How to Discuss Trade-offs in Your Coding Solutions: A Comprehensive Guide
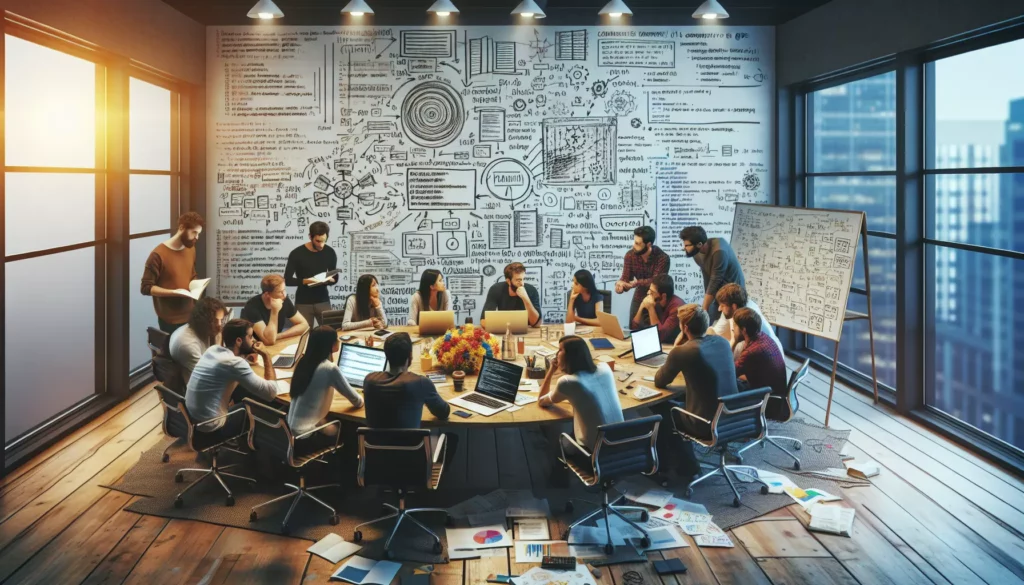
In the world of software development and algorithmic problem-solving, there’s rarely a one-size-fits-all solution. Every coding decision comes with its own set of trade-offs, and being able to articulate these trade-offs is a crucial skill for any programmer. Whether you’re a beginner just starting your coding journey or an experienced developer preparing for technical interviews at top tech companies, understanding and discussing trade-offs can set you apart. In this comprehensive guide, we’ll explore the art of discussing trade-offs in your coding solutions, providing you with the tools to make informed decisions and communicate your reasoning effectively.
Why Are Trade-offs Important in Coding?
Before we dive into the how-to, let’s understand why trade-offs are so crucial in the world of programming:
- Real-world constraints: In practical scenarios, you often have to balance multiple factors such as time, space, readability, and scalability.
- Problem-solving skills: Recognizing and evaluating trade-offs demonstrates your ability to think critically about your code.
- Interview performance: Many technical interviews, especially at FAANG companies, assess your ability to discuss trade-offs.
- Code optimization: Understanding trade-offs helps you write more efficient and appropriate code for specific situations.
- Team collaboration: Being able to explain your choices helps in code reviews and team discussions.
Common Types of Trade-offs in Coding
Before we get into how to discuss trade-offs, let’s familiarize ourselves with some common types you might encounter:
1. Time Complexity vs. Space Complexity
This is perhaps the most classic trade-off in algorithm design. You often have to choose between an algorithm that runs faster (better time complexity) but uses more memory, or one that uses less memory but takes longer to execute.
2. Readability vs. Performance
Sometimes, the most performant code isn’t the most readable. You might have to decide between writing code that’s easier for humans to understand and maintain, or code that runs more efficiently.
3. Flexibility vs. Simplicity
Do you write a more generic, flexible solution that can handle various scenarios, or a simpler, more straightforward solution tailored to the specific problem at hand?
4. Development Time vs. Runtime Efficiency
In some cases, you might need to choose between spending more time developing a highly optimized solution, or quickly implementing a “good enough” solution that meets immediate needs.
