Essential Math Skills Needed for Programming: A Comprehensive Guide
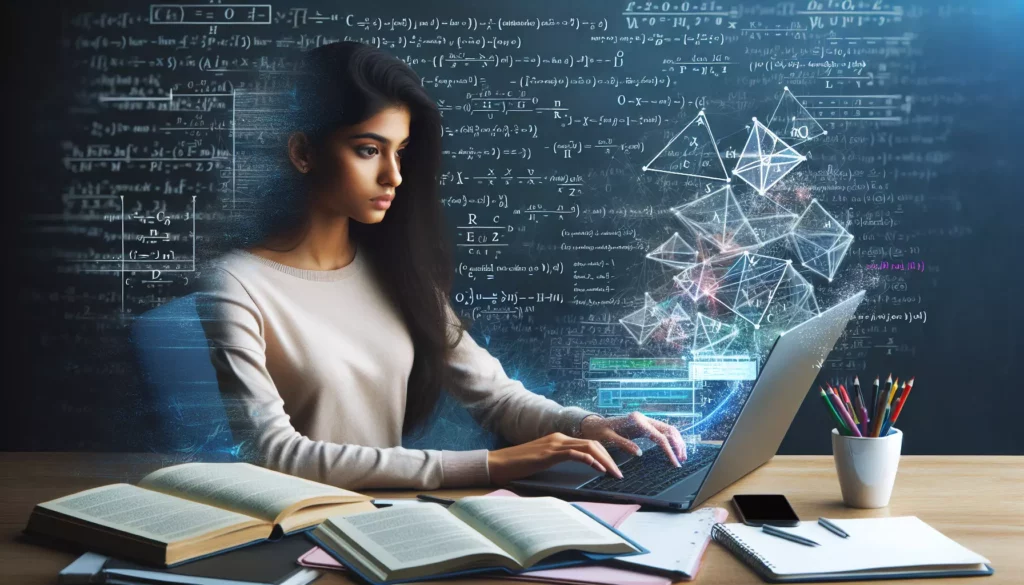
In the ever-evolving world of technology, programming has become an indispensable skill. Whether you’re a seasoned developer or just starting your coding journey, having a solid foundation in mathematics can significantly enhance your programming abilities. This comprehensive guide will explore the essential math skills needed for programming, helping you understand their importance and how they apply to various aspects of software development.
Table of Contents
- Introduction: The Intersection of Math and Programming
- Algebra: The Foundation of Programming Logic
- Arithmetic: Basic Operations and Their Importance
- Geometry: Spatial Reasoning in Programming
- Trigonometry: Angular Calculations in Graphics and Games
- Calculus: Optimization and Rate of Change
- Statistics and Probability: Data Analysis and Machine Learning
- Discrete Mathematics: The Core of Computer Science
- Linear Algebra: Matrices and Vectors in Programming
- Boolean Algebra: The Logic Behind Programming
- Number Systems and Binary: The Language of Computers
- Algorithmic Thinking: Problem-Solving in Programming
- Practical Applications of Math in Programming
- Resources for Improving Your Math Skills
- Conclusion: Embracing Math in Your Coding Journey
1. Introduction: The Intersection of Math and Programming
Mathematics and programming are intrinsically linked. While it’s possible to write code without an extensive mathematical background, understanding key mathematical concepts can significantly enhance your ability to solve complex problems, optimize algorithms, and create more efficient and elegant solutions.
As you progress in your programming career, you’ll encounter various scenarios where mathematical knowledge becomes crucial. From basic arithmetic operations to complex statistical analysis, math plays a vital role in numerous programming tasks. Let’s dive into the essential math skills that every programmer should strive to master.
2. Algebra: The Foundation of Programming Logic
Algebra is perhaps the most fundamental mathematical skill for programmers. It forms the basis of logical thinking and problem-solving in programming. Here’s why algebra is crucial:
- Variables and expressions: Just as in algebra, programming uses variables to represent unknown values. Understanding how to manipulate algebraic expressions translates directly to working with variables in code.
- Functions: Algebraic functions are similar to programming functions. Both take inputs, perform operations, and return outputs.
- Equations: Solving equations in algebra is analogous to debugging in programming. Both require logical thinking to isolate and solve for unknown values.
- Inequalities: These are useful in programming for creating conditional statements and defining ranges.
