Understanding Recursion and Its Applications in Coding Problems
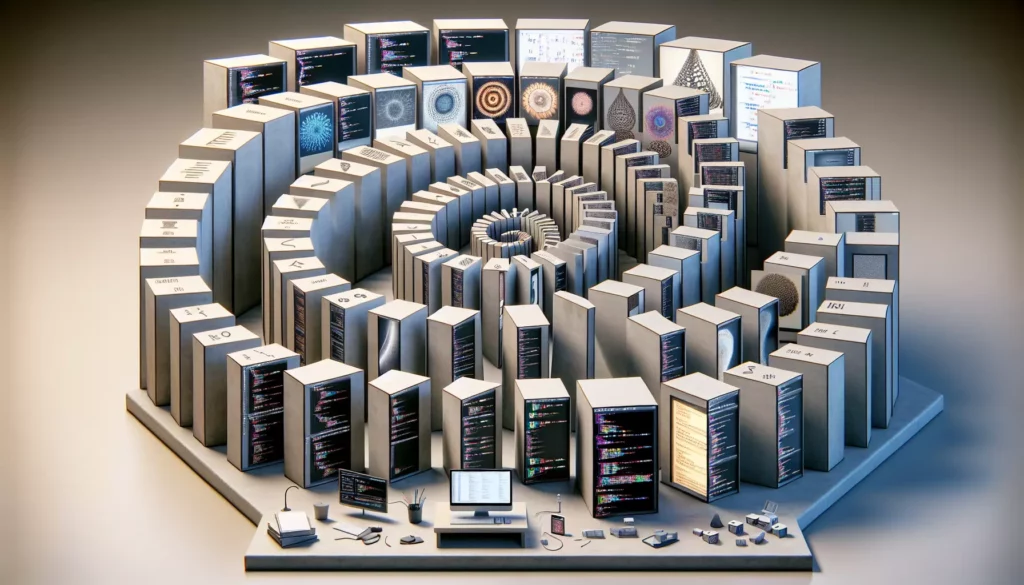
Recursion is a fundamental concept in computer science and programming that often challenges beginners but proves to be an incredibly powerful tool when mastered. In this comprehensive guide, we’ll dive deep into the world of recursion, exploring its core principles, applications, and how it can be leveraged to solve complex coding problems. Whether you’re preparing for technical interviews at top tech companies or simply looking to enhance your programming skills, understanding recursion is crucial for your growth as a developer.
What is Recursion?
At its core, recursion is a problem-solving technique where a function calls itself to solve a smaller instance of the same problem. This process continues until a base case is reached, at which point the function stops calling itself and begins to return results back up the chain of recursive calls.
To understand recursion, it’s helpful to break it down into two essential components:
- Base Case: This is the condition that stops the recursion. It’s typically a simple scenario where the function can return a result without making any further recursive calls.
- Recursive Case: This is where the function calls itself with a modified input, typically moving closer to the base case with each call.
The Anatomy of a Recursive Function
A typical recursive function follows this general structure:
function recursiveFunction(input) {
if (baseCondition) {
return baseResult;
} else {
// Recursive case
return recursiveFunction(modifiedInput);
}
}
Let’s break this down:
- The function first checks if the base condition is met.
- If the base condition is true, it returns the base result without any further recursive calls.
- If the base condition is false, the function calls itself with a modified input, moving closer to the base case.
A Simple Example: Factorial Calculation
One of the classic examples used to introduce recursion is calculating the factorial of a number. The factorial of a non-negative integer n, denoted as n!, is the product of all positive integers less than or equal to n.
Here’s how we can implement a factorial function recursively:
function factorial(n) {
// Base case
if (n === 0 || n === 1) {
return 1;
}
// Recursive case
return n * factorial(n - 1);
}
Let’s break down how this works:
- The base case is when n is 0 or 1, in which case the factorial is 1.
- For any other positive integer, we multiply n by the factorial of (n-1).
- This process continues, with each recursive call reducing n by 1, until we reach the base case.
The Power of Recursion: Solving Complex Problems
While the factorial example is straightforward, recursion truly shines when dealing with more complex problems. Let’s explore some areas where recursion is particularly useful:
1. Tree and Graph Traversal
Recursion is a natural fit for traversing tree-like structures or graphs. Consider a binary tree traversal:
function inorderTraversal(node) {
if (node === null) {
return;
}
inorderTraversal(node.left);
console.log(node.value);
inorderTraversal(node.right);
}
This recursive function elegantly handles the complex task of traversing a binary tree in-order, visiting the left subtree, then the current node, and finally the right subtree.
2. Divide and Conquer Algorithms
Many efficient algorithms use a divide-and-conquer approach, which is inherently recursive. A classic example is the Merge Sort algorithm:
function mergeSort(arr) {
if (arr.length <= 1) {
return arr;
}
const mid = Math.floor(arr.length / 2);
const left = arr.slice(0, mid);
const right = arr.slice(mid);
return merge(mergeSort(left), mergeSort(right));
}
function merge(left, right) {
let result = [];
let leftIndex = 0;
let rightIndex = 0;
while (leftIndex < left.length && rightIndex < right.length) {
if (left[leftIndex] < right[rightIndex]) {
result.push(left[leftIndex]);
leftIndex++;
} else {
result.push(right[rightIndex]);
rightIndex++;
}
}
return result.concat(left.slice(leftIndex)).concat(right.slice(rightIndex));
}
Merge Sort recursively divides the array into smaller subarrays, sorts them, and then merges them back together.
