Understanding Memory Management Concepts: A Comprehensive Guide for Programmers
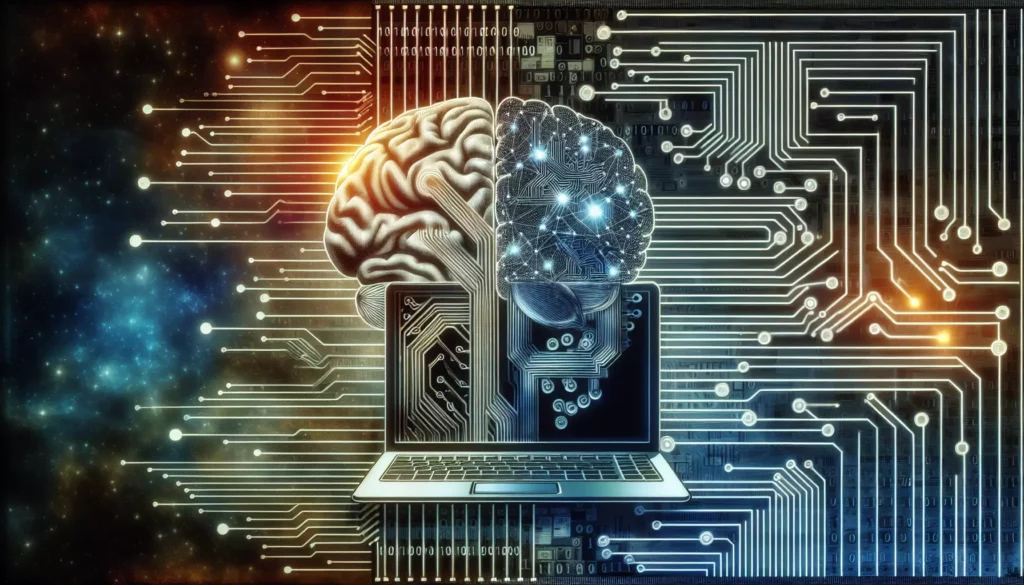
Memory management is a crucial aspect of programming that every developer should understand. Whether you’re a beginner just starting your coding journey or an experienced programmer preparing for technical interviews at major tech companies, having a solid grasp of memory management concepts is essential. In this comprehensive guide, we’ll dive deep into the world of memory management, exploring its importance, key concepts, and practical applications in various programming languages.
Table of Contents
- The Importance of Memory Management
- Key Memory Management Concepts
- Stack vs. Heap Memory
- Memory Allocation and Deallocation
- Garbage Collection
- Memory Leaks and How to Prevent Them
- Memory Management in Different Programming Languages
- Best Practices for Efficient Memory Management
- Memory Management in Technical Interviews
- Conclusion
1. The Importance of Memory Management
Memory management is the process of controlling and coordinating how a program uses computer memory. It involves allocating memory when needed, freeing it when no longer required, and ensuring that memory is used efficiently throughout a program’s execution. Understanding memory management is crucial for several reasons:
- Performance optimization: Efficient memory management leads to better program performance and faster execution times.
- Resource utilization: Proper memory management ensures that system resources are used effectively, allowing programs to run smoothly on devices with limited memory.
- Preventing crashes: Good memory management practices help avoid common issues like memory leaks and segmentation faults, which can cause programs to crash.
- Scalability: As programs grow in size and complexity, effective memory management becomes increasingly important for maintaining performance and stability.
- Cross-platform development: Understanding memory management concepts helps developers write more portable code that can run efficiently on different platforms and devices.
2. Key Memory Management Concepts
Before diving into the details of memory management, it’s essential to familiarize yourself with some key concepts:
2.1. Memory Allocation
Memory allocation is the process of reserving a portion of computer memory for a specific purpose. This can be done statically (at compile-time) or dynamically (at runtime).
2.2. Memory Deallocation
Memory deallocation is the process of releasing previously allocated memory when it’s no longer needed, making it available for reuse by other parts of the program or other applications.
2.3. Memory Leak
A memory leak occurs when a program fails to release memory that is no longer being used, leading to a gradual loss of available memory over time.
2.4. Garbage Collection
Garbage collection is an automatic memory management technique used by some programming languages to identify and remove objects that are no longer needed by the program.
2.5. Pointer
A pointer is a variable that stores the memory address of another variable, allowing direct access to that memory location.
2.6. Reference
A reference is an alias for an existing variable, providing an alternative name to access the same memory location.
