Coding vs Programming: Understanding the Key Differences
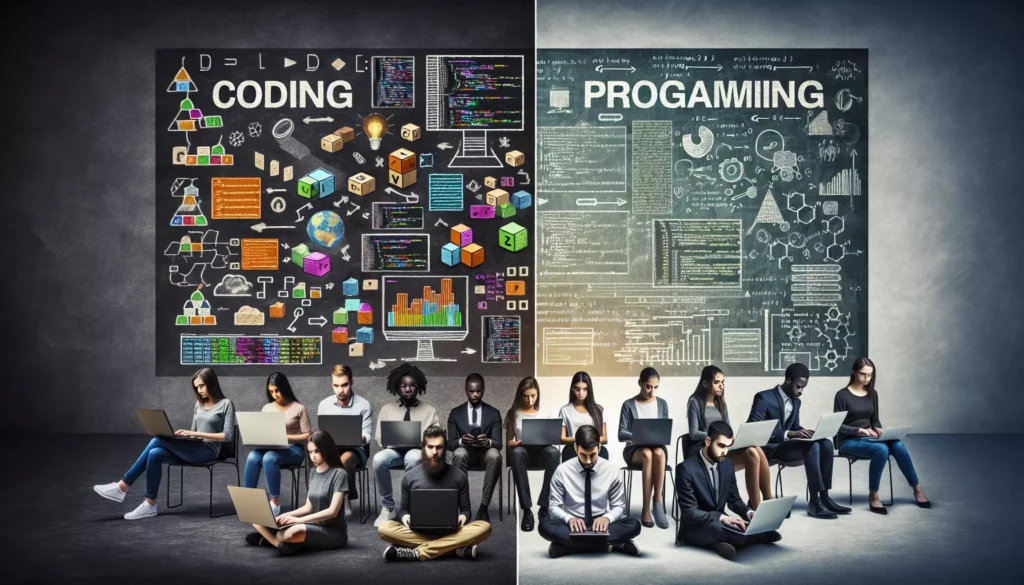
In the ever-evolving world of technology, two terms that are often used interchangeably are “coding” and “programming.” While they are closely related and share many similarities, there are subtle yet important differences between the two. This comprehensive guide will delve into the nuances that set coding and programming apart, exploring their definitions, scope, and applications in the context of modern software development.
Defining Coding and Programming
Before we dive into the differences, let’s establish clear definitions for both coding and programming:
What is Coding?
Coding refers to the act of writing code in a specific programming language. It involves translating human-readable instructions into a language that computers can understand and execute. Coding is often considered a subset of programming and focuses on the actual implementation of algorithms and logic using a particular syntax.
What is Programming?
Programming, on the other hand, encompasses a broader set of activities involved in creating software applications. It includes not only writing code but also designing algorithms, planning software architecture, debugging, testing, and maintaining the codebase. Programming is about solving complex problems through computational thinking and creating efficient, scalable solutions.
Key Differences Between Coding and Programming
Now that we have established the basic definitions, let’s explore the key differences between coding and programming:
1. Scope and Complexity
- Coding: Generally involves working on smaller, more specific tasks or components of a larger project. It’s about implementing predefined logic or algorithms.
- Programming: Encompasses the entire software development lifecycle, from conceptualization to maintenance. It involves higher-level thinking and problem-solving skills.
2. Skills Required
- Coding: Primarily requires knowledge of programming languages, syntax, and basic problem-solving skills.
- Programming: Demands a broader skill set, including algorithm design, data structures, software architecture, and system design, in addition to coding skills.
3. Tools and Resources
- Coding: Typically involves using code editors, integrated development environments (IDEs), and version control systems.
- Programming: Utilizes a wider range of tools, including project management software, modeling tools, debugging tools, and testing frameworks.
4. Output and Deliverables
- Coding: Produces specific pieces of code or scripts that perform particular functions.
- Programming: Results in complete software applications, systems, or solutions that address complex problems or user needs.
5. Problem-Solving Approach
- Coding: Focuses on implementing solutions to well-defined problems or tasks.
- Programming: Involves analyzing complex problems, breaking them down into smaller components, and designing comprehensive solutions.
6. Time and Resource Management
- Coding: Often deals with shorter-term tasks and immediate implementation of features or fixes.
- Programming: Requires long-term planning, resource allocation, and consideration of scalability and maintainability.
The Relationship Between Coding and Programming
While we’ve highlighted the differences between coding and programming, it’s important to understand that they are not mutually exclusive concepts. In fact, coding is an integral part of programming, and the two are deeply interconnected in the software development process.
Coding as a Foundation for Programming
Coding serves as the foundation upon which programming skills are built. Learning to code is often the first step for aspiring programmers, as it introduces them to the fundamental concepts of working with computers and writing instructions in a structured manner. As individuals progress in their journey, they naturally expand their skills beyond just coding to encompass the broader aspects of programming.
The Progression from Coder to Programmer
Many professionals in the tech industry start their careers as coders and gradually evolve into programmers. This progression involves:
- Mastering multiple programming languages and paradigms
- Developing a deeper understanding of software architecture and design patterns
- Gaining experience in large-scale project management and collaboration
- Enhancing problem-solving and analytical skills
- Learning to consider factors beyond just code, such as user experience, performance, and scalability
The Importance of Both Coding and Programming Skills
In today’s technology-driven world, both coding and programming skills are highly valuable. Let’s explore why each set of skills is crucial in different contexts:
The Value of Coding Skills
- Quick Problem-Solving: Coding skills allow for rapid prototyping and implementation of solutions to immediate problems.
