10 Beginner-Friendly Programming Projects to Kickstart Your Coding Journey
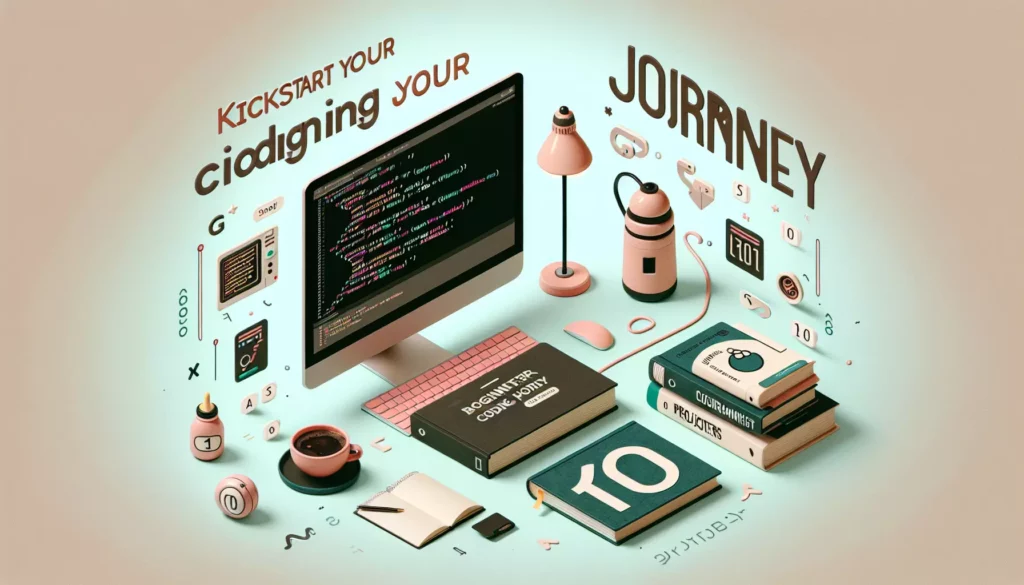
Are you eager to dive into the world of programming but unsure where to start? Look no further! In this comprehensive guide, we’ll explore 10 beginner-friendly programming projects that will help you build a solid foundation in coding while creating practical, fun applications. These projects are designed to be approachable for newcomers to programming, yet challenging enough to help you grow your skills.
Whether you’re a complete novice or have some basic coding knowledge, these projects will provide hands-on experience and boost your confidence as you embark on your programming journey. Let’s dive in!
1. Calculator App
Building a calculator app is a classic beginner project that helps you understand basic programming concepts and user interface design. Here’s why it’s a great starting point:
- Learn about variables and data types
- Practice implementing mathematical operations
- Gain experience with user input and output
- Understand conditional statements and control flow
You can start with a simple command-line calculator and gradually add more features or even create a graphical user interface (GUI) as you progress.
Sample Code (Python):
def add(x, y):
return x + y
def subtract(x, y):
return x - y
def multiply(x, y):
return x * y
def divide(x, y):
if y == 0:
return "Error: Division by zero"
return x / y
print("Select operation:")
print("1. Add")
print("2. Subtract")
print("3. Multiply")
print("4. Divide")
choice = input("Enter choice (1/2/3/4): ")
num1 = float(input("Enter first number: "))
num2 = float(input("Enter second number: "))
if choice == '1':
print(num1, "+", num2, "=", add(num1, num2))
elif choice == '2':
print(num1, "-", num2, "=", subtract(num1, num2))
elif choice == '3':
print(num1, "*", num2, "=", multiply(num1, num2))
elif choice == '4':
print(num1, "/", num2, "=", divide(num1, num2))
else:
print("Invalid input")
2. To-Do List Application
A to-do list application is an excellent project for beginners as it introduces several important programming concepts:
- Working with data structures (e.g., lists or arrays)
- Implementing CRUD operations (Create, Read, Update, Delete)
- Managing user input and data persistence
- Understanding basic file I/O operations
You can start with a simple command-line interface and later expand it to include a graphical user interface or even turn it into a web application.
