Best Practices for Writing Clean Code: A Comprehensive Guide
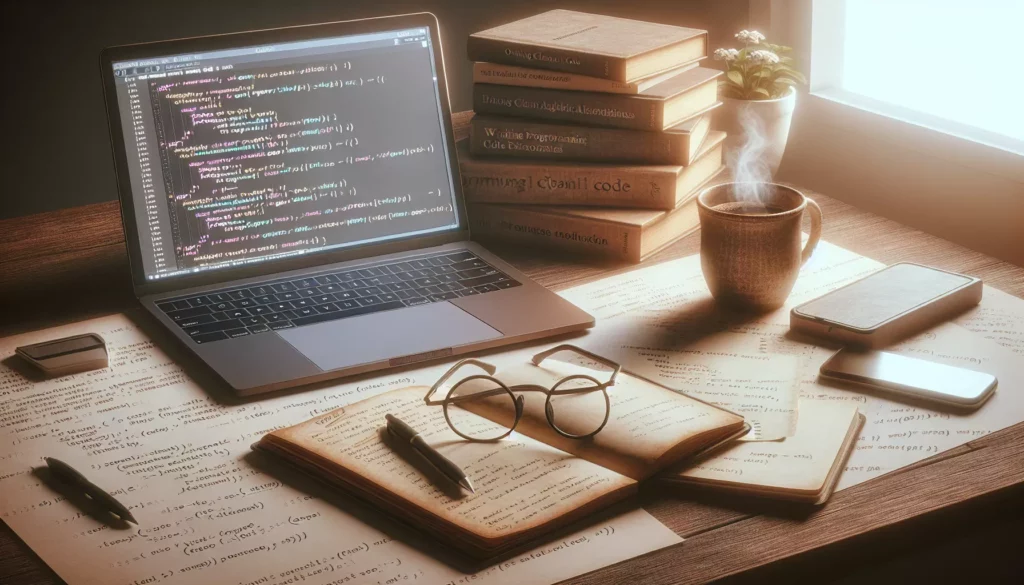
In the world of software development, writing clean code is an essential skill that separates amateur programmers from professionals. Clean code not only makes your work more readable and maintainable but also enhances collaboration and reduces the likelihood of bugs. In this comprehensive guide, we’ll explore the best practices for writing clean code, providing you with the tools and knowledge to elevate your coding skills to the next level.
Table of Contents
- Understanding Clean Code
- Naming Conventions
- Code Organization
- Commenting and Documentation
- Error Handling
- DRY Principle
- SOLID Principles
- Code Formatting
- Testing
- Version Control
- Continuous Improvement
- Tools for Clean Code
- Conclusion
1. Understanding Clean Code
Before diving into specific practices, it’s crucial to understand what clean code really means. Clean code is code that is easy to read, understand, and maintain. It’s not just about making your code work; it’s about making it work elegantly and efficiently.
Robert C. Martin, author of “Clean Code: A Handbook of Agile Software Craftsmanship,” describes clean code as follows:
“Clean code always looks like it was written by someone who cares. There is nothing obvious that you can do to make it better.”
The benefits of writing clean code include:
- Improved readability and maintainability
- Easier debugging and troubleshooting
- Enhanced collaboration among team members
- Reduced technical debt
- Increased productivity in the long run
2. Naming Conventions
One of the most fundamental aspects of clean code is using clear and meaningful names for variables, functions, classes, and other code elements. Good naming conventions can significantly improve code readability and reduce the need for excessive comments.
Guidelines for Naming:
- Be descriptive: Choose names that clearly describe the purpose or function of the element.
- Use intention-revealing names: The name should answer why the variable exists, what it does, or how it is used.
- Avoid abbreviations: Unless they are widely known and accepted in your domain.
- Be consistent: Use the same naming conventions throughout your codebase.
- Follow language-specific conventions: Adhere to the naming conventions of the programming language you’re using.
Examples:
// Bad naming
int d; // elapsed time in days
// Good naming
int elapsedTimeInDays;
// Bad naming
function getUserInfo() {
// ...
}
// Good naming
function fetchUserProfile() {
// ...
}
3. Code Organization
Properly organizing your code is crucial for maintaining clean and manageable codebases. Good organization helps in navigating through the code, understanding its structure, and making changes when necessary.
Best Practices for Code Organization:
- Use meaningful file and folder structures: Organize your code into logical directories and files.
- Keep functions small and focused: Each function should do one thing and do it well.
- Group related code together: Keep related functions and classes in the same file or module.
- Separate concerns: Divide your code into distinct sections that handle different aspects of the program (e.g., UI, business logic, data access).
- Use design patterns: Implement appropriate design patterns to solve common problems in a standardized way.
Example of a Well-Organized Project Structure:
project/
│
├── src/
│ ├── components/
│ │ ├── Header.js
│ │ └── Footer.js
│ ├── pages/
│ │ ├── Home.js
│ │ └── About.js
│ ├── utils/
│ │ └── helpers.js
│ └── App.js
│
├── tests/
│ ├── components/
│ │ ├── Header.test.js
│ │ └── Footer.test.js
│ └── utils/
│ └── helpers.test.js
│
├── public/
│ └── index.html
│
└── package.json
4. Commenting and Documentation
While clean code should be self-explanatory to a large extent, comments and documentation play a crucial role in explaining complex logic, providing context, and helping other developers understand your code quickly.
