How to Learn Programming Logic and Fundamentals: A Comprehensive Guide
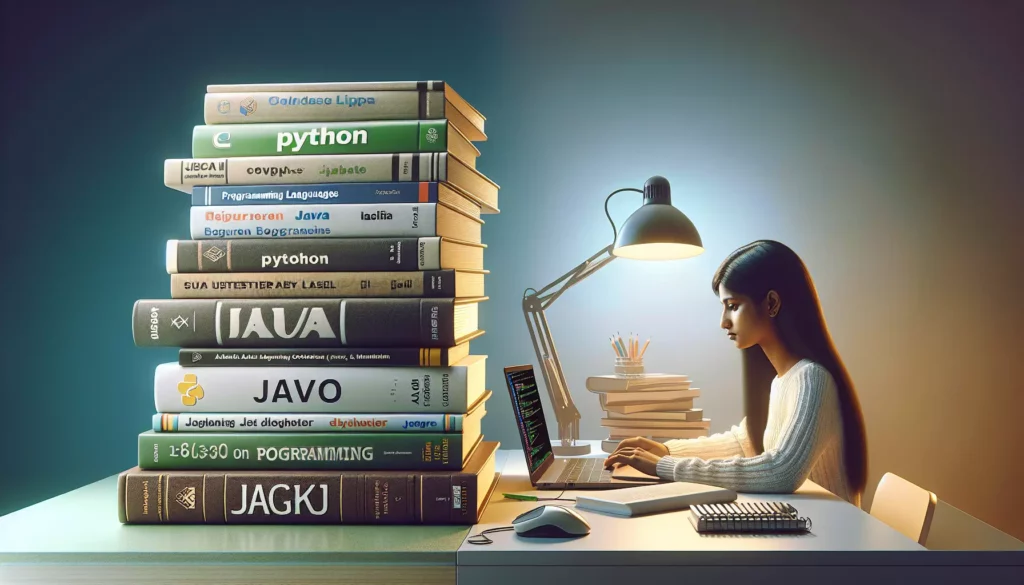
Programming is an essential skill in today’s digital world, and mastering programming logic and fundamentals is crucial for anyone looking to excel in the field of software development. Whether you’re a complete beginner or someone looking to solidify your foundational knowledge, this comprehensive guide will walk you through the process of learning programming logic and fundamentals effectively.
Table of Contents
- Understanding Programming Logic
- Key Programming Fundamentals
- Choosing a Programming Language
- Resources for Learning
- Practice and Projects
- Problem-Solving Techniques
- Developing Algorithmic Thinking
- Debugging and Troubleshooting
- Staying Motivated and Overcoming Challenges
- Advancing Your Skills
1. Understanding Programming Logic
Programming logic forms the foundation of all software development. It’s the way programmers think about solving problems and creating algorithms. Here are some key aspects of programming logic:
1.1 Sequential Execution
In programming, instructions are executed in a specific order, one after another. Understanding this sequential flow is crucial for writing effective code.
1.2 Conditional Statements
Conditional statements allow programs to make decisions based on certain conditions. The most common forms are if-else statements and switch-case structures.
1.3 Loops
Loops enable repetition of code blocks, which is essential for performing tasks multiple times or iterating through data structures.
1.4 Functions and Modularity
Functions help in organizing code into reusable blocks, promoting modularity and making programs easier to understand and maintain.
1.5 Data Structures
Understanding various data structures like arrays, lists, stacks, and queues is crucial for efficient data management in programs.
2. Key Programming Fundamentals
To build a strong foundation in programming, you need to grasp these fundamental concepts:
2.1 Variables and Data Types
Learn how to declare variables and understand different data types such as integers, floating-point numbers, strings, and booleans.
2.2 Operators
Familiarize yourself with arithmetic, comparison, and logical operators used in programming languages.
2.3 Control Structures
Master the use of if-else statements, switch cases, for loops, while loops, and do-while loops.
2.4 Functions and Methods
Learn how to define and use functions, understand parameters and return values, and grasp the concept of scope.
2.5 Object-Oriented Programming (OOP)
Understand the basics of OOP, including classes, objects, inheritance, and encapsulation.
3. Choosing a Programming Language
Selecting the right programming language to start with can significantly impact your learning journey. Here are some popular options for beginners:
3.1 Python
Known for its simplicity and readability, Python is an excellent choice for beginners. It has a wide range of applications, from web development to data science.
3.2 JavaScript
If you’re interested in web development, JavaScript is a must-learn language. It’s versatile and can be used for both front-end and back-end development.
3.3 Java
Java is widely used in enterprise environments and Android app development. It’s a good choice if you’re aiming for a career in these areas.
3.4 C++
While more complex, C++ is excellent for understanding low-level programming concepts and is widely used in game development and system programming.
3.5 Ruby
Known for its simplicity and productivity, Ruby is popular in web development, particularly with the Ruby on Rails framework.
4. Resources for Learning
There are numerous resources available to help you learn programming logic and fundamentals:
4.1 Online Platforms
- Codecademy: Offers interactive coding lessons in various programming languages.
- freeCodeCamp: Provides free coding courses and certifications.
- Coursera: Offers university-level courses in computer science and programming.
- edX: Provides courses from top universities worldwide.
- AlgoCademy: Focuses on algorithmic thinking and problem-solving skills.
