Understanding Object-Oriented Design Principles for Interviews: A Comprehensive Guide
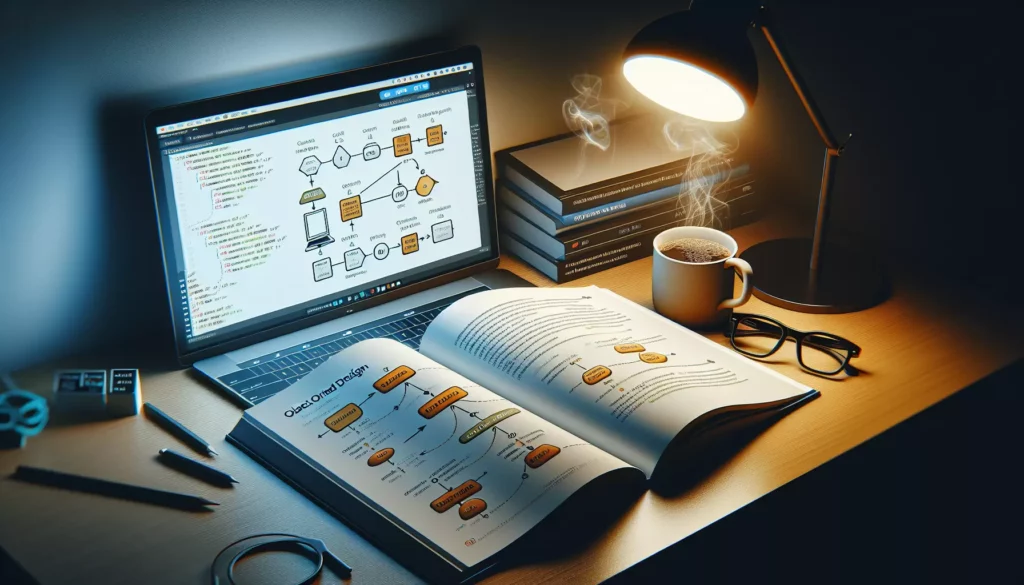
In the world of software development, object-oriented programming (OOP) has become a cornerstone of modern application design. As you prepare for technical interviews, especially with major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), having a solid grasp of object-oriented design principles is crucial. This comprehensive guide will walk you through the fundamental concepts and best practices of OOP, helping you ace your next interview and become a more proficient programmer.
1. Introduction to Object-Oriented Programming
Object-Oriented Programming is a programming paradigm that organizes code into objects, which are instances of classes. This approach allows for better code organization, reusability, and maintainability. Before diving into the design principles, let’s review the four main pillars of OOP:
- Encapsulation: Bundling data and methods that operate on that data within a single unit (class).
- Inheritance: Creating new classes based on existing classes, allowing for code reuse and establishing a hierarchical relationship between classes.
- Polymorphism: The ability of objects to take on multiple forms and behave differently based on the context.
- Abstraction: Hiding complex implementation details and exposing only the necessary features of an object.
Understanding these concepts is essential for mastering object-oriented design principles and excelling in technical interviews.
2. SOLID Principles
The SOLID principles are a set of five design principles that help developers create more maintainable, flexible, and scalable software. These principles were introduced by Robert C. Martin and have become a fundamental part of object-oriented design. Let’s explore each principle in detail:
2.1 Single Responsibility Principle (SRP)
The Single Responsibility Principle states that a class should have only one reason to change. In other words, a class should have a single, well-defined responsibility.
Example of violating SRP:
class User {
public void createUser(String name, String email) {
// Code to create user
}
public void sendEmail(String message) {
// Code to send email
}
public void generateReport() {
// Code to generate report
}
}
In this example, the User class has multiple responsibilities: creating users, sending emails, and generating reports. To adhere to SRP, we should separate these responsibilities into different classes:
class User {
public void createUser(String name, String email) {
// Code to create user
}
}
class EmailService {
public void sendEmail(String message) {
// Code to send email
}
}
class ReportGenerator {
public void generateReport() {
// Code to generate report
}
}
2.2 Open-Closed Principle (OCP)
The Open-Closed Principle states that software entities (classes, modules, functions, etc.) should be open for extension but closed for modification. This means you should be able to extend a class’s behavior without modifying its existing code.