Guidelines for Commenting:
- Write self-documenting code: Your code should be clear enough that it doesn’t require extensive comments to explain what it does.
- Use comments to explain why, not what: The code itself should show what it does; comments should explain the reasoning behind it.
- Keep comments up-to-date: Outdated comments can be misleading and cause confusion.
- Use documentation generators: Tools like JSDoc or Sphinx can help create standardized documentation from your code comments.
Example of Good Commenting:
/**
* Calculates the factorial of a number.
* @param {number} n - The number to calculate the factorial for.
* @returns {number} The factorial of the input number.
* @throws {Error} If the input is negative or not an integer.
*/
function factorial(n) {
// Validate input
if (n < 0 || !Number.isInteger(n)) {
throw new Error('Input must be a non-negative integer');
}
// Base case: factorial of 0 or 1 is 1
if (n <= 1) return 1;
// Recursive case: n! = n * (n-1)!
return n * factorial(n - 1);
}
5. Error Handling
Proper error handling is a crucial aspect of writing clean and robust code. It helps in identifying and resolving issues quickly, improving the overall reliability of your software.
Best Practices for Error Handling:
- Use try-catch blocks: Wrap code that may throw exceptions in try-catch blocks to handle errors gracefully.
- Throw specific exceptions: Create and throw custom exceptions that provide meaningful information about the error.
- Log errors: Use a logging system to record errors for later analysis and debugging.
- Fail fast: Detect and report errors as early as possible in the execution flow.
- Provide meaningful error messages: Error messages should be clear and actionable, helping users or developers understand and resolve the issue.
Example of Good Error Handling:
class InsufficientFundsError extends Error {
constructor(message) {
super(message);
this.name = 'InsufficientFundsError';
}
}
function withdrawMoney(account, amount) {
try {
if (amount <= 0) {
throw new Error('Withdrawal amount must be positive');
}
if (amount > account.balance) {
throw new InsufficientFundsError('Insufficient funds for withdrawal');
}
account.balance -= amount;
return amount;
} catch (error) {
console.error(`Error during withdrawal: ${error.message}`);
// Re-throw the error for the caller to handle
throw error;
}
}
6. DRY Principle
DRY stands for “Don’t Repeat Yourself.” This principle emphasizes the importance of avoiding code duplication by abstracting common functionality into reusable components or functions.
Benefits of Following DRY:
- Reduced code redundancy
- Easier maintenance and updates
- Improved code consistency
- Reduced chances of errors when making changes
Example of Applying DRY:
// Before applying DRY
function calculateCircleArea(radius) {
return 3.14 * radius * radius;
}
function calculateCircleCircumference(radius) {
return 2 * 3.14 * radius;
}
// After applying DRY
const PI = 3.14;
function calculateCircleArea(radius) {
return PI * radius * radius;
}
function calculateCircleCircumference(radius) {
return 2 * PI * radius;
}
7. SOLID Principles
SOLID is an acronym for five principles of object-oriented programming and design that help create more maintainable, flexible, and scalable software.
The SOLID Principles:
- Single Responsibility Principle (SRP): A class should have only one reason to change.
- Open-Closed Principle (OCP): Software entities should be open for extension but closed for modification.
- Liskov Substitution Principle (LSP): Objects of a superclass should be replaceable with objects of its subclasses without affecting the correctness of the program.
- Interface Segregation Principle (ISP): Many client-specific interfaces are better than one general-purpose interface.
- Dependency Inversion Principle (DIP): High-level modules should not depend on low-level modules. Both should depend on abstractions.
Example of Applying SOLID Principles:
// Single Responsibility Principle
class User {
constructor(name) {
this.name = name;
}
}
class UserRepository {
save(user) {
// Logic to save user to database
}
}
// Open-Closed Principle
class Shape {
area() {
throw new Error('area() method must be implemented');
}
}
class Rectangle extends Shape {
constructor(width, height) {
super();
this.width = width;
this.height = height;
}
area() {
return this.width * this.height;
}
}
class Circle extends Shape {
constructor(radius) {
super();
this.radius = radius;
}
area() {
return Math.PI * this.radius * this.radius;
}
}
8. Code Formatting
Consistent code formatting is essential for readability and maintainability. It helps developers quickly understand the structure and flow of the code.
Best Practices for Code Formatting:
- Use consistent indentation: Stick to either spaces or tabs for indentation throughout your project.
- Limit line length: Keep lines of code reasonably short (e.g., 80-120 characters) to improve readability.
- Use whitespace effectively: Add blank lines to separate logical sections of code and use spaces around operators and after commas.
- Align related code: Vertically align related pieces of code when it improves readability.
- Be consistent with braces: Choose a brace style (e.g., K&R, Allman) and stick to it throughout your codebase.
