How to Become a Data Scientist from a Programming Background: A Comprehensive Guide
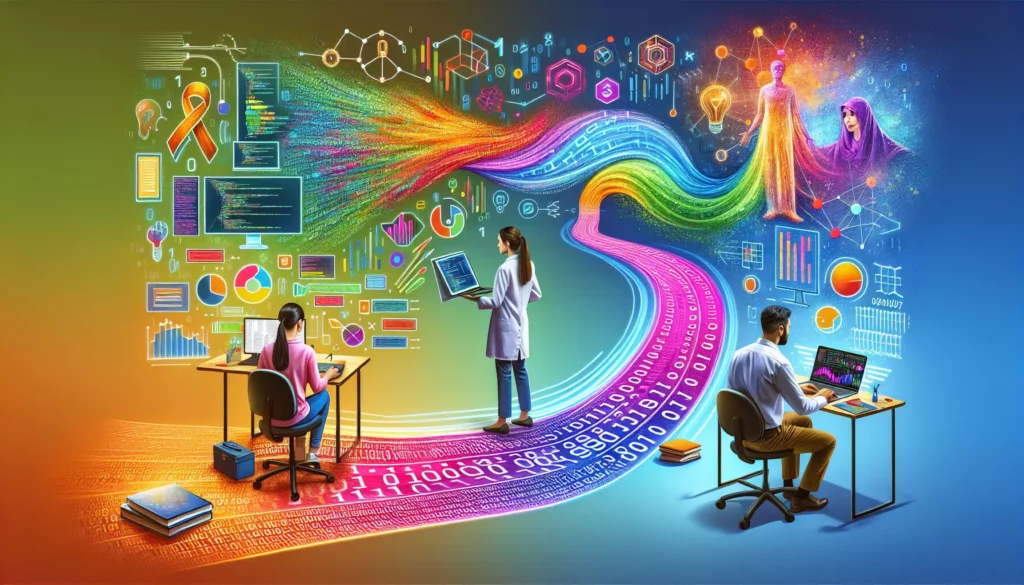
In today’s data-driven world, the role of a data scientist has become increasingly crucial across various industries. For programmers looking to transition into this exciting field, the journey can be both challenging and rewarding. This comprehensive guide will walk you through the steps to become a data scientist, leveraging your existing programming skills and knowledge.
Table of Contents
- Understanding Data Science
- Assessing Your Current Skills
- Essential Skills for Data Scientists
- Building Your Data Science Foundation
- Mastering Data Analysis and Visualization
- Diving into Machine Learning
- Gaining Practical Experience
- Networking and Community Involvement
- Continuing Education and Staying Updated
- Landing Your First Data Science Job
1. Understanding Data Science
Before diving into the transition process, it’s essential to understand what data science entails. Data science is an interdisciplinary field that uses scientific methods, processes, algorithms, and systems to extract knowledge and insights from structured and unstructured data. It combines elements of mathematics, statistics, computer science, and domain expertise to solve complex problems and make data-driven decisions.
As a programmer, you already have a solid foundation in computer science, which gives you a significant advantage. However, data science requires additional skills and knowledge that you’ll need to acquire.
2. Assessing Your Current Skills
Start by evaluating your existing skills and identifying areas where you need improvement. As a programmer, you likely have strengths in:
- Programming languages (e.g., Python, Java, C++)
- Algorithmic thinking and problem-solving
- Software development practices
- Version control systems (e.g., Git)
- Database management
These skills provide a strong foundation for your transition to data science. However, you’ll need to expand your skillset to include:
- Statistical analysis and probability
- Data manipulation and cleaning
- Machine learning algorithms
- Data visualization
- Big data technologies
3. Essential Skills for Data Scientists
To become a successful data scientist, you’ll need to develop proficiency in the following areas:
3.1. Programming Languages
While you may already be proficient in one or more programming languages, focus on mastering languages commonly used in data science:
- Python: The most popular language for data science, known for its simplicity and extensive libraries.
- R: Widely used for statistical computing and graphics.
- SQL: Essential for working with relational databases and querying large datasets.
