Java vs JavaScript: Understanding the Key Differences
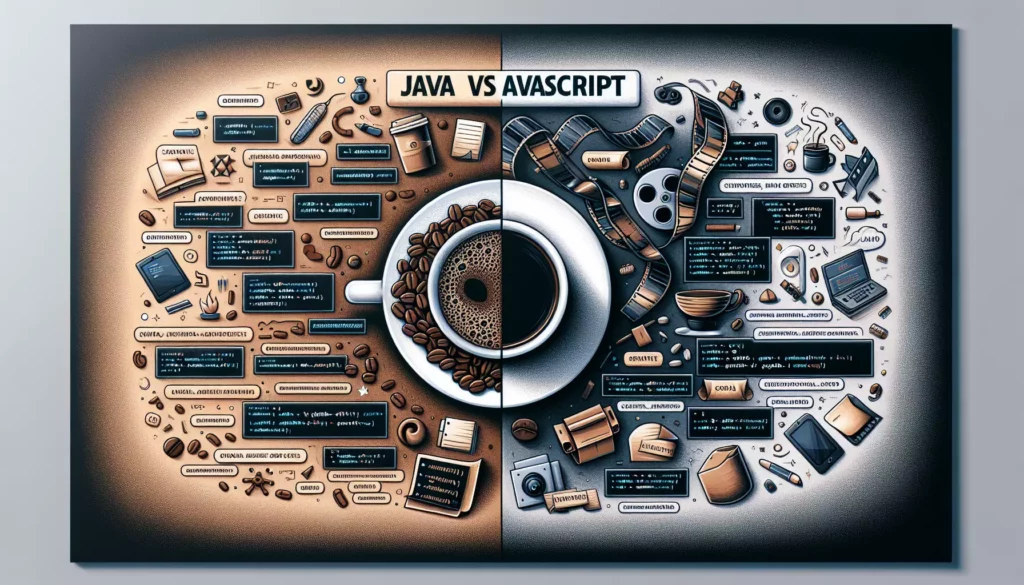
In the world of programming, Java and JavaScript are two of the most popular and widely used languages. Despite their similar names, these languages have distinct characteristics, use cases, and applications. For beginners venturing into the realm of coding, it’s crucial to understand the differences between Java and JavaScript to make informed decisions about which language to learn and use for specific projects. In this comprehensive guide, we’ll explore the key differences between Java and JavaScript, their strengths and weaknesses, and help you determine which language might be best suited for your coding journey.
1. Origin and Purpose
Java
Java was developed by James Gosling and his team at Sun Microsystems (now owned by Oracle Corporation) in 1995. It was designed as a general-purpose programming language with the principle of “Write Once, Run Anywhere” (WORA). This means that Java code can run on any platform that supports the Java Virtual Machine (JVM) without needing to be recompiled.
JavaScript
JavaScript, on the other hand, was created by Brendan Eich at Netscape in 1995. It was initially designed as a scripting language for web browsers to add interactivity to web pages. Over time, JavaScript has evolved to become a versatile language used for both client-side and server-side development.
2. Language Type and Compilation
Java
Java is a statically-typed, compiled language. This means that variable types are checked at compile-time, and the code is compiled into bytecode before it’s run on the Java Virtual Machine (JVM). The compilation process helps catch errors early and can lead to better performance.
JavaScript
JavaScript is a dynamically-typed, interpreted language. Variables in JavaScript can hold values of any type, and type checking occurs at runtime. JavaScript code is interpreted and executed directly by the JavaScript engine in web browsers or runtime environments like Node.js.
3. Syntax and Structure
Java
Java has a more verbose and structured syntax. It requires explicit type declarations, and all code must be part of a class. Here’s a simple “Hello, World!” program in Java:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
JavaScript
JavaScript has a more flexible and concise syntax. It doesn’t require type declarations, and code can be written outside of classes. Here’s the same “Hello, World!” program in JavaScript:
console.log("Hello, World!");
4. Object-Oriented Programming (OOP)
Java
Java is a purely object-oriented programming language. Everything in Java is an object (except for primitive data types), and all code must be written within classes. It supports key OOP concepts like encapsulation, inheritance, and polymorphism.
JavaScript
JavaScript is a multi-paradigm language that supports object-oriented, functional, and imperative programming styles. While it has object-oriented features, it uses prototype-based inheritance rather than class-based inheritance. ES6 and later versions have introduced class syntax, making it more similar to traditional OOP languages.
5. Platform and Runtime Environment
Java
Java applications run on the Java Virtual Machine (JVM), which needs to be installed on the host system. This allows Java programs to be platform-independent, as the JVM handles the interaction between the Java bytecode and the underlying operating system.
JavaScript
JavaScript was originally designed to run in web browsers. However, with the introduction of Node.js, JavaScript can now also run on servers and as standalone applications. Modern browsers come with built-in JavaScript engines, making it easy to run JavaScript code without additional installations.
6. Use Cases and Applications
Java
- Enterprise-level applications
- Android mobile app development
- Server-side web applications
- Big data processing
- Scientific and financial applications
JavaScript
- Front-end web development
- Single-page applications (SPAs)
- Server-side development with Node.js
- Cross-platform mobile app development (e.g., React Native)
- Browser extensions and add-ons
7. Performance and Speed
Java
Java generally offers better performance for computationally intensive tasks due to its compiled nature and the optimizations performed by the JVM. It’s well-suited for large-scale applications that require high performance and scalability.
