Algorithms: The Building Blocks of Programming and Their Impact on Software Development
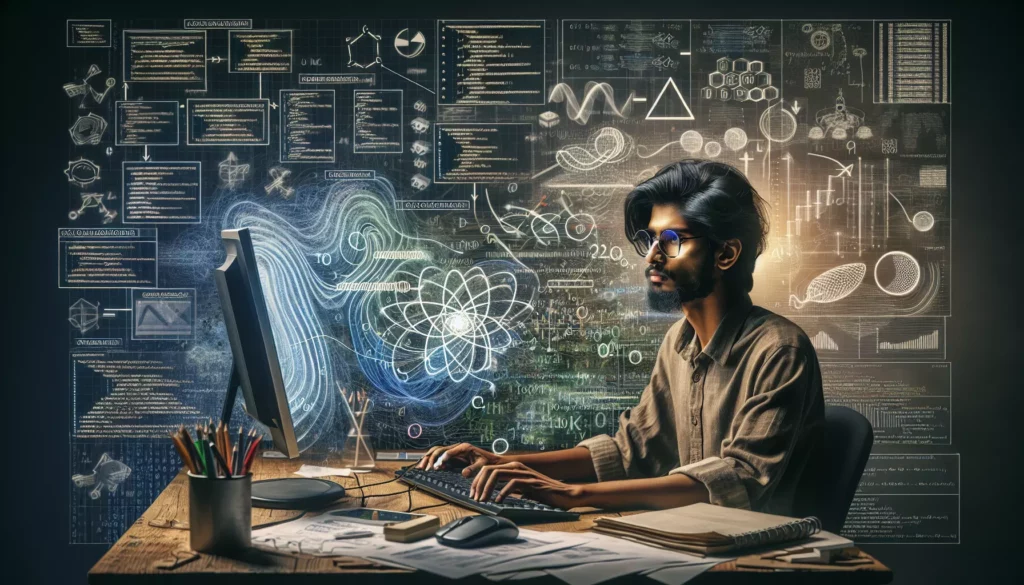
In the world of computer science and programming, algorithms play a crucial role in solving complex problems and creating efficient software solutions. Whether you’re a beginner just starting your coding journey or an experienced developer preparing for technical interviews at major tech companies, understanding algorithms is essential. In this comprehensive guide, we’ll explore what algorithms are, how they work, and their significant impact on programming and software development.
What Are Algorithms?
At its core, an algorithm is a step-by-step procedure or set of rules designed to solve a specific problem or perform a particular task. In the context of programming, algorithms serve as the foundation for writing efficient and effective code. They provide a systematic approach to problem-solving, allowing developers to break down complex tasks into manageable steps.
Think of an algorithm as a recipe. Just as a recipe provides instructions for preparing a dish, an algorithm outlines the steps needed to solve a problem or complete a task in programming. These steps are precise, unambiguous, and can be followed by both humans and computers.
Key Characteristics of Algorithms
- Input: Algorithms typically take one or more inputs, which are the data or parameters needed to perform the task.
- Output: After processing the input, algorithms produce one or more outputs, which are the results or solutions to the problem.
- Finiteness: An algorithm must terminate after a finite number of steps, ensuring it doesn’t run indefinitely.
- Definiteness: Each step in an algorithm must be precisely defined, leaving no room for ambiguity.
- Effectiveness: The algorithm should be practical and executable using available resources within a reasonable time frame.
