How to Ace Coding Interviews for Front-End Developer Roles: A Comprehensive Guide
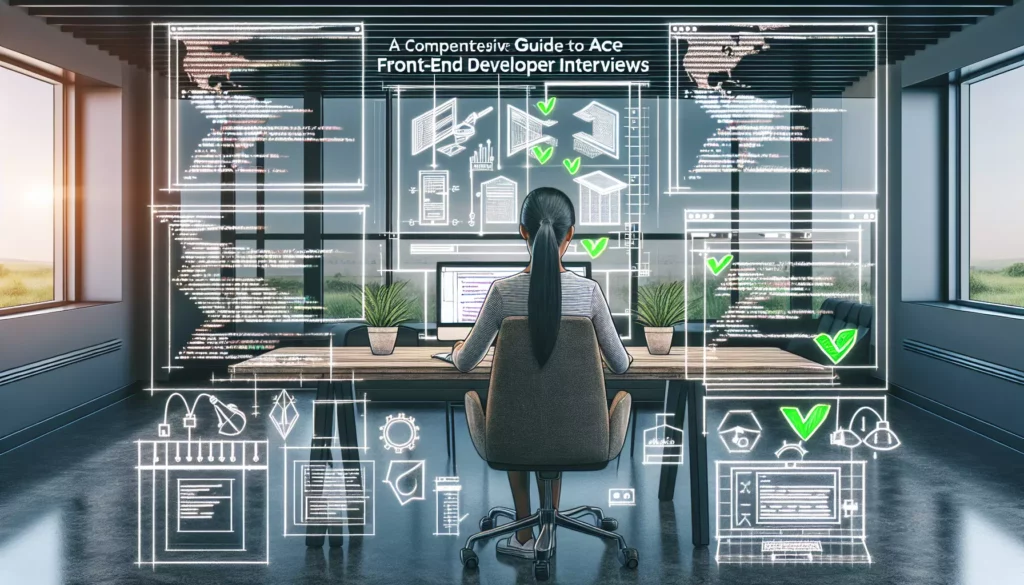
Are you a front-end developer looking to land your dream job at a top tech company? Coding interviews can be intimidating, but with the right preparation and mindset, you can ace them and showcase your skills to potential employers. In this comprehensive guide, we’ll walk you through everything you need to know to succeed in front-end developer coding interviews, from essential concepts to practice strategies and tips for interview day.
Table of Contents
- Understanding the Interview Process
- Essential Front-End Concepts to Master
- Data Structures and Algorithms for Front-End Developers
- Common Front-End Coding Challenges
- Front-End System Design Questions
- Behavioral Questions and Soft Skills
- Effective Practice Strategies
- Interview Day Tips and Techniques
- Additional Resources for Interview Preparation
- Conclusion
1. Understanding the Interview Process
Before diving into the technical aspects, it’s crucial to understand the typical interview process for front-end developer roles:
- Initial Screening: This often involves a phone call or online assessment to gauge your basic qualifications and interest in the role.
- Technical Phone Screen: A more in-depth discussion of your technical skills, often including some coding questions or small challenges.
- Take-Home Assignment: Some companies may give you a project to complete on your own time, showcasing your coding abilities and problem-solving skills.
- On-Site Interviews: This typically involves multiple rounds of interviews, including:
- Coding interviews focused on algorithms and data structures
- Front-end specific coding challenges
- System design discussions
- Behavioral interviews
Understanding this process will help you prepare effectively for each stage and manage your expectations throughout the interview journey.
2. Essential Front-End Concepts to Master
To excel in front-end developer interviews, you need a strong foundation in core concepts. Make sure you’re comfortable with the following areas:
HTML
- Semantic HTML
- Accessibility best practices
- HTML5 features and APIs
CSS
- Box model and layout techniques
- Flexbox and Grid
- Responsive design and media queries
- CSS preprocessors (e.g., Sass, Less)
- CSS-in-JS solutions
JavaScript
- ES6+ features and syntax
- Closures and scope
- Promises and async/await
- Event handling and DOM manipulation
- Functional programming concepts
Front-End Frameworks
- React, Vue, or Angular (depending on the job requirements)
- State management (e.g., Redux, Vuex)
- Component lifecycle and hooks
- Virtual DOM and rendering optimization
Build Tools and Module Bundlers
- Webpack, Rollup, or Parcel
- Babel for transpilation
- npm or Yarn for package management
Testing
- Unit testing with Jest or Mocha
- Integration testing with tools like React Testing Library
- End-to-end testing with Cypress or Selenium
Performance Optimization
- Lazy loading and code splitting
- Caching strategies
- Minimizing reflows and repaints
- Browser rendering process and critical rendering path
While you may not be tested on all of these concepts in every interview, having a solid understanding of these areas will give you the confidence to tackle a wide range of front-end challenges.
