Common Mistakes to Avoid When Learning to Code: A Comprehensive Guide
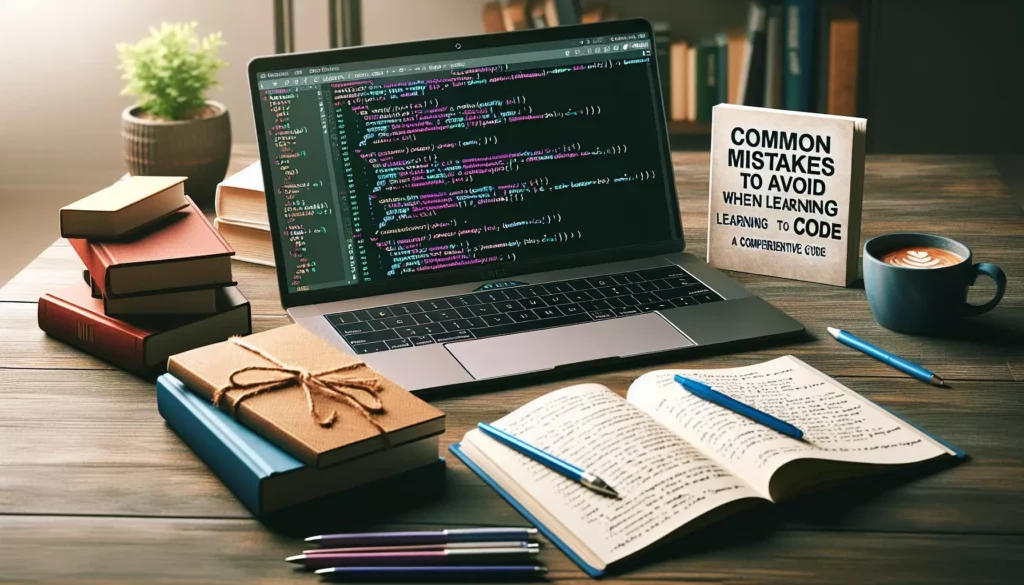
Learning to code is an exciting journey that opens up a world of possibilities in the tech industry. Whether you’re aspiring to become a software engineer, web developer, or data scientist, mastering programming skills is essential. However, the path to becoming a proficient coder is not without its challenges. Many beginners make common mistakes that can hinder their progress and lead to frustration. In this comprehensive guide, we’ll explore the most frequent pitfalls encountered by coding novices and provide actionable advice on how to avoid them.
1. Trying to Learn Everything at Once
One of the biggest mistakes newcomers make is attempting to learn multiple programming languages or concepts simultaneously. The world of coding is vast, with numerous languages, frameworks, and tools available. It’s easy to feel overwhelmed and try to tackle everything at once.
Why it’s a problem: Spreading yourself too thin can lead to confusion and a lack of depth in your understanding. It’s challenging to master any single language or concept when you’re juggling multiple ones at the same time.
How to avoid it:
- Start with one programming language and focus on mastering its fundamentals.
- Choose a language that aligns with your goals (e.g., Python for data science, JavaScript for web development).
- Create a structured learning plan that progresses from basic to advanced concepts within that language.
- Only move on to another language or framework once you have a solid foundation in your primary language.
2. Neglecting the Fundamentals
In their eagerness to build complex projects or dive into advanced topics, many beginners skip over the crucial fundamentals of programming.
Why it’s a problem: Without a strong grasp of the basics, you’ll struggle to understand more complex concepts and write efficient, clean code. This can lead to frustration and difficulty in solving problems as you progress.
How to avoid it:
- Dedicate ample time to learning core programming concepts such as variables, data types, control structures, and functions.
- Practice writing simple programs that reinforce these fundamental concepts.
- Use resources like AlgoCademy that provide structured learning paths focusing on building a strong foundation.
- Don’t rush to advanced topics until you’re comfortable with the basics.
3. Not Practicing Enough
Reading tutorials and watching coding videos are essential parts of learning, but many beginners make the mistake of not putting their knowledge into practice.
Why it’s a problem: Programming is a skill that requires hands-on experience. Without regular practice, you won’t develop the problem-solving skills and muscle memory needed to write code efficiently.
How to avoid it:
- Set aside dedicated time for coding practice every day, even if it’s just for 30 minutes.
- Work on small projects or coding challenges to apply what you’ve learned.
