How to Protect Your Code from Security Vulnerabilities: A Comprehensive Guide
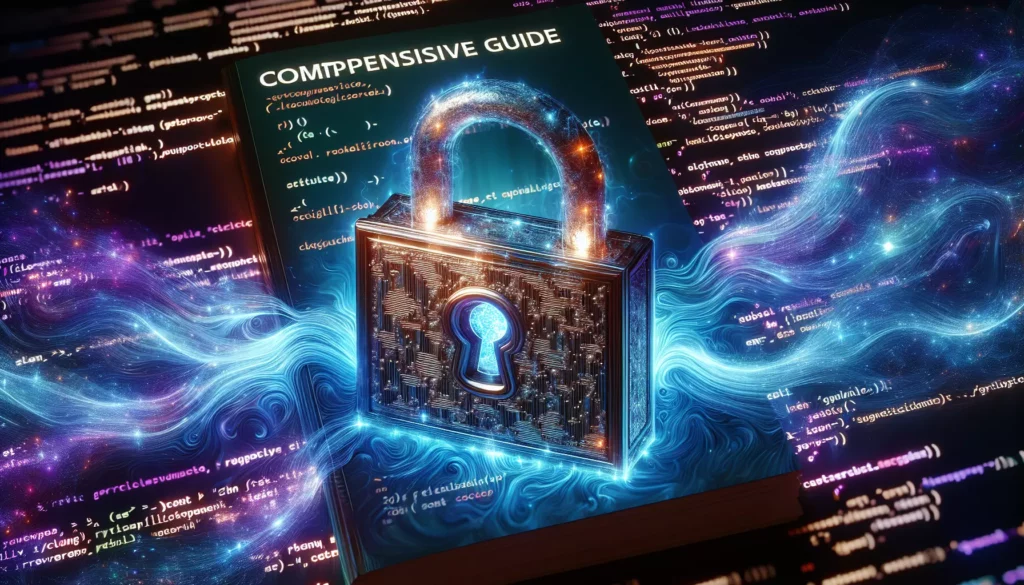
In today’s digital landscape, where cyber threats are becoming increasingly sophisticated, protecting your code from security vulnerabilities is more crucial than ever. As developers, we have a responsibility to ensure that the software we create is not only functional but also secure. This comprehensive guide will walk you through various strategies and best practices to safeguard your code against potential security breaches.
Table of Contents
- Understanding Security Vulnerabilities
- Secure Coding Practices
- Input Validation and Sanitization
- Authentication and Authorization
- Encryption and Data Protection
- Dependency Management
- Security Testing and Code Review
- Logging and Monitoring
- Regular Updates and Patch Management
- Security Training and Awareness
- Conclusion
1. Understanding Security Vulnerabilities
Before we dive into protection strategies, it’s essential to understand what security vulnerabilities are and how they can affect your code. Security vulnerabilities are weaknesses or flaws in your software that can be exploited by attackers to compromise the system’s confidentiality, integrity, or availability.
Common types of security vulnerabilities include:
- SQL Injection
- Cross-Site Scripting (XSS)
- Buffer Overflows
- Broken Authentication
- Sensitive Data Exposure
- XML External Entities (XXE)
- Insecure Deserialization
Understanding these vulnerabilities is the first step in protecting your code. Let’s explore various strategies to mitigate these risks.
2. Secure Coding Practices
Adopting secure coding practices is fundamental to creating robust and secure software. Here are some key practices to follow:
2.1 Principle of Least Privilege
Always grant the minimum level of access or permissions necessary for a component or user to perform its function. This limits the potential damage if a breach occurs.
2.2 Defense in Depth
Implement multiple layers of security controls throughout your application. This approach ensures that if one layer is compromised, other layers can still protect the system.
2.3 Fail Securely
Design your application to fail in a secure manner. When an error occurs, ensure that it doesn’t reveal sensitive information or leave the system in a vulnerable state.
2.4 Separation of Concerns
Divide your application into distinct features with as little overlap in functionality as possible. This makes it easier to maintain and secure each component independently.
2.5 Use Safe APIs
Whenever possible, use built-in or well-established APIs and libraries that have been thoroughly tested for security. Avoid reinventing the wheel, especially for critical security functions.
3. Input Validation and Sanitization
One of the most critical aspects of securing your code is properly handling user input. Malicious users often exploit vulnerabilities by injecting harmful data into your application.
3.1 Validate All Input
Always validate user input on both the client and server sides. This includes checking for expected data types, lengths, formats, and ranges.
3.2 Sanitize Input
After validation, sanitize the input by removing or encoding potentially dangerous characters. This helps prevent attacks like XSS and SQL injection.
3.3 Use Parameterized Queries
When interacting with databases, use parameterized queries or prepared statements instead of concatenating user input directly into SQL statements. This effectively prevents SQL injection attacks.
Here’s an example of a parameterized query in Python using SQLite:
import sqlite3
def get_user(username):
conn = sqlite3.connect('users.db')
cursor = conn.cursor()
# Use parameterized query
cursor.execute("SELECT * FROM users WHERE username = ?", (username,))
user = cursor.fetchone()
conn.close()
return user
# Usage
user = get_user("john_doe")
4. Authentication and Authorization
Proper authentication and authorization mechanisms are crucial for protecting your application and its users.
