Approaching Bit Manipulation Problems: A Comprehensive Guide for Coding Interviews
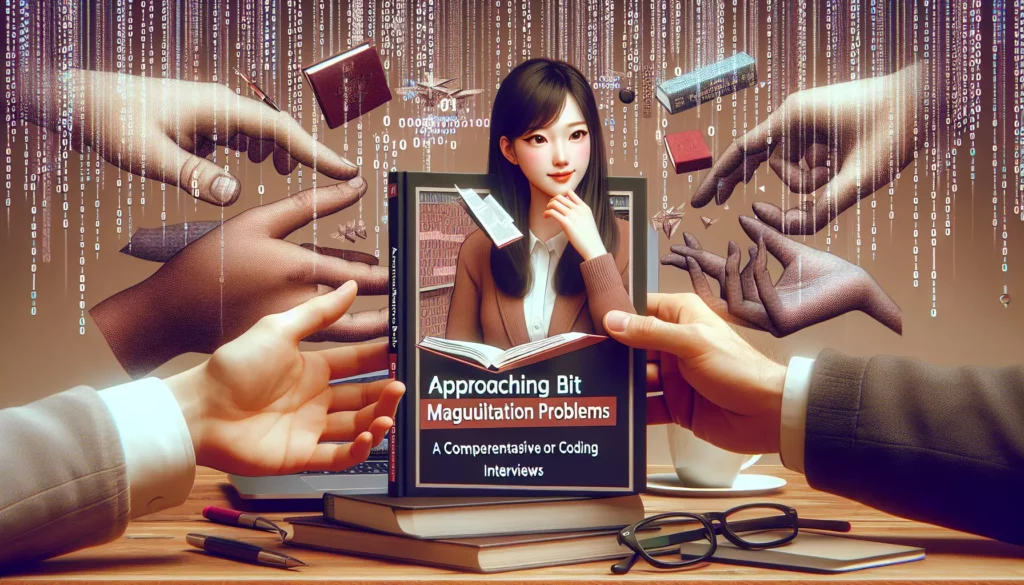
In the world of coding interviews, particularly those for major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), bit manipulation problems are a common and often challenging type of question. These problems test a candidate’s understanding of how computers represent and manipulate data at the lowest level. Mastering bit manipulation can give you a significant edge in technical interviews and improve your overall programming skills. In this comprehensive guide, we’ll explore strategies for approaching bit manipulation problems, common techniques, and practical examples to help you excel in your coding interviews.
Understanding Bit Manipulation
Before diving into problem-solving strategies, it’s crucial to have a solid understanding of what bit manipulation is and why it’s important in computer science.
What is Bit Manipulation?
Bit manipulation refers to the process of applying logical operations on a sequence of bits to achieve a desired result. In programming, we often work with data at a higher level of abstraction, but understanding how to manipulate bits directly can lead to more efficient algorithms and solutions to certain problems.
Why is Bit Manipulation Important?
- Efficiency: Bit manipulation operations are typically very fast, as they are directly supported by hardware.
- Space optimization: Using bits to represent data can significantly reduce memory usage in certain scenarios.
- Low-level operations: Some tasks, particularly in systems programming or embedded systems, require direct bit manipulation.
- Interview questions: Bit manipulation problems are popular in coding interviews as they test a candidate’s understanding of fundamental computer science concepts.
Basic Bit Manipulation Operations
Before we can effectively solve bit manipulation problems, we need to be familiar with the basic operations. Here are the fundamental bitwise operators in most programming languages:
- AND (&): Returns 1 if both bits are 1, otherwise 0.
- OR (|): Returns 1 if at least one of the bits is 1, otherwise 0.
- XOR (^): Returns 1 if the bits are different, otherwise 0.
- NOT (~): Inverts all the bits.
- Left Shift (<<): Shifts all bits to the left by a specified number of positions.
- Right Shift (>>): Shifts all bits to the right by a specified number of positions.
Let’s look at some examples of these operations:
// AND operation
5 & 3 = 101 & 011 = 001 = 1
// OR operation
5 | 3 = 101 | 011 = 111 = 7
// XOR operation
5 ^ 3 = 101 ^ 011 = 110 = 6
// NOT operation
~5 = ~(00000101) = 11111010 = -6 (in two's complement)
// Left Shift
5 << 1 = 101 << 1 = 1010 = 10
// Right Shift
5 >> 1 = 101 >> 1 = 010 = 2
Common Bit Manipulation Techniques
Now that we’ve covered the basics, let’s explore some common techniques used in bit manipulation problems. These techniques form the building blocks for solving more complex problems.
