How to Use Git and GitHub for Version Control: A Comprehensive Guide
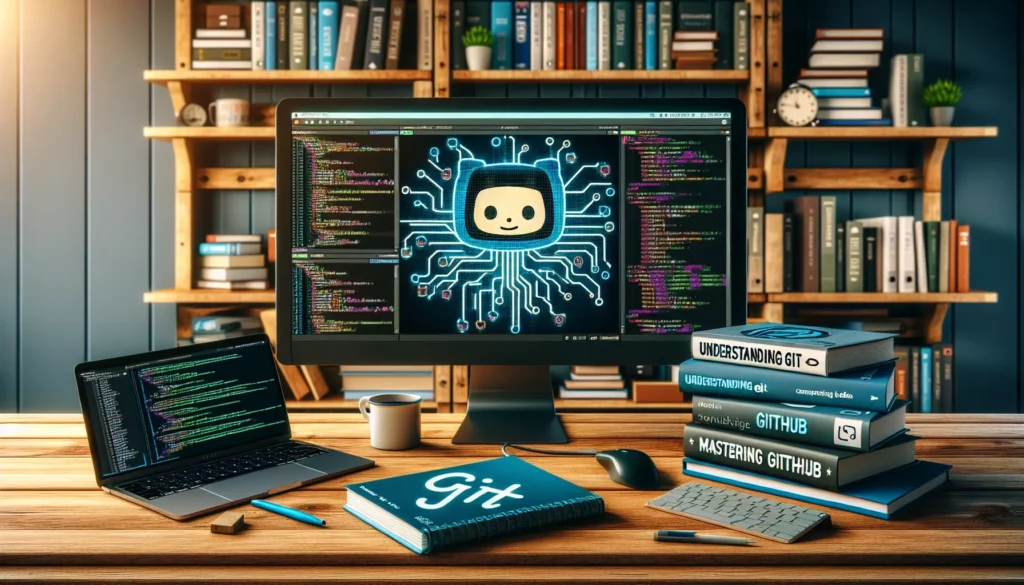
In the world of software development, version control is an essential skill that every programmer should master. Among the various version control systems available, Git stands out as the most widely used and powerful tool. Coupled with GitHub, a web-based platform for hosting Git repositories, it forms an indispensable duo for managing code, collaborating with others, and maintaining a clear history of your projects. In this comprehensive guide, we’ll dive deep into how to use Git and GitHub for effective version control, covering everything from basic concepts to advanced techniques.
Table of Contents
- Introduction to Version Control
- Git Basics
- Introduction to GitHub
- Setting Up Git and GitHub
- Basic Git Workflow
- Branching and Merging
- Collaboration with GitHub
- Advanced Git Techniques
- Best Practices for Git and GitHub
- Troubleshooting Common Issues
- Git and GitHub Integrations
- Conclusion
1. Introduction to Version Control
Version control is a system that helps track changes to files over time. It allows multiple people to work on the same project simultaneously, maintain a history of changes, and revert to previous versions if needed. Git, created by Linus Torvalds in 2005, is a distributed version control system that has become the standard in software development.
Key benefits of using version control include:
- Tracking changes and maintaining a history of your project
- Facilitating collaboration among team members
- Enabling easy experimentation with new features without affecting the main codebase
- Providing a backup mechanism for your code
- Simplifying the process of merging different versions of code
2. Git Basics
Before diving into the practical aspects of using Git and GitHub, it’s crucial to understand some fundamental concepts:
Repository
A repository, or repo, is a directory where Git tracks changes to your files. It contains all of your project’s files and the entire revision history.
Commit
A commit represents a specific point in your project’s history. It’s like a snapshot of your repository at a particular time.
Branch
A branch is a parallel version of your repository. It allows you to work on different parts of your project without affecting the main branch.
Merge
Merging is the process of combining different branches into a single branch, typically to incorporate changes from one branch into another.
Clone
Cloning creates a local copy of a remote repository on your computer.
Push
Pushing uploads your local repository content to a remote repository.
Pull
Pulling fetches content from a remote repository and immediately updates your local repository to match that content.
3. Introduction to GitHub
GitHub is a web-based platform that provides hosting for software development version control using Git. It offers all of the distributed version control and source code management (SCM) functionality of Git, plus its own features:
- A web-based graphical interface
- Access control and collaboration features such as bug tracking, feature requests, task management, and wikis for every project
- A platform for open-source projects and collaboration
- Integration with various development tools and services
GitHub has become an integral part of the software development ecosystem, serving as a hub for millions of developers worldwide to share code, collaborate on projects, and contribute to open-source software.
4. Setting Up Git and GitHub
Installing Git
To get started with Git, you’ll need to install it on your computer. Visit the official Git website and download the appropriate version for your operating system.
Configuring Git
After installation, open a terminal or command prompt and set up your Git configuration:
git config --global user.name "Your Name"
git config --global user.email "your.email@example.com"
Creating a GitHub Account
To use GitHub, you’ll need to create an account. Visit GitHub and sign up for a free account.