- Specialization: Expert coders can become specialists in specific languages or frameworks, making them valuable assets in niche areas.
- Automation: Coding skills enable the creation of scripts and tools that automate repetitive tasks, increasing efficiency.
- Entry-Level Opportunities: Strong coding skills can open doors to entry-level positions in software development.
The Importance of Programming Skills
- Holistic Problem-Solving: Programming skills allow for tackling complex, multi-faceted problems that require comprehensive solutions.
- Project Leadership: Programmers with a broader skill set are well-equipped to lead development teams and manage large-scale projects.
- Innovation: The ability to think beyond code enables programmers to innovate and create novel solutions to challenging problems.
- Career Advancement: As professionals progress in their careers, programming skills become increasingly important for senior roles and leadership positions.
Coding and Programming in the Context of AlgoCademy
At AlgoCademy, we recognize the importance of both coding and programming skills in preparing individuals for successful careers in the tech industry. Our platform is designed to support learners at various stages of their journey, from beginner coders to aspiring software engineers preparing for technical interviews at top tech companies.
How AlgoCademy Addresses Coding Skills
- Interactive Coding Tutorials: Our platform offers hands-on coding exercises that help learners master the syntax and fundamentals of various programming languages.
- AI-Powered Assistance: We provide real-time feedback and suggestions to help users improve their coding skills and overcome common challenges.
- Language-Specific Resources: AlgoCademy offers dedicated resources for popular programming languages, ensuring learners can specialize in their areas of interest.
How AlgoCademy Fosters Programming Skills
- Algorithmic Thinking: Our curriculum emphasizes problem-solving and algorithmic thinking, encouraging users to approach challenges from a programmer’s perspective.
- Project-Based Learning: We offer larger-scale projects that simulate real-world scenarios, helping users develop comprehensive programming skills.
- Interview Preparation: Our platform includes resources specifically designed to prepare users for technical interviews at major tech companies, covering both coding challenges and broader programming concepts.
Bridging the Gap: From Coding to Programming
For those looking to transition from coding to programming, or to enhance their skills in both areas, consider the following strategies:
1. Expand Your Knowledge Base
Go beyond mastering a single programming language. Learn about different programming paradigms, design patterns, and architectural styles. Resources like books, online courses, and tech conferences can be invaluable in this journey.
2. Work on Personal Projects
Undertake personal projects that challenge you to think beyond coding. Design and implement a complete application from scratch, considering aspects like user interface, database design, and scalability.
3. Collaborate with Others
Participate in open-source projects or join coding communities. Collaboration exposes you to different perspectives and approaches to problem-solving, which is crucial for developing programming skills.
4. Study System Design
Familiarize yourself with system design concepts. This involves understanding how to architect large-scale systems, which is a key skill for programmers. Platforms like AlgoCademy offer resources specifically tailored to system design preparation.
5. Practice Problem-Solving
Regularly engage in coding challenges and algorithmic problem-solving exercises. Websites like LeetCode, HackerRank, and AlgoCademy provide a wealth of problems to tackle, helping you develop both coding and programming skills.
6. Learn About Software Development Methodologies
Familiarize yourself with different software development methodologies like Agile, Scrum, or Waterfall. Understanding these approaches will give you insight into the broader context of programming in professional environments.
Code Example: Illustrating the Difference
To further illustrate the difference between coding and programming, let’s consider a simple example. Imagine we need to create a program that calculates the factorial of a number.
Coding Approach
A coder might focus on implementing the factorial function directly:
def factorial(n):
if n == 0 or n == 1:
return 1
else:
return n * factorial(n - 1)
# Test the function
print(factorial(5)) # Output: 120
This code correctly implements the factorial function and solves the immediate problem.
Programming Approach
A programmer might consider a broader context, thinking about potential use cases, error handling, and user interaction:
def factorial(n):
if not isinstance(n, int):
raise TypeError("Input must be an integer")
if n < 0:
raise ValueError("Input must be non-negative")
if n == 0 or n == 1:
return 1
else:
return n * factorial(n - 1)
def get_user_input():
while True:
try:
n = int(input("Enter a non-negative integer: "))
if n < 0:
print("Please enter a non-negative integer.")
else:
return n
except ValueError:
print("Invalid input. Please enter an integer.")
def main():
try:
n = get_user_input()
result = factorial(n)
print(f"The factorial of {n} is {result}")
except (TypeError, ValueError) as e:
print(f"Error: {e}")
if __name__ == "__main__":
main()
This programming approach considers:
- Input validation and error handling
- User interaction and experience
- Modular design with separate functions for different responsibilities
- Potential for reuse and integration into larger systems
Conclusion: Embracing Both Coding and Programming
In conclusion, while coding and programming are distinct concepts, they are both essential components of software development. Coding forms the foundation of programming, providing the necessary skills to implement solutions, while programming encompasses a broader set of skills that enable the creation of complex, scalable software systems.
As you progress in your journey as a software developer, it’s important to cultivate both coding and programming skills. Platforms like AlgoCademy offer valuable resources to help you develop these skills, from mastering coding fundamentals to preparing for technical interviews at top tech companies.
Remember that the journey from coder to programmer is a continuous one, filled with learning opportunities and challenges. Embrace both aspects of software development, and you’ll be well-equipped to tackle the diverse and exciting challenges in the world of technology.
Whether you’re just starting out or looking to advance your career, understanding the nuances between coding and programming will help you set the right goals and focus your learning efforts effectively. Keep coding, keep learning, and never stop exploring the vast and fascinating world of software development!