Example of algebraic thinking in programming:
// Algebraic expression: y = 2x + 3
function calculateY(x) {
return 2 * x + 3;
}
let result = calculateY(5);
console.log(result); // Output: 13
3. Arithmetic: Basic Operations and Their Importance
While it may seem obvious, a solid grasp of basic arithmetic is crucial for programming. The four fundamental operations (addition, subtraction, multiplication, and division) are used constantly in coding. Additionally, understanding concepts like order of operations (PEMDAS) is essential for writing and debugging mathematical expressions in code.
Key arithmetic concepts for programmers:
- Integer division and modulo operations
- Rounding and truncation
- Working with fractions and decimals
- Percentage calculations
- Exponents and roots
Example of arithmetic in programming:
let a = 10;
let b = 3;
console.log(a + b); // Addition: 13
console.log(a - b); // Subtraction: 7
console.log(a * b); // Multiplication: 30
console.log(a / b); // Division: 3.3333...
console.log(a % b); // Modulo (remainder): 1
console.log(Math.pow(a, b)); // Exponentiation: 1000
4. Geometry: Spatial Reasoning in Programming
Geometry is particularly important for programmers working on graphics, game development, or any application involving spatial relationships. Understanding geometric concepts helps in creating visual elements, handling collisions in games, and working with coordinate systems.
Key geometric concepts for programmers:
- Coordinate systems (Cartesian, polar)
- Shapes and their properties (area, perimeter, volume)
- Transformations (translation, rotation, scaling)
- Vector operations
- Collision detection algorithms
Example of geometry in programming (calculating distance between two points):
function calculateDistance(x1, y1, x2, y2) {
let dx = x2 - x1;
let dy = y2 - y1;
return Math.sqrt(dx * dx + dy * dy);
}
let distance = calculateDistance(0, 0, 3, 4);
console.log(distance); // Output: 5
5. Trigonometry: Angular Calculations in Graphics and Games
Trigonometry is essential for programmers working with graphics, animations, or game development. It’s used for calculating angles, rotations, and projections in 2D and 3D spaces.
Key trigonometric concepts for programmers:
- Sine, cosine, and tangent functions
- Radians vs. degrees
- Polar coordinates
- Rotation matrices
- Projectile motion calculations
Example of trigonometry in programming (rotating a point around the origin):
function rotatePoint(x, y, angleInDegrees) {
let angleInRadians = angleInDegrees * Math.PI / 180;
let cosTheta = Math.cos(angleInRadians);
let sinTheta = Math.sin(angleInRadians);
let newX = x * cosTheta - y * sinTheta;
let newY = x * sinTheta + y * cosTheta;
return [newX, newY];
}
let [rotatedX, rotatedY] = rotatePoint(3, 4, 90);
console.log(`Rotated point: (${rotatedX}, ${rotatedY})`);
6. Calculus: Optimization and Rate of Change
While not all programming tasks require calculus, it becomes crucial when dealing with optimization problems, physics simulations, or machine learning algorithms. Calculus helps in understanding rates of change and finding optimal solutions.
Key calculus concepts for programmers:
- Limits and continuity
- Derivatives (rates of change)
- Integrals (area under curves)
- Optimization techniques
- Differential equations (for physics simulations)
Example of calculus in programming (simple gradient descent for optimization):
function gradientDescent(initialX, learningRate, iterations) {
let x = initialX;
for (let i = 0; i < iterations; i++) {
// f(x) = x^2, so f'(x) = 2x
let gradient = 2 * x;
x = x - learningRate * gradient;
}
return x;
}
let optimizedX = gradientDescent(5, 0.1, 100);
console.log(`Optimized x: ${optimizedX}`);
7. Statistics and Probability: Data Analysis and Machine Learning
Statistics and probability are fundamental to data science, machine learning, and artificial intelligence. They help in analyzing data, making predictions, and understanding the reliability of results.