Types of Algorithms
Algorithms come in various types, each designed to solve specific classes of problems. Understanding these different types can help developers choose the most appropriate approach for a given task. Here are some common categories of algorithms:
1. Sorting Algorithms
Sorting algorithms arrange data elements in a specific order, typically ascending or descending. Some popular sorting algorithms include:
- Bubble Sort
- Insertion Sort
- Selection Sort
- Merge Sort
- Quick Sort
For example, here’s a simple implementation of the Bubble Sort algorithm in Python:
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n - i - 1):
if arr[j] > arr[j + 1]:
arr[j], arr[j + 1] = arr[j + 1], arr[j]
return arr
# Example usage
unsorted_list = [64, 34, 25, 12, 22, 11, 90]
sorted_list = bubble_sort(unsorted_list)
print("Sorted list:", sorted_list)
2. Searching Algorithms
Searching algorithms are used to find specific elements within a data structure. Common searching algorithms include:
- Linear Search
- Binary Search
- Depth-First Search (DFS)
- Breadth-First Search (BFS)
Here’s an example of a binary search algorithm implemented in Java:
public class BinarySearch {
public static int binarySearch(int[] arr, int target) {
int left = 0;
int right = arr.length - 1;
while (left <= right) {
int mid = left + (right - left) / 2;
if (arr[mid] == target) {
return mid;
} else if (arr[mid] < target) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return -1; // Element not found
}
public static void main(String[] args) {
int[] sortedArray = {1, 3, 5, 7, 9, 11, 13, 15};
int target = 7;
int result = binarySearch(sortedArray, target);
if (result != -1) {
System.out.println("Element found at index: " + result);
} else {
System.out.println("Element not found in the array.");
}
}
}
3. Graph Algorithms
Graph algorithms are used to solve problems related to graph data structures. These include:
- Dijkstra’s Algorithm (shortest path)
- Kruskal’s Algorithm (minimum spanning tree)
- Floyd-Warshall Algorithm (all-pairs shortest path)
- Topological Sorting
4. Dynamic Programming Algorithms
Dynamic programming is a technique used to solve complex problems by breaking them down into simpler subproblems. It’s particularly useful for optimization problems. Examples include:
- Fibonacci Sequence
- Knapsack Problem
- Longest Common Subsequence
5. Greedy Algorithms
Greedy algorithms make locally optimal choices at each step with the hope of finding a global optimum. They are often used for optimization problems. Examples include:
- Huffman Coding
- Prim’s Algorithm (minimum spanning tree)
- Kruskal’s Algorithm (minimum spanning tree)
The Impact of Algorithms on Programming
Understanding and implementing algorithms effectively can have a profound impact on various aspects of programming and software development. Let’s explore some of the key areas where algorithms make a significant difference:
1. Performance Optimization
One of the primary impacts of algorithms on programming is in performance optimization. Choosing the right algorithm for a specific task can dramatically improve the efficiency and speed of a program. For example, consider the task of sorting a large dataset:
- Using a simple algorithm like Bubble Sort might take O(n^2) time complexity, which becomes impractical for large datasets.
- On the other hand, using a more efficient algorithm like Quick Sort or Merge Sort can reduce the time complexity to O(n log n), making it much faster for large datasets.
By selecting appropriate algorithms, developers can create software that runs faster, uses less memory, and can handle larger volumes of data more efficiently.
2. Problem-Solving Skills
Studying and implementing algorithms helps developers improve their problem-solving skills. Algorithms provide structured approaches to breaking down complex problems into smaller, manageable steps. This skill is invaluable in all aspects of programming, from designing system architectures to debugging code.
For instance, when faced with a new programming challenge, a developer familiar with various algorithmic paradigms can more easily identify patterns and apply known solutions or adapt existing algorithms to solve the problem at hand.
3. Code Quality and Maintainability
Well-implemented algorithms often lead to cleaner, more organized code. By using established algorithms, developers can create code that is:
- More readable and understandable
- Easier to maintain and update
- Less prone to bugs and errors
For example, implementing a well-known sorting algorithm like Merge Sort not only ensures efficient sorting but also makes the code more comprehensible to other developers who may need to work on it in the future.
4. Scalability
As software applications grow and handle increasing amounts of data, the importance of efficient algorithms becomes even more pronounced. Scalable algorithms ensure that applications can handle growth without significant performance degradation.
Consider a social media platform that needs to recommend friends to users. As the user base grows from thousands to millions, an inefficient recommendation algorithm could cause the system to slow down dramatically. By implementing more scalable algorithms, such as collaborative filtering or graph-based recommendations, the platform can continue to provide quick and accurate suggestions even as it expands.
5. Resource Utilization
Efficient algorithms not only improve speed but also optimize resource utilization. This is particularly important in environments where resources are limited, such as mobile devices or embedded systems. By using algorithms that minimize memory usage or reduce CPU cycles, developers can create applications that are more energy-efficient and can run smoothly on a wider range of devices.
6. Competitive Advantage
In the competitive world of software development, the ability to implement efficient algorithms can provide a significant edge. This is especially true for companies dealing with large-scale data processing or real-time systems. For example:
- A search engine that can return results faster due to optimized search algorithms will likely attract more users.
- A financial trading platform that can execute trades more quickly using efficient algorithms can provide better services to its clients.
7. Innovation and Research
The study and development of new algorithms drive innovation in computer science and related fields. Many breakthrough technologies and applications are the result of novel algorithmic approaches. For instance:
- The development of machine learning algorithms has led to advancements in artificial intelligence and data analysis.
- Cryptographic algorithms have enabled secure communication and e-commerce on the internet.
Algorithms in Practice: Real-World Applications
To better understand the impact of algorithms on programming and everyday life, let’s look at some real-world applications:
1. Navigation Systems
GPS navigation systems rely heavily on graph algorithms to find the shortest or fastest route between two points. Algorithms like Dijkstra’s or A* are commonly used to calculate optimal paths, taking into account factors like distance, traffic, and road conditions.
