Balancing Theory and Practice in Learning to Code: A Comprehensive Guide
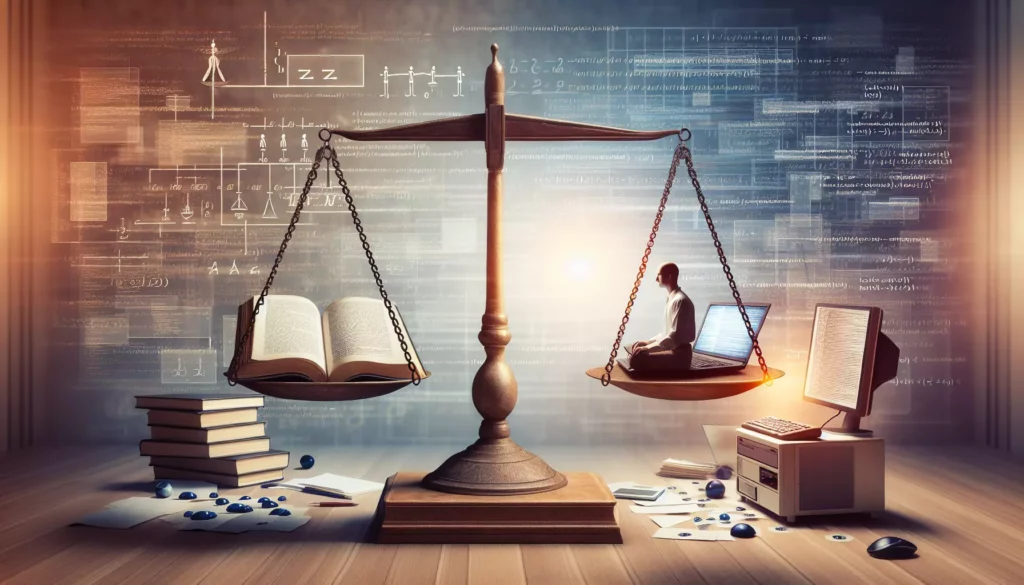
Learning to code is an exciting journey that opens up a world of possibilities in the tech industry. However, one of the most common challenges faced by aspiring programmers is finding the right balance between theoretical knowledge and practical application. In this comprehensive guide, we’ll explore how to effectively combine theory and practice in your coding education, drawing insights from platforms like AlgoCademy that specialize in interactive coding tutorials and resources for learners at various stages of their programming journey.
The Importance of Balancing Theory and Practice
Before diving into specific strategies, it’s crucial to understand why balancing theory and practice is essential in coding education:
- Comprehensive Understanding: Theory provides the foundational knowledge necessary to understand programming concepts, while practice allows you to apply this knowledge in real-world scenarios.
- Problem-Solving Skills: Combining theory and practice enhances your ability to approach and solve complex coding problems efficiently.
- Career Readiness: A balanced approach prepares you for both technical interviews and practical job responsibilities in the tech industry.
- Long-term Growth: Understanding both the ‘why’ (theory) and the ‘how’ (practice) of coding enables continuous learning and adaptation to new technologies.
Strategies for Balancing Theory and Practice
1. Start with Foundational Theory
Begin your coding journey by building a solid theoretical foundation:
- Study basic programming concepts such as variables, data types, control structures, and functions.
- Learn about algorithms and data structures, which form the backbone of efficient coding.
- Understand programming paradigms like object-oriented programming (OOP) and functional programming.
Platforms like AlgoCademy often provide structured learning paths that introduce these concepts in a logical sequence, ensuring you have a strong theoretical base before diving into more complex topics.
2. Apply Theory Through Coding Exercises
As you learn new concepts, immediately put them into practice:
- Start with small coding exercises that focus on specific concepts.
- Use interactive coding platforms that provide immediate feedback on your code.
- Gradually increase the complexity of exercises as you become more comfortable with basic concepts.
For example, after learning about loops, you might practice by writing a program that prints the first 10 Fibonacci numbers:
def print_fibonacci(n):
a, b = 0, 1
for _ in range(n):
print(a, end=" ")
a, b = b, a + b
print_fibonacci(10)
# Output: 0 1 1 2 3 5 8 13 21 34
3. Engage in Project-Based Learning
Projects are an excellent way to bridge the gap between theory and practice:
- Start with small projects that apply multiple concepts you’ve learned.
- Gradually move to larger, more complex projects that mimic real-world applications.
- Use project-based learning platforms or follow tutorials that guide you through building complete applications.
For instance, you could build a simple to-do list application that incorporates concepts like data structures, user input handling, and basic file I/O:
class ToDoList:
def __init__(self):
self.tasks = []
def add_task(self, task):
self.tasks.append(task)
print(f"Task '{task}' added successfully.")
def view_tasks(self):
if not self.tasks:
print("No tasks in the list.")
else:
for i, task in enumerate(self.tasks, 1):
print(f"{i}. {task}")
def remove_task(self, index):
if 1 <= index <= len(self.tasks):
removed_task = self.tasks.pop(index - 1)
print(f"Task '{removed_task}' removed successfully.")
else:
print("Invalid task number.")
todo_list = ToDoList()
while True:
print("\n1. Add Task")
print("2. View Tasks")
print("3. Remove Task")
print("4. Exit")
choice = input("Enter your choice (1-4): ")
if choice == '1':
task = input("Enter the task: ")
todo_list.add_task(task)
elif choice == '2':
todo_list.view_tasks()
elif choice == '3':
index = int(input("Enter the task number to remove: "))
todo_list.remove_task(index)
elif choice == '4':
print("Exiting the program. Goodbye!")
break
else:
print("Invalid choice. Please try again.")