Example of violating OCP:
class Rectangle {
public double width;
public double height;
}
class AreaCalculator {
public double calculateArea(Rectangle rectangle) {
return rectangle.width * rectangle.height;
}
}
If we want to add support for calculating the area of a circle, we would need to modify the AreaCalculator class. Instead, we can use polymorphism to adhere to OCP:
interface Shape {
double calculateArea();
}
class Rectangle implements Shape {
private double width;
private double height;
public double calculateArea() {
return width * height;
}
}
class Circle implements Shape {
private double radius;
public double calculateArea() {
return Math.PI * radius * radius;
}
}
class AreaCalculator {
public double calculateArea(Shape shape) {
return shape.calculateArea();
}
}
2.3 Liskov Substitution Principle (LSP)
The Liskov Substitution Principle states that objects of a superclass should be replaceable with objects of its subclasses without affecting the correctness of the program. In other words, derived classes must be substitutable for their base classes.
Example of violating LSP:
class Rectangle {
protected int width;
protected int height;
public void setWidth(int width) {
this.width = width;
}
public void setHeight(int height) {
this.height = height;
}
public int getArea() {
return width * height;
}
}
class Square extends Rectangle {
@Override
public void setWidth(int width) {
super.setWidth(width);
super.setHeight(width);
}
@Override
public void setHeight(int height) {
super.setWidth(height);
super.setHeight(height);
}
}
In this example, the Square class violates LSP because it changes the behavior of the setWidth and setHeight methods. To fix this, we can use a different approach:
interface Shape {
int getArea();
}
class Rectangle implements Shape {
protected int width;
protected int height;
public Rectangle(int width, int height) {
this.width = width;
this.height = height;
}
public int getArea() {
return width * height;
}
}
class Square implements Shape {
private int side;
public Square(int side) {
this.side = side;
}
public int getArea() {
return side * side;
}
}
2.4 Interface Segregation Principle (ISP)
The Interface Segregation Principle states that clients should not be forced to depend on interfaces they do not use. In other words, it’s better to have multiple smaller, specific interfaces rather than a single large, general-purpose interface.
Example of violating ISP:
interface Worker {
void work();
void eat();
void sleep();
}
class Human implements Worker {
public void work() {
// Implementation
}
public void eat() {
// Implementation
}
public void sleep() {
// Implementation
}
}
class Robot implements Worker {
public void work() {
// Implementation
}
public void eat() {
// Robots don't eat, so this is unnecessary
}
public void sleep() {
// Robots don't sleep, so this is unnecessary
}
}
To adhere to ISP, we can split the interface into smaller, more specific interfaces:
interface Workable {
void work();
}
interface Eatable {
void eat();
}
interface Sleepable {
void sleep();
}
class Human implements Workable, Eatable, Sleepable {
public void work() {
// Implementation
}
public void eat() {
// Implementation
}
public void sleep() {
// Implementation
}
}
class Robot implements Workable {
public void work() {
// Implementation
}
}
2.5 Dependency Inversion Principle (DIP)
The Dependency Inversion Principle states that high-level modules should not depend on low-level modules. Both should depend on abstractions. Additionally, abstractions should not depend on details; details should depend on abstractions.
Example of violating DIP:
class LightBulb {
public void turnOn() {
// Implementation
}
public void turnOff() {
// Implementation
}
}
class Switch {
private LightBulb bulb;
public Switch() {
bulb = new LightBulb();
}
public void operate() {
// Turn the bulb on or off
}
}
In this example, the Switch class is tightly coupled to the LightBulb class. To adhere to DIP, we can introduce an abstraction:
interface Switchable {
void turnOn();
void turnOff();
}
class LightBulb implements Switchable {
public void turnOn() {
// Implementation
}
public void turnOff() {
// Implementation
}
}
class Switch {
private Switchable device;
public Switch(Switchable device) {
this.device = device;
}
public void operate() {
// Turn the device on or off
}
}
3. Design Patterns
Design patterns are reusable solutions to common problems in software design. Understanding and implementing design patterns can greatly improve your object-oriented design skills. Here are some popular design patterns you should be familiar with for interviews:
3.1 Creational Patterns
- Singleton: Ensures a class has only one instance and provides a global point of access to it.
