Mastering Dynamic Programming: Strategies for Coding Interviews
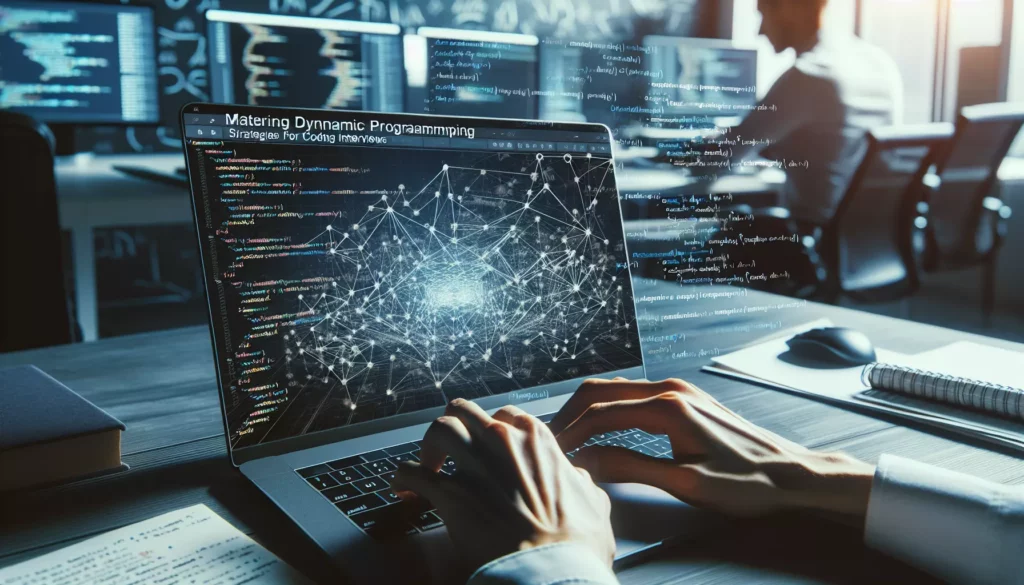
Dynamic programming (DP) is a powerful algorithmic technique that can solve complex problems by breaking them down into simpler subproblems. It’s a favorite topic in coding interviews, especially for tech giants like Google, Amazon, and Facebook. Mastering dynamic programming can significantly boost your problem-solving skills and increase your chances of acing technical interviews. In this comprehensive guide, we’ll explore various strategies for tackling dynamic programming problems in coding interviews.
Understanding Dynamic Programming
Before diving into strategies, let’s quickly recap what dynamic programming is and why it’s so important in coding interviews.
What is Dynamic Programming?
Dynamic programming is an algorithmic paradigm that solves complex problems by breaking them down into simpler subproblems. It is applicable when the problem has the following properties:
- Overlapping Subproblems: The problem can be broken down into subproblems which are reused several times.
- Optimal Substructure: The optimal solution to the problem can be constructed from optimal solutions of its subproblems.
Why is Dynamic Programming Important in Coding Interviews?
Dynamic programming is a crucial topic in coding interviews for several reasons:
- It demonstrates advanced problem-solving skills.
- It shows the ability to optimize solutions for time and space complexity.
- Many real-world problems can be solved efficiently using DP.
- It’s a common technique used in various algorithms and data structures.
Key Strategies for Dynamic Programming
Now, let’s delve into the strategies that can help you tackle dynamic programming problems effectively in coding interviews.
1. Identify the Problem Type
The first step in solving a dynamic programming problem is to identify its type. Common types of DP problems include:
- 0/1 Knapsack
- Unbounded Knapsack
- Longest Common Subsequence (LCS)
- Longest Increasing Subsequence (LIS)
- Matrix Chain Multiplication
- Shortest Path Problems
- Edit Distance
Recognizing the problem type can help you apply known patterns and solutions, saving time during the interview.
2. Identify the Subproblems
Once you’ve identified the problem type, the next step is to break down the problem into smaller subproblems. Ask yourself:
- What are the smallest instances of this problem?