Example of Good Code Formatting:
function calculateTotalPrice(items, taxRate) {
const subtotal = items.reduce((sum, item) => {
return sum + item.price * item.quantity;
}, 0);
const taxAmount = subtotal * taxRate;
const totalPrice = subtotal + taxAmount;
return {
subtotal: subtotal.toFixed(2),
tax: taxAmount.toFixed(2),
total: totalPrice.toFixed(2)
};
}
const cart = [
{ name: 'Widget', price: 9.99, quantity: 3 },
{ name: 'Gadget', price: 14.99, quantity: 2 },
{ name: 'Doohickey', price: 4.99, quantity: 5 }
];
const result = calculateTotalPrice(cart, 0.08);
console.log(result);
9. Testing
Writing clean code goes hand in hand with writing good tests. Tests help ensure that your code works as expected and make it easier to refactor and maintain your codebase over time.
Best Practices for Testing:
- Write unit tests: Test individual components or functions in isolation.
- Use test-driven development (TDD): Write tests before implementing the actual code.
- Aim for high code coverage: Try to cover as much of your code with tests as possible.
- Write meaningful test cases: Each test should have a clear purpose and test a specific behavior.
- Keep tests clean and maintainable: Apply the same clean code principles to your test code.
Example of a Unit Test:
// Function to be tested
function add(a, b) {
return a + b;
}
// Test suite
describe('add function', () => {
test('adds two positive numbers correctly', () => {
expect(add(2, 3)).toBe(5);
});
test('adds a positive and a negative number correctly', () => {
expect(add(5, -3)).toBe(2);
});
test('adds two negative numbers correctly', () => {
expect(add(-2, -4)).toBe(-6);
});
test('returns 0 when adding 0 to 0', () => {
expect(add(0, 0)).toBe(0);
});
});
10. Version Control
Using version control systems like Git is an essential practice for writing and maintaining clean code. It allows you to track changes, collaborate with others, and manage different versions of your codebase effectively.
Best Practices for Version Control:
- Commit frequently: Make small, focused commits that represent logical units of change.
- Write meaningful commit messages: Clearly describe the changes made in each commit.
- Use branches: Create separate branches for different features or bug fixes.
- Review code before merging: Use pull requests or code reviews to ensure code quality.
- Keep the main branch stable: Ensure that the main branch always contains working, production-ready code.
Example of a Good Commit Message:
Fix: Resolve null pointer exception in user authentication
- Add null check before accessing user object
- Update error handling to provide more informative messages
- Add unit tests to cover edge cases
Closes #123
11. Continuous Improvement
Writing clean code is an ongoing process that requires continuous learning and improvement. As you gain experience and encounter new challenges, you’ll discover new ways to write cleaner, more efficient code.
Tips for Continuous Improvement:
- Read books and articles: Stay updated with the latest best practices and coding techniques.
- Participate in code reviews: Both giving and receiving feedback can help you improve your coding skills.
- Refactor regularly: Set aside time to improve existing code without changing its external behavior.
- Learn from open-source projects: Study well-maintained open-source codebases to learn best practices.
- Attend workshops and conferences: Engage with the developer community to learn new approaches and share experiences.
12. Tools for Clean Code
Various tools can help you maintain clean code and enforce best practices across your projects. Here are some popular tools that can assist you in writing cleaner code:
Linters and Code Formatters:
- ESLint: A highly configurable JavaScript linter that helps identify and fix problems in your code.
- Prettier: An opinionated code formatter that supports multiple languages and integrates with most editors.
- Black: An uncompromising code formatter for Python that adheres to PEP 8 guidelines.
Static Code Analysis Tools:
- SonarQube: An open-source platform for continuous inspection of code quality.
- CodeClimate: A tool that provides automated code review for test coverage, complexity, and duplication.
Documentation Generators:
- JSDoc: A documentation generator for JavaScript.
- Sphinx: A tool that makes it easy to create intelligent and beautiful documentation for Python projects.
Version Control Systems:
- Git: A distributed version control system for tracking changes in source code during software development.
- GitHub/GitLab: Platforms that provide hosting for software development and version control using Git.
Integrated Development Environments (IDEs):
- Visual Studio Code: A free, open-source code editor with built-in support for many languages and a wide range of extensions.
- IntelliJ IDEA: A powerful IDE for Java development that also supports many other languages.
Conclusion
Writing clean code is a skill that takes time and practice to master. By following the best practices outlined in this guide, you can significantly improve the quality, readability, and maintainability of your code. Remember that clean code is not just about following rules; it’s about creating software that is easy to understand, modify, and maintain over time.
As you continue your journey in software development, keep these principles in mind and strive to apply them in your daily coding practices. Clean code is not just beneficial for you as a developer; it’s a courtesy to your future self and your colleagues who will work with your code.
By consistently applying these best practices, you’ll not only become a better programmer but also contribute to creating more robust, efficient, and maintainable software systems. As Robert C. Martin wisely said, “Clean code always looks like it was written by someone who cares.” Be that someone who cares about the craft of programming, and your code will reflect that dedication.