Synchronous vs Asynchronous Programming: Understanding the Key Differences
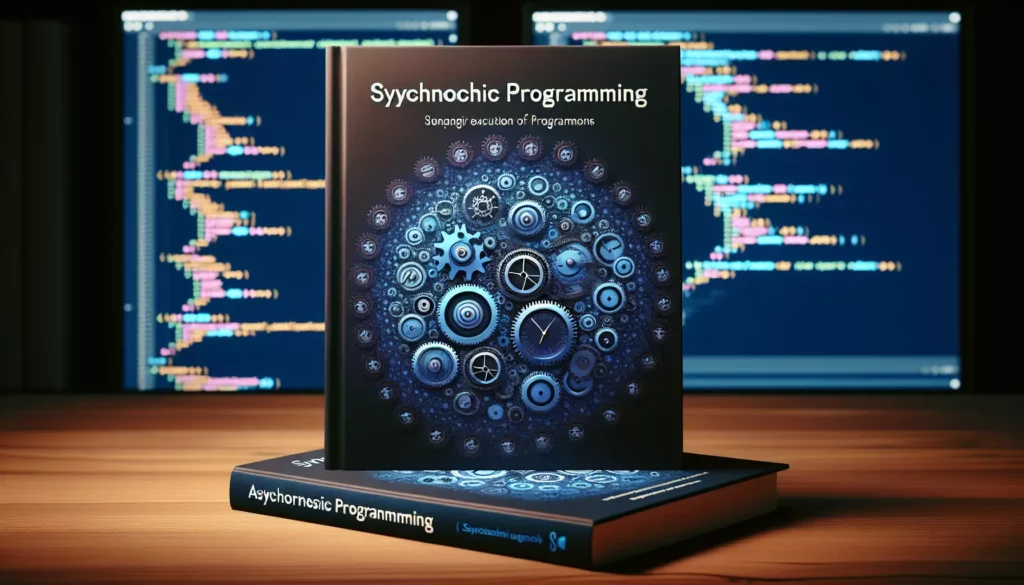
In the world of software development, understanding the difference between synchronous and asynchronous programming is crucial. These two paradigms represent fundamentally different approaches to executing code and managing tasks. Whether you’re a beginner coder or preparing for technical interviews at major tech companies, grasping these concepts is essential for writing efficient and responsive applications. In this comprehensive guide, we’ll dive deep into the world of synchronous and asynchronous programming, exploring their differences, use cases, and impact on application performance.
What is Synchronous Programming?
Synchronous programming is the traditional and more straightforward approach to executing code. In this model, tasks are performed sequentially, one after another. Each operation must complete before the next one begins, creating a predictable and easy-to-follow flow of execution.
Key Characteristics of Synchronous Programming:
- Sequential Execution: Tasks are executed in a specific order, one at a time.
- Blocking Nature: Each operation blocks the execution of subsequent tasks until it completes.
- Predictable Flow: The program’s execution path is straightforward and easy to follow.
- Simplicity: Synchronous code is often easier to write and understand, especially for beginners.
Example of Synchronous Code:
function syncOperation() {
console.log("Step 1");
console.log("Step 2");
console.log("Step 3");
}
syncOperation();
// Output:
// Step 1
// Step 2
// Step 3
In this example, each step is executed in order, and the function doesn’t move to the next step until the previous one is complete.
What is Asynchronous Programming?
Asynchronous programming is a more complex but powerful paradigm that allows multiple operations to occur concurrently. In this model, tasks can be initiated without waiting for previous operations to complete, enabling non-blocking execution and improved performance in certain scenarios.
Key Characteristics of Asynchronous Programming:
- Non-blocking Execution: Tasks can be initiated without waiting for others to complete.
- Concurrent Operations: Multiple operations can be in progress simultaneously.
- Callback Mechanisms: Asynchronous operations often use callbacks, promises, or async/await to handle results.
- Improved Responsiveness: Ideal for I/O-bound operations and maintaining responsive user interfaces.
