How to Build RESTful APIs with Express.js: A Comprehensive Guide
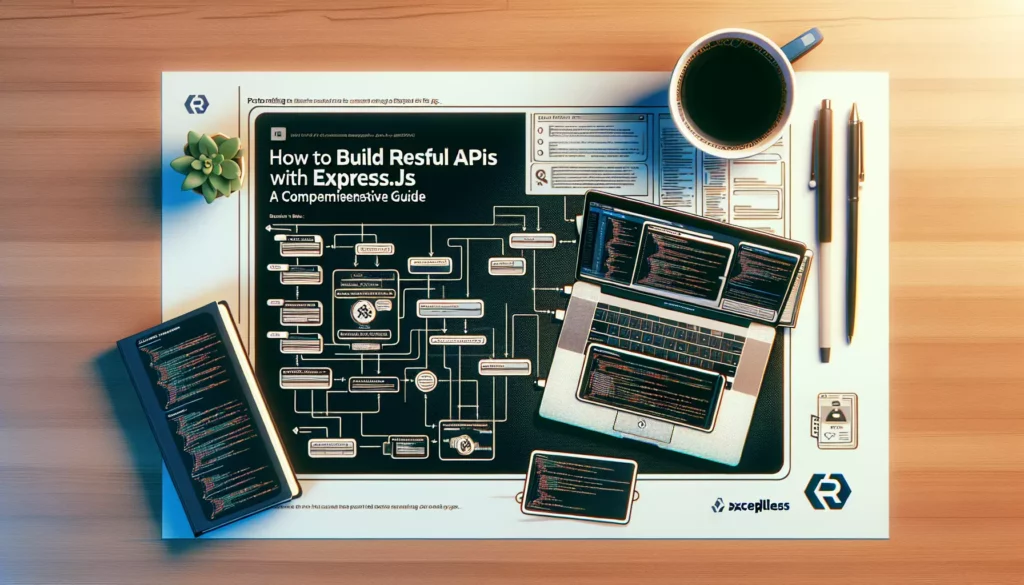
In today’s interconnected digital world, APIs (Application Programming Interfaces) play a crucial role in allowing different software systems to communicate with each other. Among the various types of APIs, RESTful APIs have become increasingly popular due to their simplicity, scalability, and stateless nature. If you’re looking to build robust and efficient web applications, understanding how to create RESTful APIs is essential. In this comprehensive guide, we’ll explore how to build RESTful APIs using Express.js, a popular web application framework for Node.js.
Table of Contents
- Introduction to RESTful APIs and Express.js
- Setting Up Your Development Environment
- Creating the Basic API Structure
- Implementing CRUD Operations
- Using Middleware in Express.js
- Error Handling and Validation
- Adding Authentication to Your API
- Testing Your RESTful API
- Best Practices and Advanced Concepts
- Conclusion
1. Introduction to RESTful APIs and Express.js
Before we dive into the practical aspects of building RESTful APIs with Express.js, let’s briefly review what RESTful APIs are and why Express.js is an excellent choice for creating them.
What are RESTful APIs?
REST (Representational State Transfer) is an architectural style for designing networked applications. RESTful APIs adhere to a set of constraints and principles that make them scalable, simple, and easy to understand. Some key characteristics of RESTful APIs include:
- Stateless communication between client and server
- Use of standard HTTP methods (GET, POST, PUT, DELETE, etc.)
- Resources identified by URLs
- Representation of resources in various formats (JSON, XML, etc.)
Why Express.js?
Express.js is a minimal and flexible Node.js web application framework that provides a robust set of features for building web and mobile applications. It’s particularly well-suited for creating RESTful APIs because:
- It’s fast and lightweight
- It has a large and active community
- It offers great flexibility and customization options
- It integrates well with various databases and third-party services
- It provides middleware support for extending functionality
2. Setting Up Your Development Environment
Before we start building our RESTful API, let’s set up our development environment. Follow these steps to get started:
Install Node.js and npm
If you haven’t already, download and install Node.js from the official website. This will also install npm (Node Package Manager), which we’ll use to manage our project dependencies.
