How to Use Docker for Containerization in Development: A Comprehensive Guide
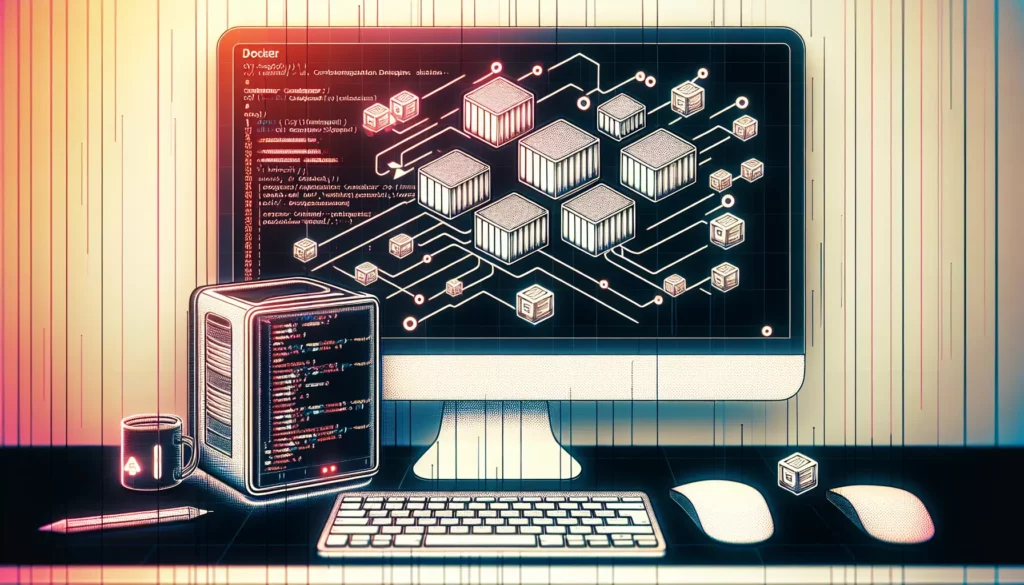
In the ever-evolving landscape of software development, containerization has emerged as a game-changing technology. At the forefront of this revolution is Docker, a powerful tool that has transformed the way developers build, ship, and run applications. This comprehensive guide will walk you through the process of using Docker for containerization in your development workflow, helping you streamline your projects and boost productivity.
Table of Contents
- Introduction to Docker and Containerization
- Benefits of Using Docker in Development
- Installing Docker
- Docker Basics: Images and Containers
- Creating a Dockerfile
- Building and Running Docker Images
- Using Docker Compose for Multi-Container Applications
- Best Practices for Docker in Development
- Debugging Docker Containers
- Integrating Docker with CI/CD Pipelines
- Advanced Docker Techniques
- Conclusion
1. Introduction to Docker and Containerization
Docker is an open-source platform that automates the deployment, scaling, and management of applications using containerization technology. Containers are lightweight, standalone, and executable packages that include everything needed to run a piece of software, including the code, runtime, system tools, libraries, and settings.
Containerization offers several advantages over traditional virtualization:
- Lightweight: Containers share the host system’s OS kernel, making them much smaller and faster to start than virtual machines.
- Portable: Containers can run consistently across different environments, from a developer’s laptop to production servers.
- Scalable: Containers can be easily scaled up or down to meet demand.
- Isolated: Each container runs in its own isolated environment, ensuring that applications don’t interfere with each other.
2. Benefits of Using Docker in Development
Incorporating Docker into your development workflow offers numerous benefits:
- Consistency: Docker ensures that your application runs the same way across different development, testing, and production environments.
- Faster onboarding: New team members can quickly set up their development environment using Docker, reducing setup time and potential configuration issues.
- Isolation: Docker containers isolate dependencies and configurations, preventing conflicts between different projects or versions.
- Version control: Docker images can be versioned, allowing you to roll back to previous versions easily.
- Microservices architecture: Docker facilitates the development and deployment of microservices-based applications.
- Resource efficiency: Containers use fewer resources than traditional virtual machines, allowing developers to run multiple containers on a single machine.
3. Installing Docker
Before you can start using Docker, you need to install it on your system. The installation process varies depending on your operating system:
For Windows:
- Download Docker Desktop for Windows from the official Docker website.
- Run the installer and follow the prompts.
- Once installed, launch Docker Desktop.
For macOS:
- Download Docker Desktop for Mac from the official Docker website.
- Double-click the .dmg file and drag the Docker icon to your Applications folder.
- Launch Docker from your Applications folder.
For Linux:
The installation process for Linux varies depending on the distribution. Here’s a general outline for Ubuntu:
$ sudo apt-get update
$ sudo apt-get install docker-ce docker-ce-cli containerd.io
$ sudo systemctl start docker
$ sudo systemctl enable docker
After installation, verify that Docker is correctly installed by running:
$ docker --version
$ docker run hello-world
4. Docker Basics: Images and Containers
To effectively use Docker, it’s crucial to understand two fundamental concepts: images and containers.
Docker Images
A Docker image is a lightweight, standalone, and executable package that includes everything needed to run a piece of software. It contains the application code, runtime, system tools, libraries, and settings. Images are built from a set of instructions called a Dockerfile.