- How can I express the solution to a larger instance in terms of solutions to smaller instances?
For example, in the Fibonacci sequence problem, the subproblem is calculating the Fibonacci number for a smaller value of n.
3. Define the Recurrence Relation
The recurrence relation is the heart of any dynamic programming solution. It expresses how the solution to a larger problem can be computed from the solutions to smaller subproblems. For the Fibonacci sequence, the recurrence relation is:
F(n) = F(n-1) + F(n-2)
Where F(n) represents the nth Fibonacci number.
4. Identify the Base Cases
Base cases are the simplest instances of the problem that can be solved directly. They serve as the foundation for building up to the solution of the original problem. In the Fibonacci sequence example, the base cases are:
F(0) = 0
F(1) = 1
5. Choose the Right DP Approach
There are two main approaches to implementing dynamic programming solutions:
Top-Down Approach (Memoization)
In this approach, you start with the original problem and recursively break it down into subproblems. You store the results of subproblems in a data structure (usually an array or a hash table) to avoid redundant computations. This is also known as memoization.
Bottom-Up Approach (Tabulation)
In this approach, you start by solving the smallest subproblems and work your way up to the original problem. You typically use an array to store the results of subproblems.
The choice between these approaches depends on the problem and personal preference. Top-down can be more intuitive and easier to implement, while bottom-up is often more efficient.
6. Optimize Space Complexity
Many DP solutions can be optimized for space complexity. Common techniques include:
- Using a 1D array instead of a 2D array when possible
- Using only a few variables instead of an array
- Overwriting the DP table to reuse space
For example, in the Fibonacci sequence problem, instead of storing all Fibonacci numbers, you can use just two variables to keep track of the last two numbers.
7. Practice Common DP Patterns
Familiarize yourself with common DP patterns. Some of these include:
- Minimum/Maximum Path to Reach a Target
- Distinct Ways
- Merging Intervals
- DP on Strings
- Decision Making
Understanding these patterns can help you quickly recognize and solve similar problems in interviews.
Implementing Dynamic Programming Solutions
Let’s look at how to implement dynamic programming solutions using both top-down and bottom-up approaches. We’ll use the classic Fibonacci sequence problem as an example.
Top-Down Approach (Memoization)
Here’s a Python implementation of the Fibonacci sequence using the top-down approach:
def fibonacci_top_down(n, memo=None):
if memo is None:
memo = {}
if n in memo:
return memo[n]
if n <= 1:
return n
memo[n] = fibonacci_top_down(n-1, memo) + fibonacci_top_down(n-2, memo)
return memo[n]
# Example usage
print(fibonacci_top_down(10)) # Output: 55
In this implementation, we use a dictionary (memo) to store the results of subproblems. This prevents redundant calculations and significantly improves the time complexity from O(2^n) to O(n).
Bottom-Up Approach (Tabulation)
Now, let’s implement the same problem using the bottom-up approach:
def fibonacci_bottom_up(n):
if n <= 1:
return n
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
# Example usage
print(fibonacci_bottom_up(10)) # Output: 55
In this implementation, we build up the solution iteratively, starting from the base cases and working our way up to n. This approach has a time complexity of O(n) and a space complexity of O(n).
Space-Optimized Bottom-Up Approach
We can further optimize the space complexity of the bottom-up approach:
def fibonacci_optimized(n):
if n <= 1:
return n
a, b = 0, 1
for _ in range(2, n + 1):
a, b = b, a + b
return b
# Example usage
print(fibonacci_optimized(10)) # Output: 55
This optimized version reduces the space complexity to O(1) while maintaining the O(n) time complexity.
Common Dynamic Programming Problems in Interviews
To help you prepare for coding interviews, let’s look at some common dynamic programming problems and their solutions.
1. Longest Common Subsequence (LCS)
Problem: Given two strings, find the length of their longest common subsequence.
