Mastering Mathematical and Logic Puzzles: A Programmer’s Guide to Problem-Solving
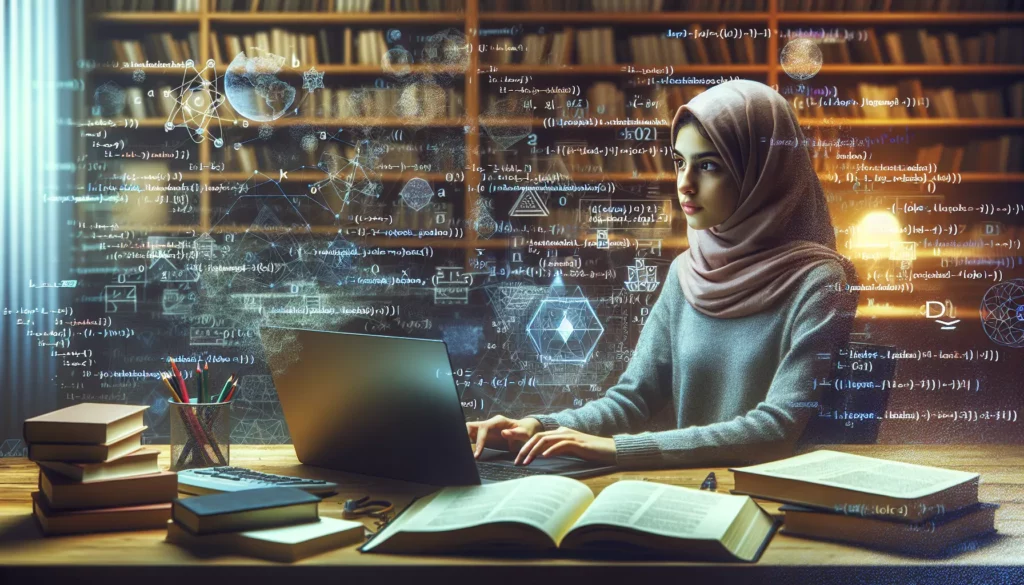
In the world of coding and software development, the ability to solve complex problems efficiently is a highly sought-after skill. Whether you’re a beginner just starting your journey or an experienced programmer preparing for technical interviews at top tech companies, honing your problem-solving abilities is crucial. One of the best ways to sharpen these skills is by tackling mathematical and logic puzzles. In this comprehensive guide, we’ll explore various approaches to solving these puzzles, discuss their relevance to programming, and provide practical strategies to improve your problem-solving prowess.
Why Mathematical and Logic Puzzles Matter for Programmers
Before diving into specific approaches, it’s essential to understand why mathematical and logic puzzles are valuable for programmers:
- Algorithmic Thinking: Puzzles often require you to develop step-by-step solutions, mirroring the process of creating algorithms.
- Pattern Recognition: Many puzzles involve identifying and leveraging patterns, a crucial skill in programming and data analysis.
- Optimization: Solving puzzles efficiently often requires optimizing your approach, similar to writing efficient code.
- Abstract Reasoning: Puzzles help develop the ability to think abstractly, which is essential when dealing with complex programming concepts.
- Interview Preparation: Many technical interviews, especially at FAANG companies, include puzzle-like questions to assess problem-solving skills.
Common Types of Mathematical and Logic Puzzles
Before we delve into specific approaches, let’s familiarize ourselves with some common types of puzzles you might encounter:
- Number Sequence Puzzles: These involve identifying patterns in a series of numbers and predicting the next number(s) in the sequence.
- Word Problems: These puzzles present a scenario in words that needs to be translated into mathematical equations or logical statements.
- Geometry Puzzles: These involve spatial reasoning and often require visualizing or manipulating shapes.
- Logic Grid Puzzles: Also known as “Einstein’s Puzzles,” these require deductive reasoning to match items in different categories based on given clues.
- Probability Puzzles: These involve calculating the likelihood of certain events occurring.
- Cryptarithmetic Puzzles: These are math problems where letters represent digits, and you need to determine which letter corresponds to which digit.
- Lateral Thinking Puzzles: These often have counterintuitive solutions and require thinking “outside the box.”
General Approaches to Solving Mathematical and Logic Puzzles
Now that we’ve covered the types of puzzles, let’s explore some general approaches that can be applied to most mathematical and logic puzzles:
1. Understand the Problem
Before attempting to solve any puzzle, it’s crucial to fully understand what’s being asked. This step involves:
- Reading the problem statement carefully, multiple times if necessary.
- Identifying the given information and the unknown elements you need to find.
- Rephrasing the problem in your own words to ensure comprehension.