- Use platforms like AlgoCademy that offer interactive coding exercises and projects.
- Participate in coding competitions or hackathons to challenge yourself and gain real-world experience.
4. Copy-Pasting Code Without Understanding
It’s tempting to find solutions online and copy-paste them into your projects, especially when you’re stuck. However, this is a common mistake that can hinder your learning progress.
Why it’s a problem: While copy-pasting may solve your immediate problem, it doesn’t help you understand the underlying concepts or improve your problem-solving skills. You may find yourself unable to modify or debug the code later.
How to avoid it:
- When you find a solution online, take the time to understand how it works before using it.
- Try to recreate the solution from scratch, referring to the original only when necessary.
- If you must use someone else’s code, add comments explaining each part to ensure you understand it.
- Use AI-powered coding assistants, like those provided by AlgoCademy, to get explanations and guidance rather than complete solutions.
5. Not Using Version Control
Many beginners overlook the importance of version control systems like Git, considering them unnecessary for small projects or solo work.
Why it’s a problem: Version control is crucial for tracking changes, collaborating with others, and maintaining a history of your project. Not using it can lead to lost work, difficulty in reverting changes, and challenges when working on team projects.
How to avoid it:
- Start using Git from the beginning, even for small personal projects.
- Learn the basic Git commands and workflow (commit, push, pull, branch, merge).
- Use platforms like GitHub or GitLab to store your repositories and collaborate with others.
- Make frequent, small commits with descriptive messages to track your progress.
6. Ignoring Code Style and Best Practices
When starting out, many programmers focus solely on making their code work, neglecting proper formatting, naming conventions, and other best practices.
Why it’s a problem: Poor code style makes your programs harder to read, maintain, and debug. It can also make it difficult for others to understand and collaborate on your code.
How to avoid it:
- Familiarize yourself with the style guide for your chosen programming language (e.g., PEP 8 for Python).
- Use consistent indentation, naming conventions, and commenting practices.
- Learn and apply principles of clean code, such as DRY (Don’t Repeat Yourself) and SOLID.
- Use linting tools and code formatters to help maintain consistent style.
7. Not Debugging Effectively
Ineffective debugging is a common issue among beginners who often resort to guessing or making random changes to fix errors.
Why it’s a problem: Poor debugging skills can lead to wasted time, frustration, and the introduction of new bugs while trying to fix existing ones.
How to avoid it:
- Learn to use debugging tools provided by your IDE or development environment.
- Practice reading and understanding error messages instead of immediately searching for solutions online.
- Use print statements or logging to track the flow of your program and identify where issues occur.
- Break down complex problems into smaller, testable parts to isolate issues.
- Learn and apply systematic debugging techniques, such as the scientific method of hypothesis and testing.
8. Overlooking the Importance of Algorithms and Data Structures
Many beginners focus solely on syntax and language features, neglecting the crucial study of algorithms and data structures.
Why it’s a problem: Understanding algorithms and data structures is fundamental to writing efficient code and solving complex problems. It’s also a key component of technical interviews at major tech companies.
How to avoid it:
- Dedicate time to studying common algorithms (sorting, searching, graph traversal) and data structures (arrays, linked lists, trees, hash tables).
- Practice implementing these concepts from scratch in your chosen programming language.
- Solve algorithmic problems on platforms like AlgoCademy, which offer a wide range of challenges tailored to different skill levels.
- Analyze the time and space complexity of your solutions to understand efficiency trade-offs.
9. Not Reading Documentation
Relying solely on tutorials or Stack Overflow answers without consulting official documentation is a common mistake among coding beginners.
Why it’s a problem: Documentation provides the most accurate and up-to-date information about languages, libraries, and frameworks. Not referring to it can lead to outdated practices or misunderstandings about how things work.
How to avoid it:
- Make a habit of reading official documentation for the languages and tools you’re using.
- Start with the “Getting Started” or beginner sections of documentation to build familiarity.
- Use documentation as your first resource when encountering new concepts or functions.
- Practice navigating and searching documentation efficiently to find the information you need.
10. Failing to Plan Before Coding
Jumping straight into coding without proper planning is a common pitfall for enthusiastic beginners.
Why it’s a problem: Lack of planning can lead to poorly structured code, frequent rewrites, and difficulty in scaling or maintaining your projects.
How to avoid it:
- Before writing any code, spend time understanding the problem and outlining your approach.