Sample Code (JavaScript):
let todoList = [];
function addTask(task) {
todoList.push(task);
console.log(`Task "${task}" added to the list.`);
}
function removeTask(index) {
if (index >= 0 && index < todoList.length) {
let removedTask = todoList.splice(index, 1);
console.log(`Task "${removedTask}" removed from the list.`);
} else {
console.log("Invalid task index.");
}
}
function displayTasks() {
console.log("To-Do List:");
todoList.forEach((task, index) => {
console.log(`${index + 1}. ${task}`);
});
}
// Example usage
addTask("Buy groceries");
addTask("Finish homework");
addTask("Call mom");
displayTasks();
removeTask(1);
displayTasks();
3. Guess the Number Game
Creating a “Guess the Number” game is a fun way to practice working with random number generation, user input, and conditional statements. This project will help you learn:
- Generating random numbers
- Implementing game logic with loops
- Handling user input and providing feedback
- Using conditional statements to check game conditions
You can start with a basic version where the computer generates a random number, and the player tries to guess it. Later, you can add features like difficulty levels or a scoring system.
Sample Code (Java):
import java.util.Random;
import java.util.Scanner;
public class GuessTheNumber {
public static void main(String[] args) {
Random random = new Random();
int numberToGuess = random.nextInt(100) + 1;
int numberOfTries = 0;
Scanner input = new Scanner(System.in);
int guess;
boolean win = false;
while (win == false) {
System.out.println("Guess a number between 1 and 100:");
guess = input.nextInt();
numberOfTries++;
if (guess == numberToGuess) {
win = true;
} else if (guess < numberToGuess) {
System.out.println("Your guess is too low");
} else if (guess > numberToGuess) {
System.out.println("Your guess is too high");
}
}
System.out.println("You win!");
System.out.println("The number was: " + numberToGuess);
System.out.println("It took you " + numberOfTries + " tries");
}
}
4. Tic-Tac-Toe Game
Developing a Tic-Tac-Toe game is an excellent way to practice more advanced programming concepts while creating a fun, interactive application. This project will help you learn:
- Implementing game logic and rules
- Working with 2D arrays or matrices
- Handling player turns and input validation
- Checking for win conditions and draw scenarios
Start with a simple command-line version of the game, and as you become more comfortable, you can create a graphical interface or even an AI opponent.
Sample Code (Python):
def print_board(board):
for row in board:
print(" | ".join(row))
print("---------")
def check_winner(board, player):
# Check rows, columns, and diagonals
for i in range(3):
if all(board[i][j] == player for j in range(3)) or \
all(board[j][i] == player for j in range(3)):
return True
if all(board[i][i] == player for i in range(3)) or \
all(board[i][2-i] == player for i in range(3)):
return True
return False
def play_game():
board = [[" " for _ in range(3)] for _ in range(3)]
current_player = "X"
while True:
print_board(board)
row = int(input(f"Player {current_player}, enter row (0-2): "))
col = int(input(f"Player {current_player}, enter column (0-2): "))
if board[row][col] == " ":
board[row][col] = current_player
if check_winner(board, current_player):
print_board(board)
print(f"Player {current_player} wins!")
break
if all(board[i][j] != " " for i in range(3) for j in range(3)):
print_board(board)
print("It's a tie!")
break
current_player = "O" if current_player == "X" else "X"
else:
print("That spot is already taken. Try again.")
play_game()
5. Password Generator
Creating a password generator is a practical project that introduces you to string manipulation, random selection, and basic security concepts. This project will help you learn:
- Working with strings and character sets
- Implementing random selection algorithms
- Understanding basic password security principles
- Handling user input for customization options
You can start with a simple generator that creates random passwords of a fixed length, then add features like customizable length, inclusion of special characters, and password strength evaluation.
Sample Code (JavaScript):
function generatePassword(length, useUppercase, useLowercase, useNumbers, useSpecial) {
const uppercaseChars = "ABCDEFGHIJKLMNOPQRSTUVWXYZ";
const lowercaseChars = "abcdefghijklmnopqrstuvwxyz";
const numberChars = "0123456789";
const specialChars = "!@#$%^&*()_+-=[]{}|;:,<>.?";
let allowedChars = "";
if (useUppercase) allowedChars += uppercaseChars;
if (useLowercase) allowedChars += lowercaseChars;
if (useNumbers) allowedChars += numberChars;
if (useSpecial) allowedChars += specialChars;
if (allowedChars.length === 0) {
return "Error: No character set selected";
}
let password = "";
for (let i = 0; i < length; i++) {
const randomIndex = Math.floor(Math.random() * allowedChars.length);
password += allowedChars[randomIndex];
}
return password;
}
// Example usage
console.log(generatePassword(12, true, true, true, true));
6. Weather App
Building a weather app is an excellent way to learn about working with APIs, handling JSON data, and displaying information to users. This project will help you understand:
- Making HTTP requests to external APIs
- Parsing and working with JSON data
- Implementing error handling for API requests
- Creating a user interface to display weather information
Start with a simple app that displays current weather for a given location, then expand to include features like forecasts, multiple locations, or weather maps.
