Understanding Combinatorics and Probability in Coding Interviews
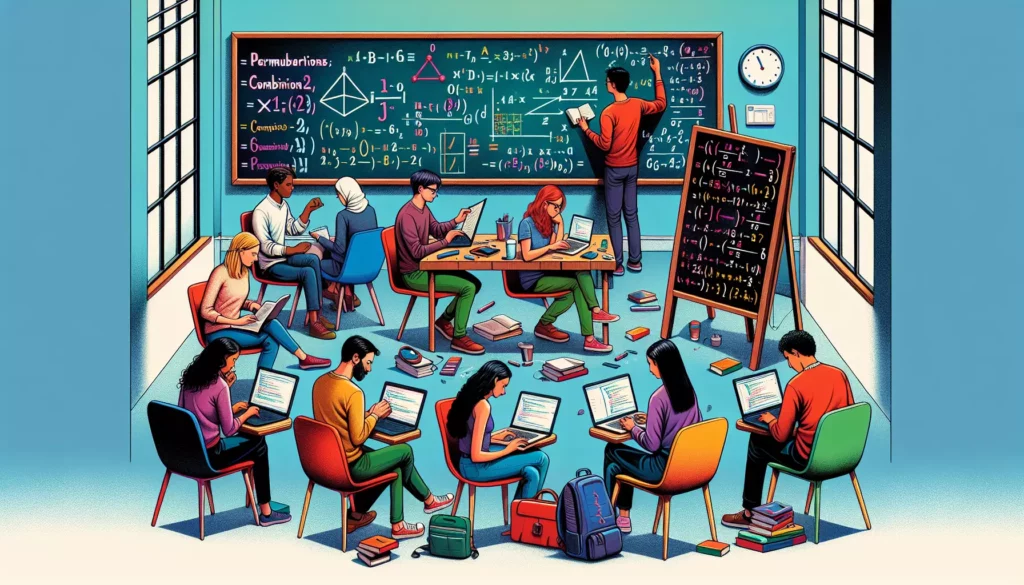
In the world of coding interviews, particularly for prestigious tech companies like FAANG (Facebook, Amazon, Apple, Netflix, and Google), a strong grasp of combinatorics and probability can be a game-changer. These mathematical concepts often underpin complex algorithms and data structures, making them essential tools in a programmer’s toolkit. In this comprehensive guide, we’ll dive deep into the realm of combinatorics and probability, exploring their applications in coding interviews and providing you with the knowledge you need to excel.
1. Introduction to Combinatorics
Combinatorics is the branch of mathematics dealing with combinations of objects belonging to a finite set in accordance with certain constraints. In the context of coding interviews, combinatorics often comes into play when solving problems related to counting, arranging, and selecting objects.
1.1 Basic Counting Principles
The foundation of combinatorics lies in two fundamental counting principles:
- Addition Principle: If event A can occur in m ways, and event B can occur in n ways, and the two events cannot occur simultaneously, then event A or B can occur in m + n ways.
- Multiplication Principle: If event A can occur in m ways, and event B can occur in n ways, then events A and B can occur together in m * n ways.
These principles form the basis for more complex combinatorial calculations and are often used in coding interview questions involving counting possibilities.
1.2 Permutations
Permutations deal with arranging objects in a specific order. The number of permutations of n distinct objects is given by n! (n factorial). In coding interviews, you might encounter problems where you need to generate all possible permutations of a given set or calculate the number of possible arrangements.
Here’s a simple Python function to generate all permutations of a list:
def generate_permutations(lst):
if len(lst) <= 1:
return [lst]
perms = []
for i in range(len(lst)):
current = lst[i]
remaining = lst[:i] + lst[i+1:]
for perm in generate_permutations(remaining):
perms.append([current] + perm)
return perms
# Example usage
print(generate_permutations([1, 2, 3]))
1.3 Combinations
Combinations involve selecting a subset of objects from a larger set, where the order doesn’t matter. The number of ways to choose k objects from a set of n objects is denoted as C(n,k) or (n choose k), and is calculated as:
C(n,k) = n! / (k! * (n-k)!)
In coding interviews, you might need to generate all possible combinations or calculate the number of combinations for a given scenario.
