How to Use Docker for Containerization in Development: A Comprehensive Guide
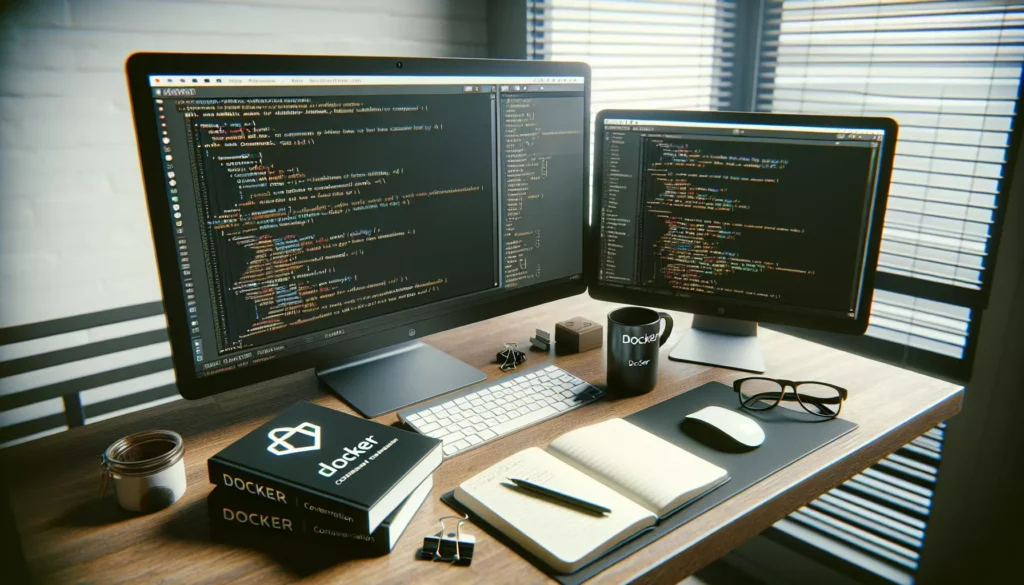
In today’s fast-paced software development world, consistency and efficiency are paramount. Enter Docker, a powerful tool that has revolutionized the way developers build, ship, and run applications. This comprehensive guide will walk you through the process of using Docker for containerization in your development workflow, helping you streamline your projects and boost productivity.
Table of Contents
- What is Docker?
- Why Use Docker for Development?
- Getting Started with Docker
- Docker Basics: Images and Containers
- Creating a Dockerfile
- Using Docker Compose for Multi-Container Applications
- Best Practices for Docker in Development
- Advanced Docker Topics
- Troubleshooting Common Docker Issues
- Conclusion
1. What is Docker?
Docker is an open-source platform that enables developers to automate the deployment, scaling, and management of applications using containerization. Containers are lightweight, standalone, and executable packages that include everything needed to run a piece of software, including the code, runtime, system tools, libraries, and settings.
At its core, Docker allows you to isolate your applications from their environment, ensuring that they work uniformly regardless of where they’re deployed. This consistency between development, testing, and production environments is one of the key benefits of using Docker.
2. Why Use Docker for Development?
There are several compelling reasons to incorporate Docker into your development workflow:
- Consistency: Docker ensures that your application runs the same way on every machine, eliminating the “it works on my machine” problem.
- Isolation: Containers provide a sandboxed environment for your applications, preventing conflicts between different projects or dependencies.
- Portability: Docker containers can run on any system that supports Docker, making it easy to move applications between different environments.
- Efficiency: Containers are lightweight and start quickly, allowing for rapid development and testing cycles.
- Version Control: Docker images can be versioned, making it easy to roll back to previous versions of your application environment if needed.
- Microservices: Docker facilitates a microservices architecture, allowing you to break down your application into smaller, manageable services.
3. Getting Started with Docker
To begin using Docker for development, you’ll need to install Docker on your machine. Here’s a quick guide to get you started:
- Visit the official Docker website (https://www.docker.com/get-started) and download the appropriate version for your operating system.
- Follow the installation instructions for your OS.
- Once installed, open a terminal or command prompt and run the following command to verify the installation:
docker --version
If Docker is installed correctly, you should see the version information displayed.
To test if Docker is working properly, try running the classic “Hello World” container:
docker run hello-world
This command will download a test image and run it in a container. If successful, you’ll see a message explaining that your Docker installation is working correctly.
4. Docker Basics: Images and Containers
Before diving deeper into using Docker for development, it’s crucial to understand two fundamental concepts: images and containers.
Docker Images
A Docker image is a lightweight, standalone, and executable package that includes everything needed to run a piece of software. It contains the application code, runtime, system tools, libraries, and settings. Images are built from a set of instructions called a Dockerfile.
Key points about Docker images:
- Images are read-only templates used to create containers.
- They can be stored in a registry (like Docker Hub) and shared with others.
- Images are composed of layers, which can be cached to speed up builds and reduce storage space.
Docker Containers
A container is a runnable instance of an image. It’s a lightweight, standalone executable package that includes everything needed to run the application.
Key points about Docker containers:
- Containers run in isolation from each other and the host system.
- They can be started, stopped, moved, and deleted.
- Multiple containers can be run from the same image.
- Containers only last as long as they are running; when stopped, any changes made to the container are lost unless volumes are used.
Basic Docker Commands
Here are some essential Docker commands to get you started:
# List all running containers
docker ps
# List all containers (including stopped ones)
docker ps -a
# Pull an image from Docker Hub
docker pull <image_name>
# Run a container from an image
docker run <image_name>
# Stop a running container
docker stop <container_id>
# Remove a container
docker rm <container_id>
# List all images on your system
docker images
# Remove an image
docker rmi <image_id>
5. Creating a Dockerfile
A Dockerfile is a text file that contains a set of instructions for building a Docker image. It specifies the base image to use, environment variables, dependencies to install, files to copy into the image, and commands to run when the container starts.