Create a New Project
Open your terminal or command prompt and create a new directory for your project. Navigate to that directory and initialize a new Node.js project:
mkdir express-rest-api
cd express-rest-api
npm init -y
Install Express.js
Install Express.js and save it as a dependency in your project:
npm install express
Install Additional Dependencies
We’ll also install a few other packages that will be useful for our API development:
npm install body-parser cors morgan
body-parser
: Middleware to parse incoming request bodiescors
: Middleware to enable Cross-Origin Resource Sharingmorgan
: HTTP request logger middleware
3. Creating the Basic API Structure
Now that we have our environment set up, let’s create the basic structure of our RESTful API. We’ll start by creating a simple Express.js server and defining some routes.
Create the Main Server File
Create a new file called server.js
in your project root directory and add the following code:
const express = require('express');
const bodyParser = require('body-parser');
const cors = require('cors');
const morgan = require('morgan');
const app = express();
const PORT = process.env.PORT || 3000;
// Middleware
app.use(bodyParser.json());
app.use(bodyParser.urlencoded({ extended: true }));
app.use(cors());
app.use(morgan('dev'));
// Routes
app.get('/', (req, res) => {
res.json({ message: 'Welcome to our RESTful API!' });
});
// Start the server
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
This code sets up a basic Express.js server with some middleware and a simple root route. To run the server, use the following command:
node server.js
You should see the message “Server is running on port 3000” in your console. You can now access your API at http://localhost:3000
.
Organizing Routes
As your API grows, it’s a good practice to organize your routes into separate files. Let’s create a simple structure for this:
mkdir routes
touch routes/users.js
touch routes/posts.js
Now, let’s add some basic routes to routes/users.js
:
const express = require('express');
const router = express.Router();
// GET all users
router.get('/', (req, res) => {
res.json({ message: 'GET all users' });
});
// GET a single user
router.get('/:id', (req, res) => {
res.json({ message: `GET user with id ${req.params.id}` });
});
// POST a new user
router.post('/', (req, res) => {
res.json({ message: 'POST a new user', data: req.body });
});
// PUT (update) a user
router.put('/:id', (req, res) => {
res.json({ message: `PUT (update) user with id ${req.params.id}`, data: req.body });
});
// DELETE a user
router.delete('/:id', (req, res) => {
res.json({ message: `DELETE user with id ${req.params.id}` });
});
module.exports = router;
Now, update your server.js
file to use these routes:
// ... (previous code)
const usersRouter = require('./routes/users');
// Routes
app.use('/api/users', usersRouter);
// ... (rest of the code)
With this structure in place, you can now access user-related endpoints at /api/users
. For example, GET /api/users/1
will return information about the user with ID 1.
4. Implementing CRUD Operations
CRUD (Create, Read, Update, Delete) operations are the foundation of most RESTful APIs. Let’s implement these operations using a simple in-memory data store for demonstration purposes. In a real-world application, you’d typically use a database to store and retrieve data.
Update your routes/users.js
file with the following code:
const express = require('express');
const router = express.Router();
// In-memory data store
let users = [
{ id: 1, name: 'John Doe', email: 'john@example.com' },
{ id: 2, name: 'Jane Smith', email: 'jane@example.com' }
];
// GET all users
router.get('/', (req, res) => {
res.json(users);
});
// GET a single user
router.get('/:id', (req, res) => {
const user = users.find(u => u.id === parseInt(req.params.id));
if (!user) return res.status(404).json({ message: 'User not found' });
res.json(user);
});
// POST a new user
router.post('/', (req, res) => {
const newUser = {
id: users.length + 1,
name: req.body.name,
email: req.body.email
};
users.push(newUser);
res.status(201).json(newUser);
});
// PUT (update) a user
router.put('/:id', (req, res) => {
const user = users.find(u => u.id === parseInt(req.params.id));
if (!user) return res.status(404).json({ message: 'User not found' });
user.name = req.body.name || user.name;
user.email = req.body.email || user.email;
res.json(user);
});
// DELETE a user
router.delete('/:id', (req, res) => {
const index = users.findIndex(u => u.id === parseInt(req.params.id));
if (index === -1) return res.status(404).json({ message: 'User not found' });
const deletedUser = users.splice(index, 1);
res.json(deletedUser[0]);
});
module.exports = router;
This implementation provides a complete set of CRUD operations for managing users. You can test these endpoints using tools like Postman or curl.
5. Using Middleware in Express.js
Middleware functions in Express.js are functions that have access to the request object (req), the response object (res), and the next middleware function in the application’s request-response cycle, commonly denoted by a variable named next
. Middleware can perform the following tasks:
- Execute any code.
