Microservices in Software Architecture: A Comprehensive Guide
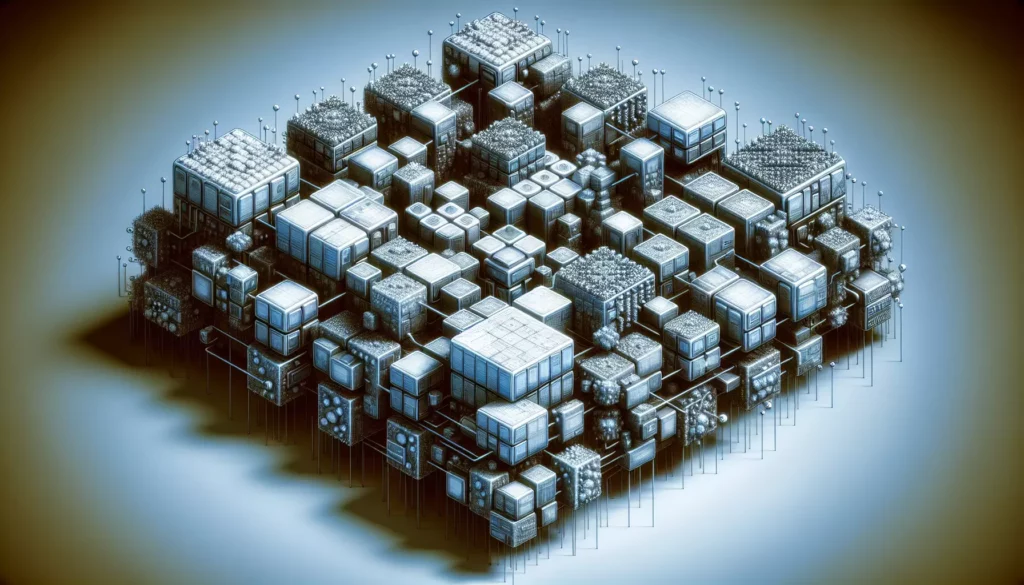
In the ever-evolving landscape of software development, microservices architecture has emerged as a powerful approach to building scalable, flexible, and maintainable applications. As we delve into this topic, it’s important to understand how microservices fit into the broader context of modern software engineering and its relevance to aspiring developers, especially those aiming to work at major tech companies.
Understanding Microservices
Microservices architecture is a method of developing software applications as a suite of small, independently deployable services. Each service runs in its own process and communicates with other services through well-defined APIs. This approach stands in contrast to the traditional monolithic architecture, where an entire application is built as a single, tightly-coupled unit.
Key Characteristics of Microservices
- Modularity: Each microservice focuses on a specific business capability.
- Independence: Services can be developed, deployed, and scaled independently.
- Decentralization: Microservices promote decentralized data management and governance.
- Flexibility: Different services can use different technologies and programming languages.
- Resilience: Failure in one service doesn’t necessarily affect the entire system.
The Evolution from Monolithic to Microservices Architecture
To appreciate the significance of microservices, it’s crucial to understand its predecessor: the monolithic architecture. In a monolithic application, all components are interconnected and interdependent, running as a single unit. While this approach has its merits, it also presents several challenges as applications grow in size and complexity:
- Difficulty in scaling specific components
- Increased risk of system-wide failures
- Challenges in adopting new technologies
- Slower development and deployment cycles
Microservices address these issues by breaking down the application into smaller, manageable services. This shift allows for greater agility, easier maintenance, and improved scalability.
Core Principles of Microservices Architecture
1. Single Responsibility Principle
Each microservice should be responsible for a single, well-defined functionality. This aligns with the software engineering principle of “Do One Thing and Do It Well.” For example, in an e-commerce application:
- One service might handle user authentication
- Another could manage product inventory
- A separate service could process payments
2. Decentralized Data Management
In a microservices architecture, each service typically manages its own database. This decentralization allows services to choose the most appropriate data storage solution for their needs. It also prevents data coupling between services, enhancing independence and scalability.
3. Design for Failure
Microservices should be designed with the expectation that failures will occur. This principle encourages developers to build resilient systems that can gracefully handle the failure of individual services. Techniques like circuit breakers, retries, and fallbacks are commonly employed to achieve this resilience.
4. Evolutionary Design
Microservices architecture supports evolutionary design, allowing systems to adapt and change over time. This flexibility is crucial in today’s fast-paced technological landscape, where requirements and technologies are constantly evolving.
Implementing Microservices: Key Considerations
Service Discovery
In a microservices environment, services need to locate and communicate with each other dynamically. Service discovery mechanisms help manage this complexity. Popular tools for service discovery include:
- Netflix Eureka
- Consul
- Etcd
API Gateway
An API Gateway acts as a single entry point for all client requests. It routes requests to the appropriate microservices, handles authentication, and can perform tasks like load balancing and caching. Examples of API Gateway solutions include:
- Netflix Zuul
- Kong
- Amazon API Gateway
Inter-service Communication
Microservices need to communicate with each other efficiently. Two primary patterns for inter-service communication are:
- Synchronous Communication: Using REST or gRPC for real-time, request-response interactions.
- Asynchronous Communication: Using message queues or event streaming platforms for decoupled, event-driven interactions.
Here’s a simple example of how two microservices might communicate using REST:
// Service A: Order Service
@RestController
public class OrderController {
@GetMapping("/order/{orderId}")
public Order getOrder(@PathVariable String orderId) {
// Retrieve order details
return orderRepository.findById(orderId);
}
}
// Service B: Payment Service
@Service
public class PaymentService {
@Autowired
private RestTemplate restTemplate;
public void processPayment(String orderId) {
String orderServiceUrl = "http://order-service/order/" + orderId;
Order order = restTemplate.getForObject(orderServiceUrl, Order.class);
// Process payment based on order details
}
}
Data Consistency
Maintaining data consistency across multiple services can be challenging. Techniques to address this include:
- Saga Pattern: Coordinating a series of local transactions to maintain consistency.
