How to Improve Coding Speed and Efficiency: A Comprehensive Guide
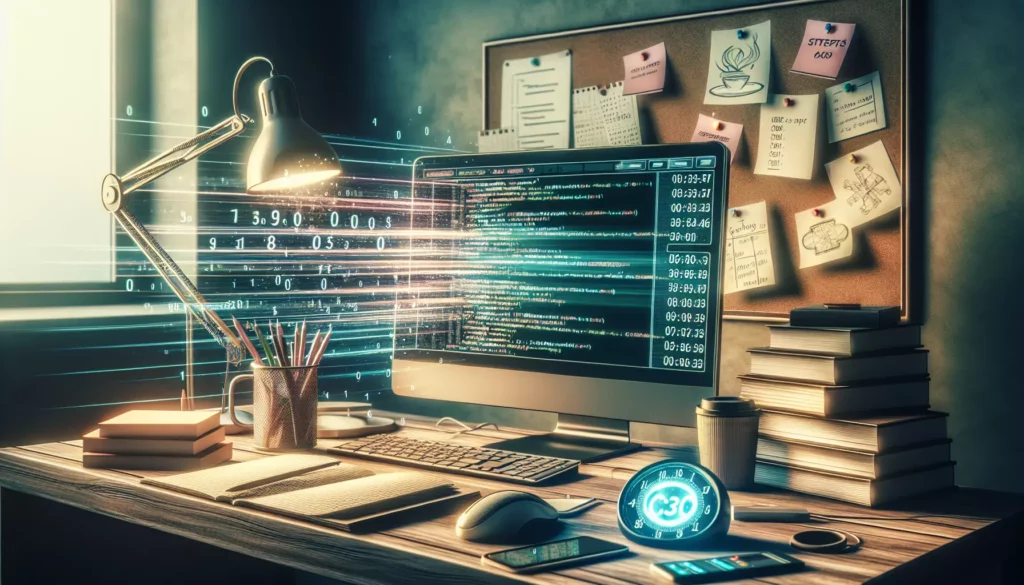
In today’s fast-paced tech world, being able to code quickly and efficiently is a valuable skill that can set you apart from other developers. Whether you’re a beginner looking to enhance your coding abilities or an experienced programmer aiming to boost your productivity, this guide will provide you with actionable strategies to improve your coding speed and efficiency.
Table of Contents
- Understanding the Importance of Coding Speed and Efficiency
- Mastering the Fundamentals
- Leveraging Tools and IDEs
- Mastering Keyboard Shortcuts
- Utilizing Code Snippets and Templates
- Improving Touch Typing Skills
- Practicing Regularly
- Developing Algorithmic Thinking
- Implementing Effective Time Management Techniques
- Optimizing Code Organization
- Enhancing Debugging Skills
- Embracing Continuous Learning
- Engaging in Pair Programming
- Participating in Code Reviews
- Mastering Version Control
- Automating Repetitive Tasks
- Staying Focused and Avoiding Distractions
- Conclusion
1. Understanding the Importance of Coding Speed and Efficiency
Before diving into specific techniques, it’s crucial to understand why coding speed and efficiency matter. In the competitive world of software development, being able to write high-quality code quickly can lead to:
- Increased productivity and output
- Faster project completion times
- More time for testing and refining code
- Improved problem-solving abilities
- Enhanced career opportunities
However, it’s important to note that speed should never come at the expense of code quality. The goal is to find the right balance between writing code quickly and maintaining high standards of readability, maintainability, and performance.
2. Mastering the Fundamentals
To improve your coding speed and efficiency, you need a solid foundation in programming fundamentals. This includes:
- Understanding core programming concepts (variables, data types, control structures, functions, etc.)
- Mastering the syntax and features of your primary programming language(s)
- Familiarizing yourself with common algorithms and data structures
- Learning design patterns and best practices
Platforms like AlgoCademy offer interactive coding tutorials and resources that can help you strengthen your foundational knowledge. By mastering these fundamentals, you’ll be able to write code more quickly and confidently, without constantly referring to documentation or searching for basic syntax.
3. Leveraging Tools and IDEs
Modern Integrated Development Environments (IDEs) and coding tools can significantly boost your productivity. Some key features to look for and utilize include:
- Code completion and suggestions
- Syntax highlighting
- Error detection and quick fixes
- Refactoring tools
- Integrated debugging
- Version control integration
Popular IDEs like Visual Studio Code, IntelliJ IDEA, and PyCharm offer these features and more. Invest time in learning your chosen IDE’s capabilities to maximize your efficiency.