4.1 Implement Strong Authentication
Use strong, industry-standard authentication methods. This may include:
- Multi-factor authentication (MFA)
- Password complexity requirements
- Account lockout policies
- CAPTCHA for login attempts
4.2 Secure Password Storage
Never store passwords in plain text. Use strong, slow hashing algorithms like bcrypt or Argon2 to hash and salt passwords before storing them.
4.3 Implement Proper Session Management
Use secure session management techniques, including:
- Generating strong, random session IDs
- Setting appropriate session timeouts
- Invalidating sessions on logout
- Using secure, HTTP-only cookies for session tokens
4.4 Fine-grained Authorization
Implement role-based access control (RBAC) or attribute-based access control (ABAC) to ensure users can only access resources they’re authorized to use.
5. Encryption and Data Protection
Protecting sensitive data through encryption is essential for maintaining confidentiality and integrity.
5.1 Use HTTPS
Always use HTTPS to encrypt data in transit. This protects against man-in-the-middle attacks and eavesdropping.
5.2 Encrypt Sensitive Data at Rest
Use strong encryption algorithms to protect sensitive data stored in databases or on file systems. Ensure proper key management practices are in place.
5.3 Implement Key Rotation
Regularly rotate encryption keys to limit the potential impact of a compromised key.
5.4 Use Secure Random Number Generation
When generating cryptographic keys or tokens, use cryptographically secure random number generators.
Here’s an example of generating a secure random token in Python:
import secrets
def generate_secure_token(length=32):
return secrets.token_hex(length)
# Usage
secure_token = generate_secure_token()
print(secure_token)
6. Dependency Management
Managing third-party dependencies is crucial for maintaining the security of your application.
6.1 Keep Dependencies Updated
Regularly update your dependencies to ensure you have the latest security patches. Use tools like npm audit (for Node.js) or safety (for Python) to check for known vulnerabilities in your dependencies.
6.2 Use a Package Manager
Utilize package managers (like npm, pip, or Maven) to manage your dependencies. These tools often provide features for checking and updating vulnerable packages.
6.3 Implement a Software Bill of Materials (SBOM)
Maintain a comprehensive list of all components and dependencies used in your application. This helps in quickly identifying and addressing vulnerabilities when they’re discovered.
7. Security Testing and Code Review
Regular security testing and code reviews are essential for identifying and addressing vulnerabilities in your code.
7.1 Static Application Security Testing (SAST)
Use SAST tools to analyze your source code for potential security vulnerabilities. These tools can identify issues like buffer overflows, SQL injection vulnerabilities, and more.
7.2 Dynamic Application Security Testing (DAST)
Employ DAST tools to test your running application for vulnerabilities. These tools simulate attacks on your application to uncover runtime issues.
7.3 Penetration Testing
Conduct regular penetration testing, either internally or through third-party security experts, to identify vulnerabilities that automated tools might miss.
7.4 Code Reviews
Implement a rigorous code review process that includes security-focused reviews. Use checklists and automated tools to ensure consistent and thorough reviews.
8. Logging and Monitoring
Proper logging and monitoring are crucial for detecting and responding to security incidents.
8.1 Implement Comprehensive Logging
Log all security-relevant events, including authentication attempts, access control decisions, and system changes. Ensure logs contain sufficient context without including sensitive data.
8.2 Use Centralized Log Management
Implement a centralized log management system to aggregate logs from all components of your application. This makes it easier to correlate events and detect anomalies.
8.3 Set Up Alerts
Configure alerts for suspicious activities or potential security incidents. This could include multiple failed login attempts, unexpected system changes, or unusual data access patterns.
8.4 Regular Log Review
Establish a process for regularly reviewing logs and investigating any suspicious activities.
9. Regular Updates and Patch Management
Keeping your systems and software up-to-date is crucial for maintaining security.
9.1 Implement a Patch Management Process
Establish a process for regularly applying security patches to all components of your application, including the operating system, web server, database, and application frameworks.
9.2 Stay Informed About Security Vulnerabilities
Subscribe to security mailing lists and vulnerability databases relevant to the technologies you use. This helps you stay informed about new vulnerabilities and patches.
9.3 Test Updates Before Deployment
Always test security updates in a staging environment before applying them to production systems to ensure they don’t introduce new issues.
10. Security Training and Awareness
Developing a security-conscious culture within your development team is crucial for maintaining code security.
10.1 Provide Regular Security Training
Conduct regular security training sessions for all developers. Cover topics like secure coding practices, common vulnerabilities, and the latest security threats.
10.2 Encourage a Security-First Mindset
Foster a culture where security is considered at every stage of the development process, not just as an afterthought.
10.3 Stay Updated with Security Trends
Encourage developers to stay updated with the latest security trends and best practices through conferences, webinars, and security-focused publications.
11. Conclusion
Protecting your code from security vulnerabilities is an ongoing process that requires vigilance, knowledge, and commitment. By implementing the strategies outlined in this guide, you can significantly reduce the risk of security breaches and create more robust, secure applications.
Remember, security is not a one-time task but a continuous process. Stay informed about new threats and vulnerabilities, regularly review and update your security practices, and always prioritize security in your development process.
By following these best practices and cultivating a security-first mindset, you’ll be well-equipped to protect your code and create safer, more secure software for your users.