5. Modularity vs. Performance
Breaking code into smaller, reusable modules can improve maintainability but might introduce some performance overhead.
How to Discuss Trade-offs Effectively
Now that we understand the importance and types of trade-offs, let’s explore how to discuss them effectively:
1. Identify the Trade-offs
The first step is to recognize that a trade-off exists. Ask yourself:
- What are the pros and cons of this approach?
- What alternative solutions exist?
- What am I gaining and what am I giving up with this choice?
2. Quantify the Impact
Where possible, use concrete numbers or Big O notation to describe the impact of your choices. For example:
“By using a hash table instead of an array, we’ve improved the lookup time from O(n) to O(1), but we’ve increased our space complexity from O(1) to O(n).”
3. Consider the Context
The best solution often depends on the specific context of the problem. Consider factors like:
- The scale of the data you’re working with
- The frequency of certain operations
- The constraints of the system (e.g., memory limitations)
- The priorities of the project (e.g., speed of development vs. long-term maintainability)
4. Present Multiple Options
Instead of just defending your chosen solution, present multiple viable options and discuss the trade-offs of each. This demonstrates your ability to see the problem from different angles.
5. Explain Your Reasoning
Clearly articulate why you’ve made a particular choice given the trade-offs. Use phrases like:
- “I chose this approach because…”
- “The main advantage of this solution is…”
- “While this increases our space complexity, it significantly improves our runtime, which is crucial for our use case because…”
6. Acknowledge the Downsides
Don’t shy away from discussing the disadvantages of your chosen approach. This shows that you’ve thoroughly considered the implications of your decision.
7. Consider Future Implications
Discuss how your choice might impact future development or scaling of the system. For example:
“While this solution works well for our current data size, if we expect the input to grow significantly, we might need to revisit this approach due to its O(n^2) time complexity.”
8. Use Analogies or Real-world Examples
Sometimes, using analogies or relating the trade-off to a real-world scenario can help make your point more understandable, especially when explaining to non-technical stakeholders.
Example: Discussing Trade-offs in a Coding Problem
Let’s walk through an example of how to discuss trade-offs for a common coding problem: finding the two numbers in an array that sum to a target value.
Problem Statement:
Given an array of integers and a target sum, find two numbers in the array that add up to the target sum.
Solution 1: Brute Force Approach
def find_two_sum_brute_force(nums, target):
for i in range(len(nums)):
for j in range(i+1, len(nums)):
if nums[i] + nums[j] == target:
return [nums[i], nums[j]]
return []
Trade-off Discussion:
“The brute force approach has a time complexity of O(n^2) because we’re using nested loops to check every possible pair of numbers. The space complexity is O(1) as we’re not using any additional data structures.
The main advantage of this approach is its simplicity and low space usage. It’s easy to implement and understand, which can be beneficial for code maintainability. However, the quadratic time complexity makes it inefficient for large input sizes.
This solution might be appropriate if we have a small input size or if memory is extremely constrained. However, for larger inputs or in scenarios where we need faster execution, we should consider alternative approaches.”
Solution 2: Hash Table Approach
def find_two_sum_hash(nums, target):
num_map = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_map:
return [num, complement]
num_map[num] = i
return []
Trade-off Discussion:
“The hash table approach improves our time complexity to O(n) as we only need to iterate through the array once. However, this comes at the cost of increased space complexity, which is now O(n) due to the hash table.
The main advantage of this solution is its efficiency for larger inputs. By trading off some memory, we’ve significantly improved the runtime. This approach is particularly beneficial when we expect to have large input sizes or when the function will be called frequently.
The downside is the increased memory usage, which might be a concern in memory-constrained environments. Additionally, while hash table operations are generally O(1), in the worst case (with many hash collisions), they can degrade to O(n), potentially affecting our average-case performance.
Given that most modern systems have sufficient memory and that the improved time complexity often outweighs the memory cost, this solution is generally preferable in practice, especially when dealing with larger datasets or in performance-critical applications.”