- Factory Method: Defines an interface for creating an object, but lets subclasses decide which class to instantiate.
- Abstract Factory: Provides an interface for creating families of related or dependent objects without specifying their concrete classes.
- Builder: Separates the construction of a complex object from its representation, allowing the same construction process to create various representations.
- Prototype: Specifies the kinds of objects to create using a prototypical instance, and creates new objects by copying this prototype.
3.2 Structural Patterns
- Adapter: Allows incompatible interfaces to work together by wrapping an object in an adapter to make it compatible with another class.
- Decorator: Attaches additional responsibilities to an object dynamically, providing a flexible alternative to subclassing for extending functionality.
- Composite: Composes objects into tree structures to represent part-whole hierarchies, allowing clients to treat individual objects and compositions uniformly.
- Proxy: Provides a surrogate or placeholder for another object to control access to it.
- Facade: Provides a unified interface to a set of interfaces in a subsystem, making the subsystem easier to use.
3.3 Behavioral Patterns
- Observer: Defines a one-to-many dependency between objects so that when one object changes state, all its dependents are notified and updated automatically.
- Strategy: Defines a family of algorithms, encapsulates each one, and makes them interchangeable, allowing the algorithm to vary independently from clients that use it.
- Command: Encapsulates a request as an object, thereby allowing for parameterization of clients with different requests, queuing of requests, and logging of the parameters.
- State: Allows an object to alter its behavior when its internal state changes, appearing to change its class.
- Template Method: Defines the skeleton of an algorithm in a method, deferring some steps to subclasses, allowing subclasses to redefine certain steps of an algorithm without changing the algorithm’s structure.
4. Practical Tips for Implementing Object-Oriented Design
When applying object-oriented design principles in your projects or during interviews, keep these practical tips in mind:
- Start with clear requirements: Before diving into design, make sure you understand the problem and requirements thoroughly.
- Identify objects and their relationships: Break down the problem into objects and determine how they interact with each other.
- Use UML diagrams: Create class diagrams and sequence diagrams to visualize your design before implementation.
- Follow the SOLID principles: Apply the SOLID principles to create a flexible and maintainable design.
- Keep it simple: Don’t over-engineer your solution. Start with a simple design and refactor as needed.
- Use design patterns appropriately: Apply design patterns when they fit the problem, but don’t force them where they’re not needed.
- Write clean, readable code: Use meaningful names for classes, methods, and variables. Follow coding standards and best practices.
- Test your design: Write unit tests to verify that your classes and their interactions work as expected.
- Refactor regularly: As you implement your design, look for opportunities to improve and refactor your code.
- Document your design decisions: Explain the rationale behind your design choices, especially during interviews.
5. Common Interview Questions on Object-Oriented Design
To help you prepare for technical interviews, here are some common questions related to object-oriented design:
- Explain the difference between composition and inheritance. When would you use one over the other?
- What is the difference between an abstract class and an interface? When would you use each?
- How does encapsulation contribute to better object-oriented design?
- Explain the concept of polymorphism and provide an example of how it can be used in practice.
- What is the purpose of the Singleton pattern, and when should it be used?
- How does the Factory Method pattern differ from the Abstract Factory pattern?
- Describe a situation where you would use the Observer pattern.
- How does the Strategy pattern promote code reusability and flexibility?
- Explain how the Decorator pattern can be used to add functionality to objects dynamically.
- What are the benefits of using the Dependency Inversion Principle in your design?
6. Conclusion
Understanding object-oriented design principles is crucial for becoming a proficient programmer and succeeding in technical interviews, especially with major tech companies. By mastering the SOLID principles, familiarizing yourself with common design patterns, and applying practical tips for implementing OOP, you’ll be well-equipped to tackle complex software design challenges.
Remember that object-oriented design is not just about following rules blindly but about creating flexible, maintainable, and scalable software. As you practice and gain experience, you’ll develop a better intuition for when and how to apply these principles effectively.
Keep honing your skills by working on diverse projects, participating in code reviews, and staying updated with the latest best practices in software design. With dedication and practice, you’ll be well-prepared to showcase your object-oriented design skills in your next technical interview and throughout your career as a software developer.