Microservices in Software Architecture: A Comprehensive Guide
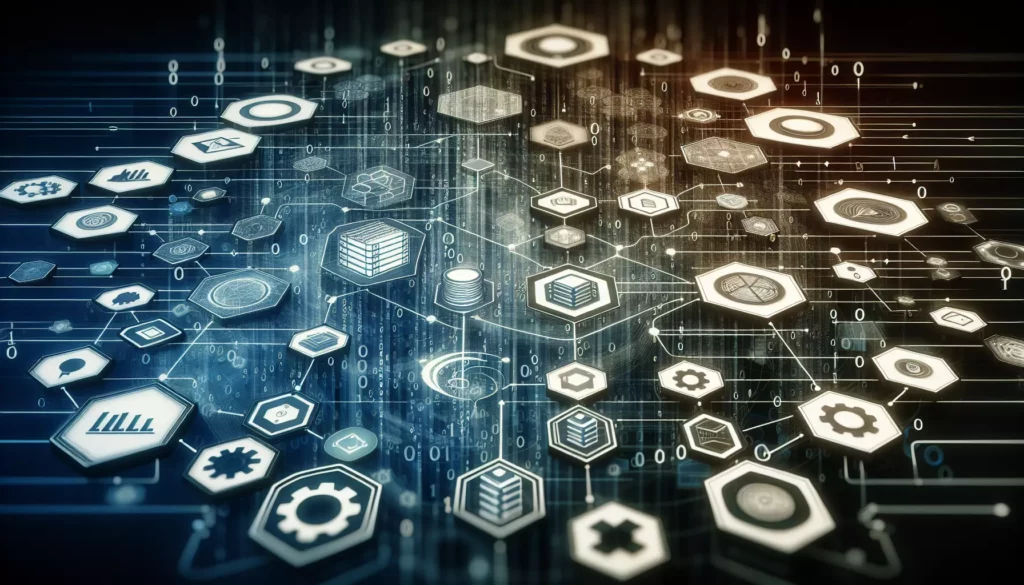
In the ever-evolving landscape of software development, microservices architecture has emerged as a powerful approach to building scalable, flexible, and maintainable applications. This architectural style has gained significant traction in recent years, with many organizations adopting it to overcome the limitations of traditional monolithic systems. In this comprehensive guide, we’ll explore the concept of microservices, their benefits, challenges, and how they fit into the broader context of modern software development.
What Are Microservices?
Microservices, also known as microservices architecture, is an architectural style that structures an application as a collection of small, loosely coupled, and independently deployable services. Each service is focused on performing a specific business function and communicates with other services through well-defined APIs.
Unlike monolithic architectures where all components of an application are tightly integrated into a single unit, microservices break down the application into smaller, more manageable pieces. This approach allows for greater flexibility, scalability, and easier maintenance of complex systems.
Key Characteristics of Microservices
To better understand microservices, let’s explore their key characteristics:
- Decomposition by Business Capability: Each microservice is designed to handle a specific business function or capability.
- Independence: Microservices can be developed, deployed, and scaled independently of one another.
- Decentralized Data Management: Each service typically manages its own database, allowing for polyglot persistence.
- Smart Endpoints and Dumb Pipes: Services communicate through simple, lightweight protocols like HTTP/REST or messaging queues.
- Autonomy: Development teams have the freedom to choose the most appropriate technology stack for each service.
- Resilience: Failure in one service doesn’t necessarily affect the entire system.
- Scalability: Individual services can be scaled independently based on demand.
- Continuous Delivery: Microservices facilitate easier and more frequent deployments.
Benefits of Microservices Architecture
The adoption of microservices brings several advantages to software development:
1. Improved Scalability
With microservices, you can scale individual components of your application independently. This granular scalability allows you to allocate resources more efficiently, scaling only the services that need it rather than the entire application.
2. Enhanced Flexibility and Agility
Microservices enable teams to develop, test, and deploy services independently. This flexibility accelerates the development process and allows for faster time-to-market for new features.
3. Technology Diversity
Different services can use different technology stacks, allowing teams to choose the best tools for each specific task. This polyglot approach can lead to more efficient and effective solutions.
