What is Continuous Integration/Continuous Deployment (CI/CD)?
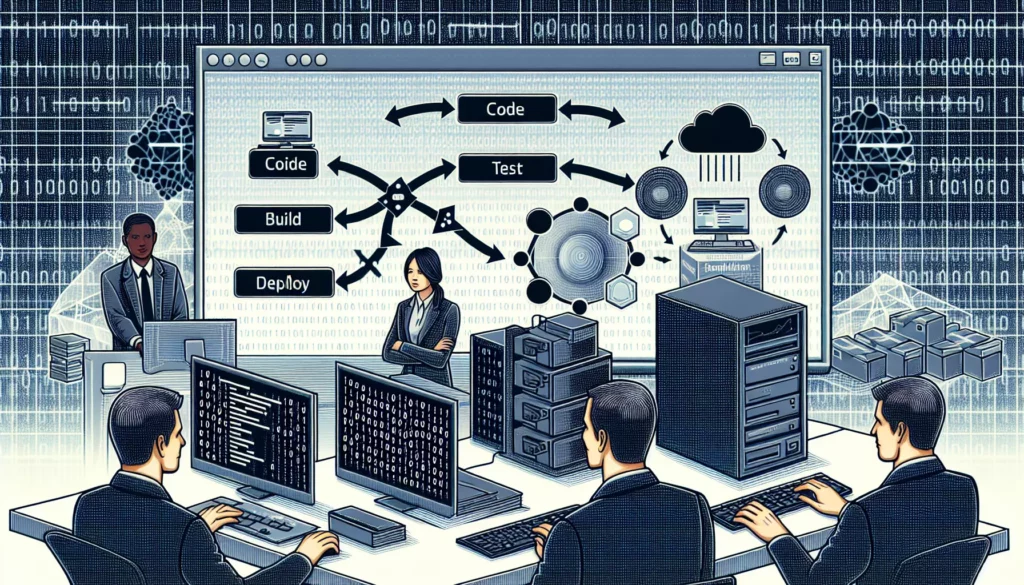
In the fast-paced world of software development, efficiency and reliability are paramount. As projects grow in complexity and teams expand, the need for streamlined processes becomes increasingly critical. This is where Continuous Integration/Continuous Deployment (CI/CD) comes into play. In this comprehensive guide, we’ll explore the ins and outs of CI/CD, its benefits, implementation strategies, and how it’s revolutionizing the way we build and deliver software.
Understanding Continuous Integration (CI)
Continuous Integration is a software development practice where developers regularly merge their code changes into a central repository, after which automated builds and tests are run. The key goals of CI are to find and address bugs quicker, improve software quality, and reduce the time it takes to validate and release new software updates.
Key Components of CI
- Version Control System: Such as Git, which allows multiple developers to work on the same project simultaneously.
- Automated Build Process: Scripts that compile code, run tests, and create deployable artifacts.
- Continuous Integration Server: Tools like Jenkins, Travis CI, or GitLab CI that automate the integration process.
- Testing Framework: Automated tests that run every time code is integrated.
Benefits of Continuous Integration
- Early Bug Detection: By integrating regularly, developers can discover and fix integration issues early.
- Reduced Integration Problems: Frequent integration minimizes the chances of major conflicts between different parts of the codebase.
- Improved Code Quality: Automated testing ensures that code meets predefined quality standards.
- Faster Development Cycles: CI enables teams to deliver updates more frequently and reliably.
Diving into Continuous Deployment (CD)
Continuous Deployment takes the concept of Continuous Integration a step further. In CD, every change that passes the automated tests is automatically deployed to production. This means that there’s no human intervention, and only a failed test will prevent a new change from going live.
Key Components of CD
- Deployment Pipeline: A series of stages that code changes must pass through before reaching production.
- Environment Management: Tools to manage and provision different environments (development, staging, production).
- Release Automation: Scripts and tools that automate the deployment process.
- Monitoring and Logging: Systems to track the performance and behavior of deployed applications.
Benefits of Continuous Deployment
- Faster Time-to-Market: Features and fixes reach users as soon as they’re ready.
- Reduced Risk: Smaller, more frequent deployments are less risky than large, infrequent ones.
- Continuous Feedback: Rapid deployment allows for quick user feedback and iterative improvements.
- Improved Developer Productivity: Automating the deployment process frees up developers to focus on writing code.
The CI/CD Pipeline
The CI/CD pipeline is the backbone of modern DevOps practices. It’s a series of steps that must be performed in order to deliver a new version of software. Let’s break down a typical CI/CD pipeline:
1. Code Commit
The pipeline begins when a developer commits code to the version control system. This triggers the CI process.
2. Build
The code is compiled, and any necessary dependencies are fetched. This step ensures that the code can be built successfully.
3. Unit Tests
Automated unit tests are run to check individual components of the code. These tests are typically written by developers alongside the code.
4. Integration Tests
These tests verify that different parts of the application work together as expected.
5. Code Analysis
Static code analysis tools check for code quality, style violations, and potential bugs.
6. Security Scans
Automated security checks look for known vulnerabilities in the code and dependencies.
7. Deployment to Staging
If all previous steps pass, the code is automatically deployed to a staging environment that mimics production.
8. Acceptance Tests
Automated acceptance tests are run in the staging environment to ensure the application behaves as expected from an end-user perspective.
9. Deployment to Production
If all tests pass, the code is automatically deployed to the production environment.
10. Post-Deployment Tests
Final checks are performed in the production environment to ensure everything is working correctly.