- Recognizing any constraints or conditions mentioned in the problem.
2. Break Down the Problem
Complex puzzles can often be solved by breaking them down into smaller, more manageable sub-problems. This approach, known as “divide and conquer” in programming, involves:
- Identifying the main components or steps required to solve the puzzle.
- Tackling each sub-problem individually.
- Combining the solutions to sub-problems to solve the overall puzzle.
3. Visualize the Problem
Many puzzles become clearer when represented visually. Visualization techniques include:
- Drawing diagrams or sketches to represent the problem scenario.
- Creating tables or charts to organize given information.
- Using graphs for number sequence or probability puzzles.
4. Look for Patterns
Pattern recognition is a crucial skill in both puzzle-solving and programming. To identify patterns:
- Examine the given information for recurring elements or relationships.
- Consider mathematical sequences, geometric progressions, or logical rules that might apply.
- Test potential patterns with additional examples or data points.
5. Use Logical Reasoning
Logical reasoning is the foundation of problem-solving. Key logical approaches include:
- Deductive Reasoning: Drawing conclusions based on given premises.
- Inductive Reasoning: Making generalizations based on specific observations.
- Abductive Reasoning: Forming the most likely explanation based on limited information.
6. Apply Relevant Formulas or Theorems
For mathematical puzzles, having a solid grasp of fundamental formulas and theorems can be invaluable. This involves:
- Identifying which mathematical concepts are relevant to the puzzle.
- Recalling and correctly applying appropriate formulas or theorems.
- Adapting known formulas to fit the specific problem context.
7. Work Backwards
Sometimes, starting from the desired result and working backwards can lead to a solution. This approach includes:
- Identifying the end goal or desired outcome.
- Determining the steps or conditions necessary to achieve that outcome.
- Reverse-engineering the solution by following these steps in reverse order.
8. Use Process of Elimination
When faced with multiple possible solutions, systematically eliminating incorrect options can be effective. This method involves:
- Listing all possible solutions or scenarios.
- Testing each option against the given information and constraints.
- Eliminating options that don’t meet all criteria until the correct solution remains.
9. Make Educated Guesses
Sometimes, making an informed guess and then verifying it can lead to the solution. This approach includes:
- Using available information to make a logical guess.
- Testing the guess against the problem constraints.
- Refining the guess based on the results and repeating the process.
10. Think Creatively
Some puzzles require thinking outside conventional patterns. Creative problem-solving involves:
- Considering unconventional approaches or interpretations of the problem.
- Combining different concepts or ideas in novel ways.
- Challenging assumptions about the problem or potential solutions.
Specific Strategies for Different Types of Puzzles
While the general approaches above can be applied to most puzzles, certain types of puzzles benefit from specific strategies:
Number Sequence Puzzles
- Look for arithmetic or geometric progressions.
- Consider operations between consecutive terms (addition, subtraction, multiplication, division).
- Check for alternating patterns or cycles.
- Examine the differences between consecutive terms for patterns.
Word Problems
- Identify key information and translate it into mathematical notation.
- Define variables for unknown quantities.
- Set up equations based on the relationships described in the problem.
- Solve the equations using algebraic methods.
Geometry Puzzles
- Draw accurate diagrams to represent the problem.
- Label known measurements and angles.
- Use geometric theorems (e.g., Pythagorean theorem, angle sum properties).
- Consider symmetry and congruence in shapes.
Logic Grid Puzzles
- Create a grid with all possible combinations of categories.
- Start with the most specific clues and mark them on the grid.
- Use elimination to rule out impossible combinations.
- Make deductions based on the information filled in and repeat the process.
Probability Puzzles
- Identify the total number of possible outcomes.
- Determine the number of favorable outcomes.
- Use the basic probability formula: P(event) = (favorable outcomes) / (total outcomes).
- Consider whether events are independent or dependent.
Cryptarithmetic Puzzles
- Identify the possible range of values for each letter (usually 0-9).
- Look for constraints (e.g., leading digits can’t be zero).
- Use logical deductions to narrow down possibilities.
- Consider using a systematic trial-and-error approach or backtracking algorithm.
Lateral Thinking Puzzles
- Question your assumptions about the problem.
- Consider multiple interpretations of the given information.
- Think about unconventional uses for objects or concepts mentioned.
- Try to approach the problem from different perspectives.