Key statistical and probability concepts for programmers:
- Descriptive statistics (mean, median, mode, standard deviation)
- Probability distributions
- Hypothesis testing
- Regression analysis
- Bayesian inference
- Sampling techniques
Example of statistics in programming (calculating mean and standard deviation):
function calculateStats(numbers) {
let sum = numbers.reduce((a, b) => a + b, 0);
let mean = sum / numbers.length;
let squaredDiffs = numbers.map(num => Math.pow(num - mean, 2));
let variance = squaredDiffs.reduce((a, b) => a + b, 0) / numbers.length;
let stdDev = Math.sqrt(variance);
return { mean, stdDev };
}
let data = [2, 4, 4, 4, 5, 5, 7, 9];
let { mean, stdDev } = calculateStats(data);
console.log(`Mean: ${mean}, Standard Deviation: ${stdDev}`);
8. Discrete Mathematics: The Core of Computer Science
Discrete mathematics is at the heart of computer science. It deals with mathematical structures that are fundamentally discrete (as opposed to continuous) and is essential for understanding algorithms, data structures, and computational theory.
Key discrete mathematics concepts for programmers:
- Set theory
- Graph theory
- Combinatorics
- Recurrence relations
- Mathematical induction
- Formal logic
Example of discrete mathematics in programming (finding the shortest path in a graph using Dijkstra’s algorithm):
function dijkstra(graph, start, end) {
let distances = {};
let previous = {};
let nodes = new PriorityQueue();
// Initialize distances
for (let vertex in graph) {
if (vertex === start) {
distances[vertex] = 0;
nodes.enqueue(vertex, 0);
} else {
distances[vertex] = Infinity;
nodes.enqueue(vertex, Infinity);
}
previous[vertex] = null;
}
while (!nodes.isEmpty()) {
let smallest = nodes.dequeue();
if (smallest === end) {
let path = [];
while (previous[smallest]) {
path.push(smallest);
smallest = previous[smallest];
}
path.push(start);
return path.reverse();
}
if (!smallest || distances[smallest] === Infinity) {
continue;
}
for (let neighbor in graph[smallest]) {
let alt = distances[smallest] + graph[smallest][neighbor];
if (alt < distances[neighbor]) {
distances[neighbor] = alt;
previous[neighbor] = smallest;
nodes.enqueue(neighbor, alt);
}
}
}
return distances;
}
// Example usage (requires implementation of PriorityQueue)
let graph = {
'A': {'B': 4, 'C': 2},
'B': {'D': 3, 'E': 1},
'C': {'B': 1, 'D': 5},
'D': {'E': 2},
'E': {}
};
let shortestPath = dijkstra(graph, 'A', 'E');
console.log(`Shortest path: ${shortestPath.join(' -> ')}`);
9. Linear Algebra: Matrices and Vectors in Programming
Linear algebra is crucial for many areas of programming, including computer graphics, machine learning, and data analysis. It provides powerful tools for handling multidimensional data and transformations.
Key linear algebra concepts for programmers:
- Vectors and matrices
- Matrix operations (addition, multiplication, transposition)
- Systems of linear equations
- Eigenvalues and eigenvectors
- Vector spaces and linear transformations
Example of linear algebra in programming (matrix multiplication):
function multiplyMatrices(a, b) {
let rowsA = a.length, colsA = a[0].length,
rowsB = b.length, colsB = b[0].length;
if (colsA !== rowsB) throw new Error("Matrices cannot be multiplied");
let result = new Array(rowsA);
for (let i = 0; i < rowsA; i++) {
result[i] = new Array(colsB).fill(0);
for (let j = 0; j < colsB; j++) {
for (let k = 0; k < colsA; k++) {
result[i][j] += a[i][k] * b[k][j];
}
}
}
return result;
}
let matrixA = [[1, 2], [3, 4]];
let matrixB = [[5, 6], [7, 8]];
let product = multiplyMatrices(matrixA, matrixB);
console.log("Matrix product:", product);
10. Boolean Algebra: The Logic Behind Programming
Boolean algebra is fundamental to understanding how computers process logic. It’s essential for creating conditional statements, designing circuits, and optimizing code.
Key Boolean algebra concepts for programmers:
- Truth tables
- Logical operations (AND, OR, NOT, XOR)
- De Morgan’s laws
- Boolean functions and expressions
- Karnaugh maps for simplification
Example of Boolean algebra in programming:
function booleanLogic(a, b) {
console.log(`a AND b: ${a && b}`);
console.log(`a OR b: ${a || b}`);
console.log(`NOT a: ${!a}`);
console.log(`a XOR b: ${a ^ b}`);
}
booleanLogic(true, false);
11. Number Systems and Binary: The Language of Computers
Understanding different number systems, especially binary, is crucial for low-level programming and working with computer memory. It’s also important for tasks like bitwise operations and data compression.
