Procedural vs Object-Oriented Programming: Understanding the Key Differences
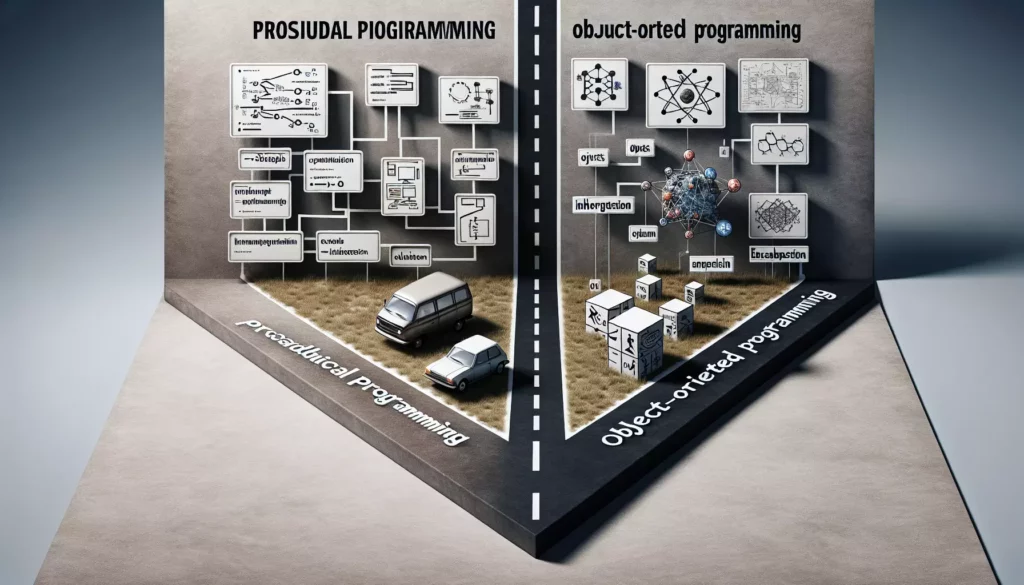
In the world of software development, two major programming paradigms have dominated the landscape for decades: Procedural Programming and Object-Oriented Programming (OOP). As aspiring developers or those looking to enhance their coding skills, it’s crucial to understand the differences between these two approaches. This comprehensive guide will delve into the intricacies of procedural and object-oriented programming, exploring their strengths, weaknesses, and use cases. By the end of this article, you’ll have a clear understanding of when to use each paradigm and how they can impact your coding projects.
1. Introduction to Programming Paradigms
Before we dive into the specifics of procedural and object-oriented programming, let’s first understand what a programming paradigm is and why it matters.
1.1 What is a Programming Paradigm?
A programming paradigm is a fundamental style of computer programming that provides a way of viewing and organizing the structure and elements of code. It represents a specific approach to solving problems and designing software. Different paradigms can lead to vastly different code organization, syntax, and overall program structure.
1.2 Why Are Programming Paradigms Important?
Understanding various programming paradigms is crucial for several reasons:
- Problem-solving approach: Different paradigms offer unique ways to tackle programming challenges.
- Code organization: Paradigms influence how you structure your code, affecting readability and maintainability.
- Efficiency: Certain paradigms may be more efficient for specific types of problems or applications.
- Versatility: Knowing multiple paradigms makes you a more versatile programmer, able to adapt to different project requirements.
- Career growth: Many programming languages support multiple paradigms, so understanding them can broaden your skillset and career opportunities.
2. Procedural Programming: An Overview
Procedural programming is one of the oldest and most straightforward programming paradigms. It’s based on the concept of procedure calls, where the program is divided into procedures (also known as routines or functions) that perform specific tasks.
2.1 Key Characteristics of Procedural Programming
- Sequential execution: Code is executed in a top-down, step-by-step manner.
- Modularity: Programs are divided into functions or procedures.
- Data and procedures are separate: Data structures are distinct from the procedures that manipulate them.
- Emphasis on algorithms: Focus is on creating step-by-step instructions to solve problems.
- Global data: Often relies on global variables accessible throughout the program.
2.2 Advantages of Procedural Programming
- Simplicity: Easy to learn and understand, especially for beginners.
- Efficiency: Can be very efficient for straightforward, linear tasks.
- Low overhead: Typically requires less memory and processing power compared to OOP.
- Reusability: Functions can be reused in different parts of the program or in other programs.
- Structured approach: Encourages a clear, logical flow of code execution.
2.3 Disadvantages of Procedural Programming
- Limited code reusability: While functions are reusable, it’s not as extensive as in OOP.
- Difficulty in managing large programs: As programs grow, they can become complex and hard to maintain.