Here’s an example of a simple Dockerfile for a Python application:
# Use an official Python runtime as the base image
FROM python:3.9-slim
# Set the working directory in the container
WORKDIR /app
# Copy the current directory contents into the container at /app
COPY . /app
# Install any needed packages specified in requirements.txt
RUN pip install --no-cache-dir -r requirements.txt
# Make port 80 available to the world outside this container
EXPOSE 80
# Define environment variable
ENV NAME World
# Run app.py when the container launches
CMD ["python", "app.py"]
To build an image from this Dockerfile, navigate to the directory containing the Dockerfile and run:
docker build -t my-python-app .
This command builds an image and tags it as “my-python-app”. The dot at the end specifies the build context (current directory).
6. Using Docker Compose for Multi-Container Applications
Docker Compose is a tool for defining and running multi-container Docker applications. It allows you to use a YAML file to configure your application’s services, networks, and volumes, and then create and start all the services from your configuration with a single command.
Here’s an example of a simple docker-compose.yml file for a web application with a database:
version: '3'
services:
web:
build: .
ports:
- "5000:5000"
volumes:
- .:/code
environment:
FLASK_ENV: development
redis:
image: "redis:alpine"
To start your application using Docker Compose, run:
docker-compose up
This command will build the images if they don’t exist, create the containers, and start the services defined in your docker-compose.yml file.
7. Best Practices for Docker in Development
To make the most of Docker in your development workflow, consider these best practices:
- Use .dockerignore: Create a .dockerignore file to exclude files and directories that aren’t needed in your Docker image, similar to .gitignore.
- Minimize layers: Combine RUN commands in your Dockerfile to reduce the number of layers and image size.
- Use multi-stage builds: For compiled languages, use multi-stage builds to keep your final image small and free of build tools.
- Don’t run containers as root: Create a non-root user in your Dockerfile and switch to it using the USER instruction.
- Use volumes for persistent data: Use Docker volumes to persist data and share files between the host and containers.
- Tag your images properly: Use meaningful tags for your images to keep track of different versions.
- Use environment variables: Externalize configuration using environment variables instead of hardcoding values.
- Keep images small: Use lightweight base images and remove unnecessary files to keep your images small and efficient.
8. Advanced Docker Topics
As you become more comfortable with Docker, you may want to explore some advanced topics:
Docker Networking
Docker provides several networking options to allow containers to communicate with each other and the outside world. The main types of Docker networks are:
- Bridge: The default network driver. Containers on the same bridge network can communicate.
- Host: Removes network isolation between the container and the Docker host.
- Overlay: Enables communication between containers across multiple Docker daemon hosts.
- Macvlan: Allows you to assign a MAC address to a container, making it appear as a physical device on your network.
- None: Disables all networking for a container.
Docker Volumes
Volumes are the preferred mechanism for persisting data generated by and used by Docker containers. They are completely managed by Docker and are more efficient than bind mounts. Some key benefits of volumes include:
- Easier to back up or migrate than bind mounts
- Can be managed using Docker CLI commands or the Docker API
- Work on both Linux and Windows containers
- Can be safely shared among multiple containers
Docker Swarm
Docker Swarm is a native clustering and orchestration solution for Docker. It allows you to create and manage a swarm of Docker nodes, turning them into a single, virtual Docker host. Some features of Docker Swarm include:
- Cluster management integrated with Docker Engine
- Decentralized design
- Declarative service model
- Scaling
- Desired state reconciliation
- Multi-host networking
- Service discovery
- Load balancing
- Secure by default
- Rolling updates
9. Troubleshooting Common Docker Issues
Even with careful planning and implementation, you may encounter issues when working with Docker. Here are some common problems and their solutions:
Container won’t start
If a container fails to start, try the following:
- Check the container logs:
docker logs <container_id>
- Ensure all required environment variables are set
- Verify that all necessary ports are exposed and not in use by other processes
- Check for conflicts with existing containers or networks
Image build fails
If your image build fails, consider these steps:
- Review your Dockerfile for syntax errors
- Ensure all required files are present in the build context
- Check if you have the necessary permissions to access all resources
- Verify that your base image is compatible with your build instructions
Network connectivity issues
For network-related problems:
- Inspect your Docker networks:
docker network ls
anddocker network inspect <network_name>
- Ensure containers are on the same network if they need to communicate
- Check firewall settings on your host machine
- Verify that required ports are properly exposed and mapped
Performance issues
If you’re experiencing performance problems:
- Monitor resource usage:
docker stats
- Optimize your Dockerfile to reduce image size and build time
- Use multi-stage builds for compiled languages
- Consider using Docker’s experimental features for improved performance
10. Conclusion
Docker has become an indispensable tool in modern software development, offering a powerful solution for containerization that enhances consistency, portability, and efficiency across different environments. By mastering Docker, you can streamline your development workflow, improve collaboration with your team, and ensure that your applications run smoothly from development to production.
As you continue to work with Docker, remember that the ecosystem is constantly evolving. Stay updated with the latest Docker features and best practices to make the most of this powerful technology. Whether you’re building simple web applications or complex microservices architectures, Docker provides the flexibility and tools you need to succeed in today’s fast-paced development landscape.
By following the guidelines and best practices outlined in this comprehensive guide, you’ll be well-equipped to leverage Docker for containerization in your development projects. As you gain experience, don’t hesitate to explore more advanced topics and contribute to the vibrant Docker community. Happy containerizing!