4. Improved Fault Isolation
In a microservices architecture, a failure in one service doesn’t necessarily bring down the entire application. This isolation improves the overall resilience and reliability of the system.
5. Easier Maintenance and Updates
Smaller, focused services are easier to understand, maintain, and update. This simplifies the process of making changes and reduces the risk associated with updates.
6. Better Alignment with Business Needs
By organizing services around business capabilities, microservices can better align with the organizational structure and business goals.
Challenges of Implementing Microservices
While microservices offer numerous benefits, they also come with their own set of challenges:
1. Increased Complexity
Managing a distributed system of multiple services can be more complex than a monolithic application. This complexity extends to deployment, monitoring, and troubleshooting.
2. Inter-service Communication
As services need to communicate with each other, managing these interactions and ensuring reliability can be challenging, especially in a distributed environment.
3. Data Consistency
With each service potentially having its own database, maintaining data consistency across the system becomes more difficult.
4. Testing
Testing a microservices-based application can be more complex, as it involves testing not only individual services but also their interactions.
5. Operational Overhead
Microservices require more operational support, including deployment, monitoring, and management of multiple services.
Implementing Microservices: Best Practices
To successfully implement microservices, consider the following best practices:
1. Start Small
Begin by identifying a small, well-defined part of your application that can be separated into a microservice. Gradually expand from there.
2. Use Domain-Driven Design (DDD)
Apply DDD principles to define service boundaries based on business domains, ensuring each service aligns with a specific business capability.
3. Implement API Gateways
Use API gateways to manage and route requests to appropriate services, providing a single entry point for clients.
4. Adopt Containerization
Use container technologies like Docker to package and deploy microservices, ensuring consistency across different environments.
5. Implement Robust Monitoring and Logging
Set up comprehensive monitoring and logging solutions to track the performance and health of your microservices ecosystem.
6. Use Circuit Breakers
Implement circuit breakers to prevent cascading failures when a service becomes unavailable or experiences high latency.
7. Automate Deployment and Testing
Leverage CI/CD pipelines to automate the building, testing, and deployment of microservices.
Microservices and DevOps
Microservices architecture and DevOps practices often go hand in hand. The principles of DevOps, such as continuous integration, continuous delivery, and automation, align well with the microservices approach. Here’s how they complement each other:
Continuous Integration and Delivery
Microservices enable more frequent and smaller deployments, which is a core principle of CI/CD. Each service can have its own CI/CD pipeline, allowing for faster and more targeted updates.
Infrastructure as Code
Using infrastructure as code (IaC) tools like Terraform or Ansible becomes crucial in managing the complex infrastructure required for microservices.
Automated Testing
Comprehensive automated testing, including unit tests, integration tests, and end-to-end tests, is essential for ensuring the reliability of microservices-based applications.
Monitoring and Observability
DevOps practices emphasize the importance of monitoring and observability, which are critical for managing the complexity of microservices architectures.
Microservices and Cloud Computing
Cloud computing platforms provide an ideal environment for deploying and managing microservices. Here’s how cloud services support microservices architecture:
Containerization and Orchestration
Cloud providers offer managed container services (like Kubernetes) that simplify the deployment and scaling of microservices.
Serverless Computing
Serverless platforms (like AWS Lambda or Azure Functions) can be used to implement microservices, allowing for even greater scalability and reduced operational overhead.
Managed Databases
Cloud-based managed database services support the polyglot persistence often used in microservices architectures.
API Management
Cloud providers offer API management services that can be used to create and manage APIs for microservices.
Microservices in Practice: A Simple Example
To illustrate how microservices work in practice, let’s consider a simple e-commerce application. In a monolithic architecture, all functionalities would be part of a single application. In a microservices architecture, it might be broken down as follows:
- User Service: Handles user authentication and management
- Product Catalog Service: Manages product information
- Order Service: Processes and manages orders
- Payment Service: Handles payment processing
- Shipping Service: Manages shipping and delivery
Each of these services would have its own database and API. They would communicate with each other as needed to fulfill user requests. For example, when a user places an order, the process might look like this:
- The User Service authenticates the user.