Key concepts related to number systems:
- Binary, octal, and hexadecimal representations
- Conversion between number systems
- Bitwise operations
- Two’s complement representation
- Floating-point representation
Example of number system conversions in programming:
function numberSystemConversions(decimal) {
console.log(`Decimal: ${decimal}`);
console.log(`Binary: ${decimal.toString(2)}`);
console.log(`Octal: ${decimal.toString(8)}`);
console.log(`Hexadecimal: ${decimal.toString(16)}`);
}
numberSystemConversions(42);
12. Algorithmic Thinking: Problem-Solving in Programming
While not strictly a mathematical skill, algorithmic thinking is closely related to mathematical problem-solving. It involves breaking down complex problems into smaller, manageable steps and designing efficient solutions.
Key aspects of algorithmic thinking:
- Problem decomposition
- Pattern recognition
- Abstract thinking
- Logical reasoning
- Efficiency analysis (time and space complexity)
Example of algorithmic thinking (implementing binary search):
function binarySearch(arr, target) {
let left = 0;
let right = arr.length - 1;
while (left <= right) {
let mid = Math.floor((left + right) / 2);
if (arr[mid] === target) {
return mid;
} else if (arr[mid] < target) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return -1; // Target not found
}
let sortedArray = [1, 3, 5, 7, 9, 11, 13, 15];
let targetValue = 7;
let result = binarySearch(sortedArray, targetValue);
console.log(`Index of ${targetValue}: ${result}`);
13. Practical Applications of Math in Programming
Now that we’ve covered the essential math skills, let’s look at some practical applications in various programming domains:
- Web Development: Calculating layouts, animations, and responsive designs often involve geometry and trigonometry.
- Game Development: Physics simulations, collision detection, and 3D graphics heavily rely on linear algebra, calculus, and trigonometry.
- Data Science: Statistical analysis, machine learning algorithms, and data visualization use various mathematical concepts, including linear algebra, calculus, and probability theory.
- Cryptography: Number theory and discrete mathematics are fundamental to creating secure encryption algorithms.
- Computer Graphics: Rendering 3D scenes, creating special effects, and image processing involve complex mathematical operations.
- Artificial Intelligence: Machine learning algorithms, neural networks, and optimization techniques are built on a foundation of linear algebra, calculus, and statistics.
- Robotics: Control systems, motion planning, and computer vision in robotics rely heavily on various mathematical disciplines.
14. Resources for Improving Your Math Skills
If you want to enhance your mathematical skills for programming, here are some valuable resources:
- Online Courses:
- Coursera: “Mathematics for Computer Science” by MIT
- edX: “Introduction to Discrete Mathematics for Computer Science” by UC San Diego
- Khan Academy: Various math courses from basic to advanced levels
- Books:
- “Concrete Mathematics” by Ronald Graham, Donald Knuth, and Oren Patashnik
- “Mathematics for Computer Science” by Eric Lehman, F. Thomson Leighton, and Albert R. Meyer
- “Introduction to Algorithms” by Thomas H. Cormen, Charles E. Leiserson, Ronald L. Rivest, and Clifford Stein
- Websites:
- Project Euler: Mathematical/programming problems
- Brilliant.org: Interactive math and science courses
- 3Blue1Brown: YouTube channel with excellent math visualizations
- Practice Platforms:
- LeetCode: Programming challenges with a strong emphasis on algorithms and data structures
- HackerRank: Coding challenges covering various domains, including mathematics
- CodeSignal: Coding practice with a focus on interview preparation
15. Conclusion: Embracing Math in Your Coding Journey
While it’s possible to be a programmer without being a math expert, having a solid foundation in mathematical concepts can significantly enhance your problem-solving skills and open up new possibilities in your coding career. The essential math skills we’ve discussed – from basic arithmetic to complex topics like linear algebra and calculus – form the backbone of many advanced programming concepts and applications.
Remember that improving your math skills is a gradual process. Start with the basics and progressively work your way up to more advanced topics. As you learn, try to apply these mathematical concepts in your programming projects. This practical application will help reinforce your understanding and demonstrate the real-world relevance of these skills.
Embrace the challenge of learning math for programming, and you’ll find that it not only makes you a better coder but also enhances your analytical thinking and problem-solving abilities in all areas of life. Happy coding and math learning!