Mastering Multiple Programming Languages: Effective Strategies for Polyglot Programmers
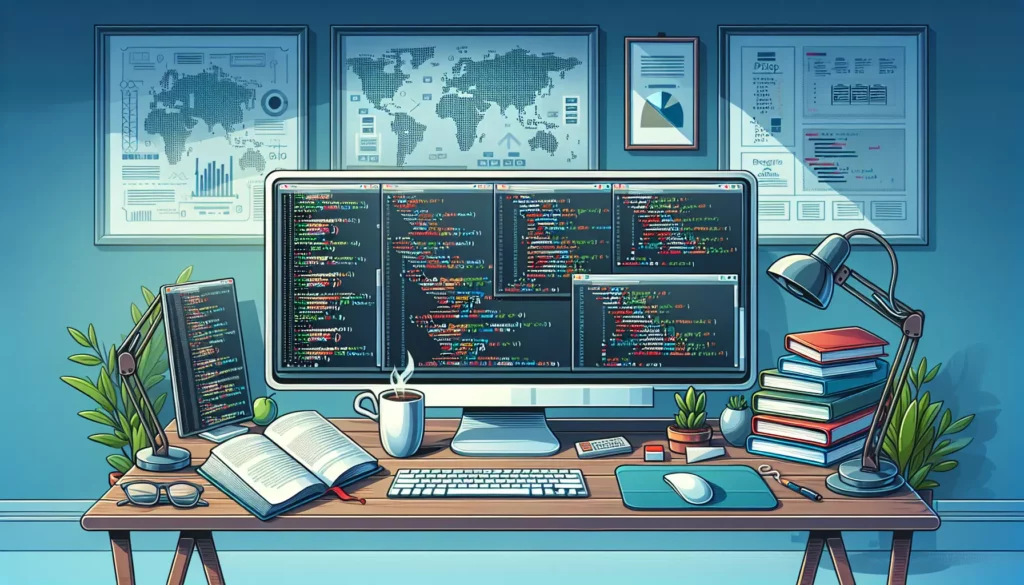
In today’s rapidly evolving tech landscape, being proficient in multiple programming languages is becoming increasingly valuable. Whether you’re a beginner looking to expand your skill set or an experienced developer aiming to stay competitive, learning multiple programming languages can significantly boost your career prospects and problem-solving abilities. In this comprehensive guide, we’ll explore effective strategies for learning and mastering multiple programming languages, drawing insights from the world of coding education and programming skills development.
Why Learn Multiple Programming Languages?
Before diving into strategies, let’s briefly discuss why learning multiple programming languages is beneficial:
- Versatility: Different languages are suited for different tasks. Knowing multiple languages allows you to choose the best tool for each job.
- Broader job opportunities: Many companies use multiple languages in their tech stack. Being proficient in several languages makes you a more attractive candidate.
- Enhanced problem-solving skills: Each language has its own paradigms and approaches, which can broaden your thinking and improve your overall coding abilities.
- Better understanding of programming concepts: Learning multiple languages helps reinforce fundamental concepts and exposes you to different programming paradigms.
- Adaptability: The tech world evolves quickly. Knowing multiple languages makes it easier to adapt to new technologies and frameworks.
Effective Strategies for Learning Multiple Programming Languages
1. Start with a Strong Foundation
Before attempting to learn multiple languages, ensure you have a solid grasp of programming fundamentals. This includes understanding concepts like:
- Variables and data types
- Control structures (if statements, loops)
- Functions and methods
- Object-oriented programming principles
- Data structures and algorithms
Platforms like AlgoCademy offer interactive coding tutorials and resources that can help you build this foundation. Their focus on algorithmic thinking and problem-solving is particularly valuable when preparing to learn multiple languages.
2. Choose Languages Strategically
When deciding which languages to learn, consider the following factors:
- Career goals: Research which languages are in demand in your desired field or industry.
- Complementary languages: Choose languages that complement each other or are commonly used together (e.g., JavaScript and Python, or Java and Kotlin).
- Diverse paradigms: Learn languages that represent different programming paradigms (e.g., object-oriented, functional, procedural) to broaden your understanding.
- Project requirements: If you have specific projects in mind, choose languages that are well-suited for those tasks.