3.2. Statistics and Mathematics
A strong foundation in statistics and mathematics is crucial for data science. Focus on:
- Descriptive and inferential statistics
- Probability theory
- Linear algebra
- Calculus
3.3. Machine Learning
Understanding machine learning algorithms and their applications is a core component of data science. Key areas to study include:
- Supervised learning (e.g., regression, classification)
- Unsupervised learning (e.g., clustering, dimensionality reduction)
- Deep learning and neural networks
- Ensemble methods
3.4. Data Manipulation and Analysis
Learn to work with various data formats and perform data cleaning, transformation, and analysis using libraries such as:
- Pandas
- NumPy
- SciPy
3.5. Data Visualization
Develop skills in creating compelling visualizations to communicate insights effectively. Popular libraries and tools include:
- Matplotlib
- Seaborn
- Plotly
- Tableau
3.6. Big Data Technologies
Familiarize yourself with big data technologies and distributed computing frameworks:
- Apache Hadoop
- Apache Spark
- Apache Kafka
4. Building Your Data Science Foundation
Now that you understand the essential skills required, it’s time to start building your data science foundation. Here’s a step-by-step approach:
4.1. Strengthen Your Mathematical and Statistical Knowledge
Begin by refreshing your mathematics and statistics skills. Online courses and textbooks can help you build a solid foundation. Some recommended resources include:
- Khan Academy’s Statistics and Probability course
- “Statistics for Data Science” on Coursera
- “Introduction to Statistical Learning” by Gareth James et al.
4.2. Master Python for Data Science
If you’re not already proficient in Python, focus on learning it specifically for data science applications. Key libraries to learn include:
- NumPy for numerical computing
- Pandas for data manipulation and analysis
- Matplotlib and Seaborn for data visualization
Here’s a simple example of using Pandas to read a CSV file and perform basic data analysis:
import pandas as pd
# Read the CSV file
df = pd.read_csv('data.csv')
# Display the first few rows
print(df.head())
# Get basic statistics of the dataset
print(df.describe())
# Calculate the correlation between columns
print(df.corr())
4.3. Learn SQL for Data Manipulation
Enhance your SQL skills to efficiently work with relational databases. Practice writing complex queries and understand concepts like joins, subqueries, and window functions.
4.4. Explore Data Visualization Techniques
Learn to create various types of visualizations using libraries like Matplotlib and Seaborn. Here’s an example of creating a simple scatter plot using Matplotlib:
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y = [2, 4, 6, 8, 10]
plt.scatter(x, y)
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.title('Simple Scatter Plot')
plt.show()
5. Mastering Data Analysis and Visualization
As you build your foundation, focus on developing strong data analysis and visualization skills. These are crucial for extracting insights from data and communicating them effectively.
5.1. Data Cleaning and Preprocessing
Learn techniques for handling missing data, outliers, and inconsistencies in datasets. Practice data cleaning using Pandas:
import pandas as pd
# Load the dataset
df = pd.read_csv('messy_data.csv')
# Handle missing values
df.fillna(df.mean(), inplace=True)
# Remove duplicates
df.drop_duplicates(inplace=True)
# Convert data types
df['date'] = pd.to_datetime(df['date'])
# Normalize numerical columns
from sklearn.preprocessing import MinMaxScaler
scaler = MinMaxScaler()
df[['age', 'salary']] = scaler.fit_transform(df[['age', 'salary']])
5.2. Exploratory Data Analysis (EDA)
Develop skills in exploring and understanding datasets through statistical summaries and visualizations. Use techniques like:
- Descriptive statistics
- Correlation analysis
- Distribution plots
- Box plots and violin plots
5.3. Advanced Visualization Techniques
Learn to create more complex and interactive visualizations using libraries like Plotly and Bokeh. Here’s an example of creating an interactive scatter plot with Plotly:
import plotly.express as px
# Load the dataset
df = px.data.iris()
# Create an interactive scatter plot
fig = px.scatter(df, x="sepal_width", y="sepal_length", color="species",
hover_data=['petal_length', 'petal_width'])
fig.show()
6. Diving into Machine Learning
Machine learning is a core component of data science. As you progress in your journey, focus on understanding and implementing various machine learning algorithms.