JavaScript
JavaScript’s performance has improved significantly over the years with advancements in JavaScript engines. While it may not match Java’s performance for heavy computational tasks, it excels in handling asynchronous operations and is well-suited for I/O-bound applications.
8. Memory Management
Java
Java uses automatic memory management through garbage collection. The JVM handles memory allocation and deallocation, which can help prevent memory leaks but may introduce occasional pauses in execution for garbage collection cycles.
JavaScript
JavaScript also uses automatic memory management, but the specifics depend on the JavaScript engine. Modern JavaScript engines employ sophisticated garbage collection algorithms to manage memory efficiently.
9. Typing System
Java
Java is strongly typed, meaning that once a variable is declared with a specific type, it can only hold values of that type. This helps catch type-related errors at compile-time. For example:
int number = 5;
number = "Hello"; // This will result in a compile-time error
JavaScript
JavaScript is loosely typed, allowing variables to hold values of any type. This provides flexibility but can lead to unexpected behavior if not managed carefully. For example:
let number = 5;
number = "Hello"; // This is valid in JavaScript
10. Concurrency and Multithreading
Java
Java has built-in support for multithreading and concurrency. It provides a rich set of tools and libraries for creating and managing threads, synchronization, and parallel processing. This makes Java well-suited for developing complex, concurrent applications.
JavaScript
JavaScript is single-threaded by nature, but it supports asynchronous programming through callbacks, promises, and async/await syntax. While it doesn’t have native multithreading, JavaScript’s event-driven, non-blocking I/O model allows it to handle concurrent operations efficiently, especially in server-side environments like Node.js.
11. Standard Library and Ecosystem
Java
Java has a comprehensive standard library (Java Class Library) that provides a wide range of utilities and APIs for various tasks, from basic data structures to network programming and GUI development. The Java ecosystem also includes a vast collection of third-party libraries and frameworks.
JavaScript
JavaScript has a smaller standard library compared to Java, but it compensates with a vibrant ecosystem of third-party libraries and frameworks. The npm (Node Package Manager) registry hosts millions of packages that can be easily integrated into JavaScript projects.
12. Learning Curve and Accessibility
Java
Java has a steeper learning curve due to its strict syntax, type system, and object-oriented nature. It requires a solid understanding of programming concepts and can be challenging for beginners. However, its structured approach can be beneficial for learning software engineering principles.
JavaScript
JavaScript is often considered more accessible for beginners due to its flexible syntax and the ability to see results quickly in a web browser. Its loosely typed nature allows newcomers to focus on core programming concepts without getting bogged down in type declarations and complex class structures.
13. Community and Support
Java
Java has a large, mature community with extensive documentation, tutorials, and resources. It’s widely used in enterprise environments, ensuring long-term support and regular updates. The Java Community Process (JCP) governs the evolution of the language and platform.
JavaScript
JavaScript boasts one of the largest and most active developer communities. The language evolves rapidly, with new features and improvements introduced regularly through the ECMAScript specifications. The vast ecosystem of libraries and frameworks is supported by a passionate community of developers.
14. Security
Java
Java is known for its robust security features, including the Security Manager, which enforces access controls and protects against malicious code. Its strong typing and compile-time checks also contribute to creating more secure applications.
JavaScript
JavaScript’s security model is primarily based on the same-origin policy and sandboxing in web browsers. While it has improved over time, JavaScript’s dynamic nature and the ability to inject code at runtime can pose security challenges if not properly managed.
15. Mobile App Development
Java
Java is the primary language for native Android app development. Android Studio, the official IDE for Android development, uses Java as one of its main languages (along with Kotlin). Java’s performance and extensive libraries make it well-suited for building robust mobile applications.
JavaScript
JavaScript has gained popularity in mobile app development through frameworks like React Native, Ionic, and PhoneGap. These frameworks allow developers to create cross-platform mobile apps using JavaScript, HTML, and CSS, which can run on both iOS and Android devices.
Conclusion
Java and JavaScript, despite their similar names, are distinct languages with different strengths and use cases. Java excels in building large-scale, enterprise-level applications, Android development, and scenarios requiring high performance and strong typing. JavaScript shines in web development, both on the client and server-side, and offers flexibility and rapid development capabilities.
When choosing between Java and JavaScript, consider the following factors:
- Project requirements and target platform
- Performance needs
- Development speed and flexibility
- Team expertise and available resources
- Long-term maintainability and scalability
For aspiring developers, it’s worth noting that many professionals learn both languages to broaden their skill set and tackle a wider range of projects. Java’s structured approach can provide a solid foundation in software engineering principles, while JavaScript’s ubiquity in web development makes it an essential skill in today’s tech landscape.
Ultimately, the choice between Java and JavaScript depends on your specific goals, project requirements, and personal preferences. Both languages have their place in the programming world, and mastering either (or both) can open up exciting opportunities in software development.
As you embark on your coding journey, remember that the key to success lies not just in choosing the right language, but in developing strong problem-solving skills, understanding fundamental programming concepts, and continuously learning and adapting to new technologies. Whether you start with Java, JavaScript, or both, the world of programming offers endless possibilities for creativity, innovation, and career growth.