3. Focus on One Language at a Time
While the goal is to learn multiple languages, it’s generally more effective to focus on one language at a time, especially when you’re just starting. This approach allows you to:
- Avoid confusion between syntax and concepts of different languages
- Develop a deeper understanding of each language
- Build confidence and proficiency before moving on to the next language
Once you’ve gained proficiency in one language, you can start learning another while continuing to practice and improve in the first.
4. Leverage Similarities Between Languages
Many programming languages share similar concepts and structures. When learning a new language, identify these similarities to accelerate your learning process. For example:
- If you know Java, learning C# will be easier due to their similar syntax and object-oriented nature.
- Understanding JavaScript can help you quickly grasp TypeScript, as TypeScript is a superset of JavaScript.
- Knowledge of Python can make learning Ruby smoother, as both languages emphasize readability and have similar high-level abstractions.
5. Practice Regularly with Coding Challenges
Consistent practice is key to mastering any programming language. Engage in regular coding exercises and challenges to reinforce your learning. Platforms like AlgoCademy offer a variety of coding challenges that can help you:
- Apply theoretical knowledge to practical problems
- Improve your problem-solving skills
- Prepare for technical interviews, especially for major tech companies
Try solving the same problem in multiple languages to understand the differences and similarities in implementation.
6. Build Projects in Each Language
Theory and small exercises are important, but building actual projects is crucial for truly understanding a language. For each language you’re learning:
- Start with small, manageable projects
- Gradually increase complexity as you become more comfortable
- Try to build similar projects in different languages to compare approaches
- Contribute to open-source projects to gain real-world experience
7. Understand the Ecosystem and Tools
Learning a programming language isn’t just about syntax; it’s also about understanding the ecosystem surrounding it. For each language, familiarize yourself with:
- Popular frameworks and libraries
- Development tools and IDEs
- Package managers
- Testing frameworks
- Community resources and best practices
8. Join Language-Specific Communities
Engaging with the community can significantly enhance your learning experience. For each language you’re learning:
- Join online forums and discussion groups
- Participate in local meetups or user groups
- Attend conferences or webinars
- Follow influential developers and thought leaders on social media
These communities can provide support, answer questions, and keep you updated on the latest developments in each language.
9. Teach Others
One of the most effective ways to solidify your understanding of a language is to teach it to others. This can be done through:
- Writing blog posts or tutorials
- Creating video content
- Mentoring junior developers
- Giving presentations at local meetups
Teaching forces you to articulate concepts clearly and often reveals gaps in your own understanding, prompting further learning.
10. Use Spaced Repetition and Interleaving
To effectively learn and retain information about multiple languages, consider using learning techniques such as:
- Spaced repetition: Review concepts and practice coding in each language at increasing intervals to improve long-term retention.
- Interleaving: Mix your practice of different languages rather than focusing on one for extended periods. This can help you make connections between languages and reinforce your understanding of common programming concepts.
11. Leverage AI-Powered Learning Tools
Take advantage of AI-powered learning tools, like those offered by platforms such as AlgoCademy. These tools can provide:
- Personalized learning paths
- Intelligent code analysis and feedback
- Adaptive difficulty in coding challenges
- Recommendations for areas of improvement
AI assistance can help you identify patterns across languages and provide targeted practice to reinforce your skills.
12. Focus on Problem-Solving, Not Just Syntax
While syntax is important, the ability to solve problems is a more valuable and transferable skill. When learning multiple languages:
- Focus on understanding the problem-solving approach
- Practice breaking down complex problems into smaller, manageable parts
- Learn to think algorithmically and optimize your solutions
- Study design patterns and how they’re implemented in different languages
This approach will make it easier to switch between languages and adapt to new ones in the future.
13. Compare and Contrast Languages
As you learn multiple languages, actively compare and contrast them. This can help you:
- Understand the strengths and weaknesses of each language
- Identify which language is best suited for specific tasks
- Recognize patterns and principles that are common across languages
- Appreciate the unique features and philosophies of each language
Create a comparison chart or document to track these differences and similarities.
14. Set Realistic Goals and Track Progress
Learning multiple programming languages is a significant undertaking. To stay motivated and make steady progress:
- Set clear, achievable goals for each language you’re learning
- Break these goals down into smaller, manageable milestones
- Use tools like GitHub to track your coding activity and projects
- Regularly review your progress and adjust your learning plan as needed
15. Stay Updated with Language Evolution
Programming languages are constantly evolving. To truly master multiple languages:
- Keep up with new language features and updates
- Regularly review and update your existing projects to incorporate new best practices
- Experiment with new language features as they’re released
- Follow language-specific blogs, newsletters, and official documentation
Practical Examples: Learning Across Languages
To illustrate how these strategies can be applied, let’s look at some practical examples of learning across different programming languages.