- Complex Flow: The execution path can be more challenging to follow and debug.
Example of Asynchronous Code:
function asyncOperation() {
console.log("Step 1");
setTimeout(() => {
console.log("Step 2 (after 1 second)");
}, 1000);
console.log("Step 3");
}
asyncOperation();
// Output:
// Step 1
// Step 3
// Step 2 (after 1 second)
In this asynchronous example, Step 3 is executed immediately after Step 1, without waiting for the setTimeout callback to complete. The output order differs from the code sequence, demonstrating the non-blocking nature of asynchronous programming.
Key Differences Between Synchronous and Asynchronous Programming
Now that we’ve introduced both paradigms, let’s explore the key differences between synchronous and asynchronous programming:
1. Execution Order
Synchronous: Tasks are executed in a strict, sequential order. Each operation must complete before the next one begins.
Asynchronous: Tasks can be initiated and executed independently, often resulting in a non-sequential execution order.
2. Blocking vs. Non-blocking
Synchronous: Operations are blocking, meaning the program waits for each task to complete before moving on to the next one.
Asynchronous: Operations are non-blocking, allowing the program to continue executing other tasks while waiting for long-running operations to complete.
3. Concurrency
Synchronous: Limited concurrency, as tasks are executed one at a time.
Asynchronous: Enables concurrency, allowing multiple operations to be in progress simultaneously.
4. Complexity
Synchronous: Generally simpler to write, understand, and debug due to its straightforward execution flow.
Asynchronous: Often more complex, requiring additional constructs like callbacks, promises, or async/await to manage the flow of execution.
5. Performance in I/O-bound Operations
Synchronous: Can lead to inefficient use of resources, especially when dealing with I/O-bound operations that involve waiting for external resources.
Asynchronous: Excels in handling I/O-bound operations, allowing the program to perform other tasks while waiting for external resources.
6. Error Handling
Synchronous: Traditional try-catch blocks are typically sufficient for error handling.
Asynchronous: Error handling can be more challenging, often requiring specific patterns or constructs to catch and handle errors in asynchronous operations.
When to Use Synchronous Programming
While asynchronous programming offers many advantages, synchronous programming still has its place in software development. Here are some scenarios where synchronous programming might be preferred:
- Simple, Sequential Tasks: When operations need to be performed in a specific order, and each step depends on the result of the previous one.
- Small Data Sets: For processing small amounts of data where the performance impact of blocking operations is negligible.
- Initialization Routines: When setting up application state or configurations that must be completed before proceeding.
- Scripting and Automation: For simple scripts or automation tasks where a straightforward, sequential execution is desired.
- Learning and Teaching: Synchronous code is often easier for beginners to understand and is useful for teaching basic programming concepts.
When to Use Asynchronous Programming
Asynchronous programming shines in many modern development scenarios, particularly in web and network applications. Here are some situations where asynchronous programming is particularly beneficial:
- I/O-bound Operations: When dealing with operations that involve waiting for external resources, such as file systems, databases, or network requests.
- User Interface Responsiveness: In applications with graphical user interfaces, asynchronous programming helps maintain responsiveness by preventing long-running tasks from blocking the UI thread.
- Parallel Processing: When multiple independent tasks can be executed concurrently to improve overall performance.
- Real-time Applications: For systems that need to handle multiple concurrent connections or events, such as chat applications or real-time data processing.
- API Design: When creating APIs that need to handle multiple requests efficiently without blocking.
