Top Tips for Acing Your Java Coding Interviews: A Comprehensive Guide
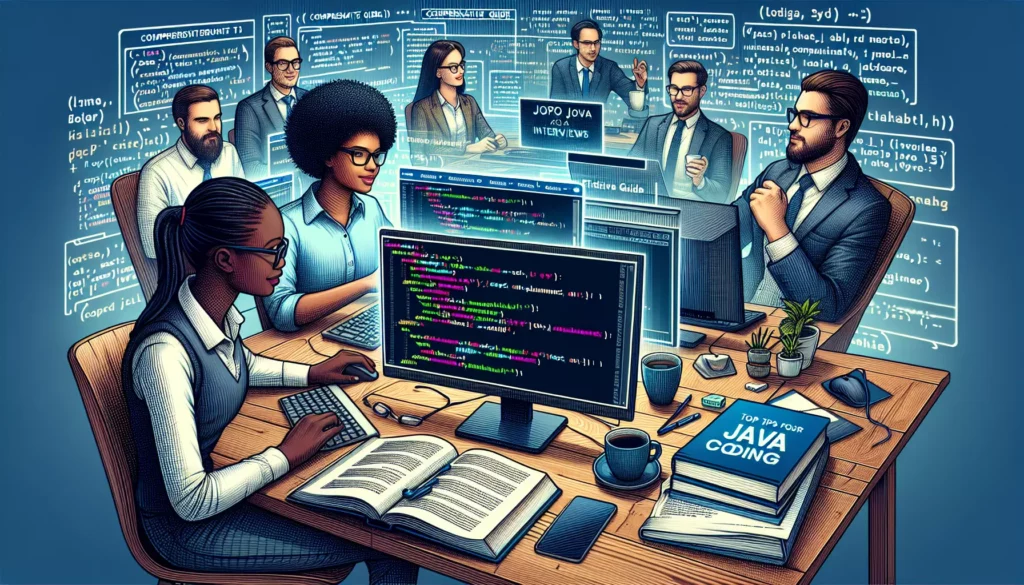
Are you gearing up for a Java coding interview at a top tech company? Whether you’re aiming for a position at one of the FAANG (Facebook, Amazon, Apple, Netflix, Google) companies or any other tech giant, being well-prepared is crucial. In this comprehensive guide, we’ll walk you through essential tips and strategies to help you excel in your Java coding interviews. From mastering core concepts to tackling common interview questions, we’ve got you covered.
1. Brush Up on Java Fundamentals
Before diving into complex algorithms and data structures, ensure you have a solid grasp of Java fundamentals. Interviewers often start with basic questions to assess your foundational knowledge. Here are some key areas to focus on:
- Object-Oriented Programming (OOP) concepts
- Java syntax and language features
- Exception handling
- Multithreading and concurrency
- Collections framework
- Generics
- Lambda expressions and functional interfaces
Make sure you can explain these concepts clearly and provide examples of how to implement them in code.
2. Master Data Structures and Algorithms
A strong understanding of data structures and algorithms is crucial for any coding interview, especially at top tech companies. Focus on the following:
Data Structures:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees (Binary Trees, Binary Search Trees, AVL Trees)
- Graphs
- Hash Tables
- Heaps
Algorithms:
- Sorting (QuickSort, MergeSort, HeapSort)
- Searching (Binary Search, Depth-First Search, Breadth-First Search)
- Dynamic Programming
- Greedy Algorithms
- Recursion and Backtracking
- Graph Algorithms (Dijkstra’s, Kruskal’s, Prim’s)
Practice implementing these data structures and algorithms in Java. Understand their time and space complexities, and be prepared to explain when to use one over another.
3. Practice Coding Problems Regularly
Consistent practice is key to success in coding interviews. Set aside time each day to solve coding problems. Here are some resources to help you practice:
- LeetCode: Offers a vast collection of coding problems, many of which are frequently asked in tech interviews.
- HackerRank: Provides a platform for coding challenges and competitions.
- CodeSignal: Offers coding assessments and interview practice.
- AlgoCademy: Provides interactive coding tutorials and AI-powered assistance for learning algorithms and data structures.
When practicing, focus on understanding the problem, developing an efficient algorithm, and implementing a clean, well-documented solution in Java.
4. Optimize Your Problem-Solving Approach
During a coding interview, your problem-solving approach is just as important as the final solution. Follow these steps:
- Clarify the problem: Ask questions to ensure you fully understand the requirements.
- Discuss potential approaches: Before coding, explain different ways to solve the problem.
- Choose an approach: Select the most efficient solution and explain your reasoning.
- Plan your code: Outline the structure of your solution before diving into implementation.
- Write clean, modular code: Use meaningful variable names and break down complex logic into smaller functions.
- Test your solution: Walk through your code with sample inputs and edge cases.
- Optimize: Discuss potential improvements in terms of time and space complexity.
Practice verbalizing your thought process as you solve problems. This helps interviewers understand your reasoning and problem-solving skills.
5. Learn to Analyze Time and Space Complexity
Understanding the efficiency of your algorithms is crucial. Be prepared to analyze and discuss the time and space complexity of your solutions using Big O notation. Here’s a quick refresher on common time complexities:
- O(1): Constant time
- O(log n): Logarithmic time (e.g., binary search)
- O(n): Linear time
- O(n log n): Linearithmic time (e.g., efficient sorting algorithms like MergeSort)
- O(n^2): Quadratic time
- O(2^n): Exponential time
Practice identifying the time and space complexity of different algorithms and data structures. This skill will help you compare different solutions and choose the most efficient one during interviews.
