5 Essential Tips for Improving Problem-Solving Speed in Coding Interviews
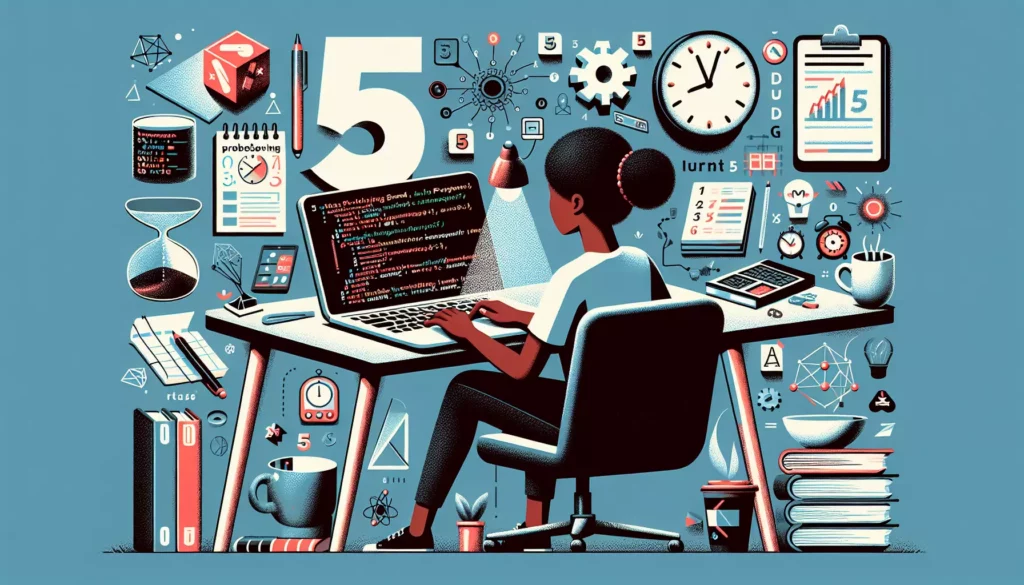
In the competitive world of tech interviews, particularly for positions at FAANG (Facebook, Amazon, Apple, Netflix, Google) companies, your ability to solve coding problems quickly and efficiently can make or break your chances of landing that dream job. While having a solid foundation in data structures and algorithms is crucial, the speed at which you can apply this knowledge is equally important. In this comprehensive guide, we’ll explore five essential tips to help you improve your problem-solving speed for coding interviews, giving you the edge you need to succeed.
1. Master the Fundamentals
Before diving into speed-enhancing techniques, it’s crucial to have a strong grasp of the fundamentals. This includes:
- Data Structures: Arrays, Linked Lists, Stacks, Queues, Trees, Graphs, Hash Tables
- Algorithms: Sorting, Searching, Recursion, Dynamic Programming, Greedy Algorithms
- Time and Space Complexity Analysis
- Basic Programming Concepts: Variables, Loops, Conditionals, Functions
Without a solid foundation, attempting to solve problems quickly will likely lead to errors and confusion. Spend time reinforcing these concepts through regular practice and study.
Practice Tip:
Use platforms like AlgoCademy to work through interactive tutorials and coding challenges that focus on these fundamental concepts. The step-by-step guidance and AI-powered assistance can help you build a strong foundation more efficiently than self-study alone.
2. Develop a Systematic Approach
Having a structured approach to problem-solving can significantly improve your speed and accuracy. Follow these steps for each problem:
- Understand the problem: Read the question carefully and ask clarifying questions if needed.
- Identify the inputs and outputs: Clearly define what you’re given and what you need to produce.
- Consider edge cases: Think about potential special cases or extreme inputs.
- Brainstorm solutions: Consider multiple approaches before settling on one.
- Plan your approach: Outline your solution in pseudocode or high-level steps.
- Implement the solution: Write clean, efficient code.
- Test and debug: Run through test cases and fix any issues.
- Optimize: If time allows, consider ways to improve your solution.
