How to Optimize Code for Space and Time Complexity: A Comprehensive Guide
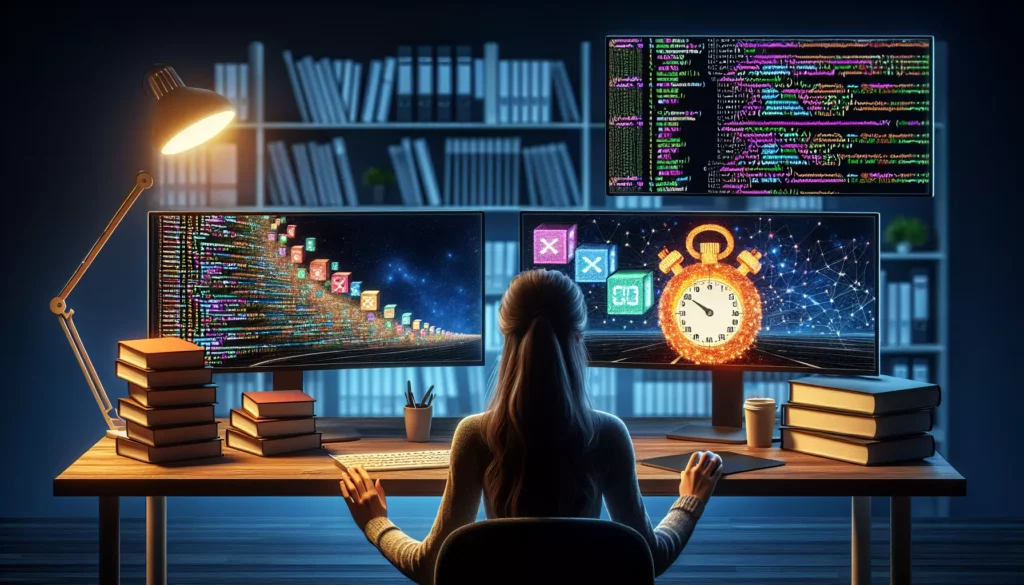
In the world of software development, writing code that works is just the first step. To create truly efficient and scalable applications, developers must optimize their code for both space and time complexity. This process, known as code optimization, is a crucial skill for programmers at all levels, from beginners to those preparing for technical interviews at major tech companies. In this comprehensive guide, we’ll explore the concepts of space and time complexity, and provide practical strategies to optimize your code for better performance.
Understanding Space and Time Complexity
Before diving into optimization techniques, it’s essential to understand what space and time complexity mean in the context of programming:
Time Complexity
Time complexity refers to the amount of time an algorithm takes to complete as a function of the input size. It’s typically expressed using Big O notation, which describes the upper bound of an algorithm’s growth rate. Common time complexities include:
- O(1) – Constant time
- O(log n) – Logarithmic time
- O(n) – Linear time
- O(n log n) – Linearithmic time
- O(n^2) – Quadratic time
- O(2^n) – Exponential time
Space Complexity
Space complexity refers to the amount of memory an algorithm uses relative to the input size. Like time complexity, it’s also expressed using Big O notation. Common space complexities include:
- O(1) – Constant space
- O(n) – Linear space
- O(n^2) – Quadratic space
Now that we have a basic understanding of these concepts, let’s explore strategies to optimize code for both time and space complexity.
Strategies for Optimizing Time Complexity
1. Choose the Right Data Structures
Selecting appropriate data structures can significantly impact the time complexity of your code. For example:
- Use hash tables (dictionaries in Python, objects in JavaScript) for fast lookups (O(1) average case)
- Use balanced binary search trees (like Red-Black trees) for ordered data with O(log n) operations
- Use arrays or linked lists for sequential access patterns
Example of using a hash table for fast lookups:
def find_duplicate(arr):
seen = {}
for num in arr:
if num in seen:
return num
seen[num] = True
return None
2. Implement Efficient Algorithms
Choosing the right algorithm can dramatically improve time complexity. Some examples include:
- Use quicksort or mergesort (O(n log n)) instead of bubble sort (O(n^2)) for sorting
- Implement binary search (O(log n)) instead of linear search (O(n)) for sorted data
- Use dynamic programming to avoid redundant calculations in recursive problems
Example of binary search implementation:
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
3. Avoid Nested Loops When Possible
Nested loops often lead to quadratic time complexity (O(n^2)). Try to reduce the number of nested loops or find alternative approaches. For example, instead of using two nested loops to find a pair of numbers that sum to a target, you can use a hash table:
def find_pair_sum(arr, target):
complement_map = {}
for num in arr:
if num in complement_map:
return (complement_map[num], num)
complement_map[target - num] = num
return None
4. Use Memoization and Caching
Memoization is a technique used to store the results of expensive function calls and return the cached result when the same inputs occur again. This can significantly reduce time complexity in recursive algorithms or functions that are called multiple times with the same arguments.