Advanced Considerations in Trade-off Discussions
As you become more proficient in discussing trade-offs, consider incorporating these advanced topics:
1. Asymptotic vs. Practical Performance
While Big O notation is crucial for understanding algorithmic efficiency, it’s also important to consider practical performance for your specific use case. Sometimes, an algorithm with a worse asymptotic complexity might perform better for small inputs due to lower constant factors or better cache efficiency.
For example, you might say:
“Although quicksort has a worst-case time complexity of O(n^2) compared to mergesort’s O(n log n), in practice, quicksort often outperforms mergesort for smaller arrays due to its better cache locality and lower constant factors. However, for very large datasets or when worst-case performance guarantees are crucial, mergesort might be the safer choice.”
2. Amortized Analysis
Some data structures, like dynamic arrays (e.g., ArrayList in Java or list in Python), have operations that occasionally take longer but result in better performance over a series of operations. Understanding and discussing amortized complexity can provide a more nuanced view of your solution’s efficiency.
For instance:
“While adding an element to a dynamic array can sometimes take O(n) time when resizing is needed, the amortized time complexity is O(1). This means that over a series of operations, using a dynamic array can be more efficient than using a linked list for certain scenarios, despite the occasional costly resize operation.”
3. System-Level Considerations
As you tackle more complex problems, consider broader system-level trade-offs:
- Vertical vs. Horizontal Scaling: Discuss the trade-offs between optimizing a single machine’s performance vs. distributing the workload across multiple machines.
- Consistency vs. Availability: In distributed systems, you often can’t have both perfect consistency and 100% availability (as per the CAP theorem). Discuss which is more important for your specific use case.
- Latency vs. Throughput: Sometimes, optimizing for one can negatively impact the other.
4. Language and Platform-Specific Trade-offs
Different programming languages and platforms come with their own sets of trade-offs. Being aware of these can add depth to your discussions:
“While Python’s dynamic typing and high-level abstractions make it excellent for rapid development and readability, it typically has slower execution speeds compared to statically-typed, compiled languages like C++ or Rust. For our data processing pipeline, we’ve chosen Python for the prototyping phase to quickly iterate on ideas, but we plan to reimplement performance-critical sections in C++ once the architecture is finalized.”
5. Security Implications
In many real-world scenarios, security considerations can introduce additional trade-offs:
“Using a more secure encryption algorithm increases our data protection but also increases our processing time and energy consumption. For this financial application, we’ve prioritized security over performance, but for less sensitive data, we might make a different choice.”
Practicing Trade-off Discussions
Improving your ability to discuss trade-offs is largely a matter of practice. Here are some ways to hone this skill:
1. Solve Problems with Multiple Approaches
When practicing coding problems, don’t stop at finding a working solution. Try to come up with multiple valid approaches and compare them.
2. Participate in Code Reviews
Whether at work or in open-source projects, code reviews are an excellent opportunity to discuss trade-offs in a real-world context.
3. Explain Your Code to Others
Teaching or explaining your code to others, whether in person or through blog posts or forums, can help you articulate your thoughts more clearly.
4. Analyze Existing Systems
Look at popular open-source projects or well-known systems and try to understand and articulate the trade-offs in their design decisions.
5. Mock Interviews
Practice discussing trade-offs in mock interview settings. Many online platforms offer this service, or you can practice with peers.
Conclusion
The ability to effectively discuss trade-offs in your coding solutions is a hallmark of an experienced and thoughtful programmer. It demonstrates not just your technical knowledge, but also your problem-solving skills, your ability to see the bigger picture, and your capacity to make informed decisions in complex scenarios.
Remember, there’s rarely a perfect solution in software development. Each choice comes with its own set of pros and cons, and the best decision often depends on the specific context and requirements of your project. By mastering the art of trade-off discussions, you’ll be better equipped to make these decisions and to communicate your reasoning to others.
As you continue your journey in coding education and skills development, whether you’re working through interactive tutorials, preparing for technical interviews, or building real-world projects, always take a moment to consider the trade-offs in your solutions. This practice will not only improve your coding skills but also enhance your overall effectiveness as a software developer.
Keep coding, keep analyzing, and keep discussing those trade-offs!