3. Data Structures and Algorithms for Front-End Developers
Although front-end development often focuses more on UI and user experience, many companies still include algorithm and data structure questions in their interviews. Here are some key areas to focus on:
Data Structures
- Arrays and Objects
- Linked Lists
- Stacks and Queues
- Trees (especially DOM tree manipulation)
- Graphs (for more advanced positions)
- Hash Tables
Algorithms
- Array manipulation (sorting, searching, filtering)
- String manipulation
- Tree traversal (particularly relevant for DOM manipulation)
- Basic graph algorithms (for more advanced positions)
- Dynamic Programming (understanding the concept, even if not directly applied)
Big O Notation
Understanding time and space complexity is crucial for optimizing your code and discussing trade-offs in your solutions.
Example: Implementing a Debounce Function
Here’s an example of a common front-end algorithm question: implementing a debounce function. This is useful for optimizing event handlers that might be called frequently, such as resize or scroll events.
function debounce(func, delay) {
let timeoutId;
return function (...args) {
clearTimeout(timeoutId);
timeoutId = setTimeout(() => {
func.apply(this, args);
}, delay);
};
}
// Usage
const debouncedResize = debounce(() => {
console.log('Resized!');
}, 300);
window.addEventListener('resize', debouncedResize);
This implementation showcases closures, timers, and practical application of a common front-end optimization technique.
4. Common Front-End Coding Challenges
Front-end coding interviews often include challenges specific to web development. Here are some common types of questions you might encounter:
DOM Manipulation
You might be asked to create, modify, or traverse the DOM using vanilla JavaScript. For example:
// Create a function that generates a table from an array of objects
function generateTable(data) {
const table = document.createElement('table');
const thead = document.createElement('thead');
const tbody = document.createElement('tbody');
// Create header row
const headerRow = document.createElement('tr');
Object.keys(data[0]).forEach(key => {
const th = document.createElement('th');
th.textContent = key;
headerRow.appendChild(th);
});
thead.appendChild(headerRow);
// Create data rows
data.forEach(item => {
const row = document.createElement('tr');
Object.values(item).forEach(value => {
const td = document.createElement('td');
td.textContent = value;
row.appendChild(td);
});
tbody.appendChild(row);
});
table.appendChild(thead);
table.appendChild(tbody);
return table;
}
Event Handling
You might need to implement custom event listeners or create interactive components. For instance:
// Implement a custom dropdown component
class Dropdown {
constructor(container) {
this.container = container;
this.trigger = container.querySelector('.dropdown-trigger');
this.content = container.querySelector('.dropdown-content');
this.isOpen = false;
this.trigger.addEventListener('click', this.toggle.bind(this));
document.addEventListener('click', this.handleOutsideClick.bind(this));
}
toggle() {
this.isOpen = !this.isOpen;
this.content.style.display = this.isOpen ? 'block' : 'none';
}
handleOutsideClick(event) {
if (!this.container.contains(event.target) && this.isOpen) {
this.toggle();
}
}
}
// Usage
const dropdownElement = document.querySelector('.dropdown');
const dropdown = new Dropdown(dropdownElement);
Asynchronous Programming
You may be asked to work with APIs, handle promises, or implement async/await. Here’s an example:
// Implement a function that fetches user data and their todos
async function getUserWithTodos(userId) {
try {
const [userResponse, todosResponse] = await Promise.all([
fetch(`https://jsonplaceholder.typicode.com/users/${userId}`),
fetch(`https://jsonplaceholder.typicode.com/todos?userId=${userId}`)
]);
if (!userResponse.ok || !todosResponse.ok) {
throw new Error('Failed to fetch data');
}
const userData = await userResponse.json();
const todos = await todosResponse.json();
return {
user: userData,
todos: todos
};
} catch (error) {
console.error('Error fetching user data:', error);
throw error;
}
}
// Usage
getUserWithTodos(1)
.then(data => console.log(data))
.catch(error => console.error(error));
State Management
You might be asked to implement a simple state management solution or discuss how you would manage state in a complex application. Here’s a basic example using the observer pattern:
class Store {
constructor(initialState) {
this.state = initialState;
this.listeners = [];
}
getState() {
return this.state;
}
setState(newState) {
this.state = { ...this.state, ...newState };
this.notify();
}
subscribe(listener) {
this.listeners.push(listener);
return () => {
this.listeners = this.listeners.filter(l => l !== listener);
};
}
notify() {
this.listeners.forEach(listener => listener(this.state));
}
}
// Usage
const store = new Store({ count: 0 });
const unsubscribe = store.subscribe(state => {
console.log('State updated:', state);
});
store.setState({ count: 1 });
store.setState({ count: 2 });
unsubscribe();
Practicing these types of challenges will help you become more comfortable with front-end specific problems and showcase your skills during interviews.