1. Check if a number is even or odd
We can use the AND operator to check if a number is even or odd. The least significant bit of an even number is always 0, while for an odd number, it’s 1.
bool isEven(int n) {
return (n & 1) == 0;
}
bool isOdd(int n) {
return (n & 1) == 1;
}
2. Check if the i-th bit is set
To check if the i-th bit of a number is set (1), we can use the left shift operator to create a mask and then use the AND operator.
bool isBitSet(int n, int i) {
return (n & (1 << i)) != 0;
}
3. Set the i-th bit
To set the i-th bit of a number to 1, we can use the OR operator with a mask created using the left shift operator.
int setBit(int n, int i) {
return n | (1 << i);
}
4. Clear the i-th bit
To clear (set to 0) the i-th bit of a number, we can use the AND operator with the complement of a mask created using the left shift operator.
int clearBit(int n, int i) {
return n & ~(1 << i);
}
5. Toggle the i-th bit
To toggle (flip) the i-th bit of a number, we can use the XOR operator with a mask created using the left shift operator.
int toggleBit(int n, int i) {
return n ^ (1 << i);
}
6. Count the number of set bits
Counting the number of set bits (1s) in a number is a common problem. Here’s an efficient method known as Brian Kernighan’s algorithm:
int countSetBits(int n) {
int count = 0;
while (n) {
n &= (n - 1);
count++;
}
return count;
}
This algorithm works by repeatedly clearing the least significant set bit until the number becomes 0.
Approaching Bit Manipulation Problems
Now that we’ve covered the basics and some common techniques, let’s discuss strategies for approaching bit manipulation problems in coding interviews.
1. Understand the problem thoroughly
Before diving into the solution, make sure you fully understand the problem statement. Ask clarifying questions if needed. Some key points to consider:
- What is the range of input values?
- Are there any constraints on time or space complexity?
- Are there any special cases or edge cases to consider?
2. Visualize the binary representation
When dealing with bit manipulation problems, it’s often helpful to visualize the binary representation of the numbers involved. Write out the binary representation of example inputs and outputs to gain insights into the problem.
3. Identify relevant bit patterns
Look for patterns in the binary representation that might be useful for solving the problem. For example:
- Are all bits set (1111…)?
- Is there a specific pattern of alternating bits?
- Are there any repeating subsequences of bits?
4. Consider using standard bit manipulation techniques
Many bit manipulation problems can be solved using a combination of the standard techniques we discussed earlier. Consider if any of these techniques are applicable to your problem:
- Setting, clearing, or toggling specific bits
- Counting set bits
- Using masks to isolate specific bits
- Shifting bits to multiply or divide by powers of 2
5. Think about the properties of bitwise operations
Remember the properties of bitwise operations and how they might be useful in solving the problem. For example:
- XOR of a number with itself is 0
- XOR of a number with 0 is the number itself
- AND of a number with a power of 2 minus 1 gives the remainder when divided by that power of 2
6. Consider edge cases
Don’t forget to consider edge cases in your solution. Some common edge cases in bit manipulation problems include:
- Dealing with negative numbers (if applicable)
- Handling the maximum or minimum possible values for the given data type
- Dealing with all bits set or all bits clear
7. Optimize your solution
Once you have a working solution, consider if there are ways to optimize it. Bit manipulation operations are generally very fast, but there might be ways to reduce the number of operations or improve the space complexity.
Examples of Bit Manipulation Problems
Let’s look at some example problems and how to approach them using the strategies we’ve discussed.
Problem 1: Find the single number
Problem statement: Given an array of integers where every element appears twice except for one element which appears only once, find that single element.
Approach:
- Understand the problem: We need to find the unique element in an array where all other elements appear exactly twice.
- Visualize: Not particularly helpful in this case, as we’re dealing with an array of numbers.
- Identify relevant bit patterns: No specific patterns, but we can use the properties of XOR.
- Use standard techniques: XOR of all elements will give us the unique element.
- Properties of bitwise operations: XOR of a number with itself is 0, and XOR of a number with 0 is the number itself.
Solution:
int findSingleNumber(vector<int>& nums) {
int result = 0;
for (int num : nums) {
result ^= num;
}
return result;
}
This solution works because XORing all numbers will cancel out the pairs, leaving only the unique number.
Problem 2: Reverse bits of an integer
Problem statement: Reverse the bits of a given 32-bit unsigned integer.
Approach:
- Understand the problem: We need to reverse the order of bits in a 32-bit integer.
- Visualize: Consider an example like 43261596 (00000010100101000001111010011100 in binary).