4. Mastering Keyboard Shortcuts
One of the quickest ways to improve your coding speed is to reduce your reliance on the mouse. Learn and practice keyboard shortcuts for common actions in your IDE and operating system. Some essential shortcuts to master include:
- Copy, cut, and paste
- Find and replace
- Navigate between files and within a file
- Comment/uncomment code
- Refactor code
- Run and debug your application
Most IDEs allow you to customize shortcuts to suit your preferences. Create a cheat sheet of your most-used shortcuts and practice them regularly until they become second nature.
5. Utilizing Code Snippets and Templates
Code snippets and templates can save you time by providing reusable blocks of code for common patterns or structures. Many IDEs offer built-in snippet functionality, allowing you to create and use custom snippets. For example, in Visual Studio Code, you can create a snippet for a basic HTML structure:
<!-- HTML Boilerplate Snippet -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>${1:Document}</title>
</head>
<body>
${2}
</body>
</html>
By using snippets, you can quickly generate boilerplate code and focus on the unique aspects of your project.
6. Improving Touch Typing Skills
While it may seem basic, improving your typing speed and accuracy can have a significant impact on your overall coding efficiency. Practice touch typing regularly using online tools like TypeRacer or 10FastFingers. Aim to increase your words per minute (WPM) while maintaining high accuracy. Additionally, practice typing code-specific characters and symbols, as these are often used in programming but less common in regular typing exercises.
7. Practicing Regularly
As with any skill, regular practice is key to improving your coding speed and efficiency. Set aside time each day to work on coding exercises, algorithms, and personal projects. Platforms like AlgoCademy offer a wide range of coding challenges and problems that can help you hone your skills. Try to solve problems under time constraints to simulate real-world scenarios and improve your ability to code quickly under pressure.
8. Developing Algorithmic Thinking
Improving your algorithmic thinking skills can help you approach problems more efficiently and write optimized code faster. Practice breaking down complex problems into smaller, manageable steps. Study common algorithms and data structures, and learn when to apply them. Resources like AlgoCademy’s algorithm tutorials can be invaluable in developing this skill.
For example, consider the following problem: “Find the two numbers in an array that add up to a given target sum.” An efficient solution using a hash table might look like this:
def find_two_sum(nums, target):
num_dict = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_dict:
return [num_dict[complement], i]
num_dict[num] = i
return []
# Example usage
numbers = [2, 7, 11, 15]
target_sum = 9
result = find_two_sum(numbers, target_sum)
print(f"Indices of the two numbers: {result}")
By understanding and applying efficient algorithms like this, you can solve problems more quickly and write optimized code.
9. Implementing Effective Time Management Techniques
Efficient coding isn’t just about writing code quickly; it’s also about managing your time effectively. Consider implementing time management techniques such as:
- The Pomodoro Technique: Work in focused 25-minute intervals, followed by short breaks
- Time boxing: Allocate specific time blocks for different tasks or features
- To-do lists and prioritization: Organize your tasks and focus on high-priority items first
These techniques can help you maintain focus, avoid burnout, and make steady progress on your coding projects.
10. Optimizing Code Organization
Well-organized code is easier to navigate, understand, and modify, which can significantly improve your coding speed. Follow these best practices:
- Use meaningful variable and function names
- Keep functions small and focused on a single task
- Group related code together
- Use comments to explain complex logic or algorithms
- Follow consistent formatting and style guidelines
Consider using a style guide or linter to enforce consistent code style across your projects. For example, in Python, you might use the PEP 8 style guide and a tool like flake8 to check your code for style violations.
11. Enhancing Debugging Skills
Efficient debugging can save you hours of frustration and wasted time. Improve your debugging skills by:
- Learning to use debugging tools in your IDE effectively
- Understanding common error messages and their causes
- Implementing logging in your code for easier troubleshooting
- Practicing rubber duck debugging (explaining your code to an inanimate object to find issues)
For example, in Python, you can use the built-in logging module to add informative log messages to your code:
import logging
logging.basicConfig(level=logging.DEBUG, format='%(asctime)s - %(levelname)s - %(message)s')
def complex_function(x, y):
logging.debug(f"Entering complex_function with x={x}, y={y}")
result = x * y
logging.info(f"Calculation result: {result}")
return result
complex_function(5, 3)
By adding strategic log messages, you can more easily track the flow of your program and identify issues.