3. Stack vs. Heap Memory
Understanding the difference between stack and heap memory is crucial for effective memory management. Let’s explore these two types of memory and their characteristics:
3.1. Stack Memory
Stack memory is a region of memory used for storing local variables, function parameters, and return addresses. Key characteristics of stack memory include:
- Fast allocation and deallocation
- Limited size (typically much smaller than heap memory)
- Automatic memory management (variables are automatically deallocated when they go out of scope)
- LIFO (Last-In-First-Out) data structure
- Used for storing primitive data types and small objects
Example of stack memory usage in C++:
void stackExample() {
int x = 5; // Allocated on the stack
double y = 3.14; // Also allocated on the stack
// x and y are automatically deallocated when the function ends
}
3.2. Heap Memory
Heap memory is a larger region of memory used for dynamic memory allocation. Key characteristics of heap memory include:
- Slower allocation and deallocation compared to stack memory
- Larger size (typically limited only by the available system memory)
- Manual memory management (in languages without garbage collection)
- No specific order of allocation or deallocation
- Used for storing large objects and data structures with a longer lifetime
Example of heap memory usage in C++:
void heapExample() {
int* ptr = new int; // Allocates memory on the heap
*ptr = 10;
// ... use the allocated memory
delete ptr; // Manually deallocate the memory
}
4. Memory Allocation and Deallocation
Memory allocation and deallocation are fundamental operations in memory management. Let’s explore how these processes work in different programming languages:
4.1. C and C++
In C and C++, developers have direct control over memory allocation and deallocation:
C Memory Management:
#include <stdlib.h>
// Allocation
int* ptr = (int*)malloc(sizeof(int));
// Deallocation
free(ptr);
C++ Memory Management:
// Allocation
int* ptr = new int;
// Deallocation
delete ptr;
// Array allocation
int* arr = new int[10];
// Array deallocation
delete[] arr;
4.2. Java
Java uses automatic memory management through garbage collection:
// Object creation (allocation)
Object obj = new Object();
// No explicit deallocation needed
// obj will be automatically garbage collected when no longer referenced
4.3. Python
Python also uses automatic memory management:
# Object creation (allocation)
obj = SomeClass()
# No explicit deallocation needed
# obj will be automatically garbage collected when no longer referenced
5. Garbage Collection
Garbage collection is an automatic memory management technique used by many modern programming languages. It relieves developers from manually deallocating memory by automatically identifying and removing objects that are no longer needed.
5.1. How Garbage Collection Works
The basic process of garbage collection involves:
- Mark: The garbage collector identifies and marks all objects that are still in use (reachable from the program’s root set).
- Sweep: It then frees the memory occupied by unmarked (unreachable) objects.
- Compact: Optionally, it may reorganize the remaining objects to reduce fragmentation.
5.2. Garbage Collection Strategies
Different programming languages and runtime environments may use various garbage collection strategies:
- Mark-and-Sweep: The basic algorithm described above.
- Copying Collection: Divides the heap into two spaces, copying live objects from one space to another.
- Generational Collection: Separates objects based on their age, focusing collection efforts on younger objects.
- Incremental Collection: Performs garbage collection in small increments to reduce pause times.
- Concurrent Collection: Allows the garbage collector to run concurrently with the application.
5.3. Advantages and Disadvantages of Garbage Collection
Advantages:
- Eliminates many common memory-related errors, such as dangling pointers and double frees
- Simplifies memory management for developers
- Can lead to better memory utilization in some cases
Disadvantages:
- Can introduce performance overhead and unpredictable pauses
- May consume more memory than manual management in some scenarios
- Reduces control over when memory is freed
6. Memory Leaks and How to Prevent Them
Memory leaks occur when a program fails to release memory that is no longer needed, leading to a gradual loss of available memory. This can cause performance degradation and eventually program crashes. Let’s explore common causes of memory leaks and how to prevent them:
6.1. Common Causes of Memory Leaks
- Forgetting to free allocated memory: In languages with manual memory management, failing to deallocate memory when it’s no longer needed.
- Losing references to objects: In garbage-collected languages, keeping unnecessary references to objects, preventing them from being collected.
- Circular references: Objects referencing each other in a cycle, making it difficult for some garbage collectors to identify them as unreachable.
- Improper use of static variables: Static variables that accumulate data over time without being cleared.
- Resource leaks: Failing to close or release system resources like file handles, database connections, or network sockets.
6.2. Preventing Memory Leaks
To prevent memory leaks, consider the following best practices:
- Use smart pointers (C++): Utilize smart pointers like
std::unique_ptr
andstd::shared_ptr
to manage memory automatically. - Implement proper resource management: Use RAII (Resource Acquisition Is Initialization) principles to ensure resources are properly released.
- Avoid unnecessary object retention: Remove references to objects when they’re no longer needed, allowing them to be garbage collected.
- Use weak references: In languages that support them, use weak references to break circular dependencies.
- Regularly profile your code: Use memory profiling tools to identify potential leaks and memory usage patterns.
- Implement proper error handling: Ensure resources are released even in the case of exceptions or errors.
- Use static analysis tools: Employ tools that can detect potential memory leaks through static code analysis.
6.3. Example: Preventing a Memory Leak in C++
Here’s an example of how to prevent a memory leak using smart pointers in C++:
#include <memory>
class Resource {
public:
Resource() { /* Initialize resource */ }
~Resource() { /* Clean up resource */ }
void use() { /* Use the resource */ }
};
void potentialLeak() {
// Potential memory leak:
// Resource* res = new Resource();
// res->use();
// What if we forget to delete res?