Solution:
def lcs(X, Y):
m, n = len(X), len(Y)
L = [[0] * (n + 1) for _ in range(m + 1)]
for i in range(1, m + 1):
for j in range(1, n + 1):
if X[i-1] == Y[j-1]:
L[i][j] = L[i-1][j-1] + 1
else:
L[i][j] = max(L[i-1][j], L[i][j-1])
return L[m][n]
# Example usage
X = "ABCDGH"
Y = "AEDFHR"
print(lcs(X, Y)) # Output: 3 (The LCS is "ADH")
2. 0/1 Knapsack Problem
Problem: Given weights and values of n items, put these items in a knapsack of capacity W to get the maximum total value in the knapsack.
Solution:
def knapsack(W, wt, val, n):
K = [[0 for x in range(W + 1)] for x in range(n + 1)]
for i in range(n + 1):
for w in range(W + 1):
if i == 0 or w == 0:
K[i][w] = 0
elif wt[i-1] <= w:
K[i][w] = max(val[i-1] + K[i-1][w-wt[i-1]], K[i-1][w])
else:
K[i][w] = K[i-1][w]
return K[n][W]
# Example usage
val = [60, 100, 120]
wt = [10, 20, 30]
W = 50
n = len(val)
print(knapsack(W, wt, val, n)) # Output: 220
3. Coin Change Problem
Problem: Given a set of coin denominations and a target amount, find the minimum number of coins needed to make up that amount.
Solution:
def coin_change(coins, amount):
dp = [float('inf')] * (amount + 1)
dp[0] = 0
for coin in coins:
for x in range(coin, amount + 1):
dp[x] = min(dp[x], dp[x - coin] + 1)
return dp[amount] if dp[amount] != float('inf') else -1
# Example usage
coins = [1, 2, 5]
amount = 11
print(coin_change(coins, amount)) # Output: 3 (5 + 5 + 1)
Tips for Solving DP Problems in Interviews
Here are some additional tips to help you tackle dynamic programming problems effectively in coding interviews:
1. Start with a Recursive Solution
If you’re struggling to come up with a DP solution immediately, start by writing a recursive solution. This can help you identify the subproblems and the recurrence relation. Once you have a working recursive solution, you can optimize it using memoization or convert it to a bottom-up approach.
2. Visualize the Problem
Many DP problems can be visualized using tables or matrices. Drawing these out can help you understand the problem better and identify patterns. For example, in the Longest Common Subsequence problem, visualizing the 2D DP table can make the solution much clearer.
3. Think About the State
In DP problems, the “state” represents the current situation in the problem. Identifying what information needs to be kept in the state is crucial. For example, in the Knapsack problem, the state includes the current item being considered and the current capacity of the knapsack.
4. Consider the Time and Space Complexity
Always analyze the time and space complexity of your solution. Interviewers often ask about this, and it’s a great way to show your understanding of the algorithm. Look for ways to optimize your solution, such as reducing a 2D DP table to a 1D array if possible.
5. Practice, Practice, Practice
The key to mastering dynamic programming is practice. Solve a variety of DP problems to familiarize yourself with different patterns and techniques. Websites like LeetCode, HackerRank, and AlgoCademy offer a wide range of DP problems to practice.
6. Explain Your Thought Process
During the interview, explain your thought process as you work through the problem. Start by identifying the problem type, then explain how you’re breaking it down into subproblems, defining the recurrence relation, and choosing between top-down and bottom-up approaches. This demonstrates your problem-solving skills and can earn you points even if you don’t arrive at the perfect solution.
Conclusion
Dynamic programming is a powerful technique that can solve a wide range of complex problems efficiently. By mastering the strategies outlined in this guide and practicing regularly, you can significantly improve your ability to tackle DP problems in coding interviews.
Remember, the key to success with dynamic programming is to break down the problem into smaller subproblems, identify the recurrence relation, and choose the appropriate implementation approach. With practice, you’ll become more comfortable recognizing DP patterns and applying them to new problems.
As you prepare for your coding interviews, don’t forget to leverage resources like AlgoCademy, which offers interactive coding tutorials and AI-powered assistance to help you master dynamic programming and other crucial algorithmic concepts. Good luck with your interview preparation!