12. Embracing Continuous Learning
The field of programming is constantly evolving, with new languages, frameworks, and best practices emerging regularly. Stay up-to-date with the latest developments in your field by:
- Following tech blogs and news sites
- Attending conferences and meetups (virtual or in-person)
- Participating in online coding communities
- Taking online courses or tutorials on new technologies
Continuous learning will help you discover new tools and techniques that can improve your coding efficiency.
13. Engaging in Pair Programming
Pair programming, where two developers work together on the same code, can be an excellent way to improve your coding speed and efficiency. Benefits of pair programming include:
- Immediate code review and feedback
- Sharing of knowledge and best practices
- Reduced likelihood of errors and bugs
- Improved problem-solving through collaboration
If you’re working remotely, tools like Visual Studio Code’s Live Share extension or JetBrains’ Code With Me feature can facilitate pair programming sessions.
14. Participating in Code Reviews
Regularly participating in code reviews, both as a reviewer and a submitter, can help you identify areas for improvement in your coding speed and efficiency. Code reviews provide opportunities to:
- Learn from others’ coding styles and techniques
- Identify common mistakes or inefficiencies in your code
- Practice explaining your code and design decisions
- Stay accountable for writing clean, efficient code
Many version control platforms, such as GitHub and GitLab, offer built-in code review features to streamline this process.
15. Mastering Version Control
Proficiency with version control systems like Git can significantly improve your coding efficiency. Key skills to develop include:
- Creating and managing branches
- Committing changes frequently and with meaningful messages
- Merging and resolving conflicts
- Using advanced features like rebasing and cherry-picking
For example, here’s a series of Git commands to create a new branch, make changes, and merge them back to the main branch:
# Create and switch to a new branch
git checkout -b feature/new-feature
# Make changes and commit them
git add .
git commit -m "Implement new feature"
# Switch back to the main branch and merge changes
git checkout main
git merge feature/new-feature
# Delete the feature branch after successful merge
git branch -d feature/new-feature
By mastering version control, you can work more confidently and efficiently, especially when collaborating with others.
16. Automating Repetitive Tasks
Identify repetitive tasks in your development workflow and look for ways to automate them. This can include:
- Writing scripts for common operations
- Setting up continuous integration/continuous deployment (CI/CD) pipelines
- Using task runners or build tools
- Creating custom commands or aliases for frequently used operations
For instance, you could create a shell script to set up a new project with a standard directory structure and initial files:
#!/bin/bash
# Create a new project directory
mkdir $1
cd $1
# Create standard directories
mkdir src tests docs
# Initialize a Git repository
git init
# Create initial files
touch README.md
touch .gitignore
touch src/main.py
touch tests/test_main.py
echo "Project $1 created successfully!"
By automating repetitive tasks, you can focus more on actual coding and problem-solving.
17. Staying Focused and Avoiding Distractions
Maintaining focus is crucial for coding efficiency. Implement strategies to minimize distractions and stay on task:
- Use website blockers to limit access to distracting sites during work hours
- Set up a dedicated workspace free from interruptions
- Use noise-cancelling headphones or background music to maintain focus
- Practice mindfulness or meditation to improve concentration
- Take regular breaks to avoid burnout and maintain productivity
Remember, sustained focus is often more valuable than long hours of distracted work.
Conclusion
Improving your coding speed and efficiency is a continuous journey that requires dedication, practice, and a willingness to learn and adapt. By implementing the strategies outlined in this guide, you can significantly enhance your productivity and effectiveness as a programmer.
Remember that the goal is not just to write code faster, but to produce high-quality, maintainable code more efficiently. As you apply these techniques, always keep code readability and best practices in mind.
Platforms like AlgoCademy can be valuable resources in your journey to become a more efficient coder. With interactive tutorials, coding challenges, and AI-powered assistance, you can practice and refine your skills in a structured environment.
Ultimately, becoming a faster and more efficient coder is about continuous improvement. Regularly assess your progress, identify areas for improvement, and stay curious about new tools and techniques. With persistence and dedication, you’ll be well on your way to becoming a more productive and skilled programmer.