate thoroughness and foresight.
2. Use the Input-Process-Output (IPO) Model
The IPO model is a systematic approach to testing:
- Input: What are the possible inputs? Consider different data types, sizes, and formats.
- Process: How does your code handle these inputs? What transformations or calculations occur?
- Output: What are the expected outputs for each input? Are there any special cases to consider?
Using this model helps ensure you’ve covered all aspects of your code’s functionality.
3. Implement Test-Driven Development (TDD)
While you may not have time for full TDD in an interview, adopting its principles can be beneficial:
- Write a failing test case before implementing the solution
- Write the minimum code necessary to pass the test
- Refactor the code while ensuring the test still passes
This approach helps you stay focused on the requirements and ensures your code meets the specified criteria.
4. Use Assert Statements
Many programming languages provide assert statements, which are excellent for testing. They allow you to specify conditions that must be true for your code to be correct. For example, in Python:
def add(a, b):
return a + b
assert add(2, 3) == 5, "Addition of 2 and 3 should equal 5"
assert add(-1, 1) == 0, "Addition of -1 and 1 should equal 0"
assert add(0, 0) == 0, "Addition of 0 and 0 should equal 0"
Using assert statements demonstrates your commitment to code correctness and makes your testing process explicit.
5. Consider Time and Space Complexity
While testing for correctness, also consider the efficiency of your solution. Test with larger inputs to ensure your code performs well in terms of both time and space complexity. This shows that you’re thinking beyond just functionality and considering real-world application scenarios.
Effective Debugging Strategies for Coding Interviews
Debugging is the process of identifying and fixing errors in your code. In a coding interview, your ability to debug efficiently can set you apart. Here are some strategies to enhance your debugging skills:
1. Use Print Statements Strategically
While more advanced debugging tools exist, print statements remain a quick and effective way to understand what’s happening in your code. Use them to:
- Track variable values at different points in your code
- Verify the flow of execution
- Identify where unexpected behavior occurs
For example, in JavaScript:
function binarySearch(arr, target) {
let left = 0;
let right = arr.length - 1;
while (left <= right) {
let mid = Math.floor((left + right) / 2);
console.log(`Left: ${left}, Right: ${right}, Mid: ${mid}, Value: ${arr[mid]}`);
if (arr[mid] === target) {
return mid;
} else if (arr[mid] < target) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return -1;
}
These print statements help visualize the algorithm’s progress and can quickly reveal issues in the logic.
2. Implement Rubber Duck Debugging
Rubber duck debugging is a method where you explain your code line-by-line to an inanimate object (traditionally, a rubber duck). This process often helps you spot errors or inconsistencies in your logic. In an interview setting, you can use this technique by explaining your code to the interviewer, which has the added benefit of demonstrating your communication skills.
3. Use Debugging Tools
If the interview environment allows, familiarize yourself with debugging tools provided by your IDE or programming language. Common features include:
- Breakpoints: Pause execution at specific lines of code
- Step-through execution: Run your code line-by-line
- Variable inspection: Examine the values of variables at different points in execution
- Call stack examination: Understand the sequence of function calls leading to the current point
Being proficient with these tools can significantly speed up your debugging process.
4. Implement Binary Search Debugging
For larger codebases or complex algorithms, use a binary search approach to debugging:
- Identify a point in the code where everything is working correctly
- Identify a point where the bug manifests
- Check the midpoint between these two points
- Repeat the process, narrowing down the problematic section
This method can quickly isolate issues in larger pieces of code.
5. Check for Off-by-One Errors
Off-by-one errors are common in programming, especially when dealing with loops and array indices. Always double-check your loop conditions and array access. For example:
// Incorrect: This will cause an off-by-one error
for (let i = 0; i <= arr.length; i++) {
console.log(arr[i]);
}
// Correct
for (let i = 0; i < arr.length; i++) {
console.log(arr[i]);
}
6. Use Version Control for Debugging
While not always applicable in interview settings, understanding how to use version control systems like Git for debugging is valuable. Techniques like bisecting can help identify when a bug was introduced in larger projects.
Putting It All Together: A Practical Example
Let’s walk through a typical coding interview problem, demonstrating how to incorporate testing and debugging throughout the process.
Problem: Write a function that finds the longest palindromic substring in a given string.
Here’s an implementation with integrated testing and debugging:
function longestPalindromicSubstring(s) {
if (!s || s.length < 2) {
return s;
}
let start = 0;
let maxLength = 1;
function expandAroundCenter(left, right) {
while (left >= 0 && right < s.length && s[left] === s[right]) {
const currentLength = right - left + 1;
if (currentLength > maxLength) {
start = left;
maxLength = currentLength;
}
left--;
right++;
}
}
for (let i = 0; i < s.length; i++) {
expandAroundCenter(i, i); // Odd length palindromes
expandAroundCenter(i, i + 1); // Even length palindromes
// Debugging: Print current state
console.log(`Index: ${i}, Current longest: ${s.substring(start, start + maxLength)}`);
}
return s.substring(start, start + maxLength);
}
// Testing
function runTests() {
const testCases = [
{ input: "babad", expected: "bab" },
{ input: "cbbd", expected: "bb" },
{ input: "a", expected: "a" },
{ input: "", expected: "" },
{ input: "abcde", expected: "a" },
{ input: "abcba", expected: "abcba" },
];
testCases.forEach((testCase, index) => {
const result = longestPalindromicSubstring(testCase.input);
console.log(`Test ${index + 1}:`);
console.log(`Input: "${testCase.input}"`);
console.log(`Expected: "${testCase.expected}"`);
console.log(`Got: "${result}"`);
console.log(`Pass: ${result === testCase.expected}`);
console.log("---");
});
}
runTests();
In this example, we’ve incorporated several testing and debugging techniques:
- We start with edge cases (empty string, single character) in our implementation.
- We use console.log statements to track the algorithm’s progress, helping us understand its behavior and identify potential issues.
- We implement a separate testing function with multiple test cases, covering various scenarios.
- We compare the expected output with the actual output, clearly indicating whether each test passes.
By presenting your solution with integrated testing and debugging, you demonstrate to the interviewer that you prioritize code quality and have a methodical approach to problem-solving.
Conclusion: Elevating Your Coding Interview Performance
Mastering testing and debugging skills is crucial for success in coding interviews, particularly when aiming for positions at top tech companies. These skills demonstrate your ability to write robust, error-free code and showcase your problem-solving capabilities.
Remember these key points:
- Always consider edge cases and use systematic testing approaches like the IPO model.
- Implement debugging techniques such as strategic print statements and rubber duck debugging.
- Practice explaining your testing and debugging process clearly, as communication is a vital part of the interview.
- Use tools and methodologies like assert statements, debugging tools, and version control when appropriate.
- Incorporate testing and debugging naturally into your problem-solving process during interviews.
By honing these skills and integrating them into your coding practice, you’ll not only perform better in interviews but also develop habits that will serve you well throughout your programming career. Platforms like AlgoCademy offer invaluable resources and interactive tutorials to help you refine these skills, providing a solid foundation for tackling complex coding challenges and preparing for technical interviews at top tech companies.
Remember, becoming proficient in testing and debugging is an ongoing process. Continual practice and real-world application will help you internalize these skills, making them second nature during high-pressure interview situations. With dedication and the right approach, you’ll be well-equipped to showcase your abilities and stand out in the competitive tech job market.