Implementing Asynchronous Programming
Asynchronous programming can be implemented using various techniques and patterns. Here are some common approaches:
1. Callbacks
Callbacks are functions passed as arguments to other functions, which are then invoked when the asynchronous operation completes.
function fetchData(callback) {
setTimeout(() => {
const data = { id: 1, name: "John Doe" };
callback(data);
}, 1000);
}
fetchData((result) => {
console.log(result);
});
// Output (after 1 second):
// { id: 1, name: "John Doe" }
2. Promises
Promises provide a more structured way to handle asynchronous operations, allowing for better error handling and chaining of asynchronous tasks.
function fetchData() {
return new Promise((resolve, reject) => {
setTimeout(() => {
const data = { id: 1, name: "John Doe" };
resolve(data);
}, 1000);
});
}
fetchData()
.then((result) => {
console.log(result);
})
.catch((error) => {
console.error(error);
});
// Output (after 1 second):
// { id: 1, name: "John Doe" }
3. Async/Await
Async/await is a syntactic sugar built on top of promises, providing a more synchronous-looking way to write asynchronous code.
async function fetchData() {
return new Promise((resolve) => {
setTimeout(() => {
const data = { id: 1, name: "John Doe" };
resolve(data);
}, 1000);
});
}
async function processData() {
try {
const result = await fetchData();
console.log(result);
} catch (error) {
console.error(error);
}
}
processData();
// Output (after 1 second):
// { id: 1, name: "John Doe" }
Performance Considerations
When deciding between synchronous and asynchronous programming, performance is often a key consideration. Here are some points to keep in mind:
- I/O-bound Operations: Asynchronous programming significantly outperforms synchronous programming for I/O-bound tasks, as it allows the program to continue executing while waiting for external resources.
- CPU-bound Operations: For CPU-intensive tasks, the performance difference between synchronous and asynchronous code may be less pronounced, and synchronous code might sometimes be more efficient due to its simplicity.
- Overhead: Asynchronous programming introduces some overhead due to the management of callbacks, promises, or async/await constructs. For very small or quick operations, this overhead might outweigh the benefits.
- Scalability: Asynchronous programming generally offers better scalability, especially in scenarios with many concurrent operations or connections.
Challenges in Asynchronous Programming
While asynchronous programming offers many benefits, it also comes with its own set of challenges:
- Callback Hell: Deeply nested callbacks can lead to code that’s difficult to read and maintain, often referred to as “callback hell” or the “pyramid of doom.”
- Error Handling: Proper error handling in asynchronous code can be more complex, requiring careful consideration of how errors are propagated and caught.
- Race Conditions: Asynchronous operations can lead to race conditions if not properly managed, where the outcome depends on the timing of events.
- Debugging: Asynchronous code can be more challenging to debug due to its non-linear execution flow.
- Mental Model: Developers need to shift their thinking from a sequential to a concurrent model, which can be challenging, especially for those new to asynchronous programming.
Best Practices for Asynchronous Programming
To make the most of asynchronous programming and mitigate its challenges, consider these best practices:
- Use Modern Async Patterns: Prefer promises and async/await over nested callbacks to improve code readability and maintainability.
- Error Handling: Always include proper error handling in asynchronous operations, using try-catch blocks with async/await or .catch() with promises.
- Avoid Blocking the Event Loop: In environments like Node.js, be cautious not to block the event loop with long-running synchronous operations.
- Parallel Execution: Use methods like Promise.all() to run multiple asynchronous operations in parallel when appropriate.
- Timeouts: Implement timeouts for asynchronous operations to prevent indefinite waiting.
- Testing: Write comprehensive tests for asynchronous code, including edge cases and error scenarios.
Conclusion
Understanding the difference between synchronous and asynchronous programming is crucial for developing efficient, responsive, and scalable applications. While synchronous programming offers simplicity and predictability, asynchronous programming provides powerful tools for handling concurrent operations and improving performance in I/O-bound scenarios.
As you progress in your coding journey, whether you’re working on personal projects or preparing for technical interviews at major tech companies, mastering both paradigms will give you the flexibility to choose the right approach for each situation. Remember that the choice between synchronous and asynchronous programming often depends on the specific requirements of your application, the nature of the operations you’re performing, and the overall architecture of your system.
By leveraging the strengths of both synchronous and asynchronous programming, you can create robust, efficient, and maintainable code that meets the demands of modern software development. Continue to practice and explore these concepts, and you’ll be well-equipped to tackle a wide range of programming challenges in your career.