// Better approach using smart pointer:
std::unique_ptr<Resource> res = std::make_unique<Resource>();
res->use();
// No need to manually delete; memory will be automatically freed
}
7. Memory Management in Different Programming Languages
Different programming languages handle memory management in various ways. Let’s explore how memory is managed in some popular languages:
7.1. C and C++
C and C++ provide manual memory management, giving developers full control over allocation and deallocation:
- Use
malloc()
/free()
in C ornew
/delete
in C++ for dynamic memory allocation/deallocation - Require careful management to prevent memory leaks and dangling pointers
- C++ offers additional tools like smart pointers for safer memory management
7.2. Java
Java uses automatic memory management with garbage collection:
- Objects are allocated on the heap using the
new
keyword - The garbage collector automatically frees memory when objects are no longer reachable
- Developers don’t need to explicitly deallocate memory
7.3. Python
Python also employs automatic memory management:
- Uses reference counting as the primary method for garbage collection
- Also includes a cyclic garbage collector to handle circular references
- Developers rarely need to worry about memory management directly
7.4. JavaScript
JavaScript, typically run in browsers or Node.js environments, uses automatic memory management:
- Employs a mark-and-sweep garbage collector
- Automatically allocates memory when objects are created and frees it when they’re no longer used
- Developers should be aware of potential memory leaks, especially in long-running applications or when dealing with closures
7.5. Rust
Rust takes a unique approach to memory management:
- Uses a system of ownership, borrowing, and lifetimes to manage memory safely without a garbage collector
- The compiler enforces rules that prevent common memory-related errors at compile-time
- Provides fine-grained control over memory without sacrificing safety
8. Best Practices for Efficient Memory Management
Regardless of the programming language you’re using, following these best practices can help ensure efficient memory management:
- Understand your language’s memory model: Familiarize yourself with how memory is managed in your chosen language.
- Minimize allocations: Avoid unnecessary object creation and prefer stack allocation when possible.
- Reuse objects: Implement object pooling for frequently created and destroyed objects.
- Release resources promptly: Close files, database connections, and other system resources as soon as you’re done with them.
- Use appropriate data structures: Choose data structures that are memory-efficient for your specific use case.
- Avoid premature optimization: Focus on writing clear, correct code first, then optimize if profiling indicates a need.
- Profile your application: Use memory profiling tools to identify memory usage patterns and potential issues.
- Consider memory fragmentation: Be aware of how your allocations and deallocations might lead to memory fragmentation.
- Use smart pointers in C++: Leverage
std::unique_ptr
,std::shared_ptr
, andstd::weak_ptr
for safer memory management. - Implement proper error handling: Ensure resources are released even in the case of exceptions or errors.
9. Memory Management in Technical Interviews
Understanding memory management is crucial for technical interviews, especially when applying to major tech companies. Here are some key areas to focus on:
9.1. Common Interview Questions
- Explain the difference between stack and heap memory.
- How does garbage collection work?
- What is a memory leak, and how can it be prevented?
- Describe the concept of memory fragmentation.
- How would you implement a memory pool?
- Explain the concept of virtual memory.
- How does reference counting work in memory management?
- What are the pros and cons of manual memory management vs. garbage collection?
9.2. Coding Challenges
Be prepared to tackle coding challenges that involve memory management, such as:
- Implementing a custom memory allocator
- Detecting and fixing memory leaks in a given piece of code
- Optimizing memory usage in a data structure or algorithm
- Implementing a simple garbage collector
9.3. Language-Specific Knowledge
Depending on the position and company, you may need to demonstrate in-depth knowledge of memory management in specific languages:
- C/C++: Manual memory management, smart pointers, RAII
- Java: Understanding the JVM, garbage collection algorithms, memory profiling
- Python: Reference counting, cyclic garbage collection
- Rust: Ownership, borrowing, and lifetimes
9.4. System Design Considerations
In system design interviews, be prepared to discuss how memory management affects system architecture and performance:
- Caching strategies and their impact on memory usage
- Memory considerations in distributed systems
- Balancing memory usage and performance in large-scale applications
- Strategies for handling out-of-memory situations in production environments
10. Conclusion
Memory management is a fundamental concept in computer programming that plays a crucial role in developing efficient, reliable, and scalable software. Whether you’re working with low-level languages like C and C++ or high-level languages with automatic garbage collection, understanding memory management principles is essential for writing high-quality code and optimizing performance.
By mastering concepts such as stack and heap memory, allocation and deallocation, garbage collection, and memory leak prevention, you’ll be better equipped to tackle complex programming challenges and excel in technical interviews. Remember to apply best practices for efficient memory management in your projects and continue exploring this topic as you advance in your programming career.
As you prepare for technical interviews or work on personal projects, keep in mind that memory management is not just about avoiding errors—it’s about writing code that is efficient, maintainable, and scalable. With a solid understanding of memory management concepts and techniques, you’ll be well-prepared to tackle a wide range of programming challenges and contribute to the development of robust software systems.