Here’s a Python function to calculate the number of combinations:
def calculate_combinations(n, k):
from math import factorial
return factorial(n) // (factorial(k) * factorial(n - k))
# Example usage
print(calculate_combinations(5, 2)) # Outputs: 10
2. Probability in Coding Interviews
Probability theory is the study of random events and their outcomes. In coding interviews, probability concepts often arise in questions related to randomized algorithms, game theory, and statistical analysis.
2.1 Basic Probability Concepts
Some key probability concepts you should be familiar with include:
- Sample Space: The set of all possible outcomes of an experiment.
- Event: A subset of the sample space.
- Probability of an Event: The likelihood of an event occurring, usually expressed as a number between 0 and 1.
- Conditional Probability: The probability of an event occurring given that another event has already occurred.
- Independence: Two events are independent if the occurrence of one does not affect the probability of the other.
2.2 Probability Distributions
In coding interviews, you might encounter questions involving various probability distributions. Some common ones include:
- Uniform Distribution: All outcomes are equally likely.
- Binomial Distribution: Models the number of successes in a fixed number of independent Bernoulli trials.
- Poisson Distribution: Models the number of events occurring in a fixed interval of time or space.
Understanding these distributions can be crucial for solving problems related to randomized algorithms or simulations.
2.3 Expected Value
The expected value of a random variable is the sum of all possible values, each multiplied by its probability of occurrence. In coding interviews, you might need to calculate expected values for various scenarios, such as the expected number of iterations in a randomized algorithm.
Here’s a simple Python function to calculate the expected value of a discrete random variable:
def expected_value(values, probabilities):
return sum(v * p for v, p in zip(values, probabilities))
# Example usage
values = [1, 2, 3, 4, 5]
probabilities = [0.1, 0.2, 0.3, 0.2, 0.2]
print(expected_value(values, probabilities)) # Outputs: 3.1
3. Applications in Coding Interviews
Now that we’ve covered the basics of combinatorics and probability, let’s explore how these concepts are applied in coding interviews.
3.1 Randomized Algorithms
Randomized algorithms use random numbers to make decisions during execution. They often provide efficient solutions to problems that are difficult to solve deterministically. Understanding probability is crucial for analyzing the performance and correctness of these algorithms.
Example: QuickSort with Random Pivot
import random
def quicksort(arr):
if len(arr) <= 1:
return arr
else:
pivot = random.choice(arr)
left = [x for x in arr if x < pivot]
middle = [x for x in arr if x == pivot]
right = [x for x in arr if x > pivot]
return quicksort(left) + middle + quicksort(right)
# Example usage
print(quicksort([3, 6, 8, 10, 1, 2, 1]))
In this implementation, we randomly choose the pivot element. The expected time complexity of this randomized QuickSort is O(n log n), which is better than the worst-case O(n^2) of the deterministic version.
3.2 Sampling and Reservoir Sampling
Sampling problems often appear in coding interviews, especially when dealing with large datasets or streams of data. Reservoir sampling is a family of randomized algorithms for randomly choosing k samples from a list of n items, where n is either a very large or unknown number.
Here’s an implementation of reservoir sampling to select k items from a stream:
import random
def reservoir_sampling(stream, k):
reservoir = []
for i, item in enumerate(stream):
if i < k:
reservoir.append(item)
else:
j = random.randint(0, i)
if j < k:
reservoir[j] = item
return reservoir
# Example usage
stream = range(1000000)
k = 10
print(reservoir_sampling(stream, k))
This algorithm maintains a uniform distribution over all items in the stream, regardless of its length.
3.3 Probability in Game Theory
Game theory problems often involve probability calculations. For example, you might be asked to implement a fair coin toss using a biased coin, or to calculate the probability of winning a certain game.
Example: Implementing a fair coin toss with a biased coin
import random
def biased_coin_flip(p):
return random.random() < p
def fair_coin_flip():
while True:
flip1 = biased_coin_flip(0.7) # Assume the biased coin has 70% chance of heads
flip2 = biased_coin_flip(0.7)
if flip1 and not flip2:
return True # Heads
if not flip1 and flip2:
return False # Tails
# If both flips are the same, we ignore the result and try again
# Example usage
results = [fair_coin_flip() for _ in range(1000)]
print(f"Proportion of heads: {sum(results) / len(results)}")
This algorithm uses the principle that P(H,T) = P(T,H) for any biased coin, allowing us to create a fair coin toss from a biased one.
3.4 Combinatorics in Dynamic Programming
Many dynamic programming problems involve combinatorial calculations. Understanding combinatorics can help you recognize patterns and formulate efficient solutions.