- Break down large projects into smaller, manageable tasks or user stories.
- Create flowcharts or pseudocode to map out the logic of your program.
- Consider potential edge cases and how your solution will handle them.
- Use design patterns and architectural principles to guide your overall project structure.
11. Not Seeking Help or Feedback
Many beginners hesitate to ask for help or share their code for feedback, often due to fear of judgment or a desire to figure everything out on their own.
Why it’s a problem: Coding in isolation can slow down your learning process and lead to the reinforcement of bad habits or misunderstandings.
How to avoid it:
- Join coding communities, forums, or local meetups to connect with other learners and experienced developers.
- Don’t be afraid to ask questions when you’re stuck, but make sure to do your research first and provide context about what you’ve already tried.
- Share your code for review and be open to constructive criticism.
- Participate in pair programming sessions or coding workshops to learn from others.
- Use platforms like AlgoCademy that offer mentorship or AI-powered assistance to get personalized feedback on your code.
12. Neglecting to Learn About Time and Space Complexity
Understanding the efficiency of algorithms in terms of time and space usage is often overlooked by coding beginners.
Why it’s a problem: Without knowledge of time and space complexity, you may write inefficient code that performs poorly on large datasets or consumes excessive memory.
How to avoid it:
- Study Big O notation and how it’s used to describe algorithm efficiency.
- Analyze the time and space complexity of common algorithms and data structures.
- Practice optimizing your code for better performance.
- Use tools and profilers to measure the actual performance of your programs.
- Solve coding challenges that require efficient solutions, such as those found on competitive programming platforms or in technical interview preparation resources like AlgoCademy.
13. Not Writing Tests
Many beginners view writing tests as an optional or advanced practice, often skipping it entirely.
Why it’s a problem: Lack of testing can lead to undetected bugs, difficulty in refactoring code, and decreased confidence in your program’s correctness.
How to avoid it:
- Learn about different types of tests (unit tests, integration tests, end-to-end tests) and when to use each.
- Start with simple unit tests for individual functions or methods.
- Practice Test-Driven Development (TDD) by writing tests before implementing features.
- Use testing frameworks appropriate for your programming language (e.g., pytest for Python, Jest for JavaScript).
- Aim for good test coverage, especially for critical parts of your codebase.
14. Focusing Too Much on Tools and IDEs
Some beginners become overly reliant on specific development environments or tools, neglecting to understand the underlying principles.
Why it’s a problem: Over-reliance on tools can limit your flexibility and understanding of how things work under the hood. It may also make it difficult to adapt to different development environments.
How to avoid it:
- Learn to use a simple text editor and command-line interface for basic coding tasks.
- Understand how to compile and run your code without relying on an IDE’s “Run” button.
- Experiment with different development environments to understand their pros and cons.
- Focus on learning the language and concepts rather than memorizing IDE shortcuts.
- Gradually introduce more advanced tools and features as you become comfortable with the basics.
15. Not Contributing to Open Source
Many beginners overlook the valuable learning opportunity that comes from contributing to open-source projects.
Why it’s a problem: Not participating in open source can limit your exposure to real-world codebases, collaboration practices, and the opportunity to build a public portfolio of your work.
How to avoid it:
- Start by contributing to documentation or fixing small bugs in open-source projects.
- Use platforms like GitHub to find beginner-friendly issues labeled as “good first issue” or “help wanted”.
- Learn how to fork repositories, create pull requests, and participate in code reviews.
- Join open-source communities and attend contribution events or sprints.
- Use your contributions to build a portfolio and demonstrate your skills to potential employers.
Conclusion
Learning to code is a challenging but rewarding journey. By being aware of these common mistakes and actively working to avoid them, you can accelerate your learning process and become a more effective programmer. Remember that everyone makes mistakes when learning to code – the key is to learn from them and continuously improve.
Platforms like AlgoCademy can be invaluable in this journey, offering structured learning paths, interactive coding exercises, and AI-powered assistance to help you navigate the complexities of programming. By focusing on building a strong foundation, practicing regularly, and engaging with the coding community, you’ll be well on your way to becoming a skilled and confident programmer.
Keep in mind that learning to code is a lifelong process. Even experienced developers continue to learn and adapt to new technologies and best practices. Embrace the challenges, stay curious, and don’t be afraid to make mistakes – they’re all part of the learning process. Happy coding!