- Identify relevant bit patterns: We need to swap bits from left to right.
- Use standard techniques: We can use bit shifting and masking.
- Properties of bitwise operations: No specific properties needed for this problem.
Solution:
uint32_t reverseBits(uint32_t n) {
uint32_t result = 0;
for (int i = 0; i < 32; i++) {
result = (result << 1) | (n & 1);
n >>= 1;
}
return result;
}
This solution works by iterating through each bit of the input number, extracting the least significant bit, and adding it to the result (which is shifted left each time).
Problem 3: Count the number of different bits between two integers
Problem statement: Given two integers, count the number of bits that are different at the same positions in their binary representations.
Approach:
- Understand the problem: We need to count the positions where the binary representations of two numbers differ.
- Visualize: Consider examples like 1 (001) and 4 (100) – they differ in all three positions.
- Identify relevant bit patterns: We’re looking for differences in corresponding bits.
- Use standard techniques: XOR can be used to identify different bits, then we can count the set bits.
- Properties of bitwise operations: XOR of two numbers will have set bits where they differ.
Solution:
int countDifferentBits(int a, int b) {
return countSetBits(a ^ b);
}
int countSetBits(int n) {
int count = 0;
while (n) {
n &= (n - 1);
count++;
}
return count;
}
This solution first XORs the two numbers to get a number where the set bits represent the positions where the original numbers differed. Then it counts the set bits in this result.
Advanced Bit Manipulation Techniques
As you become more comfortable with basic bit manipulation, you can explore more advanced techniques that are often useful in solving complex problems:
1. Isolating the rightmost set bit
To isolate the rightmost set bit of a number, you can use the expression n & (-n)
. This is useful in many algorithms, including those dealing with binary indexed trees (Fenwick trees).
int isolateRightmostSetBit(int n) {
return n & (-n);
}
2. Removing the rightmost set bit
To remove the rightmost set bit of a number, you can use the expression n & (n - 1)
. This is the key operation in Brian Kernighan’s algorithm for counting set bits.
int removeRightmostSetBit(int n) {
return n & (n - 1);
}
3. Generating all subsets of a set
Bit manipulation can be used to generate all subsets of a set efficiently. Each subset can be represented by a bit mask where a set bit indicates the presence of an element.
vector<vector<int>> generateSubsets(vector<int>& nums) {
int n = nums.size();
vector<vector<int>> subsets;
for (int i = 0; i < (1 << n); i++) {
vector<int> subset;
for (int j = 0; j < n; j++) {
if (i & (1 << j)) {
subset.push_back(nums[j]);
}
}
subsets.push_back(subset);
}
return subsets;
}
4. Swapping values without using a temporary variable
XOR can be used to swap two values without using a temporary variable:
void swapWithoutTemp(int& a, int& b) {
a ^= b;
b ^= a;
a ^= b;
}
Common Pitfalls and How to Avoid Them
When working with bit manipulation, there are several common pitfalls that you should be aware of:
1. Ignoring the sign bit
When working with signed integers, be careful not to ignore the sign bit. Operations like right shift behave differently for signed and unsigned integers in many programming languages.
2. Overflow and underflow
Be cautious of overflow and underflow when performing bit operations, especially when shifting bits. For example, left-shifting a 1 by 31 positions in a 32-bit integer can lead to unexpected results.
3. Assuming a specific bit representation
Don’t assume a specific bit representation for negative numbers. While two’s complement is common, it’s not guaranteed by all systems.
4. Forgetting about endianness
When working with multi-byte data types, remember that different systems may use different endianness (byte order).
5. Overcomplicating solutions
While bit manipulation can lead to efficient solutions, sometimes a straightforward approach is clearer and less error-prone. Don’t use bit manipulation just for the sake of it.
Conclusion
Mastering bit manipulation is a valuable skill for any programmer, especially those preparing for coding interviews at top tech companies. By understanding the fundamental operations, common techniques, and problem-solving strategies, you’ll be well-equipped to tackle a wide range of bit manipulation problems.
Remember, the key to success with bit manipulation problems is practice. Start with simple problems and gradually work your way up to more complex ones. Visualize the binary representations, identify patterns, and always consider the properties of bitwise operations.
As you continue to develop your skills, you’ll find that bit manipulation can often lead to elegant and efficient solutions to various programming problems. Keep practicing, stay curious, and don’t hesitate to explore more advanced bit manipulation techniques as you grow more comfortable with the basics.
With dedication and consistent practice, you’ll be well-prepared to tackle any bit manipulation problem that comes your way in your coding interviews and beyond. Good luck with your coding journey!