By following this systematic approach, you’ll avoid getting stuck or wasting time on unproductive paths. With practice, you’ll be able to move through these steps more quickly and efficiently.
Implementation Example:
Let’s apply this approach to a simple problem: reversing a string.
// 1. Understand the problem: We need to reverse a given string.
// 2. Identify inputs and outputs: Input is a string, output is the reversed string.
// 3. Consider edge cases: Empty string, single character, special characters.
// 4. Brainstorm solutions: Use a stack, two-pointer approach, built-in functions.
// 5. Plan the approach: We'll use a two-pointer approach for efficiency.
// 6. Implement the solution:
function reverseString(s) {
const arr = s.split('');
let left = 0;
let right = arr.length - 1;
while (left < right) {
// Swap characters
[arr[left], arr[right]] = [arr[right], arr[left]];
left++;
right--;
}
return arr.join('');
}
// 7. Test and debug:
console.log(reverseString('hello')); // Should output: 'olleh'
console.log(reverseString('')); // Should output: ''
console.log(reverseString('a')); // Should output: 'a'
// 8. Optimize: This solution is already O(n) time and O(1) space (excluding the string-to-array conversion),
// which is optimal for this problem.
3. Practice Time Management
In coding interviews, time is of the essence. You need to balance thoroughness with speed. Here are some strategies to improve your time management:
- Use a timer: When practicing, set a timer for each problem to simulate interview conditions.
- Allocate time wisely: Spend about 5-10 minutes understanding the problem and planning your approach, 20-30 minutes coding, and 5-10 minutes testing and optimizing.
- Know when to move on: If you’re stuck on a particular part of the problem, make a note and move on to other parts you can solve.
- Practice estimating time: Before solving a problem, estimate how long it will take you. Compare your estimate to the actual time taken to improve your time awareness.
Time Management Exercise:
Try this exercise to improve your time management skills:
- Choose 5 coding problems of varying difficulty.
- For each problem, set a timer for 45 minutes.
- Solve the problem following the systematic approach outlined earlier.
- Record how long you spent on each step of the process.
- Analyze your results: Where did you spend too much time? Where can you improve?
Repeat this exercise regularly, aiming to become more efficient with each iteration.
4. Learn and Apply Problem-Solving Patterns
Many coding interview questions follow common patterns. By recognizing these patterns, you can quickly identify the type of problem you’re dealing with and apply the appropriate solution strategy. Some common patterns include:
- Two Pointers
- Sliding Window
- Fast and Slow Pointers
- Merge Intervals
- Cyclic Sort
- In-place Reversal of a Linked List
- Tree Breadth-First Search
- Tree Depth-First Search
- Two Heaps
- Subsets
- Modified Binary Search
- Top K Elements
- K-way Merge
- Topological Sort
Familiarizing yourself with these patterns will allow you to quickly categorize problems and apply the most efficient solution approach.
Pattern Recognition Example:
Let’s look at how recognizing a pattern can speed up problem-solving. Consider this problem:
Given an array of integers, find if the array contains any duplicates. Return true if any value appears at least twice in the array, and return false if every element is distinct.
Recognizing this as a problem that can be solved using a Hash Table pattern, we can quickly implement an efficient solution:
function containsDuplicate(nums) {
const seen = new Set();
for (const num of nums) {
if (seen.has(num)) {
return true;
}
seen.add(num);
}
return false;
}
// Test the function
console.log(containsDuplicate([1,2,3,1])); // Should output: true
console.log(containsDuplicate([1,2,3,4])); // Should output: false
By recognizing the pattern, we were able to implement a solution with O(n) time complexity and O(n) space complexity, which is optimal for this problem.
5. Build a Code Snippet Library
Creating a personal library of code snippets for common operations can significantly speed up your problem-solving process. These snippets should be well-understood, optimized, and easily adaptable to different scenarios. Some useful snippets to include in your library are:
- Array manipulation (sorting, reversing, finding max/min)
- String operations (splitting, joining, reversing)
- Basic data structure implementations (Stack, Queue, LinkedList, Tree, Graph)
- Common algorithms (Binary Search, Depth-First Search, Breadth-First Search)
- Utility functions (swapping elements, checking for primality)
Having these snippets at your fingertips allows you to focus on the unique aspects of each problem rather than reimplementing basic operations every time.
