Mastering Multi-Threading and Concurrency: Essential Preparation for Coding Interviews
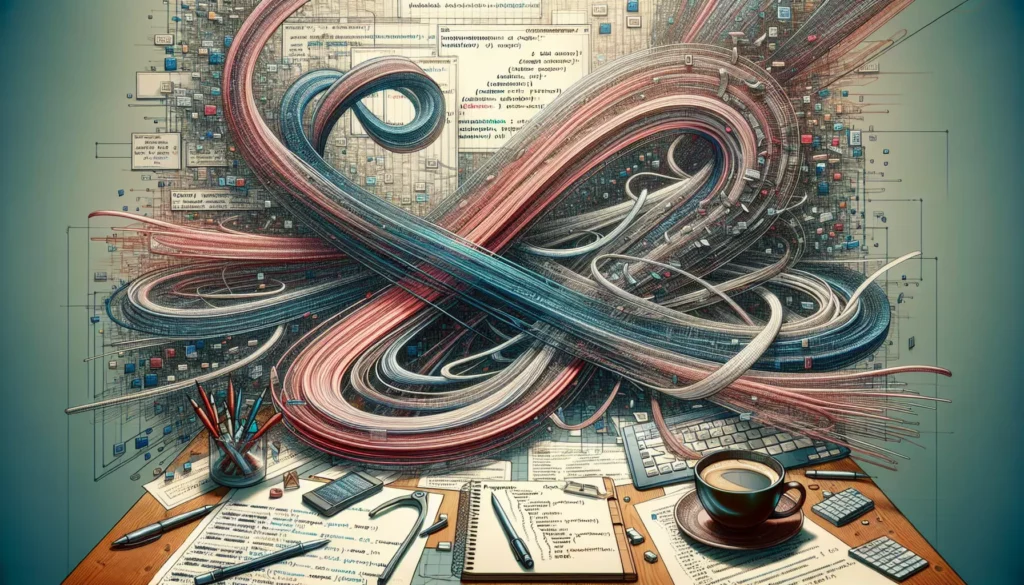
In the world of software development, multi-threading and concurrency are crucial concepts that can make or break the performance and efficiency of applications. As you prepare for coding interviews, especially those targeting major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, and Google), understanding these topics is essential. This comprehensive guide will walk you through the fundamentals of multi-threading and concurrency, provide practical examples, and offer strategies to tackle related interview questions.
Understanding Multi-Threading and Concurrency
Before diving into the specifics, it’s important to clarify the distinction between multi-threading and concurrency:
- Multi-threading refers to the ability of a CPU or a single core in a multi-core processor to provide multiple threads of execution concurrently.
- Concurrency is a broader concept that deals with managing and coordinating multiple tasks or processes that may or may not execute simultaneously.
While these terms are often used interchangeably, understanding their nuances is crucial for mastering interview questions on this topic.
Key Concepts in Multi-Threading
1. Threads
A thread is the smallest unit of execution within a process. Multiple threads can exist within the same process, sharing the same memory space and resources. This allows for efficient communication between threads but also introduces potential synchronization issues.
2. Process vs. Thread
While a process is an independent program with its own memory space, threads within a process share the same memory space. This distinction is important when considering the overhead and communication mechanisms between different units of execution.
3. Thread States
Threads can exist in various states throughout their lifecycle:
- New: The thread has been created but not yet started.
- Runnable: The thread is ready to run and waiting for CPU time.
- Running: The thread is currently executing.
- Blocked: The thread is waiting for a resource or synchronization.
- Terminated: The thread has completed its execution.
4. Thread Synchronization
When multiple threads access shared resources, synchronization mechanisms are necessary to prevent race conditions and ensure data integrity. Common synchronization techniques include:
- Locks and Mutexes
- Semaphores
- Monitors
- Atomic Operations
Concurrency Concepts
1. Parallelism vs. Concurrency
While concurrency deals with managing multiple tasks, parallelism involves executing multiple tasks simultaneously. Understanding this distinction is crucial for designing efficient multi-threaded systems.
2. Deadlocks and Livelocks
Deadlocks occur when two or more threads are unable to proceed because each is waiting for the other to release a resource. Livelocks are similar, but threads are actively trying to resolve the situation, yet making no progress.
3. Race Conditions
A race condition happens when the behavior of a program depends on the relative timing of events, such as the order of thread execution. Identifying and resolving race conditions is a critical skill in concurrent programming.
4. Thread-Safe Data Structures
Certain data structures are designed to be safely used in multi-threaded environments without external synchronization. Examples include ConcurrentHashMap and BlockingQueue in Java.