Connecting Git to GitHub
To connect your local Git installation to GitHub, you’ll need to set up SSH keys. This allows secure communication between your computer and GitHub. Follow these steps:
- Generate an SSH key pair:
ssh-keygen -t rsa -b 4096 -C "your.email@example.com"
- Copy the public key to your clipboard:
cat ~/.ssh/id_rsa.pub | clip
- Go to GitHub Settings > SSH and GPG keys > New SSH key
- Paste your public key and save
5. Basic Git Workflow
Now that you have Git and GitHub set up, let’s walk through a basic workflow:
Creating a New Repository
To create a new repository on GitHub:
- Log in to GitHub
- Click the “+” icon in the top-right corner
- Select “New repository”
- Fill in the repository name and other details
- Click “Create repository”
Cloning a Repository
To clone a repository to your local machine:
git clone https://github.com/username/repository.git
Making Changes
After making changes to your files, you’ll need to stage and commit them:
git add .
git commit -m "Describe your changes here"
Pushing Changes to GitHub
To upload your changes to GitHub:
git push origin main
Pulling Changes from GitHub
To update your local repository with changes from GitHub:
git pull origin main
6. Branching and Merging
Branching is a powerful feature in Git that allows you to work on different versions of your project simultaneously. Here’s how to work with branches:
Creating a New Branch
git branch new-feature
git checkout new-feature
Or, you can use the shorthand:
git checkout -b new-feature
Switching Between Branches
git checkout main
git checkout new-feature
Merging Branches
To merge changes from one branch into another:
git checkout main
git merge new-feature
Resolving Merge Conflicts
Sometimes, Git can’t automatically merge changes. When this happens, you’ll need to resolve the conflicts manually:
- Open the conflicting files and look for the conflict markers (<<<<<<<, =======, >>>>>>>)
- Edit the files to resolve the conflicts
- Stage the resolved files using
git add
- Complete the merge by creating a new commit
7. Collaboration with GitHub
GitHub provides several features to facilitate collaboration among developers:
Forking a Repository
Forking creates a copy of someone else’s repository in your GitHub account. To fork a repository, click the “Fork” button on the repository’s GitHub page.
Creating Pull Requests
Pull requests (PRs) are a way to suggest changes to a repository. To create a PR:
- Fork the repository
- Create a new branch in your fork
- Make your changes and commit them
- Push the branch to your fork on GitHub
- Go to the original repository and click “New pull request”
- Select your fork and the branch containing your changes
- Fill in the PR description and submit
Reviewing and Merging Pull Requests
As a repository maintainer, you can review PRs, leave comments, and merge them if they’re satisfactory. GitHub provides a web interface for this process, making it easy to collaborate with others.
8. Advanced Git Techniques
Rebasing
Rebasing is an alternative to merging that can create a cleaner project history. To rebase:
git checkout feature-branch
git rebase main
Interactive Rebasing
Interactive rebasing allows you to modify commits as you move them to a new base:
git rebase -i HEAD~3
Cherry-picking
Cherry-picking allows you to apply specific commits from one branch to another:
git cherry-pick <commit-hash>
Stashing
Stashing allows you to temporarily save changes that you don’t want to commit immediately:
git stash
git stash pop
9. Best Practices for Git and GitHub
- Commit often and write meaningful commit messages
- Use branches for new features and bug fixes
- Keep your commits atomic (i.e., each commit should represent a single logical change)
- Pull changes from the remote repository before pushing your own changes
- Use a .gitignore file to exclude unnecessary files from version control
- Review your changes before committing using
git diff
- Use GitHub Issues to track bugs and feature requests
- Utilize GitHub Actions for continuous integration and deployment
10. Troubleshooting Common Issues
Undoing the Last Commit
git reset --soft HEAD~1
Reverting a Commit
git revert <commit-hash>
Resolving “fatal: refusing to merge unrelated histories”
git pull origin main --allow-unrelated-histories
Fixing “fatal: remote origin already exists”
git remote remove origin
git remote add origin <repository-url>
11. Git and GitHub Integrations
Git and GitHub can be integrated with various tools and services to enhance your development workflow:
Continuous Integration/Continuous Deployment (CI/CD)
Use GitHub Actions or integrate with services like Jenkins, Travis CI, or CircleCI to automate your build, test, and deployment processes.
Code Review Tools
Integrate tools like Reviewable or Gerrit to streamline your code review process.
Project Management
Connect GitHub with project management tools like Jira, Trello, or Asana to link commits and pull requests with tasks and issues.
IDE Integration
Most modern Integrated Development Environments (IDEs) like Visual Studio Code, IntelliJ IDEA, and Eclipse offer built-in Git and GitHub integration.
12. Conclusion
Mastering Git and GitHub is crucial for any developer looking to improve their workflow, collaborate effectively, and manage their code efficiently. By understanding the concepts and techniques covered in this guide, you’ll be well-equipped to handle version control in your projects, whether you’re working alone or as part of a team.
Remember that becoming proficient with Git and GitHub takes practice. Don’t be afraid to experiment with different commands and workflows to find what works best for you and your team. As you continue to use these tools, you’ll discover more advanced features and techniques that can further enhance your development process.
For those looking to deepen their understanding of algorithms and data structures, platforms like AlgoCademy offer interactive coding tutorials and resources that can help you apply your Git and GitHub skills to more complex programming challenges. By combining version control proficiency with strong algorithmic thinking, you’ll be well-prepared for technical interviews and real-world software development scenarios.
Keep learning, keep practicing, and don’t hesitate to explore the vast ecosystem of tools and resources available to Git and GitHub users. Happy coding!