Mastering Live Coding Assessments: Your Ultimate Guide to Interview Success
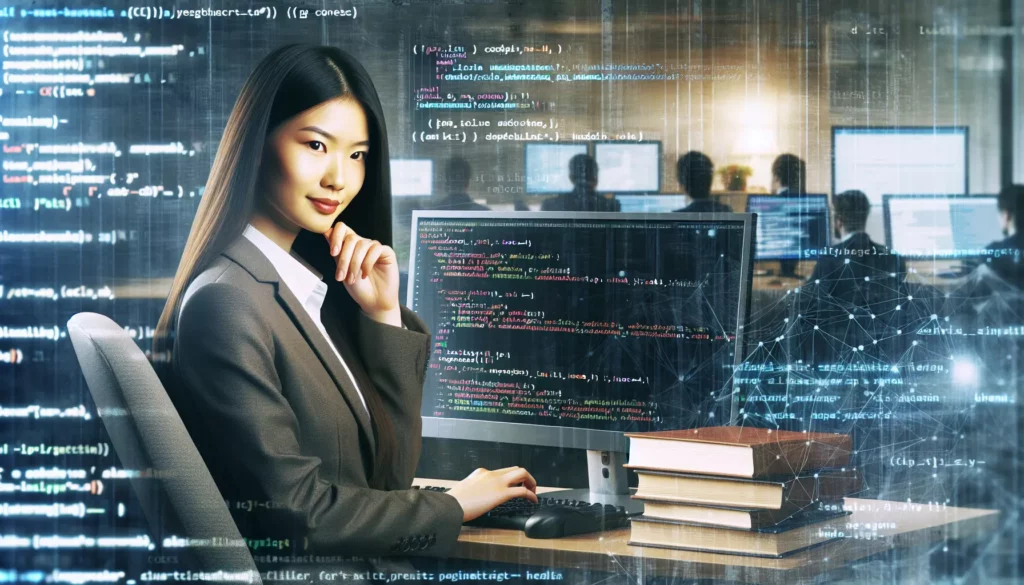
In today’s competitive tech industry, live coding assessments have become an integral part of the interview process for software engineering positions. These real-time evaluations challenge candidates to demonstrate their problem-solving skills, coding proficiency, and ability to think on their feet. For many aspiring developers, these assessments can be a source of anxiety and stress. However, with the right preparation and mindset, you can turn these challenges into opportunities to showcase your skills and land your dream job.
In this comprehensive guide, we’ll explore strategies, tips, and best practices for handling live coding assessments during interviews. Whether you’re a fresh graduate or an experienced developer looking to make a career move, this article will equip you with the tools you need to excel in your next coding interview.
Understanding Live Coding Assessments
Before we dive into the strategies for success, let’s first understand what live coding assessments entail and why companies use them.
What Are Live Coding Assessments?
Live coding assessments are real-time evaluations where candidates are asked to solve programming problems or implement specific features while the interviewer observes. These assessments can take various forms:
- Whiteboard coding: Writing code on a physical or virtual whiteboard
- Pair programming: Collaborating with the interviewer to solve a problem
- Online coding platforms: Using web-based IDEs to write and run code
- Take-home projects: Completing a coding task within a specified timeframe
Why Do Companies Use Live Coding Assessments?
Companies employ live coding assessments for several reasons:
- To evaluate problem-solving skills in real-time
- To assess coding proficiency and style
- To observe how candidates handle pressure and communicate their thought process
- To gauge a candidate’s ability to collaborate and receive feedback
- To simulate real-world scenarios that developers might encounter on the job
Preparing for Live Coding Assessments
Success in live coding assessments starts with thorough preparation. Here are some key steps to help you get ready:
1. Master the Fundamentals
Ensure you have a solid grasp of fundamental programming concepts, data structures, and algorithms. Review topics such as:
- Arrays and strings
- Linked lists
- Trees and graphs
- Stacks and queues
- Hash tables
- Sorting and searching algorithms
- Dynamic programming
- Object-oriented programming principles
2. Practice, Practice, Practice
Regular practice is crucial for building confidence and improving your problem-solving skills. Here are some ways to practice:
- Solve coding challenges on platforms like LeetCode, HackerRank, or CodeSignal
- Participate in coding competitions or hackathons
- Contribute to open-source projects
- Work on personal coding projects
- Engage in mock interviews with peers or mentors
3. Familiarize Yourself with Common Interview Questions
While you can’t predict exactly what questions you’ll be asked, familiarizing yourself with common interview questions can help you feel more prepared. Some popular types of questions include:
- String manipulation
- Array operations
- Tree traversal
- Graph algorithms
- Dynamic programming problems
- System design questions
4. Study the Company and Role
Research the company you’re interviewing with and the specific role you’re applying for. This can help you tailor your preparation and demonstrate your interest during the interview. Pay attention to:
- The company’s tech stack and programming languages
- Recent projects or products the company has launched
- The company’s values and culture
- Specific requirements mentioned in the job description
Strategies for Success During the Assessment
Now that you’re prepared, let’s explore strategies to help you excel during the live coding assessment itself.
1. Clarify the Problem
Before you start coding, make sure you fully understand the problem at hand. Don’t hesitate to ask questions to clarify any ambiguities. This shows the interviewer that you’re thorough and helps ensure you’re solving the right problem.
- Ask about input constraints and edge cases
- Confirm the expected output format
- Discuss any assumptions you’re making
2. Plan Your Approach
Take a moment to plan your approach before diving into coding. This demonstrates your ability to think strategically and can help you avoid getting stuck later on.