2. Recommendation Systems
E-commerce platforms, streaming services, and social media sites use sophisticated recommendation algorithms to suggest products, content, or connections to users. These algorithms often combine collaborative filtering, content-based filtering, and machine learning techniques to provide personalized recommendations.
3. Search Engines
Search engines like Google use complex algorithms to index, rank, and retrieve relevant web pages based on user queries. These algorithms consider numerous factors, including keyword relevance, page authority, and user behavior, to deliver the most pertinent results.
4. Image and Video Processing
Algorithms play a crucial role in various image and video processing tasks, such as:
- Facial recognition
- Object detection
- Image compression
- Video encoding and streaming
For example, the JPEG image compression algorithm uses discrete cosine transform (DCT) to reduce file sizes while maintaining acceptable image quality.
5. Financial Systems
The financial industry relies heavily on algorithms for various purposes:
- High-frequency trading algorithms that make split-second decisions to buy or sell stocks
- Risk assessment algorithms used by banks and insurance companies
- Fraud detection algorithms that identify suspicious transactions
6. Machine Learning and AI
Machine learning algorithms form the backbone of many AI applications. These algorithms enable computers to learn from data and improve their performance over time. Examples include:
- Neural networks for image and speech recognition
- Decision trees and random forests for predictive modeling
- Clustering algorithms for data segmentation
Challenges and Considerations in Algorithm Design
While algorithms offer numerous benefits, their design and implementation come with several challenges and considerations:
1. Time and Space Complexity
Balancing time complexity (how long an algorithm takes to run) and space complexity (how much memory it uses) is a constant challenge. Developers often need to make trade-offs between speed and memory usage based on the specific requirements of their application.
2. Scalability
Ensuring that algorithms can handle increasing amounts of data without significant performance degradation is crucial, especially for applications expected to grow over time.
3. Edge Cases and Error Handling
Algorithms must be designed to handle various edge cases and unexpected inputs gracefully. Proper error handling and input validation are essential to create robust and reliable software.
4. Maintainability and Readability
While optimizing for performance is important, algorithms should also be designed with maintainability in mind. Clear, well-documented code ensures that other developers can understand and modify the algorithm if needed.
5. Ethical Considerations
As algorithms increasingly influence various aspects of our lives, ethical considerations become crucial. Issues such as bias in AI algorithms, privacy concerns in data processing algorithms, and the societal impact of recommendation algorithms need to be carefully addressed.
Learning and Mastering Algorithms
For aspiring programmers and experienced developers alike, continual learning and practice are key to mastering algorithms. Here are some strategies to improve your algorithmic skills:
1. Study Data Structures
A solid understanding of data structures is fundamental to working with algorithms effectively. Familiarize yourself with arrays, linked lists, trees, graphs, hash tables, and other common data structures.
2. Practice Problem-Solving
Regularly solve coding challenges and algorithmic problems on platforms like LeetCode, HackerRank, or CodeSignal. These platforms offer a wide range of problems that can help you apply algorithmic concepts in practice.
3. Analyze Existing Algorithms
Study well-known algorithms and their implementations. Understand why they work and analyze their time and space complexities. This will help you recognize patterns and apply similar approaches to new problems.
4. Implement Algorithms from Scratch
Try implementing common algorithms yourself, without relying on built-in library functions. This hands-on approach will deepen your understanding of how algorithms work under the hood.
5. Participate in Coding Competitions
Join coding competitions or hackathons to challenge yourself and learn from others. These events often present complex problems that require efficient algorithmic solutions.
6. Read Books and Research Papers
Explore classic textbooks on algorithms and data structures, such as “Introduction to Algorithms” by Cormen et al. or “The Algorithm Design Manual” by Skiena. Additionally, reading research papers can expose you to cutting-edge algorithmic developments.
7. Collaborate and Share Knowledge
Engage with other developers through online forums, local meetups, or open-source projects. Discussing algorithmic approaches and reviewing others’ code can provide valuable insights and alternative perspectives.
Conclusion
Algorithms are the backbone of efficient and effective programming. They provide structured approaches to problem-solving, enable performance optimization, and drive innovation in software development. From simple sorting tasks to complex AI applications, algorithms impact every aspect of modern computing.
As a programmer, investing time in understanding and mastering algorithms will significantly enhance your problem-solving skills, improve the quality of your code, and prepare you for the challenges of building scalable and efficient software solutions. Whether you’re just starting your coding journey or preparing for technical interviews at major tech companies, a strong foundation in algorithms will serve you well throughout your career in software development.
Remember that becoming proficient in algorithms is an ongoing process. Continuous learning, practice, and application of algorithmic concepts to real-world problems will help you grow as a developer and tackle increasingly complex challenges in the ever-evolving field of computer science.