- Make changes to the request and response objects.
- End the request-response cycle.
- Call the next middleware in the stack.
Let’s create a simple middleware function to log requests and demonstrate how to use it in our API.
Create a new file called middleware/logger.js
:
function logger(req, res, next) {
console.log(`${req.method} ${req.url} - ${new Date().toISOString()}`);
next();
}
module.exports = logger;
Now, update your server.js
file to use this middleware:
// ... (previous imports)
const logger = require('./middleware/logger');
// ... (other middleware)
app.use(logger);
// ... (rest of the code)
This middleware will log every request to the console, showing the HTTP method, URL, and timestamp.
Error Handling Middleware
Express.js also allows you to create error-handling middleware. Let’s create a simple error handler:
Add the following code at the end of your server.js
file:
// Error handling middleware
app.use((err, req, res, next) => {
console.error(err.stack);
res.status(500).json({ message: 'Something went wrong!' });
});
This middleware will catch any errors that occur in your application and send a 500 Internal Server Error response to the client.
6. Error Handling and Validation
Proper error handling and input validation are crucial for building robust and secure APIs. Let’s improve our user routes by adding some basic validation and error handling.
First, install the joi
library for input validation:
npm install joi
Now, update your routes/users.js
file with the following code:
const express = require('express');
const Joi = require('joi');
const router = express.Router();
// ... (previous in-memory data store code)
// Validation schema
const userSchema = Joi.object({
name: Joi.string().min(3).required(),
email: Joi.string().email().required()
});
// Validation middleware
function validateUser(req, res, next) {
const { error } = userSchema.validate(req.body);
if (error) return res.status(400).json({ message: error.details[0].message });
next();
}
// GET all users
router.get('/', (req, res) => {
res.json(users);
});
// GET a single user
router.get('/:id', (req, res) => {
const user = users.find(u => u.id === parseInt(req.params.id));
if (!user) return res.status(404).json({ message: 'User not found' });
res.json(user);
});
// POST a new user
router.post('/', validateUser, (req, res) => {
const newUser = {
id: users.length + 1,
name: req.body.name,
email: req.body.email
};
users.push(newUser);
res.status(201).json(newUser);
});
// PUT (update) a user
router.put('/:id', validateUser, (req, res) => {
const user = users.find(u => u.id === parseInt(req.params.id));
if (!user) return res.status(404).json({ message: 'User not found' });
user.name = req.body.name;
user.email = req.body.email;
res.json(user);
});
// DELETE a user
router.delete('/:id', (req, res) => {
const index = users.findIndex(u => u.id === parseInt(req.params.id));
if (index === -1) return res.status(404).json({ message: 'User not found' });
const deletedUser = users.splice(index, 1);
res.json(deletedUser[0]);
});
module.exports = router;
This updated code includes input validation for the POST and PUT routes using Joi. It also includes better error handling for cases where a user is not found.
7. Adding Authentication to Your API
Authentication is a crucial aspect of most APIs. Let’s implement a simple JWT (JSON Web Token) based authentication system for our API.
First, install the required packages:
npm install jsonwebtoken bcryptjs
Now, create a new file called routes/auth.js
and add the following code:
const express = require('express');
const jwt = require('jsonwebtoken');
const bcrypt = require('bcryptjs');
const router = express.Router();
// In-memory user store (replace with a database in a real application)
const users = [
{ id: 1, username: 'user1', password: '$2a$10$XOPbrlUPQdwdJUpSrIF6X.LbE14qsMmKGhM1A8W9iqDOMk9jc8uV.' }, // Password: password1
];
// Login route
router.post('/login', async (req, res) => {
const { username, password } = req.body;
// Find user
const user = users.find(u => u.username === username);
if (!user) return res.status(400).json({ message: 'Invalid username or password' });
// Check password
const validPassword = await bcrypt.compare(password, user.password);
if (!validPassword) return res.status(400).json({ message: 'Invalid username or password' });
// Create and assign token
const token = jwt.sign({ id: user.id }, 'your-secret-key', { expiresIn: '1h' });
res.json({ token });
});
module.exports = router;
Next, create a middleware to verify the JWT token. Create a new file called middleware/auth.js
:
const jwt = require('jsonwebtoken');
function auth(req, res, next) {
const token = req.header('Authorization');
if (!token) return res.status(401).json({ message: 'Access denied. No token provided.' });
try {
const decoded = jwt.verify(token, 'your-secret-key');
req.user = decoded;
next();
} catch (ex) {
res.status(400).json({ message: 'Invalid token.' });
}
}
module.exports = auth;
Now, update your server.js
file to include the new auth routes and use the auth middleware for protected routes:
// ... (previous imports)
const authRouter = require('./routes/auth');
const auth = require('./middleware/auth');
// ... (other middleware)
// Routes
app.use('/api/auth', authRouter);
app.use('/api/users', auth, usersRouter); // Protect user routes
// ... (rest of the code)
With these changes, users will need to authenticate by sending a POST request to /api/auth/login
with their username and password. The server will respond with a JWT token, which should be included in the Authorization header for subsequent requests to protected routes.