5. Front-End System Design Questions
System design questions for front-end roles often focus on architecting scalable and maintainable user interfaces. Here are some topics you should be prepared to discuss:
Component Architecture
- Designing reusable and modular components
- Deciding between class components and functional components with hooks
- Implementing component composition and inheritance
State Management
- Choosing between local state, context API, and global state management solutions (e.g., Redux, MobX)
- Implementing efficient state updates and avoiding unnecessary re-renders
- Handling shared state between components
Performance Optimization
- Implementing code splitting and lazy loading
- Optimizing rendering performance (e.g., using memoization, virtualization for long lists)
- Implementing efficient data fetching and caching strategies
Scalability and Maintainability
- Designing a folder structure for a large-scale application
- Implementing a design system and component library
- Setting up a robust testing strategy
Example: Designing a News Feed Application
Here’s an example of how you might approach a front-end system design question:
Question: Design a news feed application similar to Facebook or Twitter.
Answer:
- Component Structure:
- App (root component)
- Header (with navigation and search)
- NewsFeed (container for posts)
- Post (individual post component)
- CommentSection (for each post)
- UserProfile (for viewing and editing user information)
- State Management:
- Use Redux for global state management
- Store user data, posts, and authentication state in Redux
- Use local state for UI-specific states (e.g., comment input fields)
- Data Fetching:
- Implement infinite scrolling for the news feed
- Use a library like React Query for efficient data fetching and caching
- Implement optimistic updates for actions like likes and comments
- Performance Optimization:
- Implement virtualization for the news feed to handle large numbers of posts
- Use React.memo and useMemo for expensive computations
- Implement code splitting for different routes (e.g., news feed, user profile)
- Scalability and Maintainability:
- Implement a design system with reusable UI components
- Use TypeScript for improved type safety and developer experience
- Set up unit tests for components and integration tests for key user flows
Being able to articulate your thought process and make informed decisions about architecture and design will impress interviewers and demonstrate your ability to handle complex front-end challenges.
6. Behavioral Questions and Soft Skills
While technical skills are crucial, don’t underestimate the importance of behavioral questions and soft skills in front-end developer interviews. Here are some areas to focus on:
Teamwork and Collaboration
- Be prepared to discuss your experience working in cross-functional teams
- Highlight your ability to communicate technical concepts to non-technical stakeholders
- Describe how you handle conflicts or disagreements within a team
Problem-Solving and Decision Making
- Prepare examples of challenging problems you’ve solved in previous projects
- Discuss your approach to making technical decisions and weighing trade-offs
- Show how you stay updated with the latest front-end technologies and best practices
Adaptability and Learning
- Highlight your ability to quickly learn new technologies or frameworks
- Discuss how you’ve adapted to changing project requirements or technologies
- Show your enthusiasm for continuous learning and improvement
Leadership and Initiative
- Describe situations where you’ve taken the lead on a project or feature
- Highlight any mentoring or knowledge-sharing activities you’ve been involved in
- Discuss how you’ve contributed to improving development processes or team productivity
Example Behavioral Questions
- “Tell me about a time when you had to work on a project with a tight deadline. How did you manage your time and ensure quality?”
- “Describe a situation where you had to explain a complex technical concept to a non-technical stakeholder. How did you approach this?”
- “Can you give an example of a time when you disagreed with a team member’s approach to solving a problem? How did you resolve the situation?”
- “Tell me about a project where you had to learn a new technology or framework quickly. How did you approach the learning process?”
- “Describe a situation where you identified and implemented an improvement to your team’s development process. What was the impact?”
When answering behavioral questions, use the STAR method (Situation, Task, Action, Result) to structure your responses and provide concrete examples from your experience.