6.1. Supervised Learning
Start with supervised learning algorithms, including:
- Linear Regression
- Logistic Regression
- Decision Trees
- Random Forests
- Support Vector Machines (SVM)
Here’s an example of implementing a simple linear regression model using scikit-learn:
from sklearn.linear_model import LinearRegression
from sklearn.model_selection import train_test_split
import numpy as np
# Generate sample data
X = np.random.rand(100, 1)
y = 2 * X + 1 + np.random.randn(100, 1) * 0.1
# Split the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Create and train the model
model = LinearRegression()
model.fit(X_train, y_train)
# Make predictions
y_pred = model.predict(X_test)
# Evaluate the model
from sklearn.metrics import mean_squared_error, r2_score
mse = mean_squared_error(y_test, y_pred)
r2 = r2_score(y_test, y_pred)
print(f"Mean Squared Error: {mse}")
print(f"R-squared Score: {r2}")
6.2. Unsupervised Learning
Explore unsupervised learning techniques, such as:
- K-means clustering
- Hierarchical clustering
- Principal Component Analysis (PCA)
- t-SNE
6.3. Deep Learning
Dive into deep learning concepts and frameworks like TensorFlow and PyTorch. Study neural network architectures, including:
- Convolutional Neural Networks (CNNs)
- Recurrent Neural Networks (RNNs)
- Long Short-Term Memory (LSTM) networks
6.4. Model Evaluation and Validation
Learn techniques for evaluating and validating machine learning models, including:
- Cross-validation
- Hyperparameter tuning
- Confusion matrices
- ROC curves and AUC
7. Gaining Practical Experience
To solidify your skills and build a portfolio, focus on gaining practical experience through various projects and real-world applications.
7.1. Personal Projects
Develop personal data science projects that showcase your skills. Some ideas include:
- Analyzing and visualizing public datasets
- Building a recommendation system
- Creating a sentiment analysis model for social media data
- Developing a predictive model for stock prices
7.2. Kaggle Competitions
Participate in Kaggle competitions to practice your skills, learn from others, and potentially earn recognition in the data science community.
7.3. Contribute to Open Source Projects
Find open source data science projects on platforms like GitHub and contribute to them. This will help you gain experience working on real-world problems and collaborating with other data scientists.
7.4. Internships and Freelance Work
Look for internships or freelance opportunities in data science to gain professional experience. Websites like Upwork and Freelancer.com often have data science projects available for freelancers.
8. Networking and Community Involvement
Building a professional network and engaging with the data science community can significantly boost your career transition.
8.1. Attend Data Science Meetups and Conferences
Participate in local data science meetups and attend conferences to learn from experts, share your knowledge, and network with professionals in the field.
8.2. Join Online Communities
Engage with online data science communities on platforms like:
- Reddit (r/datascience, r/MachineLearning)
- Stack Overflow
- Data Science Stack Exchange
- LinkedIn groups
8.3. Follow Influential Data Scientists
Follow and engage with influential data scientists on social media platforms like Twitter and LinkedIn to stay updated on industry trends and insights.
9. Continuing Education and Staying Updated
The field of data science is constantly evolving, so it’s crucial to continue learning and staying updated on the latest developments.
9.1. Online Courses and MOOCs
Regularly take online courses and MOOCs to deepen your knowledge and learn about new techniques and technologies. Some popular platforms include:
- Coursera
- edX
- Udacity
- DataCamp
9.2. Read Research Papers and Blogs
Stay informed about the latest advancements in data science by reading research papers and following influential data science blogs.
9.3. Attend Workshops and Webinars
Participate in workshops and webinars focused on specific data science topics to gain in-depth knowledge and practical skills.
10. Landing Your First Data Science Job
As you build your skills and gain experience, focus on positioning yourself for your first data science role.
10.1. Update Your Resume and LinkedIn Profile
Tailor your resume and LinkedIn profile to highlight your data science skills, projects, and relevant experience. Emphasize how your programming background adds value to your data science capabilities.
10.2. Build an Online Portfolio
Create a personal website or GitHub repository to showcase your data science projects, demonstrating your skills and problem-solving abilities to potential employers.
10.3. Practice Interview Questions
Prepare for data science interviews by practicing common interview questions, including technical questions, case studies, and behavioral questions.
10.4. Consider Entry-Level Positions
Look for entry-level data science positions or roles that combine your programming skills with data analysis, such as data analyst or machine learning engineer roles.
10.5. Leverage Your Network
Utilize your professional network, including contacts from your programming career, to find job opportunities and get referrals.
Conclusion
Transitioning from a programming background to a career in data science is an exciting and rewarding journey. By leveraging your existing skills, focusing on building a strong foundation in statistics and machine learning, and gaining practical experience, you can successfully make the transition to become a data scientist.
Remember that the path to becoming a data scientist is not linear, and it may take time to develop all the necessary skills. Stay persistent, continue learning, and embrace the challenges along the way. With dedication and hard work, you can establish yourself as a valuable data scientist in this rapidly growing field.