8. Testing Your RESTful API
Testing is an essential part of API development. Let’s set up some basic tests for our API using Mocha and Chai.
First, install the necessary packages:
npm install --save-dev mocha chai supertest
Create a new directory called test
and add a file called users.test.js
:
const chai = require('chai');
const expect = chai.expect;
const request = require('supertest');
const app = require('../server'); // Make sure to export the app from server.js
describe('Users API', () => {
let token;
before(async () => {
// Login and get token
const res = await request(app)
.post('/api/auth/login')
.send({ username: 'user1', password: 'password1' });
token = res.body.token;
});
describe('GET /api/users', () => {
it('should return all users', async () => {
const res = await request(app)
.get('/api/users')
.set('Authorization', token);
expect(res.status).to.equal(200);
expect(res.body).to.be.an('array');
});
});
describe('POST /api/users', () => {
it('should create a new user', async () => {
const res = await request(app)
.post('/api/users')
.set('Authorization', token)
.send({ name: 'Test User', email: 'test@example.com' });
expect(res.status).to.equal(201);
expect(res.body).to.have.property('id');
expect(res.body.name).to.equal('Test User');
});
});
// Add more tests for other routes (GET /:id, PUT, DELETE)
});
Update your package.json
file to include a test script:
"scripts": {
"test": "mocha --exit"
}
Now you can run your tests using the command:
npm test
9. Best Practices and Advanced Concepts
As you continue to develop your RESTful API with Express.js, keep these best practices and advanced concepts in mind:
1. Use Environment Variables
Store sensitive information like database credentials and JWT secret keys in environment variables. You can use the dotenv
package to manage these variables.
2. Implement Rate Limiting
Protect your API from abuse by implementing rate limiting. The express-rate-limit
package is a good option for this.
3. Use HTTPS
Always use HTTPS in production to encrypt data in transit. You can use services like Let’s Encrypt to obtain free SSL certificates.
4. Implement Proper Logging
Use a logging library like Winston to implement proper logging in your application. This will help with debugging and monitoring.
5. Use Compression
Implement response compression to reduce the size of the response body. The compression
middleware can be used for this purpose.
6. Implement Caching
Use caching to improve the performance of your API. You can use in-memory caching with libraries like node-cache
or distributed caching with Redis.
7. Use a Database
For production applications, use a proper database instead of in-memory storage. Popular options include MongoDB for NoSQL and PostgreSQL for relational databases.
8. Implement Pagination
For endpoints that return large collections of data, implement pagination to improve performance and reduce bandwidth usage.
9. Use Async/Await
Utilize async/await syntax for handling asynchronous operations. This makes your code more readable and easier to reason about.
10. Implement API Versioning
Consider implementing API versioning to make it easier to introduce breaking changes without affecting existing clients.
10. Conclusion
Building RESTful APIs with Express.js is a powerful way to create scalable and efficient web applications. In this comprehensive guide, we’ve covered the basics of setting up an Express.js server, implementing CRUD operations, adding authentication, handling errors, and testing your API. We’ve also touched on some best practices and advanced concepts to consider as you continue to develop your API.
Remember that building a robust API is an iterative process. As you gain more experience and your application grows, you’ll likely need to refine your API design, improve performance, and add more advanced features.
Keep learning and exploring the vast ecosystem of Node.js and Express.js. There are many excellent resources available, including the official Express.js documentation, Node.js tutorials, and online courses that can help you deepen your understanding of RESTful API development.
Happy coding, and may your APIs be ever RESTful!