- Sketch out a high-level algorithm
- Consider different approaches and their trade-offs
- Discuss your thought process with the interviewer
3. Start with a Simple Solution
Begin with a straightforward, working solution before optimizing. This ensures you have something functional to show and discuss, even if you don’t have time to implement the most efficient solution.
- Implement a brute-force approach if necessary
- Explain that you’re starting with a simple solution and plan to optimize later
- Focus on correctness before efficiency
4. Think Aloud
Verbalize your thought process as you work through the problem. This gives the interviewer insight into your problem-solving approach and allows them to provide guidance if needed.
- Explain your reasoning for choosing a particular approach
- Discuss any challenges or roadblocks you encounter
- Share your thoughts on potential optimizations
5. Write Clean, Readable Code
Even under pressure, strive to write clean, well-organized code. This demonstrates your ability to produce maintainable code in real-world scenarios.
- Use meaningful variable and function names
- Include comments to explain complex logic
- Follow consistent indentation and formatting
6. Test Your Code
Once you’ve implemented a solution, take the time to test it. This shows attention to detail and a commitment to quality.
- Walk through your code with a sample input
- Consider edge cases and boundary conditions
- Discuss potential test cases with the interviewer
7. Optimize and Refactor
If time allows, discuss and implement optimizations to your initial solution. This demonstrates your ability to iterate and improve your code.
- Analyze the time and space complexity of your solution
- Identify areas for improvement
- Explain the trade-offs of different optimization strategies
8. Stay Calm and Positive
Maintain a positive attitude throughout the assessment, even if you encounter difficulties. Your ability to handle pressure and maintain composure is just as important as your technical skills.
- Take deep breaths if you feel stressed
- View challenges as opportunities to learn and showcase your problem-solving skills
- Remember that it’s okay to ask for hints or clarification if you’re stuck
Common Pitfalls to Avoid
Being aware of common mistakes can help you navigate live coding assessments more successfully. Here are some pitfalls to watch out for:
1. Jumping into Coding Too Quickly
Resist the urge to start coding immediately without fully understanding the problem or planning your approach. Taking the time to clarify and plan can save you time and frustration in the long run.
2. Overcomplicating the Solution
Don’t try to impress the interviewer with an overly complex solution. Start with a simple, working approach and optimize from there. Remember, a clear, functional solution is often better than a partially implemented complex one.
3. Ignoring Edge Cases
Pay attention to potential edge cases and boundary conditions. Failing to consider these scenarios can lead to bugs in your code and demonstrate a lack of thoroughness.
4. Staying Silent
Avoid long periods of silence during the assessment. Even if you’re stuck, communicate your thoughts and challenges to the interviewer. They may provide hints or guidance to help you move forward.
5. Neglecting Time Management
Keep an eye on the time and pace yourself accordingly. If you’re spending too long on one part of the problem, consider moving on and coming back to it later if time allows.
6. Becoming Defensive
If the interviewer provides feedback or suggestions, receive them graciously. Showing that you can take constructive criticism and collaborate effectively is crucial in a team-oriented work environment.
After the Assessment
Once you’ve completed the live coding assessment, there are still opportunities to leave a positive impression:
1. Reflect on Your Solution
Take a moment to review your code and discuss any areas you think could be improved. This shows self-awareness and a commitment to continuous improvement.
2. Ask Thoughtful Questions
Use any remaining time to ask questions about the role, team, or company. This demonstrates your genuine interest and engagement in the opportunity.
3. Express Gratitude
Thank the interviewer for their time and the opportunity to showcase your skills. Regardless of the outcome, maintaining a positive and professional demeanor is important.
4. Follow Up
After the interview, consider sending a thank-you email to reiterate your interest in the position and briefly mention any key points from your discussion.
Leveraging Tools and Resources
To enhance your preparation for live coding assessments, consider leveraging the following tools and resources:
1. Online Coding Platforms
Familiarize yourself with popular online coding platforms that are often used in technical interviews. Some examples include:
- HackerRank
- LeetCode
- CodeSignal
- CoderPad
Practice using these platforms to get comfortable with their interfaces and features.
2. Interactive Learning Resources
Take advantage of interactive coding tutorials and courses to strengthen your skills. Some popular options include:
- Codecademy
- freeCodeCamp
- AlgoCademy
- Coursera
3. Algorithm Visualization Tools
Use algorithm visualization tools to better understand complex algorithms and data structures. Some helpful resources include:
- VisuAlgo
- Algorithm Visualizer
- Data Structure Visualizations
4. Mock Interview Platforms
Practice with mock interview platforms to simulate real interview conditions. Some options to consider:
- Pramp
- InterviewBit
- Gainlo
5. Version Control Systems
Familiarize yourself with version control systems, particularly Git. Many live coding assessments may involve using Git for version control or discussing your experience with it.
Conclusion
Live coding assessments can be challenging, but with the right preparation and mindset, they’re also an excellent opportunity to showcase your skills and problem-solving abilities. Remember that the goal of these assessments is not just to arrive at the correct solution, but to demonstrate your thought process, coding style, and ability to communicate effectively under pressure.
By following the strategies outlined in this guide, practicing regularly, and leveraging available tools and resources, you can approach your next live coding assessment with confidence. Remember that every interview, regardless of the outcome, is a valuable learning experience that can help you grow as a developer.
As you continue your journey in software development, platforms like AlgoCademy can be invaluable resources for honing your skills and preparing for technical interviews. With interactive tutorials, AI-powered assistance, and a focus on algorithmic thinking and problem-solving, AlgoCademy can help you build the confidence and competence needed to excel in live coding assessments and beyond.
Good luck with your upcoming interviews, and remember: with dedication, practice, and the right approach, you have the power to turn live coding assessments from a source of stress into a platform for success!