- The Order Service creates a new order.
- The Order Service communicates with the Product Catalog Service to update inventory.
- The Payment Service processes the payment.
- Upon successful payment, the Shipping Service is notified to prepare for shipment.
Here’s a simple example of how the Order Service might be implemented in Node.js:
const express = require('express');
const app = express();
const axios = require('axios');
app.use(express.json());
app.post('/orders', async (req, res) => {
try {
const { userId, products } = req.body;
// Validate user
const userResponse = await axios.get(`http://user-service/users/${userId}`);
if (!userResponse.data.isValid) {
return res.status(401).json({ error: 'Invalid user' });
}
// Check product availability
const productResponse = await axios.post('http://product-service/check-availability', { products });
if (!productResponse.data.available) {
return res.status(400).json({ error: 'Products not available' });
}
// Create order
const order = {
userId,
products,
status: 'created',
createdAt: new Date()
};
// Save order to database (implementation omitted)
const savedOrder = await saveOrderToDatabase(order);
// Initiate payment
await axios.post('http://payment-service/process', { orderId: savedOrder.id });
res.status(201).json(savedOrder);
} catch (error) {
res.status(500).json({ error: 'Internal server error' });
}
});
app.listen(3000, () => console.log('Order Service listening on port 3000'));
This example demonstrates how the Order Service interacts with other services (User Service, Product Service, Payment Service) to process an order. Each service is responsible for its own specific functionality, and they communicate via HTTP requests.
Microservices and Algorithmic Thinking
While microservices architecture is more about system design than algorithms, it does require a certain type of algorithmic thinking. Here are some ways in which algorithmic thinking applies to microservices:
1. Service Decomposition
Breaking down a monolithic application into microservices requires analytical thinking similar to breaking down a complex problem into smaller, manageable sub-problems in algorithm design.
2. Inter-service Communication
Designing efficient communication patterns between services involves considerations similar to designing efficient algorithms, such as minimizing network calls and optimizing data transfer.
3. Data Consistency
Maintaining data consistency across distributed services often involves implementing distributed algorithms, such as two-phase commit or eventual consistency mechanisms.
4. Service Discovery
Implementing service discovery in a microservices architecture involves algorithms for efficiently locating and routing requests to the appropriate service instances.
5. Load Balancing
Designing efficient load balancing algorithms is crucial for distributing traffic across multiple instances of a service.
Preparing for Microservices-related Interview Questions
If you’re preparing for technical interviews, especially for positions involving microservices architecture, here are some topics you should be familiar with:
- Principles of microservices architecture
- Advantages and disadvantages of microservices compared to monolithic architectures
- Strategies for breaking down monolithic applications into microservices
- Inter-service communication patterns (synchronous vs asynchronous, REST vs message queues)
- API gateway patterns and implementation
- Data management in microservices (database per service, event sourcing)
- Service discovery and registration
- Containerization and orchestration (Docker, Kubernetes)
- Monitoring and logging in distributed systems
- Handling failures and implementing resilience patterns (circuit breakers, retries, timeouts)
- Testing strategies for microservices
- CI/CD practices for microservices
Be prepared to discuss real-world scenarios, trade-offs in design decisions, and how you would approach solving specific problems in a microservices context.
Conclusion
Microservices architecture represents a significant shift in how we design and build software systems. By breaking down applications into smaller, manageable services, it offers improved scalability, flexibility, and maintainability. However, it also introduces new challenges in terms of complexity and operational overhead.
As with any architectural approach, the decision to adopt microservices should be based on careful consideration of your specific needs and constraints. It’s not a one-size-fits-all solution, and in some cases, a monolithic or modular monolithic approach might be more appropriate.
Understanding microservices is increasingly important for software developers, especially those aspiring to work in large-scale, distributed systems. Whether you’re building a new application from scratch or considering how to evolve an existing system, a solid grasp of microservices principles and best practices can be a valuable addition to your skill set.
As you continue your journey in software development, remember that microservices are just one tool in your architectural toolbox. The key is to understand the principles behind them and know when and how to apply them effectively to solve real-world problems.