Implementing CI/CD: Best Practices
Implementing a CI/CD pipeline can be challenging, but following these best practices can help ensure success:
1. Automate Everything
The key to effective CI/CD is automation. Aim to automate as much of the build, test, and deployment process as possible.
2. Build Fast
Keep your build times short. Long build times can slow down development and reduce the effectiveness of CI.
3. Test in Production-Like Environments
Ensure your staging environments closely mirror production to catch environment-specific issues early.
4. Make Small, Frequent Changes
Smaller changes are easier to test and less likely to cause problems. Encourage developers to commit frequently.
5. Use Feature Flags
Feature flags allow you to deploy code to production but only activate it for certain users or under certain conditions. This can help manage risk in CD.
6. Monitor and Log Everything
Comprehensive monitoring and logging are crucial for quickly identifying and resolving issues in production.
7. Embrace Infrastructure as Code
Use tools like Terraform or Ansible to manage your infrastructure programmatically, ensuring consistency across environments.
CI/CD Tools and Technologies
There are numerous tools available to help implement CI/CD. Here are some popular options:
CI Servers
- Jenkins: Open-source automation server with a vast plugin ecosystem.
- Travis CI: Cloud-based CI service that integrates well with GitHub.
- GitLab CI: Integrated CI/CD solution within the GitLab platform.
- CircleCI: Cloud-based CI/CD platform with a focus on speed and efficiency.
Version Control Systems
- Git: Distributed version control system, widely used in the industry.
- Subversion (SVN): Centralized version control system, still used in some enterprises.
Containerization and Orchestration
- Docker: Platform for developing, shipping, and running applications in containers.
- Kubernetes: Open-source system for automating deployment, scaling, and management of containerized applications.
Configuration Management
- Ansible: Simple, agentless automation tool.
- Puppet: Configuration management tool for defining infrastructure as code.
- Chef: Automation platform that configures and manages infrastructure.
Monitoring and Logging
- Prometheus: Open-source monitoring and alerting toolkit.
- ELK Stack (Elasticsearch, Logstash, Kibana): Popular stack for log analysis and visualization.
- Grafana: Platform for monitoring and observability.
CI/CD in Practice: A Simple Example
Let’s look at a simple example of how a CI/CD pipeline might be set up using GitHub Actions for a Python web application:
<!-- .github/workflows/ci-cd.yml -->
name: CI/CD Pipeline
on:
push:
branches: [ main ]
pull_request:
branches: [ main ]
jobs:
build-and-test:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Set up Python
uses: actions/setup-python@v2
with:
python-version: '3.x'
- name: Install dependencies
run: |
python -m pip install --upgrade pip
pip install -r requirements.txt
- name: Run tests
run: python -m pytest tests/
deploy:
needs: build-and-test
runs-on: ubuntu-latest
if: github.ref == 'refs/heads/main'
steps:
- uses: actions/checkout@v2
- name: Deploy to Heroku
uses: akhileshns/heroku-deploy@v3.12.12
with:
heroku_api_key: ${{secrets.HEROKU_API_KEY}}
heroku_app_name: "your-app-name"
heroku_email: "your-email@example.com"
This workflow does the following:
- Triggers on pushes to the main branch or pull requests targeting main.
- Sets up a Python environment and installs dependencies.
- Runs tests using pytest.
- If tests pass and the change is on the main branch, it deploys to Heroku.
Challenges and Considerations
While CI/CD offers numerous benefits, it’s not without its challenges:
1. Initial Setup Complexity
Setting up a CI/CD pipeline can be complex and time-consuming, especially for large, existing projects.
2. Test Coverage
Effective CI/CD relies heavily on comprehensive test coverage. Writing and maintaining good tests can be challenging.
3. Cultural Shift
Moving to CI/CD often requires a significant cultural shift within an organization, which can be met with resistance.
4. Infrastructure Costs
Running a CI/CD pipeline, especially with extensive testing, can be resource-intensive and potentially costly.
5. Security Concerns
Automating deployment to production raises security concerns that need to be carefully addressed.
The Future of CI/CD
As software development continues to evolve, so too does CI/CD. Here are some trends shaping the future of CI/CD:
1. AI and Machine Learning Integration
AI could be used to optimize test selection, predict build failures, and even suggest code improvements.
2. Serverless CI/CD
Serverless architectures are being applied to CI/CD, potentially reducing costs and improving scalability.
3. GitOps
GitOps extends the idea of infrastructure as code, using Git as the single source of truth for declarative infrastructure and applications.
4. Increased Focus on Security
With the rise of DevSecOps, security is being integrated more deeply into the CI/CD pipeline.
5. Low-Code/No-Code CI/CD
Tools are emerging that allow for the creation of CI/CD pipelines with minimal coding, making the practice more accessible.
Conclusion
Continuous Integration/Continuous Deployment has revolutionized software development, enabling teams to deliver high-quality software faster and more reliably than ever before. By automating the build, test, and deployment processes, CI/CD minimizes human error, provides rapid feedback, and allows developers to focus on what they do best: writing code.
While implementing CI/CD can be challenging, the benefits in terms of productivity, code quality, and time-to-market make it a worthwhile investment for most software development teams. As the field continues to evolve, embracing CI/CD practices will likely become not just a competitive advantage, but a necessity for staying relevant in the fast-paced world of software development.
Whether you’re a seasoned developer or just starting your journey in software engineering, understanding and implementing CI/CD principles will undoubtedly make you a more effective and efficient developer. So dive in, start small, and gradually build up your CI/CD pipeline. Your future self (and your team) will thank you for it!