6. Familiarize Yourself with Java 8+ Features
Make sure you’re up to date with the latest Java features, especially those introduced in Java 8 and later versions. Some key features to focus on include:
- Lambda expressions
- Stream API
- Optional class
- Default and static methods in interfaces
- Method references
- CompletableFuture for asynchronous programming
Here’s an example of using lambda expressions and the Stream API to filter and manipulate a list of integers:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
List<Integer> evenSquares = numbers.stream()
.filter(n -> n % 2 == 0)
.map(n -> n * n)
.collect(Collectors.toList());
System.out.println(evenSquares); // Output: [4, 16, 36, 64, 100]
Being familiar with these modern Java features can help you write more concise and efficient code during interviews.
7. Practice Whiteboard Coding
Many coding interviews, especially for on-site rounds, involve writing code on a whiteboard. This can be challenging if you’re not used to it. Here are some tips to improve your whiteboard coding skills:
- Practice writing code by hand or on a physical whiteboard.
- Focus on writing clean, legible code.
- Leave space between lines for potential additions or corrections.
- Use proper indentation to make your code structure clear.
- Practice explaining your code as you write it.
If your interview is conducted remotely, you may be asked to use a shared online coding environment. Familiarize yourself with popular platforms like CoderPad or HackerRank’s CodePair to be prepared for this scenario.
8. Understand and Implement Design Patterns
Knowledge of common design patterns can be valuable in coding interviews, especially for more senior positions. Familiarize yourself with popular design patterns and their implementations in Java. Some important patterns to know include:
- Singleton
- Factory
- Observer
- Strategy
- Decorator
- Adapter
Here’s a simple example of the Singleton pattern in Java:
public class Singleton {
private static Singleton instance;
private Singleton() {}
public static Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
}
Understanding when and how to apply these patterns can demonstrate your ability to write scalable and maintainable code.
9. Be Prepared for System Design Questions
For more senior positions or at companies like Amazon and Google, you may encounter system design questions. While these are not strictly Java-specific, they often involve discussing how you would implement large-scale systems using Java technologies. Some topics to study include:
- Distributed systems
- Microservices architecture
- Database design and optimization
- Caching strategies
- Load balancing
- API design
Familiarize yourself with popular Java frameworks and technologies used in building large-scale systems, such as Spring Boot, Hibernate, and Apache Kafka.
10. Mock Interviews and Peer Programming
One of the best ways to prepare for coding interviews is to simulate the interview experience. Consider the following approaches:
- Participate in mock interviews with friends, colleagues, or through online platforms.
- Join coding meetups or study groups to practice peer programming.
- Use platforms like Pramp or interviewing.io for free mock technical interviews.
These experiences will help you get comfortable with explaining your thought process, coding under pressure, and receiving feedback on your performance.
11. Review Common Java Interview Questions
While each interview is unique, there are some common Java-specific questions that frequently come up. Be prepared to answer questions on topics such as:
- Differences between abstract classes and interfaces
- Immutability in Java and how to create immutable classes
- Java memory management and garbage collection
- Differences between checked and unchecked exceptions
- Implementing thread-safe code
- SOLID principles in object-oriented design
Here’s an example of how to create an immutable class in Java:
public final class ImmutablePerson {
private final String name;
private final int age;
public ImmutablePerson(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
}
Practice explaining these concepts clearly and providing code examples when appropriate.
12. Optimize Your Java Code
During interviews, you may be asked to optimize your initial solution. Be familiar with common optimization techniques in Java, such as:
- Using appropriate data structures (e.g., HashSet for fast lookups)
- Avoiding unnecessary object creation
- Utilizing lazy initialization when appropriate
- Implementing caching mechanisms
- Using primitives instead of wrapper classes when possible
- Leveraging Java 8+ features like parallel streams for performance improvements
Here’s an example of using a HashSet for efficient lookups:
public boolean containsDuplicate(int[] nums) {
Set<Integer> seen = new HashSet<>();
for (int num : nums) {
if (seen.contains(num)) {
return true;
}
seen.add(num);
}
return false;
}
Being able to identify and implement these optimizations can set you apart in coding interviews.
13. Stay Calm and Communicate Effectively
Finally, remember that your communication skills are just as important as your technical abilities. During the interview:
- Stay calm and composed, even if you encounter a challenging problem.
- Think out loud and explain your reasoning as you work through problems.
- Ask clarifying questions when needed.
- Be open to hints or suggestions from the interviewer.
- If you get stuck, don’t be afraid to ask for help or discuss potential approaches with the interviewer.
Remember, interviewers are not just evaluating your coding skills but also assessing how you would work as part of their team.
Conclusion
Preparing for Java coding interviews at top tech companies requires dedication, practice, and a comprehensive understanding of both fundamental and advanced concepts. By following these tips and consistently honing your skills, you’ll be well-equipped to tackle even the most challenging interview questions.
Remember to leverage resources like AlgoCademy, which offers interactive coding tutorials and AI-powered assistance to help you master algorithms and data structures. With the right preparation and mindset, you can confidently showcase your Java programming skills and increase your chances of landing your dream job at a top tech company.
Good luck with your interviews, and happy coding!