Code Snippet Example:
Here’s an example of a useful code snippet for finding the maximum and minimum values in an array:
function findMaxMin(arr) {
if (arr.length === 0) {
return { max: undefined, min: undefined };
}
let max = arr[0];
let min = arr[0];
for (let i = 1; i < arr.length; i++) {
if (arr[i] > max) {
max = arr[i];
}
if (arr[i] < min) {
min = arr[i];
}
}
return { max, min };
}
// Usage example
const numbers = [3, 1, 4, 1, 5, 9, 2, 6, 5, 3, 5];
const { max, min } = findMaxMin(numbers);
console.log(`Max: ${max}, Min: ${min}`); // Should output: Max: 9, Min: 1
Having this snippet readily available can save you time when solving problems that require finding maximum or minimum values in an array.
Putting It All Together: A Holistic Approach to Improving Problem-Solving Speed
Improving your problem-solving speed for coding interviews is not just about writing code faster; it’s about developing a comprehensive set of skills and strategies that work together to enhance your overall performance. Let’s recap how these five tips interconnect and reinforce each other:
- Master the Fundamentals: This forms the bedrock of your problem-solving abilities. Without a solid understanding of data structures, algorithms, and programming concepts, you’ll struggle to implement solutions quickly and accurately.
- Develop a Systematic Approach: This structured method helps you navigate problems efficiently, ensuring you don’t miss crucial steps or waste time on unproductive paths. It’s particularly effective when combined with strong fundamental knowledge.
- Practice Time Management: This skill helps you allocate your efforts effectively during an interview, ensuring you have time to tackle all parts of a problem. It’s enhanced by having a systematic approach and recognizing problem patterns.
- Learn and Apply Problem-Solving Patterns: Recognizing common patterns allows you to quickly categorize problems and apply proven solution strategies. This is most effective when built upon a strong foundation of fundamental knowledge.
- Build a Code Snippet Library: Having ready-to-use code snippets saves time and mental energy, allowing you to focus on the unique aspects of each problem. This library is most useful when you thoroughly understand the fundamentals and can adapt the snippets as needed.
By integrating these strategies into your practice routine, you’ll create a positive feedback loop: As you master the fundamentals, you’ll more easily recognize patterns. As you recognize patterns, you’ll develop more useful code snippets. As you use these snippets and apply a systematic approach, you’ll manage your time better. And as you manage your time better, you’ll have more opportunity to deepen your fundamental knowledge.
Conclusion: Continuous Improvement is Key
Improving your problem-solving speed for coding interviews is an ongoing process that requires dedication, practice, and reflection. Remember that speed should never come at the expense of accuracy or code quality. The goal is to become efficient in your problem-solving process while still producing correct, clean, and optimized solutions.
As you continue to practice and refine your skills, consider these additional tips:
- Seek feedback: Participate in mock interviews or coding sessions with peers to get constructive criticism on your approach and speed.
- Analyze your performance: After each practice session or real interview, reflect on what went well and what could be improved.
- Stay updated: Keep abreast of new algorithms, data structures, and problem-solving techniques in the field of computer science.
- Practice regularly: Consistency is key. Set aside dedicated time each day or week for coding practice.
- Utilize resources: Take advantage of platforms like AlgoCademy that offer structured learning paths, interactive tutorials, and AI-assisted problem-solving to accelerate your progress.
By implementing these tips and maintaining a growth mindset, you’ll be well-equipped to tackle coding interviews with confidence and speed. Remember, the journey to becoming a proficient problem-solver is a marathon, not a sprint. Embrace the learning process, celebrate your progress, and keep pushing yourself to improve. With dedication and the right strategies, you’ll be well on your way to acing those technical interviews and landing your dream job in the tech industry.