4.2 Books
- “Introduction to Algorithms” by Thomas H. Cormen
- “Clean Code: A Handbook of Agile Software Craftsmanship” by Robert C. Martin
- “Code Complete” by Steve McConnell
- “The Pragmatic Programmer” by Andrew Hunt and David Thomas
4.3 YouTube Channels
- CS50: Harvard’s Introduction to Computer Science course
- Traversy Media: Web development tutorials
- The Coding Train: Creative coding tutorials
- Derek Banas: Quick programming tutorials in various languages
4.4 Coding Bootcamps
Consider joining a coding bootcamp for intensive, hands-on learning experience. Many bootcamps offer both online and in-person options.
5. Practice and Projects
Theory alone is not enough to master programming. Regular practice and working on projects are crucial for reinforcing your learning:
5.1 Coding Exercises
Platforms like LeetCode, HackerRank, and Project Euler offer coding challenges to improve your problem-solving skills.
5.2 Personal Projects
Start with small projects and gradually increase complexity. Some ideas include:
- A simple calculator
- A to-do list application
- A basic web scraper
- A simple game (like Tic-Tac-Toe or Snake)
5.3 Open Source Contributions
Contributing to open-source projects can provide real-world experience and help you learn from experienced developers.
5.4 Coding Competitions
Participate in coding competitions to challenge yourself and learn from others.
6. Problem-Solving Techniques
Developing strong problem-solving skills is essential for becoming a proficient programmer. Here are some techniques to improve your problem-solving abilities:
6.1 Break Down the Problem
Divide complex problems into smaller, manageable sub-problems. This approach makes it easier to tackle challenging tasks.
6.2 Pseudocode
Before writing actual code, outline your solution in plain language. This helps in organizing your thoughts and planning the implementation.
6.3 Flowcharts
Use flowcharts to visualize the logic and flow of your program. This is particularly helpful for understanding complex algorithms.
6.4 Test-Driven Development (TDD)
Write tests before implementing the actual code. This approach helps in clearly defining the problem and ensuring your solution meets the requirements.
6.5 Rubber Duck Debugging
Explain your code or problem to an inanimate object (like a rubber duck). This process often helps in identifying issues or coming up with solutions.
7. Developing Algorithmic Thinking
Algorithmic thinking is the ability to define clear steps to solve a problem or complete a task. Here’s how you can develop this crucial skill:
7.1 Study Classic Algorithms
Familiarize yourself with fundamental algorithms like sorting (bubble sort, quicksort), searching (binary search), and graph algorithms (breadth-first search, depth-first search).
7.2 Analyze Time and Space Complexity
Learn to evaluate the efficiency of your algorithms in terms of time (how long it takes to run) and space (how much memory it uses).
7.3 Practice Algorithm Design
Regularly challenge yourself with algorithm design problems. Platforms like AlgoCademy offer guided practice in this area.
7.4 Learn Data Structures
Understanding data structures like arrays, linked lists, trees, and graphs is crucial for efficient algorithm design.
7.5 Implement Algorithms from Scratch
Try implementing common algorithms yourself instead of relying on built-in functions. This deepens your understanding of how they work.
8. Debugging and Troubleshooting
Debugging is an essential skill for any programmer. Here are some strategies to improve your debugging skills:
8.1 Use a Debugger
Learn to use the debugging tools provided by your IDE or code editor. These allow you to step through your code line by line and inspect variables.
8.2 Print Statements
While not the most efficient method, using print statements to output variable values can be a quick way to identify issues.
8.3 Error Messages
Learn to read and understand error messages. They often provide valuable information about what went wrong and where.
8.4 Logging
Implement logging in your programs to track the flow of execution and variable states.
8.5 Code Reviews
Having others review your code can help identify bugs and improve your coding practices.
9. Staying Motivated and Overcoming Challenges
Learning programming can be challenging, but staying motivated is key to success:
9.1 Set Realistic Goals
Break your learning journey into small, achievable goals. Celebrate each milestone you reach.
9.2 Join a Community
Engage with other learners through online forums, local meetups, or coding groups. Platforms like Stack Overflow and GitHub can be great for this.
9.3 Find a Mentor
Having a mentor can provide guidance, motivation, and insights into the industry.
9.4 Take Breaks
Regular breaks can help prevent burnout and often lead to problem-solving breakthroughs.
9.5 Apply Your Learning
Try to apply what you’re learning to real-world problems or projects you’re passionate about.
10. Advancing Your Skills
Once you’ve grasped the fundamentals, consider these steps to further advance your programming skills:
10.1 Learn Multiple Languages
Each programming language has its strengths and can teach you different ways of thinking about problems.
10.2 Explore Frameworks and Libraries
Familiarize yourself with popular frameworks and libraries in your chosen language(s).
10.3 Study Software Architecture
Learn about different architectural patterns and when to apply them.
10.4 Practice Code Optimization
Learn techniques to make your code more efficient and performant.
10.5 Contribute to Open Source
Contributing to open-source projects can expose you to large codebases and collaborative development practices.
10.6 Prepare for Technical Interviews
If you’re aiming for a career in tech, start preparing for technical interviews. Platforms like AlgoCademy offer resources specifically tailored for this purpose, focusing on the types of questions often asked by major tech companies.
Conclusion
Learning programming logic and fundamentals is a journey that requires dedication, practice, and persistence. By following this comprehensive guide and utilizing resources like AlgoCademy, you can build a strong foundation in programming and develop the skills necessary to tackle complex problems and advance in your coding career.
Remember, the key to success in programming is continuous learning and practice. As you progress, you’ll find that the logical thinking and problem-solving skills you develop will be valuable not just in coding, but in many aspects of life and work. Happy coding!