3. Backtracking
Backtracking is a recursive technique used to build a solution incrementally, abandoning solutions that fail to meet the problem’s constraints. It’s particularly useful for solving puzzles, generating permutations, and more.
Here’s an example of generating all possible subsets of a set using backtracking:
function subsets(nums) {
const result = [];
function backtrack(start, current) {
result.push([...current]);
for (let i = start; i < nums.length; i++) {
current.push(nums[i]);
backtrack(i + 1, current);
current.pop();
}
}
backtrack(0, []);
return result;
}
This function generates all possible subsets by recursively including or excluding each element.
Common Pitfalls and How to Avoid Them
While recursion is powerful, it comes with its own set of challenges. Here are some common pitfalls and how to address them:
1. Stack Overflow
Recursive functions can lead to stack overflow errors if they make too many nested calls. To avoid this:
- Ensure your base case is reachable and correct.
- Consider using tail recursion optimization where possible.
- For very deep recursions, consider converting to an iterative approach or using a stack-based solution.
2. Redundant Calculations
Naive recursive implementations can sometimes perform the same calculations multiple times. To optimize:
- Use memoization to store and reuse results of expensive function calls.
- Consider a bottom-up dynamic programming approach for problems with overlapping subproblems.
3. Incorrect Base Case
An incorrect or missing base case can lead to infinite recursion. Always ensure that:
- Your base case is correctly defined and covers all terminating scenarios.
- The recursive case moves towards the base case with each call.
Advanced Recursion Techniques
As you become more comfortable with basic recursion, you can explore more advanced techniques:
1. Tail Recursion
Tail recursion is a special form of recursion where the recursive call is the last operation in the function. Some compilers can optimize tail-recursive functions to use constant stack space.
function tailFactorial(n, accumulator = 1) {
if (n === 0 || n === 1) {
return accumulator;
}
return tailFactorial(n - 1, n * accumulator);
}
2. Mutual Recursion
Mutual recursion involves two or more functions calling each other recursively. This can be useful for problems that have a natural back-and-forth structure.
function isEven(n) {
if (n === 0) return true;
return isOdd(Math.abs(n) - 1);
}
function isOdd(n) {
if (n === 0) return false;
return isEven(Math.abs(n) - 1);
}
3. Recursion with Memoization
Memoization can significantly improve the performance of recursive functions that have overlapping subproblems. Here’s an example with the Fibonacci sequence:
function fibonacciMemo(n, memo = {}) {
if (n in memo) return memo[n];
if (n <= 1) return n;
memo[n] = fibonacciMemo(n - 1, memo) + fibonacciMemo(n - 2, memo);
return memo[n];
}
Practical Applications in Coding Interviews
Understanding recursion is crucial for technical interviews, especially at top tech companies. Here are some common types of problems where recursion can be effectively applied:
1. String Manipulation
Recursion can be powerful for tasks like generating all permutations of a string or validating palindromes.
function isPalindrome(str) {
if (str.length <= 1) return true;
if (str[0] !== str[str.length - 1]) return false;
return isPalindrome(str.slice(1, -1));
}
2. Dynamic Programming
Many dynamic programming problems can be initially approached using recursion with memoization before being optimized to a bottom-up approach.
3. Combinatorial Problems
Generating combinations, permutations, or subsets often involves recursive backtracking.
4. Tree and Graph Algorithms
Problems involving tree traversal, path finding, or graph exploration are natural fits for recursive solutions.
Tips for Mastering Recursion
To become proficient with recursion:
- Start Simple: Begin with basic problems like factorial or Fibonacci and gradually move to more complex scenarios.
- Visualize the Call Stack: Draw out the recursive calls and their returns to understand the flow of execution.
- Identify the Base Case: Always start by clearly defining when the recursion should stop.
- Trust the Recursion: Once you’ve handled the base case and set up the recursive call correctly, trust that the smaller subproblems will be solved correctly.
- Practice Regularly: Recursion is a skill that improves with practice. Solve a variety of recursive problems to build your intuition.
- Analyze Time and Space Complexity: Understanding the performance implications of your recursive solutions is crucial for optimization.
Conclusion
Recursion is a powerful problem-solving technique that forms the backbone of many elegant and efficient algorithms. While it may seem daunting at first, with practice and understanding, it becomes an invaluable tool in a programmer’s arsenal. Whether you’re tackling complex data structures, designing algorithms, or preparing for technical interviews, a solid grasp of recursion will serve you well.
Remember, the key to mastering recursion lies in understanding its fundamental principles, recognizing when to apply it, and practicing with a variety of problems. As you continue your journey in programming and computer science, you’ll find that recursion opens up new ways of thinking about and solving problems, enabling you to write more elegant, efficient, and powerful code.
Keep exploring, keep practicing, and embrace the recursive mindset. Happy coding!