Implementing Puzzle-Solving Strategies in Code
As a programmer, it’s valuable to translate puzzle-solving strategies into code. This practice not only reinforces your problem-solving skills but also helps you develop more efficient algorithms. Let’s look at some examples of how to implement puzzle-solving approaches in code:
1. Recursive Problem-Solving
Recursion is a powerful technique for solving problems that can be broken down into smaller, similar sub-problems. Here’s an example of using recursion to solve the Fibonacci sequence puzzle:
def fibonacci(n):
if n <= 1:
return n
else:
return fibonacci(n-1) + fibonacci(n-2)
# Example usage
print(fibonacci(10)) # Output: 55
2. Dynamic Programming
Dynamic programming is an optimization technique that solves complex problems by breaking them down into simpler subproblems. It’s particularly useful for puzzles with overlapping subproblems. Here’s an example of using dynamic programming to solve the coin change problem:
def coin_change(coins, amount):
dp = [float('inf')] * (amount + 1)
dp[0] = 0
for coin in coins:
for x in range(coin, amount + 1):
dp[x] = min(dp[x], dp[x - coin] + 1)
return dp[amount] if dp[amount] != float('inf') else -1
# Example usage
coins = [1, 2, 5]
amount = 11
print(coin_change(coins, amount)) # Output: 3 (5 + 5 + 1)
3. Backtracking
Backtracking is a general algorithm for finding all (or some) solutions to computational problems, particularly constraint satisfaction problems. It’s useful for puzzles like Sudoku or N-Queens. Here’s a simple example of using backtracking to solve a subset sum problem:
def subset_sum(numbers, target, partial=None):
if partial is None:
partial = []
s = sum(partial)
if s == target:
print(f"Sum({partial})={target}")
if s >= target:
return
for i in range(len(numbers)):
n = numbers[i]
remaining = numbers[i+1:]
subset_sum(remaining, target, partial + [n])
# Example usage
numbers = [3, 34, 4, 12, 5, 2]
target = 9
subset_sum(numbers, target)
4. Graph Algorithms
Many puzzles can be represented as graph problems. Knowledge of graph algorithms like DFS (Depth-First Search), BFS (Breadth-First Search), and Dijkstra’s algorithm can be invaluable. Here’s an example of using DFS to solve a maze puzzle:
def solve_maze(maze, start, end):
def dfs(x, y, path):
if (x, y) == end:
return path
for dx, dy in [(0, 1), (1, 0), (0, -1), (-1, 0)]: # right, down, left, up
nx, ny = x + dx, y + dy
if 0 <= nx < len(maze) and 0 <= ny < len(maze[0]) and maze[nx][ny] == 0 and (nx, ny) not in path:
result = dfs(nx, ny, path + [(nx, ny)])
if result:
return result
return None
return dfs(start[0], start[1], [start])
# Example usage
maze = [
[0, 0, 0, 0, 0],
[1, 1, 0, 1, 0],
[0, 0, 0, 0, 0],
[0, 1, 1, 1, 0],
[0, 0, 0, 1, 0]
]
start = (0, 0)
end = (4, 4)
solution = solve_maze(maze, start, end)
print(solution)
Improving Your Puzzle-Solving Skills
Becoming proficient at solving mathematical and logic puzzles takes practice and dedication. Here are some strategies to improve your skills:
- Practice Regularly: Set aside time each day to solve puzzles. Websites like Project Euler, LeetCode, and HackerRank offer a wide variety of programming puzzles and challenges.
- Start Simple: Begin with easier puzzles and gradually increase the difficulty as you improve.
- Time Yourself: Practice solving puzzles under time constraints to simulate interview conditions.
- Analyze Solutions: After solving a puzzle, study alternative solutions to learn different approaches.
- Join Coding Communities: Participate in online forums or local coding meetups to discuss problem-solving strategies with others.
- Read Puzzle Books: Classic books like “How to Solve It” by George Pólya offer valuable insights into problem-solving techniques.
- Teach Others: Explaining puzzle solutions to others can deepen your understanding and reveal gaps in your knowledge.
- Apply Puzzles to Real-World Problems: Look for opportunities to apply puzzle-solving techniques to actual programming tasks or projects.
Conclusion
Mastering mathematical and logic puzzles is an invaluable skill for programmers, particularly those aiming to excel in technical interviews at top tech companies. By understanding various puzzle types, applying general problem-solving approaches, and implementing specific strategies, you can significantly enhance your ability to tackle complex coding challenges.
Remember that improvement comes with consistent practice and a willingness to learn from each puzzle you encounter. As you continue to hone your skills, you’ll find that the problem-solving techniques you develop will not only help you in interviews but also make you a more effective and efficient programmer in your day-to-day work.
Whether you’re just starting your coding journey or preparing for interviews at FAANG companies, embracing the challenge of mathematical and logic puzzles will undoubtedly contribute to your growth as a programmer. So, dive in, start solving, and watch your problem-solving abilities soar to new heights!