- Data security: Global variables can be accessed and modified from anywhere, potentially leading to unexpected behavior.
- Less suitable for complex relationships: Struggles to represent complex relationships between data and functionality.
- Lack of data hiding: Difficult to hide implementation details from the rest of the program.
2.4 Example of Procedural Programming
Let’s look at a simple example of procedural programming in Python:
def calculate_area(length, width):
return length * width
def calculate_perimeter(length, width):
return 2 * (length + width)
def main():
length = 5
width = 3
area = calculate_area(length, width)
perimeter = calculate_perimeter(length, width)
print(f"Area: {area}")
print(f"Perimeter: {perimeter}")
if __name__ == "__main__":
main()
In this example, we have separate functions for calculating area and perimeter, and a main function that uses these calculations. This demonstrates the procedural approach of breaking down the program into distinct procedures.
3. Object-Oriented Programming: An Overview
Object-Oriented Programming (OOP) is a programming paradigm based on the concept of “objects,” which can contain data and code. The data is in the form of fields (often known as attributes or properties), and the code is in the form of procedures (often known as methods).
3.1 Key Characteristics of Object-Oriented Programming
- Objects: Programs are organized around objects that combine data and functionality.
- Classes: Serve as blueprints for creating objects.
- Encapsulation: Data and methods are bundled together within objects.
- Inheritance: Allows creation of new classes based on existing classes.
- Polymorphism: Enables objects of different classes to be treated as objects of a common base class.
- Abstraction: Hides complex implementation details and shows only the necessary features of an object.
3.2 Advantages of Object-Oriented Programming
- Modularity: Encapsulation allows objects to be self-contained, improving maintainability.
- Reusability: Classes can be reused in multiple projects, promoting code reuse.
- Flexibility: Polymorphism and inheritance make it easier to extend and modify existing code.
- Real-world modeling: OOP concepts align well with real-world objects and relationships.
- Better organization: Grouping related data and behavior into objects improves code structure.
- Data protection: Encapsulation provides better control over data access and modification.
3.3 Disadvantages of Object-Oriented Programming
- Complexity: Can be more complex to learn and implement, especially for beginners.
- Larger program size: OOP programs can be larger and require more lines of code than procedural equivalents.
- Slower execution: In some cases, OOP can be slower due to the additional layers of abstraction.
- Steep learning curve: Concepts like inheritance and polymorphism can be challenging for newcomers.
- Over-engineering: It’s easy to create overly complex class hierarchies that are difficult to maintain.
3.4 Example of Object-Oriented Programming
Here’s an example of the same rectangle calculations using OOP in Python:
class Rectangle:
def __init__(self, length, width):
self.length = length
self.width = width
def calculate_area(self):
return self.length * self.width
def calculate_perimeter(self):
return 2 * (self.length + self.width)
def main():
rectangle = Rectangle(5, 3)
area = rectangle.calculate_area()
perimeter = rectangle.calculate_perimeter()
print(f"Area: {area}")
print(f"Perimeter: {perimeter}")
if __name__ == "__main__":
main()
In this OOP example, we define a Rectangle class that encapsulates the length and width attributes along with methods to calculate area and perimeter. This demonstrates how OOP combines data and functionality into a single entity.
4. Key Differences Between Procedural and Object-Oriented Programming
Now that we’ve explored both paradigms, let’s highlight the key differences between procedural and object-oriented programming:
4.1 Program Structure
- Procedural: Organized around procedures or functions that operate on data.
- OOP: Organized around objects that combine data and methods.
4.2 Data Handling
- Procedural: Data and functions are separate entities.
- OOP: Data and methods are encapsulated within objects.
4.3 Code Reusability
- Procedural: Functions can be reused, but data structures are not as easily reusable.
- OOP: Classes and objects promote high reusability through inheritance and polymorphism.
4.4 Data Security
- Procedural: Data is often more exposed, with heavy use of global variables.
- OOP: Encapsulation provides better data protection and access control.
4.5 Scalability
- Procedural: Can become difficult to manage and maintain as the program grows larger.
- OOP: Generally scales better for large and complex programs due to its modular nature.
4.6 Relationship Modeling
- Procedural: Struggles to represent complex relationships between entities.
- OOP: Excels at modeling real-world relationships and interactions between objects.
4.7 Learning Curve
- Procedural: Generally easier to learn and understand, especially for beginners.
- OOP: Has a steeper learning curve due to more complex concepts like inheritance and polymorphism.
4.8 Program Flow
- Procedural: Follows a top-down, step-by-step execution flow.
- OOP: Flow is determined by object interactions and method calls, which can be more flexible but also more complex.
