Mastering the Art: Effective Strategies for Practicing Coding Problems
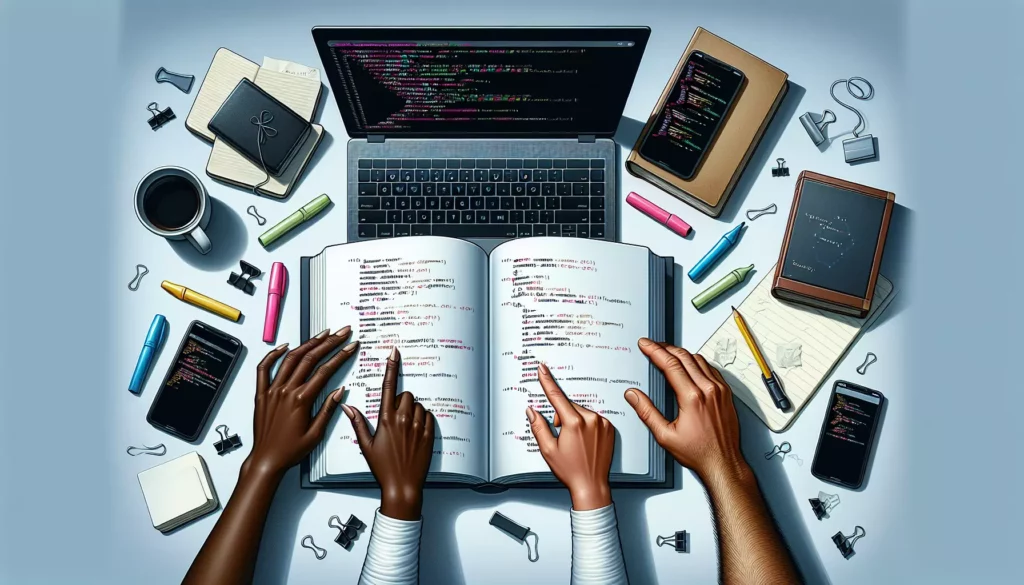
In the ever-evolving world of technology, the ability to code efficiently and solve complex problems is a highly sought-after skill. Whether you’re a beginner looking to break into the tech industry or an experienced developer aiming to land a job at a top-tier company like Google or Amazon, mastering coding problems is crucial. This comprehensive guide will explore various strategies to help you practice coding problems effectively, enhancing your skills and boosting your confidence in tackling technical interviews.
Understanding the Importance of Coding Practice
Before diving into specific strategies, it’s essential to understand why practicing coding problems is so important:
- Skill Improvement: Regular practice helps you refine your coding skills, making you more proficient and efficient.
- Problem-Solving Abilities: Coding problems enhance your analytical thinking and problem-solving capabilities.
- Interview Preparation: Many technical interviews, especially for FAANG (Facebook, Amazon, Apple, Netflix, Google) companies, involve solving coding problems on the spot.
- Algorithmic Thinking: Practice helps you recognize patterns and develop a intuition for algorithmic approaches.
- Confidence Building: As you solve more problems, you’ll gain confidence in your abilities, which is crucial for interviews and real-world coding scenarios.
1. Start with the Basics
Before tackling complex algorithms and data structures, ensure you have a solid foundation in the basics of programming. This includes:
- Understanding fundamental data types (integers, strings, booleans, etc.)
- Mastering control structures (if-else statements, loops)
- Familiarity with basic data structures (arrays, lists, dictionaries)
- Grasping the concept of functions and modular programming
Platforms like AlgoCademy offer interactive tutorials and exercises that can help you build this foundation. Start with simple problems that focus on these basics before moving on to more complex challenges.
2. Develop a Consistent Practice Routine
Consistency is key when it comes to improving your coding skills. Establish a regular practice routine that works for your schedule. Here are some tips:
- Set Aside Dedicated Time: Allocate specific time slots for coding practice, whether it’s daily or a few times a week.
- Start Small: Begin with 30 minutes to an hour per session and gradually increase as you build the habit.
- Use a Timer: Time yourself while solving problems to simulate interview conditions and improve your speed.
- Track Your Progress: Keep a log of the problems you’ve solved and areas you need to improve.
3. Choose the Right Problems
Selecting appropriate coding problems is crucial for effective practice. Here’s how to approach problem selection:
- Gradual Progression: Start with easier problems and gradually move to more difficult ones as you improve.
- Diverse Problem Types: Practice a variety of problem types, including array manipulation, string processing, dynamic programming, and graph algorithms.
- Focus on Weak Areas: Identify your weaknesses and prioritize problems that address those areas.
- Use Curated Problem Sets: Platforms like AlgoCademy often provide curated sets of problems designed to cover key concepts and common interview questions.
4. Understand the Problem Before Coding
One common mistake is jumping into coding without fully understanding the problem. Follow these steps:
- Read the Problem Statement Carefully: Ensure you understand all requirements and constraints.
- Identify Input and Output: Clearly define what inputs the problem expects and what output it should produce.
- Consider Edge Cases: Think about potential edge cases or special scenarios that your solution needs to handle.
- Write Pseudocode: Before actual coding, outline your approach in pseudocode to organize your thoughts.
5. Implement Time Management Techniques
Effective time management is crucial, especially when preparing for timed technical interviews. Try these techniques:
- The Pomodoro Technique: Work in focused 25-minute intervals, followed by short breaks.