Example 1: Implementing a Binary Search Tree
Let’s compare the implementation of a binary search tree in Python and Java:
Python Implementation:
class Node:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
class BinarySearchTree:
def __init__(self):
self.root = None
def insert(self, value):
if not self.root:
self.root = Node(value)
else:
self._insert_recursive(self.root, value)
def _insert_recursive(self, node, value):
if value < node.value:
if node.left is None:
node.left = Node(value)
else:
self._insert_recursive(node.left, value)
else:
if node.right is None:
node.right = Node(value)
else:
self._insert_recursive(node.right, value)
def search(self, value):
return self._search_recursive(self.root, value)
def _search_recursive(self, node, value):
if node is None or node.value == value:
return node
if value < node.value:
return self._search_recursive(node.left, value)
return self._search_recursive(node.right, value)
Java Implementation:
class Node {
int value;
Node left;
Node right;
Node(int value) {
this.value = value;
left = null;
right = null;
}
}
class BinarySearchTree {
Node root;
BinarySearchTree() {
root = null;
}
void insert(int value) {
root = insertRecursive(root, value);
}
Node insertRecursive(Node current, int value) {
if (current == null) {
return new Node(value);
}
if (value < current.value) {
current.left = insertRecursive(current.left, value);
} else if (value > current.value) {
current.right = insertRecursive(current.right, value);
}
return current;
}
boolean search(int value) {
return searchRecursive(root, value);
}
boolean searchRecursive(Node current, int value) {
if (current == null) {
return false;
}
if (value == current.value) {
return true;
}
return value < current.value
? searchRecursive(current.left, value)
: searchRecursive(current.right, value);
}
}
By implementing the same data structure in different languages, you can observe:
- Similarities in the overall structure and logic
- Differences in syntax and language-specific features
- How object-oriented principles are applied in each language
- The trade-offs between conciseness (Python) and explicit typing (Java)
Example 2: Solving a Coding Challenge
Let’s solve a common coding challenge – finding the first non-repeating character in a string – in JavaScript and C++:
JavaScript Solution:
function firstNonRepeatingCharacter(str) {
const charCount = {};
// Count occurrences of each character
for (let char of str) {
charCount[char] = (charCount[char] || 0) + 1;
}
// Find the first character with count 1
for (let char of str) {
if (charCount[char] === 1) {
return char;
}
}
// If no non-repeating character found
return null;
}
// Example usage
console.log(firstNonRepeatingCharacter("aabbcdeeff")); // Output: "c"
C++ Solution:
#include <iostream>
#include <string>
#include <unordered_map>
char firstNonRepeatingCharacter(const std::string& str) {
std::unordered_map<char, int> charCount;
// Count occurrences of each character
for (char c : str) {
charCount[c]++;
}
// Find the first character with count 1
for (char c : str) {
if (charCount[c] == 1) {
return c;
}
}
// If no non-repeating character found
return '\0';
}
int main() {
std::string str = "aabbcdeeff";
char result = firstNonRepeatingCharacter(str);
if (result != '\0') {
std::cout << "First non-repeating character: " << result << std::endl;
} else {
std::cout << "No non-repeating character found." << std::endl;
}
return 0;
}
By solving the same problem in different languages, you can observe:
- How each language handles data structures (objects in JavaScript vs. unordered_map in C++)
- Differences in string manipulation and iteration
- Type system differences (dynamic typing in JavaScript vs. static typing in C++)
- Language-specific conventions and best practices
Conclusion
Learning multiple programming languages is a valuable skill that can significantly enhance your capabilities as a developer. By following these strategies and consistently practicing, you can become a polyglot programmer capable of tackling a wide range of programming challenges.
Remember that the journey of learning multiple languages is ongoing. As you progress, you’ll find that each new language becomes easier to learn, and you’ll develop a deeper understanding of programming concepts that transcend any single language.
Platforms like AlgoCademy can be invaluable resources in this journey, offering structured learning paths, coding challenges, and AI-powered assistance to help you master multiple programming languages. By combining these resources with personal projects, community engagement, and consistent practice, you’ll be well on your way to becoming a versatile and highly skilled programmer.
Embrace the challenge, stay curious, and keep coding. The world of polyglot programming awaits!