Docker Containers
A Docker container is a running instance of a Docker image. You can think of it as a lightweight, sandboxed process on your machine that is isolated from all other processes. Containers are created from images and can be started, stopped, moved, and deleted.
Key Commands
Here are some essential Docker commands to get you started:
docker pull <image_name>
: Downloads an image from Docker Hubdocker images
: Lists all locally available imagesdocker run <image_name>
: Creates and starts a container from an imagedocker ps
: Lists running containersdocker stop <container_id>
: Stops a running containerdocker rm <container_id>
: Removes a stopped container
5. Creating a Dockerfile
A Dockerfile is a text file that contains a set of instructions for building a Docker image. It specifies the base image, environment variables, dependencies, and commands needed to set up your application.
Here’s an example of a simple Dockerfile for a Python application:
# Use an official Python runtime as the base image
FROM python:3.9-slim
# Set the working directory in the container
WORKDIR /app
# Copy the current directory contents into the container at /app
COPY . /app
# Install any needed packages specified in requirements.txt
RUN pip install --no-cache-dir -r requirements.txt
# Make port 80 available to the world outside this container
EXPOSE 80
# Define environment variable
ENV NAME World
# Run app.py when the container launches
CMD ["python", "app.py"]
Let’s break down the Dockerfile:
FROM
: Specifies the base image to useWORKDIR
: Sets the working directory for subsequent instructionsCOPY
: Copies files from the host to the containerRUN
: Executes commands in the containerEXPOSE
: Informs Docker that the container listens on specified network ports at runtimeENV
: Sets environment variablesCMD
: Provides defaults for executing a container
6. Building and Running Docker Images
Once you have created a Dockerfile, you can build an image and run a container based on that image.
Building an Image
To build an image from a Dockerfile, use the docker build
command:
$ docker build -t myapp:v1 .
This command builds an image named “myapp” with the tag “v1” using the Dockerfile in the current directory (indicated by the dot at the end).
Running a Container
To run a container based on your image, use the docker run
command:
$ docker run -p 4000:80 myapp:v1
This command runs a container based on the “myapp:v1” image and maps port 4000 on the host to port 80 in the container.
7. Using Docker Compose for Multi-Container Applications
Docker Compose is a tool for defining and running multi-container Docker applications. It uses a YAML file to configure your application’s services, networks, and volumes, allowing you to run multi-container applications with a single command.
Here’s an example docker-compose.yml
file for a web application with a database:
version: '3'
services:
web:
build: .
ports:
- "5000:5000"
depends_on:
- db
db:
image: postgres
environment:
POSTGRES_PASSWORD: example
To run your multi-container application, use:
$ docker-compose up
This command will build the images (if needed) and start the containers as defined in the docker-compose.yml
file.
8. Best Practices for Docker in Development
To make the most of Docker in your development workflow, consider these best practices:
- Use official base images: Start with official images from Docker Hub to ensure security and reliability.
- Minimize layers: Combine commands in your Dockerfile to reduce the number of layers and image size.
- Use .dockerignore: Create a .dockerignore file to exclude unnecessary files from your build context.
- Don’t run containers as root: Use the USER instruction to switch to a non-root user for better security.
- Use multi-stage builds: Employ multi-stage builds to create smaller production images.
- Tag your images: Use meaningful tags for your images to track versions and changes.
- Use volumes for persistent data: Mount volumes to store data that needs to persist between container restarts.
9. Debugging Docker Containers
Debugging Docker containers can be challenging, but several techniques can help:
- Use
docker logs <container_id>
to view container logs. - Execute commands inside a running container with
docker exec -it <container_id> /bin/bash
. - Use Docker’s built-in health checks to monitor container status.
- Employ Docker Compose’s
docker-compose logs
command for multi-container applications. - Utilize Docker’s debug mode with
docker --debug
for more verbose output.
10. Integrating Docker with CI/CD Pipelines
Docker can be seamlessly integrated into your Continuous Integration and Continuous Deployment (CI/CD) pipelines:
- Build Docker images as part of your CI process.
- Use Docker registries to store and version your images.
- Implement automated testing within Docker containers.
- Deploy applications using Docker in your CD pipeline.
- Utilize Docker Swarm or Kubernetes for container orchestration in production.
11. Advanced Docker Techniques
As you become more comfortable with Docker, explore these advanced techniques:
- Multi-stage builds: Create smaller production images by using multiple stages in your Dockerfile.
- Custom networks: Create isolated networks for your containers to enhance security and control communication between services.
- Docker secrets: Manage sensitive information securely using Docker’s built-in secrets management.
- Resource constraints: Limit CPU and memory usage for containers to optimize resource allocation.
- Docker plugins: Extend Docker’s functionality with plugins for networking, volume management, and more.
12. Conclusion
Docker has revolutionized the way developers build, ship, and run applications. By embracing containerization in your development workflow, you can achieve greater consistency, portability, and efficiency across your projects. From setting up your development environment to deploying applications in production, Docker provides a powerful set of tools to streamline your software development lifecycle.
As you continue to explore Docker, remember that the technology is constantly evolving. Stay updated with the latest Docker features and best practices to make the most of this powerful containerization platform. With Docker, you’re well-equipped to tackle the challenges of modern software development and deliver high-quality applications with confidence.
Happy containerizing!