Common Interview Questions and Strategies
When preparing for multi-threading and concurrency questions in coding interviews, focus on the following areas:
1. Implementing Basic Thread Operations
Be prepared to demonstrate your understanding of thread creation, starting, and joining. Here’s a simple example in Java:
public class ThreadExample extends Thread {
public void run() {
System.out.println("Thread is running");
}
public static void main(String[] args) {
ThreadExample thread = new ThreadExample();
thread.start();
try {
thread.join();
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("Main thread finished");
}
}
2. Solving Producer-Consumer Problems
The producer-consumer problem is a classic synchronization issue. Be ready to implement a solution using synchronization primitives. Here’s a basic implementation using a BlockingQueue in Java:
import java.util.concurrent.BlockingQueue;
import java.util.concurrent.LinkedBlockingQueue;
class Producer implements Runnable {
private final BlockingQueue<Integer> queue;
Producer(BlockingQueue<Integer> q) { queue = q; }
public void run() {
try {
for (int i = 0; i < 10; i++) {
queue.put(i);
System.out.println("Produced " + i);
Thread.sleep(100);
}
} catch (InterruptedException ex) {
Thread.currentThread().interrupt();
}
}
}
class Consumer implements Runnable {
private final BlockingQueue<Integer> queue;
Consumer(BlockingQueue<Integer> q) { queue = q; }
public void run() {
try {
while (true) {
Integer item = queue.take();
System.out.println("Consumed " + item);
Thread.sleep(200);
}
} catch (InterruptedException ex) {
Thread.currentThread().interrupt();
}
}
}
public class ProducerConsumerExample {
public static void main(String[] args) {
BlockingQueue<Integer> queue = new LinkedBlockingQueue<>(5);
Thread producerThread = new Thread(new Producer(queue));
Thread consumerThread = new Thread(new Consumer(queue));
producerThread.start();
consumerThread.start();
}
}
3. Implementing Thread-Safe Singleton Pattern
The Singleton pattern is often used in multi-threaded environments. Be prepared to implement a thread-safe version:
public class ThreadSafeSingleton {
private static volatile ThreadSafeSingleton instance;
private ThreadSafeSingleton() {}
public static ThreadSafeSingleton getInstance() {
if (instance == null) {
synchronized (ThreadSafeSingleton.class) {
if (instance == null) {
instance = new ThreadSafeSingleton();
}
}
}
return instance;
}
}
4. Solving Dining Philosophers Problem
This classic problem illustrates synchronization issues and deadlock avoidance. Be ready to discuss and implement a solution:
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
class Philosopher extends Thread {
private final int id;
private final Lock leftFork;
private final Lock rightFork;
public Philosopher(int id, Lock leftFork, Lock rightFork) {
this.id = id;
this.leftFork = leftFork;
this.rightFork = rightFork;
}
private void think() throws InterruptedException {
System.out.println("Philosopher " + id + " is thinking");
Thread.sleep((long) (Math.random() * 1000));
}
private void eat() throws InterruptedException {
System.out.println("Philosopher " + id + " is eating");
Thread.sleep((long) (Math.random() * 1000));
}
@Override
public void run() {
try {
while (true) {
think();
if (id % 2 == 0) {
leftFork.lock();
rightFork.lock();
} else {
rightFork.lock();
leftFork.lock();
}
eat();
leftFork.unlock();
rightFork.unlock();
}
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
}
public class DiningPhilosophers {
public static void main(String[] args) {
int numPhilosophers = 5;
Lock[] forks = new ReentrantLock[numPhilosophers];
Philosopher[] philosophers = new Philosopher[numPhilosophers];
for (int i = 0; i < numPhilosophers; i++) {
forks[i] = new ReentrantLock();
}
for (int i = 0; i < numPhilosophers; i++) {
philosophers[i] = new Philosopher(i, forks[i], forks[(i + 1) % numPhilosophers]);
philosophers[i].start();
}
}
}
Advanced Topics
As you progress in your preparation, consider exploring these advanced topics:
1. Thread Pools and Executors
Understanding thread pools and executor services is crucial for efficient multi-threaded application design. Be prepared to discuss the advantages of thread pools and implement basic examples using Java’s Executor framework.
2. Concurrent Collections
Familiarize yourself with concurrent collections like ConcurrentHashMap, CopyOnWriteArrayList, and BlockingQueue. Know when and how to use these thread-safe alternatives to standard collections.
3. Atomics and Lock-Free Programming
Explore atomic variables and lock-free programming techniques. Understand the benefits and challenges of implementing non-blocking algorithms.
4. Futures and Promises
Learn about asynchronous programming models using Futures and Promises. Be prepared to discuss how these constructs can improve the performance and responsiveness of multi-threaded applications.
Best Practices for Multi-Threading and Concurrency
When working on multi-threading and concurrency problems, keep these best practices in mind:
- Minimize shared state: Reduce the amount of shared mutable state to minimize synchronization needs.
- Use immutable objects: Immutable objects are inherently thread-safe and can simplify concurrent code.
- Prefer higher-level concurrency utilities: Use built-in concurrency utilities like ExecutorService and concurrent collections instead of low-level synchronization primitives when possible.
- Be aware of deadlock conditions: Always acquire locks in a consistent order to prevent deadlocks.
- Use thread-local storage: When appropriate, use thread-local storage to maintain thread-specific data without synchronization.
- Implement proper error handling: Handle InterruptedException and other concurrency-related exceptions appropriately.
- Use timeouts: Implement timeouts when acquiring locks or waiting for resources to prevent indefinite blocking.
- Profile and test thoroughly: Use profiling tools to identify bottlenecks and thoroughly test concurrent code under various conditions.
Conclusion
Mastering multi-threading and concurrency is a crucial skill for any software developer, especially when preparing for interviews at top tech companies. By understanding the fundamental concepts, practicing common problems, and exploring advanced topics, you’ll be well-equipped to tackle even the most challenging interview questions in this domain.
Remember that the key to success in coding interviews is not just knowing the concepts but being able to apply them to solve real-world problems. As you prepare, focus on writing clean, efficient, and thread-safe code. Practice explaining your thought process and discussing trade-offs in your solutions.
Continuous learning and practice are essential in this rapidly evolving field. Stay updated with the latest developments in concurrent programming, explore new languages and frameworks, and never stop challenging yourself with complex multi-threading scenarios.
With dedication and the right approach, you’ll be well-prepared to showcase your multi-threading and concurrency skills in your next coding interview, bringing you one step closer to landing your dream job at a top tech company.