7. Effective Practice Strategies
Consistent and focused practice is key to succeeding in front-end developer interviews. Here are some strategies to help you prepare effectively:
Coding Practice
- Solve coding challenges on platforms like LeetCode, HackerRank, or Codewars, focusing on JavaScript-specific problems
- Implement common front-end components and features from scratch (e.g., carousel, modal, autocomplete)
- Contribute to open-source projects to gain real-world experience and improve your code quality
Mock Interviews
- Practice with friends, colleagues, or mentors who can give you feedback
- Use platforms like Pramp or interviewing.io for realistic mock interview experiences
- Record yourself explaining solutions to identify areas for improvement in your communication
Project-Based Learning
- Build personal projects that showcase your front-end skills and problem-solving abilities
- Implement features using different frameworks or libraries to broaden your experience
- Document your projects and be prepared to discuss your design decisions and challenges faced
Stay Updated
- Follow front-end development blogs, podcasts, and YouTube channels
- Attend web development conferences or local meetups (virtual or in-person)
- Experiment with new tools and technologies to stay current with industry trends
Create a Study Plan
Develop a structured study plan that covers all aspects of front-end development. Here’s an example weekly plan:
- Monday: JavaScript fundamentals and ES6+ features
- Tuesday: DOM manipulation and browser APIs
- Wednesday: CSS layout techniques and responsive design
- Thursday: React concepts and state management
- Friday: Data structures and algorithms
- Saturday: System design and architecture
- Sunday: Mock interviews and project work
Adjust this plan based on your strengths and weaknesses, and be consistent in your practice.
8. Interview Day Tips and Techniques
When the big day arrives, keep these tips in mind to perform at your best:
Before the Interview
- Get a good night’s sleep and eat a healthy meal
- Review your projects and practice explaining your thought process
- Prepare questions to ask your interviewers about the role and company
- Test your technical setup if it’s a remote interview
During the Interview
- Listen carefully to the questions and ask for clarification if needed
- Think out loud and communicate your thought process
- Start with a simple solution and then optimize if time allows
- If you’re stuck, don’t be afraid to ask for hints
- Be open to feedback and willing to iterate on your solutions
Coding Interview Techniques
- Begin by clarifying the requirements and constraints
- Discuss your approach before starting to code
- Write clean, readable code with meaningful variable and function names
- Consider edge cases and handle errors appropriately
- Test your code with sample inputs and explain your testing strategy
System Design Discussion Tips
- Start by gathering requirements and defining the scope
- Break down the problem into smaller components
- Discuss trade-offs between different approaches
- Consider scalability, performance, and maintainability
- Be prepared to dive deeper into specific areas if asked
Behavioral Interview Strategies
- Use the STAR method to structure your answers
- Provide specific examples from your experience
- Be honest about your strengths and areas for improvement
- Show enthusiasm for the role and company
- Ask thoughtful questions about the team and projects
Remember, interviews are also an opportunity for you to evaluate the company and team. Stay calm, be yourself, and showcase your passion for front-end development.
9. Additional Resources for Interview Preparation
To further enhance your preparation, consider these additional resources:
Books
- “Cracking the Coding Interview” by Gayle Laakmann McDowell
- “Front-End Developer Handbook” by Cody Lindley
- “You Don’t Know JS” series by Kyle Simpson
Online Courses
- Frontend Masters courses on JavaScript, React, and system design
- Udemy courses on data structures and algorithms in JavaScript
- Coursera’s web development specializations
Websites and Blogs
- MDN Web Docs for comprehensive web development documentation
- CSS-Tricks for in-depth CSS tutorials and techniques
- React documentation for official guides and best practices
YouTube Channels
- Traversy Media for practical web development tutorials
- Fun Fun Function for in-depth JavaScript concepts
- Google Chrome Developers for the latest web technologies and best practices
GitHub Repositories
- 30 Seconds of Code for short JavaScript code snippets
- You Don’t Know JS for in-depth JavaScript knowledge
- Front-End Interview Handbook for curated front-end interview questions and answers
Coding Challenge Platforms
- LeetCode for algorithm and data structure practice
- HackerRank for a variety of coding challenges
- Codewars for JavaScript-specific coding katas
Leverage these resources to supplement your learning and practice, but remember that hands-on coding and project work are essential for internalizing concepts and improving your skills.
10. Conclusion
Preparing for front-end developer coding interviews can be challenging, but with dedication and the right approach, you can significantly improve your chances of success. Remember these key takeaways:
- Master the fundamental concepts of HTML, CSS, and JavaScript
- Practice data structures and algorithms, focusing on their application in front-end development
- Gain experience with popular front-end frameworks and libraries
- Develop your problem-solving skills through regular coding practice and projects
- Improve your system design and architecture knowledge for scalable front-end applications
- Hone your communication skills and prepare for behavioral questions
- Stay updated with the latest front-end trends and best practices
- Practice consistently and create a structured study plan
- Leverage additional resources to supplement your learning
Above all, approach your interviews with confidence and enthusiasm. Your passion for front-end development, combined with thorough preparation, will shine through and impress potential employers. Good luck with your interviews, and may you land your dream front-end developer role!