Example of memoization in a recursive Fibonacci function:
def fibonacci(n, memo={}):
if n in memo:
return memo[n]
if n <= 1:
return n
memo[n] = fibonacci(n-1, memo) + fibonacci(n-2, memo)
return memo[n]
5. Utilize Lazy Evaluation
Lazy evaluation is a strategy where you delay the evaluation of an expression until its value is needed. This can be particularly useful when dealing with large datasets or infinite sequences. In Python, you can use generators to implement lazy evaluation:
def infinite_sequence():
num = 0
while True:
yield num
num += 1
# Using the infinite sequence
for i in infinite_sequence():
print(i)
if i >= 10:
break
Strategies for Optimizing Space Complexity
1. Minimize Auxiliary Space Usage
Try to solve problems using as little extra space as possible. In-place algorithms that modify the input directly without using additional data structures can help reduce space complexity.
Example of in-place array reversal:
def reverse_array(arr):
left, right = 0, len(arr) - 1
while left < right:
arr[left], arr[right] = arr[right], arr[left]
left += 1
right -= 1
return arr
2. Use Generators for Large Datasets
When working with large datasets, generators can help reduce memory usage by yielding values one at a time instead of storing the entire dataset in memory.
def read_large_file(file_path):
with open(file_path, 'r') as file:
for line in file:
yield line.strip()
# Using the generator
for line in read_large_file('large_file.txt'):
process_line(line)
3. Use Bit Manipulation Techniques
Bit manipulation can be used to reduce space complexity in certain scenarios, especially when dealing with sets of integers or boolean flags.
Example of using bit manipulation to check if a number is even or odd:
def is_even(num):
return not (num & 1)
# Using the function
print(is_even(4)) # True
print(is_even(7)) # False
4. Reuse Variables and Data Structures
Instead of creating new variables or data structures in loops or recursive calls, try to reuse existing ones when possible.
def matrix_multiplication(A, B):
rows_A, cols_A = len(A), len(A[0])
rows_B, cols_B = len(B), len(B[0])
if cols_A != rows_B:
raise ValueError("Incompatible matrix dimensions")
result = [[0 for _ in range(cols_B)] for _ in range(rows_A)]
for i in range(rows_A):
for j in range(cols_B):
for k in range(cols_A):
result[i][j] += A[i][k] * B[k][j]
return result
5. Use Appropriate Data Types
Choose data types that use the least amount of memory for your specific use case. For example, use integers instead of floats when dealing with whole numbers, or use smaller integer types (e.g., int8, int16) when the range of values is limited.
import numpy as np
# Using appropriate data types in NumPy arrays
small_numbers = np.array([1, 2, 3, 4, 5], dtype=np.int8)
large_numbers = np.array([1000000, 2000000, 3000000], dtype=np.int32)
Advanced Optimization Techniques
1. Parallel Processing and Multithreading
For computationally intensive tasks, utilizing parallel processing or multithreading can significantly improve performance. While this doesn’t necessarily reduce time complexity, it can greatly reduce actual execution time.
import concurrent.futures
def process_item(item):
# Perform some computation
return item * item
def parallel_processing(items):
with concurrent.futures.ProcessPoolExecutor() as executor:
results = list(executor.map(process_item, items))
return results
# Usage
items = range(1000000)
results = parallel_processing(items)
2. Use Built-in Functions and Libraries
Many programming languages and libraries provide optimized built-in functions that are faster than custom implementations. Whenever possible, use these built-in functions to improve performance.
import numpy as np
# Using NumPy for fast array operations
arr1 = np.array([1, 2, 3, 4, 5])
arr2 = np.array([6, 7, 8, 9, 10])
# Fast element-wise multiplication
result = arr1 * arr2
3. Profiling and Benchmarking
Use profiling tools to identify bottlenecks in your code and focus your optimization efforts on the most critical parts. Python’s built-in cProfile module is a great tool for this purpose:
import cProfile
def expensive_function():
result = 0
for i in range(1000000):
result += i
return result
cProfile.run('expensive_function()')
4. Algorithmic Improvements
Sometimes, the best optimization comes from rethinking the problem and finding a completely different approach. For example, using the Fast Fourier Transform (FFT) algorithm to multiply large integers instead of the traditional grade-school multiplication method.
5. Trade-offs Between Time and Space
In some cases, you may need to make trade-offs between time and space complexity. For example, using more memory to store precomputed results (memoization) can lead to faster execution times. Consider the specific requirements of your application when making these trade-offs.
Conclusion
Optimizing code for space and time complexity is a crucial skill for any programmer, especially those preparing for technical interviews at top tech companies. By understanding the concepts of time and space complexity and applying the strategies outlined in this guide, you can significantly improve the efficiency and scalability of your code.
Remember that optimization is an iterative process. Start by writing clear, correct code, and then optimize incrementally. Use profiling tools to identify bottlenecks, and always consider the specific requirements of your application when making optimization decisions.
As you continue to practice and refine your skills, you’ll develop an intuition for writing efficient code from the start. Keep challenging yourself with complex problems, and don’t hesitate to explore advanced topics like parallel processing and algorithmic improvements.
By mastering these optimization techniques, you’ll not only be better prepared for technical interviews but also become a more valuable developer capable of creating high-performance, scalable applications in your professional career.