Sample Code (Python with requests library):
import requests
def get_weather(city):
api_key = "YOUR_API_KEY" # Replace with your actual API key
base_url = "http://api.openweathermap.org/data/2.5/weather"
params = {
"q": city,
"appid": api_key,
"units": "metric"
}
try:
response = requests.get(base_url, params=params)
response.raise_for_status()
weather_data = response.json()
temperature = weather_data["main"]["temp"]
description = weather_data["weather"][0]["description"]
humidity = weather_data["main"]["humidity"]
print(f"Weather in {city}:")
print(f"Temperature: {temperature}°C")
print(f"Description: {description}")
print(f"Humidity: {humidity}%")
except requests.exceptions.RequestException as e:
print(f"An error occurred: {e}")
# Example usage
get_weather("London")
7. URL Shortener
Creating a URL shortener is a great project to learn about web development, databases, and unique ID generation. This project will help you understand:
- Working with web frameworks (e.g., Flask, Express)
- Implementing database operations (e.g., SQLite, MongoDB)
- Generating unique short codes for URLs
- Handling redirects and error cases
Start with a basic version that shortens URLs and stores them in memory, then progress to using a database and adding features like custom short codes or click tracking.
Sample Code (Python with Flask and SQLite):
from flask import Flask, request, redirect
import sqlite3
import string
import random
app = Flask(__name__)
def get_db():
db = sqlite3.connect('urls.db')
db.row_factory = sqlite3.Row
return db
def init_db():
db = get_db()
db.execute('CREATE TABLE IF NOT EXISTS urls (id INTEGER PRIMARY KEY, original TEXT, short TEXT)')
db.close()
def generate_short_code():
characters = string.ascii_letters + string.digits
return ''.join(random.choice(characters) for _ in range(6))
@app.route('/', methods=['GET', 'POST'])
def home():
if request.method == 'POST':
original_url = request.form['url']
short_code = generate_short_code()
db = get_db()
db.execute('INSERT INTO urls (original, short) VALUES (?, ?)', (original_url, short_code))
db.commit()
db.close()
return f"Shortened URL: {request.host_url}{short_code}"
return '<form method="post"><input name="url"><input type="submit"></form>'
@app.route('/<short_code>')
def redirect_to_url(short_code):
db = get_db()
result = db.execute('SELECT original FROM urls WHERE short = ?', (short_code,)).fetchone()
db.close()
if result:
return redirect(result['original'])
return "URL not found", 404
if __name__ == '__main__':
init_db()
app.run(debug=True)
8. Hangman Game
Developing a Hangman game is an excellent way to practice string manipulation, user input handling, and game logic. This project will help you learn:
- Working with strings and character comparisons
- Implementing game rules and logic
- Managing game state and player progress
- Creating an interactive user interface
Start with a basic command-line version of the game, then consider adding features like difficulty levels, word categories, or even a graphical interface.
Sample Code (Python):
import random
def choose_word():
words = ["python", "programming", "computer", "algorithm", "database"]
return random.choice(words)
def display_word(word, guessed_letters):
display = ""
for letter in word:
if letter in guessed_letters:
display += letter
else:
display += "_"
return display
def hangman():
word = choose_word()
word_letters = set(word)
alphabet = set('abcdefghijklmnopqrstuvwxyz')
guessed_letters = set()
lives = 6
while len(word_letters) > 0 and lives > 0:
print("You have", lives, "lives left and you have used these letters: ", ' '.join(guessed_letters))
word_list = display_word(word, guessed_letters)
print("Current word: ", word_list)
guess = input("Guess a letter: ").lower()
if guess in alphabet - guessed_letters:
guessed_letters.add(guess)
if guess in word_letters:
word_letters.remove(guess)
else:
lives = lives - 1
print("Letter is not in the word.")
elif guess in guessed_letters:
print("You have already guessed that letter. Please try again.")
else:
print("Invalid character. Please try again.")
if lives == 0:
print("Sorry, you died. The word was", word)
else:
print("Congratulations! You guessed the word", word, "!!")
hangman()
9. Quiz Game
Creating a quiz game is a fun project that helps you practice working with data structures, user input, and score tracking. This project will teach you:
- Storing and accessing quiz questions and answers
- Implementing a scoring system
- Randomizing question order
- Providing feedback on user answers
Begin with a simple multiple-choice quiz on a single topic, then expand to include different categories, difficulty levels, or even a timer for each question.
