Approaching Algorithms and Data Structures in a Language-Agnostic Way
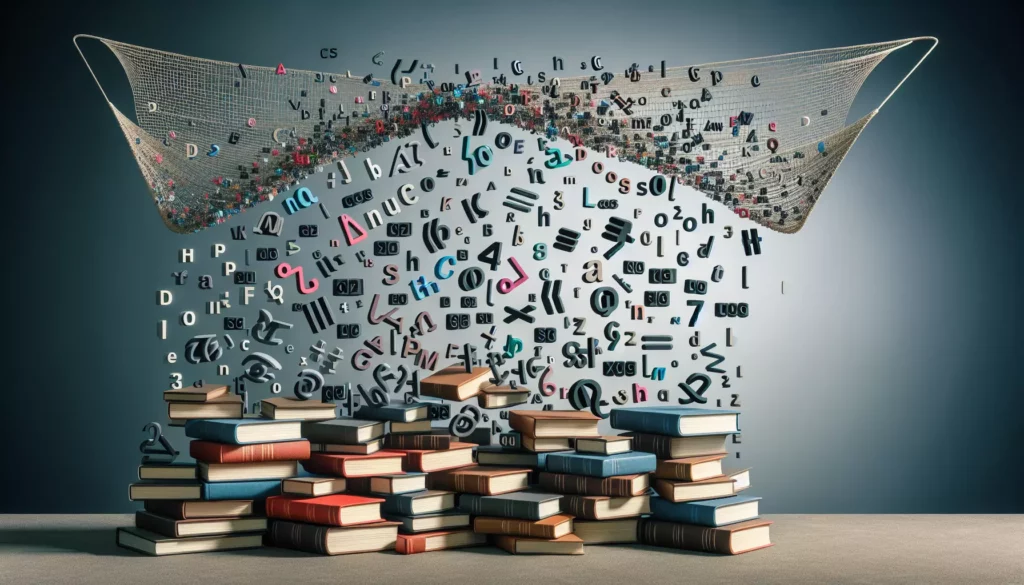
In the world of programming and software development, mastering algorithms and data structures is crucial for solving complex problems efficiently and optimizing code performance. While many programmers tend to learn these concepts within the context of a specific programming language, there’s immense value in approaching algorithms and data structures in a language-agnostic way. This approach not only broadens your understanding but also makes you a more versatile and adaptable programmer. In this comprehensive guide, we’ll explore the benefits of language-agnostic learning and provide strategies for mastering algorithms and data structures independently of any particular programming language.
Why Take a Language-Agnostic Approach?
Before diving into the strategies, let’s understand why a language-agnostic approach to algorithms and data structures is beneficial:
- Transferable Knowledge: When you understand algorithms and data structures conceptually, you can apply this knowledge across different programming languages.
- Focus on Logic: By removing language-specific syntax from the equation, you can concentrate on the underlying logic and problem-solving aspects.
- Improved Problem-Solving Skills: A language-agnostic approach encourages thinking in terms of abstract concepts, enhancing your overall problem-solving abilities.
- Better Preparation for Interviews: Many technical interviews, especially for major tech companies, focus on algorithmic thinking rather than language-specific implementations.
- Adaptability: As new programming languages emerge, your foundational knowledge of algorithms and data structures will remain relevant and easily applicable.
Strategies for Language-Agnostic Learning
1. Start with Pseudocode
Pseudocode is a method of describing algorithms using plain language and simple, generic constructs. It’s an excellent tool for thinking through problems without getting bogged down in language-specific syntax.
Example of bubble sort in pseudocode:
procedure bubbleSort(A: list of sortable items)
n = length(A)
repeat
swapped = false
for i = 1 to n-1 inclusive do
if A[i-1] > A[i] then
swap(A[i-1], A[i])
swapped = true
end if
end for
n = n - 1
until not swapped
end procedure
2. Visualize Algorithms and Data Structures
Visual representations can help you understand how algorithms work and how data structures are organized, regardless of the programming language used. Tools and resources for visualization include:
- Flowcharts
- Diagrams
- Animation tools (e.g., VisuAlgo, Algorithm Visualizer)
- Whiteboarding exercises
3. Focus on Time and Space Complexity
Understanding the efficiency of algorithms in terms of time and space complexity is crucial and language-independent. Learn to analyze algorithms using Big O notation, which describes the upper bound of an algorithm’s growth rate.
For example, the time complexity of the bubble sort algorithm is:
- Best Case: O(n) when the array is already sorted
- Average Case: O(n^2)
- Worst Case: O(n^2)
4. Study Abstract Data Types (ADTs)
Abstract Data Types are high-level descriptions of data structures that specify what operations can be performed on the data, without detailing how these operations are implemented. Common ADTs include:
- List
- Stack
- Queue
- Tree
- Graph
- Hash Table
By understanding ADTs, you can grasp the conceptual model of data structures without being tied to a specific implementation or language.
5. Implement Algorithms in Multiple Languages
While the goal is to think language-agnostically, implementing the same algorithm in multiple languages can reinforce your understanding and highlight the universal nature of algorithmic concepts. This practice also helps you appreciate the strengths and limitations of different languages.