4. Leverage Interactive Coding Platforms
Platforms like AlgoCademy offer a unique blend of theory and practice:
- Use interactive coding environments that allow you to write and run code in real-time.
- Take advantage of step-by-step tutorials that explain concepts and immediately let you apply them.
- Utilize features like AI-powered assistance to get help when you’re stuck on a problem.
5. Participate in Coding Challenges and Competitions
Coding challenges push you to apply theoretical knowledge in time-constrained, problem-solving scenarios:
- Start with platforms that offer daily coding challenges at various difficulty levels.
- Participate in online coding competitions to test your skills against others.
- Use these challenges to identify areas where you need to strengthen your theoretical understanding or practical skills.
6. Study and Implement Algorithms
Algorithmic thinking is crucial for both theoretical understanding and practical problem-solving:
- Study classic algorithms and data structures.
- Implement these algorithms from scratch to deepen your understanding.
- Analyze the time and space complexity of your implementations.
Here’s an example of implementing a binary search algorithm:
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
# Example usage
sorted_array = [1, 3, 5, 7, 9, 11, 13, 15, 17]
target = 7
result = binary_search(sorted_array, target)
print(f"Target {target} found at index: {result}")
# Output: Target 7 found at index: 3
7. Read and Analyze Real-World Code
Studying existing codebases helps bridge the gap between theory and industry practices:
- Explore open-source projects on platforms like GitHub.
- Analyze how experienced developers structure their code and implement complex features.
- Try to contribute to open-source projects to gain practical experience in collaborative coding environments.
8. Teach Others
Teaching is an excellent way to reinforce both theoretical knowledge and practical skills:
- Explain concepts to fellow learners or write blog posts about topics you’ve mastered.
- Participate in coding forums or Q&A platforms to help others solve problems.
- Consider mentoring beginners, which will challenge you to articulate complex concepts clearly.
9. Stay Updated with Industry Trends
The tech industry evolves rapidly, so it’s important to keep your knowledge current:
- Follow tech blogs, podcasts, and news sites to stay informed about new technologies and best practices.
- Attend webinars, conferences, or local meetups to learn from industry experts.
- Experiment with new programming languages, frameworks, or tools to broaden your skill set.
10. Prepare for Technical Interviews
Technical interviews often require a balance of theoretical knowledge and practical coding skills:
- Practice solving algorithmic problems on platforms like AlgoCademy that focus on interview preparation.
- Conduct mock interviews with peers to improve your ability to explain your thought process while coding.
- Review computer science fundamentals and be prepared to discuss the theoretical aspects of your solutions.
Creating a Balanced Learning Plan
To effectively balance theory and practice in your coding education, consider creating a structured learning plan:
- Set Clear Goals: Define what you want to achieve in your coding journey, whether it’s landing a job at a tech company or building a specific type of application.
- Allocate Time: Dedicate specific time slots for studying theory, practicing coding, and working on projects.
- Use a Variety of Resources: Combine textbooks, online courses, coding platforms, and real-world projects in your learning approach.
- Track Progress: Regularly assess your understanding of theoretical concepts and your ability to apply them in practical scenarios.
- Seek Feedback: Engage with coding communities, mentors, or peers to get feedback on your code and approach to problem-solving.
- Reflect and Adjust: Periodically review your learning strategy and make adjustments based on your progress and evolving goals.
Overcoming Common Challenges
As you work to balance theory and practice in your coding education, you may encounter some challenges:
1. Information Overload
Challenge: The vast amount of coding resources and information available can be overwhelming.
Solution: Focus on mastering core concepts first. Use curated learning paths like those offered by AlgoCademy to guide your learning progression.
2. Difficulty in Applying Theory to Practice
Challenge: You may struggle to translate theoretical knowledge into practical coding solutions.
Solution: Start with simple implementations of theoretical concepts. Gradually increase complexity as you become more comfortable. Use platforms that provide guided coding exercises to bridge this gap.
3. Lack of Motivation in Theoretical Study
Challenge: Theoretical aspects of coding might seem dry or disconnected from practical applications.
Solution: Tie theoretical concepts to real-world applications or interesting projects. For example, when studying sorting algorithms, implement them to solve a practical problem like organizing a music playlist.
4. Time Management
Challenge: Balancing time between learning theory and practicing coding can be difficult, especially if you’re learning alongside other commitments.
Solution: Create a structured schedule that allocates time for both theory and practice. Use techniques like the Pomodoro method to manage your study sessions effectively.
5. Imposter Syndrome
Challenge: Feeling like you don’t know enough or aren’t progressing fast enough compared to others.
Solution: Remember that learning to code is a journey. Celebrate small victories and focus on your personal growth rather than comparing yourself to others. Use platforms that provide personalized learning paths to ensure you’re progressing at a suitable pace for your skill level.
Conclusion
Balancing theory and practice in learning to code is essential for developing well-rounded programming skills. By combining foundational knowledge with hands-on coding experience, you’ll be better equipped to tackle complex problems, adapt to new technologies, and succeed in technical interviews.
Remember that platforms like AlgoCademy are designed to help you achieve this balance, offering a mix of theoretical explanations, interactive coding exercises, and real-world project simulations. Utilize these resources alongside your own projects and exploration to create a comprehensive learning experience.
As you progress in your coding journey, continually reassess and adjust your learning approach. Stay curious, embrace challenges, and don’t be afraid to dive deep into both theoretical concepts and practical applications. With persistence and a balanced approach, you’ll build a strong foundation for a successful career in programming.
Happy coding!