Sample Code (JavaScript):
const quizData = [
{
question: "What is the capital of France?",
options: ["London", "Berlin", "Paris", "Madrid"],
correctAnswer: 2
},
{
question: "Which planet is known as the Red Planet?",
options: ["Venus", "Mars", "Jupiter", "Saturn"],
correctAnswer: 1
},
{
question: "What is the largest mammal in the world?",
options: ["Elephant", "Blue Whale", "Giraffe", "Hippopotamus"],
correctAnswer: 1
}
];
function runQuiz() {
let score = 0;
for (let i = 0; i < quizData.length; i++) {
const question = quizData[i];
console.log(`Question ${i + 1}: ${question.question}`);
for (let j = 0; j < question.options.length; j++) {
console.log(`${j + 1}. ${question.options[j]}`);
}
const userAnswer = parseInt(prompt("Enter the number of your answer:")) - 1;
if (userAnswer === question.correctAnswer) {
console.log("Correct!");
score++;
} else {
console.log(`Wrong. The correct answer was: ${question.options[question.correctAnswer]}`);
}
console.log("\n");
}
console.log(`Quiz completed! Your score: ${score}/${quizData.length}`);
}
runQuiz();
10. Personal Portfolio Website
Building a personal portfolio website is an excellent project for beginners to learn web development basics while creating something useful for their career. This project will help you understand:
- HTML structure and semantic elements
- CSS styling and layout techniques
- Basic JavaScript for interactivity
- Responsive design principles
Start with a simple single-page website showcasing your skills and projects, then gradually add more features like a contact form, project galleries, or even a blog section.
Sample Code (HTML, CSS, and JavaScript):
<!-- index.html -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>My Portfolio</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<header>
<h1>John Doe</h1>
<nav>
<ul>
<li><a href="#about">About</a></li>
<li><a href="#projects">Projects</a></li>
<li><a href="#contact">Contact</a></li>
</ul>
</nav>
</header>
<main>
<section id="about">
<h2>About Me</h2>
<p>I'm a passionate web developer with experience in HTML, CSS, and JavaScript.</p>
</section>
<section id="projects">
<h2>My Projects</h2>
<div class="project">
<h3>Project 1</h3>
<p>Description of project 1</p>
</div>
<div class="project">
<h3>Project 2</h3>
<p>Description of project 2</p>
</div>
</section>
<section id="contact">
<h2>Contact Me</h2>
<form id="contact-form">
<input type="text" placeholder="Name" required>
<input type="email" placeholder="Email" required>
<textarea placeholder="Message" required></textarea>
<button type="submit">Send</button>
</form>
</section>
</main>
<footer>
<p>© 2023 John Doe. All rights reserved.</p>
</footer>
<script src="script.js"></script>
</body>
</html>
/* styles.css */
body {
font-family: Arial, sans-serif;
line-height: 1.6;
margin: 0;
padding: 0;
}
header {
background-color: #333;
color: #fff;
text-align: center;
padding: 1rem;
}
nav ul {
list-style-type: none;
padding: 0;
}
nav ul li {
display: inline;
margin-right: 10px;
}
nav ul li a {
color: #fff;
text-decoration: none;
}
main {
padding: 2rem;
}
.project {
background-color: #f4f4f4;
border-radius: 5px;
padding: 1rem;
margin-bottom: 1rem;
}
form {
display: flex;
flex-direction: column;
max-width: 300px;
}
input, textarea, button {
margin-bottom: 1rem;
padding: 0.5rem;
}
footer {
background-color: #333;
color: #fff;
text-align: center;
padding: 1rem;
position: fixed;
bottom: 0;
width: 100%;
}
// script.js
document.getElementById('contact-form').addEventListener('submit', function(event) {
event.preventDefault();
alert('Thank you for your message! I'll get back to you soon.');
});
Conclusion
These 10 beginner-friendly programming projects offer a diverse range of experiences that will help you build a strong foundation in coding. As you work through these projects, you’ll gain practical skills in various programming concepts, problem-solving techniques, and software development practices.
Remember, the key to becoming a proficient programmer is consistent practice and continuous learning. Don’t be afraid to experiment, make mistakes, and seek help when needed. As you complete these projects, consider expanding on them or combining elements from different projects to create more complex applications.
Happy coding, and best of luck on your programming journey!