6. Use Algorithmic Design Patterns
Familiarize yourself with common algorithmic design patterns that can be applied across various problems and languages. Some key patterns include:
- Divide and Conquer
- Dynamic Programming
- Greedy Algorithms
- Backtracking
- Branch and Bound
7. Practice with Coding Challenges
Engage in coding challenges and competitive programming platforms that allow submissions in multiple languages. Focus on understanding the problem and devising a solution before choosing a language for implementation. Popular platforms include:
- LeetCode
- HackerRank
- CodeForces
- Project Euler
Language-Agnostic Approach to Common Data Structures
Let’s explore how to approach some common data structures in a language-agnostic manner:
Arrays
Conceptually, an array is a collection of elements stored at contiguous memory locations. Key points to understand:
- Random access (constant time)
- Fixed size in many languages
- Time complexity for common operations:
- Access: O(1)
- Search: O(n)
- Insertion/Deletion at end: O(1)
- Insertion/Deletion at arbitrary position: O(n)
Linked Lists
A linked list is a linear data structure where elements are stored in nodes, and each node points to the next node in the sequence. Key concepts:
- Dynamic size
- Efficient insertion and deletion
- Time complexity for common operations:
- Access: O(n)
- Search: O(n)
- Insertion at beginning: O(1)
- Deletion at beginning: O(1)
Stacks and Queues
Stacks (Last-In-First-Out) and Queues (First-In-First-Out) are abstract data types that can be implemented using arrays or linked lists. Focus on understanding their behavior and use cases rather than specific implementations.
Trees
Trees are hierarchical data structures with a root node and child nodes. Key types to understand include:
- Binary Trees
- Binary Search Trees (BST)
- AVL Trees
- Red-Black Trees
For each type, focus on the properties, balancing mechanisms, and time complexities for operations like search, insertion, and deletion.
Graphs
Graphs consist of vertices (nodes) and edges connecting these vertices. Key concepts to grasp:
- Directed vs Undirected graphs
- Weighted vs Unweighted graphs
- Representation methods (Adjacency Matrix, Adjacency List)
- Graph traversal algorithms (BFS, DFS)
- Shortest path algorithms (Dijkstra’s, Bellman-Ford)
Hash Tables
Hash tables provide efficient key-value pair storage and retrieval. Focus on:
- Hash function concepts
- Collision resolution techniques (Chaining, Open Addressing)
- Time complexity analysis (average case vs worst case)
Language-Agnostic Approach to Common Algorithms
Now, let’s look at how to approach some fundamental algorithms in a language-agnostic way:
Sorting Algorithms
For each sorting algorithm, focus on:
- The core idea and steps
- Time and space complexity
- Best, average, and worst-case scenarios
- Stability
Key sorting algorithms to study:
- Bubble Sort
- Selection Sort
- Insertion Sort
- Merge Sort
- Quick Sort
- Heap Sort
Search Algorithms
Understand the principles behind:
- Linear Search
- Binary Search
- Depth-First Search (DFS)
- Breadth-First Search (BFS)
Focus on the algorithms’ logic, time complexity, and appropriate use cases.
Dynamic Programming
Dynamic Programming is a method for solving complex problems by breaking them down into simpler subproblems. Key concepts to grasp:
- Optimal Substructure
- Overlapping Subproblems
- Memoization vs Tabulation
Practice with classic dynamic programming problems like:
- Fibonacci Sequence
- Longest Common Subsequence
- Knapsack Problem
Greedy Algorithms
Greedy algorithms make locally optimal choices at each step. Understand:
- The greedy choice property
- Optimal substructure
- When greedy algorithms are applicable
Study classic greedy problems like:
- Activity Selection
- Huffman Coding
- Dijkstra’s Shortest Path Algorithm
Practical Tips for Language-Agnostic Learning
1. Create Conceptual Flowcharts
When learning a new algorithm or data structure, start by creating a flowchart or diagram that represents the concept visually. This helps in understanding the logic flow without getting caught up in code syntax.
2. Implement Abstract Data Types
Practice implementing ADTs using basic constructs available in most languages. For example, implement a stack using only arrays and basic operations, focusing on the LIFO principle rather than language-specific features.
3. Analyze Real-World Scenarios
Look for algorithms and data structures in real-world applications. For instance, analyze how a GPS navigation system might use graph algorithms to find the shortest path, or how a database might use hash tables for efficient data retrieval.
4. Participate in Algorithm Discussions
Join online forums or local meetups where algorithms and data structures are discussed. Engage in language-agnostic discussions about problem-solving approaches, algorithm efficiency, and trade-offs between different solutions.
5. Build a Personal Algorithm Library
Create a personal repository of algorithm and data structure implementations. For each entry, include:
- A language-agnostic description of the algorithm/data structure
- Pseudocode
- Time and space complexity analysis
- Example use cases
- Implementations in multiple languages (if desired)
6. Practice Whiteboarding
Regularly practice solving problems on a whiteboard or paper, focusing on high-level design and pseudocode before diving into any specific programming language. This mimics many technical interview scenarios and reinforces language-agnostic thinking.
7. Teach Others
Try explaining algorithms and data structures to others without relying on code examples. This exercise forces you to understand the concepts deeply enough to communicate them in plain language.
Conclusion
Approaching algorithms and data structures in a language-agnostic way is a powerful method for developing strong, transferable programming skills. By focusing on core concepts, logic, and problem-solving strategies rather than language-specific implementations, you’ll build a solid foundation that serves you well across different programming languages and paradigms.
Remember, the goal is not to ignore programming languages entirely, but rather to develop a deep, conceptual understanding that transcends any single language. As you progress in your learning journey, you’ll find that this approach not only makes you a more versatile programmer but also enhances your ability to choose the right tool for each task and to adapt quickly to new technologies and challenges in the ever-evolving field of software development.
By mastering algorithms and data structures in this language-agnostic manner, you’ll be well-prepared for technical interviews, complex problem-solving scenarios, and the diverse challenges of real-world software development. Keep practicing, stay curious, and always strive to understand the underlying principles that make our digital world function.