- Event Sourcing: Storing the state of an application as a sequence of events.
- CQRS (Command Query Responsibility Segregation): Separating read and write operations for better scalability and performance.
Benefits of Microservices Architecture
1. Scalability
Microservices allow for independent scaling of services. This means you can allocate more resources to high-demand services without scaling the entire application, leading to more efficient resource utilization and cost savings.
2. Technology Diversity
With microservices, teams have the flexibility to choose the best technology stack for each service. This allows for adopting new technologies and frameworks without overhauling the entire system.
3. Faster Time-to-Market
The modular nature of microservices enables parallel development and deployment. Teams can work on different services simultaneously, accelerating the development process and reducing time-to-market for new features.
4. Improved Fault Isolation
In a microservices architecture, failures are typically contained within individual services. This isolation prevents a single point of failure from bringing down the entire system, enhancing overall reliability.
5. Easier Maintenance and Updates
Microservices can be updated independently, allowing for more frequent and less risky deployments. This aligns well with continuous integration and continuous deployment (CI/CD) practices.
Challenges in Implementing Microservices
While microservices offer numerous benefits, they also come with their own set of challenges:
1. Increased Complexity
Managing a distributed system of microservices can be more complex than handling a monolithic application. This complexity extends to deployment, monitoring, and troubleshooting.
2. Network Latency
Inter-service communication over a network can introduce latency, potentially impacting overall system performance. Careful design and optimization are necessary to mitigate this issue.
3. Data Consistency
Maintaining data consistency across multiple services and databases can be challenging, especially in distributed transactions.
4. Testing Complexity
Testing microservices-based applications can be more complex due to service dependencies and distributed nature. Comprehensive integration testing becomes crucial.
5. Operational Overhead
Microservices require robust DevOps practices and tools for effective deployment, monitoring, and management. This can lead to increased operational overhead compared to monolithic systems.
Best Practices for Microservices Development
1. Design Around Business Capabilities
Structure your microservices around business domains rather than technical functions. This approach, known as Domain-Driven Design (DDD), helps create more meaningful and cohesive service boundaries.
2. Implement Proper Monitoring and Logging
Robust monitoring and centralized logging are crucial in a microservices environment. Tools like Prometheus, Grafana, and ELK stack (Elasticsearch, Logstash, Kibana) can be invaluable for maintaining visibility across services.
3. Use Containerization
Containerization technologies like Docker simplify the packaging and deployment of microservices. They ensure consistency across different environments and facilitate easier scaling and management.
4. Implement Circuit Breakers
Circuit breakers help prevent cascading failures in a microservices ecosystem. Libraries like Netflix Hystrix provide implementations of the circuit breaker pattern.
5. Automate Deployment with CI/CD
Implement robust CI/CD pipelines to automate the testing and deployment of microservices. This practice ensures faster and more reliable releases.
Microservices in Practice: Real-World Examples
Several major tech companies have successfully implemented microservices architecture:
Netflix
Netflix is often cited as a pioneer in microservices adoption. They transitioned from a monolithic DVD rental application to a cloud-based streaming service built on microservices. This architecture allows Netflix to handle millions of concurrent streams and rapidly deploy new features.
Amazon
Amazon’s e-commerce platform is built on a microservices architecture. This approach has enabled Amazon to scale its operations enormously and introduce new services like AWS with relative ease.
Uber
Uber’s ride-sharing platform utilizes microservices to manage various aspects of its service, from matching riders with drivers to handling payments and ratings.
The Future of Microservices
As microservices continue to evolve, several trends are shaping their future:
1. Serverless Architecture
Serverless computing is becoming increasingly popular for implementing microservices. Platforms like AWS Lambda and Azure Functions allow developers to focus on code without worrying about infrastructure management.
2. Service Mesh
Service mesh technologies like Istio and Linkerd are gaining traction. They provide a dedicated infrastructure layer for handling service-to-service communication, offering features like traffic management, security, and observability.
3. AI and Machine Learning Integration
As AI and ML become more prevalent, microservices architectures are being adapted to support these technologies more effectively, allowing for more intelligent and adaptive systems.
4. Edge Computing
The rise of edge computing is influencing microservices design, with a focus on deploying services closer to the end-user for improved performance and reduced latency.
Conclusion
Microservices architecture represents a significant shift in how we design, develop, and deploy software applications. Its principles of modularity, independence, and scalability align well with the demands of modern software development, especially in the context of large-scale applications developed by major tech companies.
For aspiring developers and those preparing for technical interviews, understanding microservices is increasingly important. It’s not just about knowing the theory; practical experience in designing and implementing microservices can be a valuable asset in your career, particularly when aiming for positions at companies like Netflix, Amazon, or Google.
As you continue your journey in software development, consider exploring microservices further. Experiment with building small applications using a microservices approach, familiarize yourself with containerization technologies like Docker, and practice solving system design problems that involve microservices architectures. This hands-on experience will not only deepen your understanding but also prepare you for the challenges and opportunities in modern software engineering.
Remember, while microservices offer numerous benefits, they’re not a one-size-fits-all solution. The decision to adopt microservices should be based on careful consideration of your project’s specific requirements, team capabilities, and organizational goals. As with any architectural pattern, the key is to understand its strengths and limitations and apply it judiciously to create robust, scalable, and maintainable software systems.