- Time Boxing: Allocate specific time limits for different stages of problem-solving (e.g., 5 minutes for understanding, 15 minutes for coding).
- Practice Under Timed Conditions: Regularly solve problems with a timer to simulate interview pressure.
6. Utilize the “Think Aloud” Method
The “Think Aloud” method involves verbalizing your thought process as you solve a problem. This technique is beneficial because:
- It helps you organize your thoughts more clearly.
- It simulates the interview experience where you often need to explain your approach.
- It can reveal gaps in your understanding or flaws in your logic.
Practice explaining your thought process out loud or to a study partner as you work through coding problems.
7. Embrace the Power of Whiteboarding
Many technical interviews involve solving problems on a whiteboard. To prepare:
- Practice writing code by hand or on a physical whiteboard.
- Focus on clear, legible handwriting and proper indentation.
- Learn to manage space effectively on the whiteboard.
- Get comfortable talking through your solution while writing.
8. Learn to Optimize Your Solutions
Don’t stop at finding a working solution. Learn to optimize your code for better time and space complexity:
- Analyze your initial solution for inefficiencies.
- Consider alternative data structures or algorithms that might be more efficient.
- Practice explaining the time and space complexity of your solutions using Big O notation.
- Implement and compare multiple solutions to the same problem.
9. Leverage Online Resources and Communities
Take advantage of the wealth of online resources available:
- Coding Platforms: Use sites like AlgoCademy, LeetCode, HackerRank, or CodeSignal for a wide range of practice problems.
- Video Tutorials: Watch explanations of complex algorithms and problem-solving techniques on platforms like YouTube.
- Coding Communities: Join forums or communities like Stack Overflow or Reddit’s r/learnprogramming to ask questions and share knowledge.
- Online Courses: Enroll in structured courses that cover algorithms and data structures in depth.
10. Review and Learn from Others’ Solutions
After solving a problem, take time to review other solutions:
- Compare your solution with the most upvoted or efficient solutions on coding platforms.
- Analyze different approaches to the same problem.
- Try to understand and implement solutions that are more elegant or efficient than yours.
- Learn new coding patterns and techniques from experienced programmers.
11. Practice Mock Interviews
Simulate the interview experience to become more comfortable with the process:
- Pair up with a study buddy for mock interviews.
- Use platforms that offer mock interview services with real interviewers.
- Practice explaining your thought process and engaging with your interviewer.
- Get feedback on your performance and areas for improvement.
12. Focus on Understanding, Not Just Memorization
While it’s important to know common algorithms and data structures, focus on understanding the underlying principles:
- Learn why certain approaches work better for specific problems.
- Understand the trade-offs between different algorithms and data structures.
- Practice applying concepts to new, unfamiliar problems.
- Develop the ability to combine different techniques to solve complex problems.
13. Implement a Spaced Repetition System
Spaced repetition is a learning technique that involves reviewing material at increasing intervals. Apply this to coding practice:
- Revisit problems you’ve solved after a few days or weeks.
- Try to solve them again without looking at your previous solution.
- Focus more time on problems you find challenging.
- Use tools or create your own system to track when to review certain topics or problems.
14. Break Down Complex Problems
When faced with a complex problem, break it down into smaller, manageable parts:
- Identify the main components or steps required to solve the problem.
- Solve each sub-problem independently.
- Combine the solutions to sub-problems to create the final solution.
- Practice this approach regularly to improve your problem-solving skills.
15. Learn to Test Your Code Effectively
Developing strong testing skills is crucial for writing robust code:
- Write test cases before implementing your solution.
- Consider edge cases and boundary conditions.
- Learn to use debugging tools effectively.
- Practice writing unit tests for your code.
16. Stay Updated with Industry Trends
The tech industry is constantly evolving. Stay informed about current trends and technologies:
- Follow tech blogs and news sites.
- Attend coding meetups or conferences.
- Experiment with new programming languages or frameworks.
- Understand how emerging technologies might impact coding practices and interview processes.
17. Build Projects to Apply Your Skills
Apply your coding skills to real-world projects:
- Develop personal projects that interest you.
- Contribute to open-source projects.
- Implement algorithms and data structures in practical applications.
- Use projects to showcase your skills to potential employers.
18. Embrace the Growth Mindset
Developing coding skills is a journey. Embrace a growth mindset:
- View challenges as opportunities to learn and grow.
- Don’t be discouraged by difficult problems; see them as chances to improve.
- Celebrate your progress and small victories along the way.
- Learn from your mistakes and use them to guide your future learning.
Conclusion
Mastering coding problems is a journey that requires dedication, consistency, and the right strategies. By implementing these effective practices, you can significantly enhance your problem-solving skills, ace technical interviews, and become a more proficient programmer. Remember, the key is not just to practice, but to practice smartly and consistently.
Platforms like AlgoCademy provide structured learning paths, interactive coding environments, and AI-powered assistance to support your journey. Whether you’re preparing for a FAANG interview or simply looking to improve your coding skills, these strategies will help you navigate the challenging but rewarding world of programming.
Start small, stay consistent, and gradually tackle more complex problems. With time and effort, you’ll find yourself solving coding challenges with increased confidence and efficiency. Happy coding!