Example: Calculating the number of unique paths in a grid
def unique_paths(m, n):
dp = [[1] * n for _ in range(m)]
for i in range(1, m):
for j in range(1, n):
dp[i][j] = dp[i-1][j] + dp[i][j-1]
return dp[m-1][n-1]
# Example usage
print(unique_paths(3, 7)) # Outputs: 28
This problem can be solved using combinatorics (it’s equivalent to choosing m-1 down moves from m+n-2 total moves), but the dynamic programming approach shown here is often more intuitive in coding interviews.
4. Advanced Topics
As you progress in your interview preparation, you may encounter more advanced topics that combine combinatorics, probability, and algorithmic thinking.
4.1 Expectation Maximization
Expectation Maximization (EM) is a powerful algorithm used in machine learning for finding maximum likelihood estimates of parameters in statistical models. While you’re unlikely to implement EM from scratch in an interview, understanding its principles can be valuable for discussing complex probabilistic algorithms.
4.2 Probabilistic Data Structures
Probabilistic data structures use randomization to achieve efficient space usage and query times, often at the cost of small, controllable errors. Examples include:
- Bloom Filters: Used for efficient set membership testing
- Count-Min Sketch: Used for frequency estimation in data streams
- HyperLogLog: Used for cardinality estimation of large sets
Here’s a simple implementation of a Bloom filter:
import mmh3
from bitarray import bitarray
class BloomFilter:
def __init__(self, size, hash_count):
self.size = size
self.hash_count = hash_count
self.bit_array = bitarray(size)
self.bit_array.setall(0)
def add(self, item):
for seed in range(self.hash_count):
index = mmh3.hash(item, seed) % self.size
self.bit_array[index] = 1
def check(self, item):
for seed in range(self.hash_count):
index = mmh3.hash(item, seed) % self.size
if self.bit_array[index] == 0:
return False
return True
# Example usage
bf = BloomFilter(100, 3)
bf.add("apple")
bf.add("banana")
print(bf.check("apple")) # True
print(bf.check("orange")) # False (probably)
4.3 Monte Carlo Methods
Monte Carlo methods rely on repeated random sampling to obtain numerical results. They’re often used when it’s infeasible to compute an exact result with a deterministic algorithm. In coding interviews, you might be asked to implement simple Monte Carlo simulations or discuss their applications.
Example: Estimating π using Monte Carlo method
import random
def estimate_pi(num_points):
inside_circle = 0
total_points = num_points
for _ in range(total_points):
x = random.uniform(-1, 1)
y = random.uniform(-1, 1)
if x*x + y*y <= 1:
inside_circle += 1
pi_estimate = 4 * inside_circle / total_points
return pi_estimate
# Example usage
print(estimate_pi(1000000))
5. Preparing for Combinatorics and Probability Questions in Interviews
To excel in combinatorics and probability questions during coding interviews, consider the following tips:
- Practice Fundamentals: Make sure you have a solid grasp of basic combinatorics and probability concepts. Review permutations, combinations, conditional probability, and expected value calculations.
- Solve Diverse Problems: Work on a variety of problems that involve combinatorics and probability. Websites like LeetCode, HackerRank, and Project Euler offer many such problems.
- Implement from Scratch: Try implementing probabilistic algorithms and data structures from scratch. This will deepen your understanding and prepare you for coding challenges.
- Analyze Time and Space Complexity: For any solution you develop, be prepared to discuss its time and space complexity. In probabilistic algorithms, you may need to discuss expected vs. worst-case complexity.
- Understand Real-world Applications: Familiarize yourself with how combinatorics and probability are used in real-world scenarios, especially in the context of large-scale systems and data processing.
- Practice Explaining Your Thought Process: In interviews, clearly communicating your approach is as important as arriving at the correct solution. Practice explaining your reasoning and the steps you take to solve a problem.
Conclusion
Combinatorics and probability are powerful tools in a programmer’s arsenal, especially when preparing for coding interviews at top tech companies. By mastering these concepts and their applications in algorithms and data structures, you’ll be well-equipped to tackle a wide range of interview questions and real-world programming challenges.
Remember, the key to success in coding interviews is not just knowing the concepts, but being able to apply them creatively to solve problems. Continue practicing, stay curious, and don’t hesitate to dive deep into the mathematical foundations of computer science. With dedication and the right approach, you’ll be well on your way to acing your next coding interview!