5. When to Use Procedural Programming
Procedural programming can be an excellent choice in certain scenarios:
- Small-scale programs: For simple, straightforward applications with limited complexity.
- Linear processes: When the program follows a clear, step-by-step sequence of operations.
- Performance-critical applications: In situations where every bit of performance matters, as procedural code can be more efficient.
- Limited resources: On systems with limited memory or processing power, procedural programming’s lower overhead can be advantageous.
- Quick prototyping: For rapidly creating simple prototypes or scripts.
- Beginners: As an introduction to programming concepts before moving on to more complex paradigms.
6. When to Use Object-Oriented Programming
Object-Oriented Programming shines in many modern development scenarios:
- Large-scale applications: For complex systems where modularity and organization are crucial.
- GUI programming: OOP concepts align well with graphical user interface components.
- Simulation and modeling: When representing real-world objects and their interactions.
- Game development: OOP’s ability to model objects and their behaviors is particularly useful in game design.
- Enterprise software: For building scalable, maintainable business applications.
- Collaborative development: OOP’s modularity facilitates team-based development on large projects.
- Frameworks and libraries: Many modern frameworks and libraries are built using OOP principles.
7. Hybrid Approaches: Combining Paradigms
It’s important to note that many modern programming languages support multiple paradigms, allowing developers to use a hybrid approach that combines elements of both procedural and object-oriented programming. This flexibility enables programmers to choose the best approach for each specific part of their application.
7.1 Benefits of a Hybrid Approach
- Flexibility: Use the most appropriate paradigm for different parts of your program.
- Performance optimization: Utilize procedural techniques for performance-critical sections while maintaining an overall OOP structure.
- Gradual transition: Allows for incremental adoption of OOP in existing procedural codebases.
- Best of both worlds: Combine the simplicity of procedural programming with the organization and reusability of OOP.
7.2 Example of a Hybrid Approach
Here’s an example that combines both procedural and OOP approaches:
def calculate_tax(amount, rate):
return amount * rate
class Product:
def __init__(self, name, price):
self.name = name
self.price = price
def get_price_with_tax(self, tax_rate):
return self.price + calculate_tax(self.price, tax_rate)
def main():
book = Product("Python Programming", 29.99)
tax_rate = 0.08
final_price = book.get_price_with_tax(tax_rate)
print(f"Price of {book.name} with tax: ${final_price:.2f}")
if __name__ == "__main__":
main()
In this example, we use a procedural function calculate_tax()
alongside an object-oriented Product
class. This demonstrates how both paradigms can be used together effectively.
8. Learning and Mastering Both Paradigms
As an aspiring developer or someone looking to enhance their coding skills, it’s valuable to learn and master both procedural and object-oriented programming. Here are some tips to help you on your learning journey:
8.1 Start with Procedural Programming
- Begin with the basics of procedural programming to understand fundamental programming concepts.
- Practice writing simple programs that use functions and basic data structures.
- Focus on problem-solving and algorithm development.
8.2 Transition to Object-Oriented Programming
- Once comfortable with procedural concepts, start learning OOP principles.
- Begin with simple classes and objects before moving on to more advanced concepts like inheritance and polymorphism.
- Refactor procedural code into OOP to understand the differences and benefits.
8.3 Practice with Real-World Projects
- Work on projects that can benefit from both paradigms.
- Experiment with different design patterns and architectures.
- Contribute to open-source projects to see how different paradigms are used in practice.
8.4 Utilize Learning Resources
- Take advantage of online courses, tutorials, and coding challenges.
- Read books on software design and architecture to deepen your understanding.
- Join coding communities and forums to discuss and learn from others.
9. Conclusion
Understanding the differences between procedural and object-oriented programming is crucial for any developer looking to enhance their coding skills. Both paradigms have their strengths and weaknesses, and knowing when to use each one can significantly impact the efficiency, maintainability, and scalability of your code.
Procedural programming offers simplicity and efficiency, making it ideal for small-scale projects and performance-critical applications. On the other hand, object-oriented programming provides powerful tools for organizing complex systems, promoting code reuse, and modeling real-world relationships.
As you continue your journey in software development, remember that the choice between procedural and object-oriented programming isn’t always an either-or decision. Many modern programming languages and projects benefit from a hybrid approach, combining the strengths of both paradigms to create efficient, maintainable, and scalable software solutions.
By mastering both procedural and object-oriented programming, you’ll equip yourself with a versatile skill set that can adapt to various programming challenges. Whether you’re working on a simple script or a complex enterprise application, understanding these paradigms will help you choose the right approach for each situation, ultimately making you a more effective and valuable programmer in today’s diverse software development landscape.