Design Patterns in Software Engineering: A Comprehensive Guide
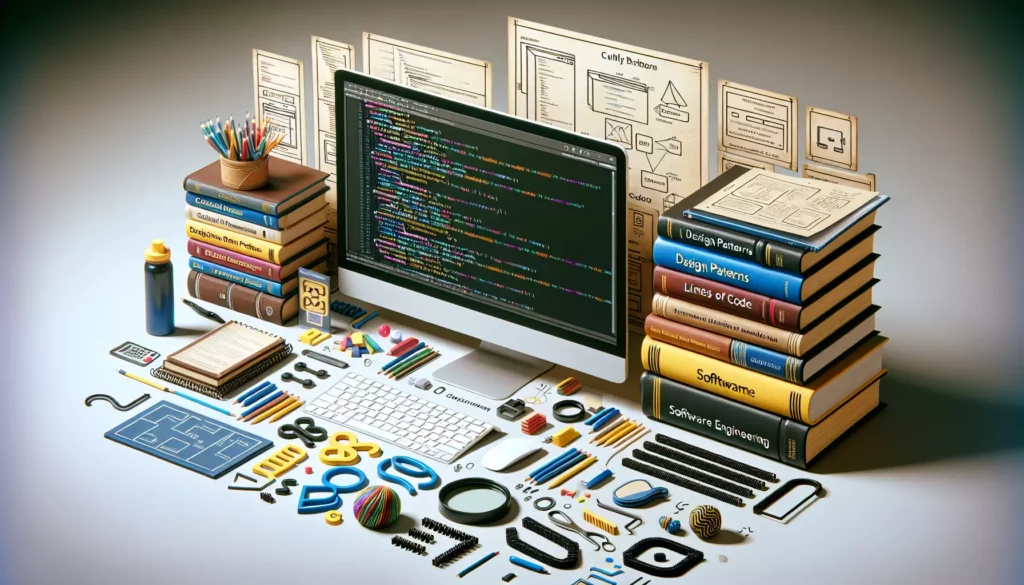
In the world of software engineering, efficiency and maintainability are paramount. As projects grow in complexity, developers need tried-and-true solutions to common problems. This is where design patterns come into play. In this comprehensive guide, we’ll explore what design patterns are, why they’re important, and how they can elevate your coding practices.
What Are Design Patterns?
Design patterns are reusable solutions to common problems in software design. They are not finished designs or pieces of code that can be directly implemented. Instead, they are templates or blueprints that can be applied to solve recurring design problems in object-oriented programming.
These patterns were first introduced in the seminal book “Design Patterns: Elements of Reusable Object-Oriented Software” by the “Gang of Four” (Erich Gamma, Richard Helm, Ralph Johnson, and John Vlissides) in 1994. Since then, design patterns have become an essential part of software engineering education and practice.
Why Are Design Patterns Important?
Design patterns offer several benefits to software developers:
- Reusability: They provide proven solutions that can be adapted to various situations, saving time and effort.
- Common vocabulary: They establish a shared language among developers, making communication about software design more efficient.
- Best practices: They encapsulate best practices developed and refined by experienced software developers.
- Scalability: They help in creating more flexible and scalable software architectures.
- Maintainability: They often result in code that is easier to understand and maintain.
Categories of Design Patterns
Design patterns are typically categorized into three main groups:
1. Creational Patterns
These patterns deal with object creation mechanisms, trying to create objects in a manner suitable to the situation. Some examples include:
- Singleton Pattern
- Factory Method Pattern
- Abstract Factory Pattern
- Builder Pattern
- Prototype Pattern
2. Structural Patterns
These patterns are concerned with how classes and objects are composed to form larger structures. Examples include:
- Adapter Pattern
- Bridge Pattern
- Composite Pattern
- Decorator Pattern
- Facade Pattern
3. Behavioral Patterns
These patterns are about identifying common communication patterns between objects and realizing these patterns. Some examples are:
- Observer Pattern
- Strategy Pattern
- Command Pattern
- State Pattern
- Template Method Pattern
Common Design Patterns in Detail
Let’s dive deeper into some of the most frequently used design patterns:
Singleton Pattern
The Singleton pattern ensures that a class has only one instance and provides a global point of access to it. This is useful when exactly one object is needed to coordinate actions across the system.
Here’s a simple implementation of the Singleton pattern in Python:
class Singleton:
_instance = None
def __new__(cls):
if cls._instance is None:
cls._instance = super(Singleton, cls).__new__(cls)
return cls._instance
# Usage
s1 = Singleton()
s2 = Singleton()
print(s1 is s2) # Output: True
Factory Method Pattern
The Factory Method pattern defines an interface for creating an object, but lets subclasses decide which class to instantiate. This pattern is particularly useful when a class can’t anticipate the type of objects it needs to create.
Here’s a simple example in Python:
from abc import ABC, abstractmethod
class Animal(ABC):
@abstractmethod
def speak(self):
pass
class Dog(Animal):
def speak(self):
return "Woof!"
class Cat(Animal):
def speak(self):
return "Meow!"
class AnimalFactory:
def create_animal(self, animal_type):
if animal_type == "dog":
return Dog()
elif animal_type == "cat":
return Cat()
else:
raise ValueError("Unknown animal type")
# Usage
factory = AnimalFactory()
dog = factory.create_animal("dog")
cat = factory.create_animal("cat")
print(dog.speak()) # Output: Woof!
print(cat.speak()) # Output: Meow!
Observer Pattern
The Observer pattern defines a one-to-many dependency between objects so that when one object changes state, all its dependents are notified and updated automatically. This pattern is commonly used in event handling systems.
Here’s a basic implementation in Python:
class Subject:
def __init__(self):
self._observers = []
self._state = None
def attach(self, observer):
self._observers.append(observer)
def detach(self, observer):
self._observers.remove(observer)
def notify(self):
for observer in self._observers:
observer.update(self._state)
def set_state(self, state):
self._state = state
self.notify()
class Observer:
def update(self, state):
pass
class ConcreteObserver(Observer):
def update(self, state):
print(f"State changed to: {state}")
# Usage
subject = Subject()
observer1 = ConcreteObserver()
observer2 = ConcreteObserver()
subject.attach(observer1)
subject.attach(observer2)
subject.set_state("New State")
# Output:
# State changed to: New State
# State changed to: New State
Choosing the Right Design Pattern
Selecting the appropriate design pattern for a given problem is crucial. Here are some guidelines to help you choose:
- Understand the problem: Clearly define the problem you’re trying to solve.
- Consider the context: Think about the broader system architecture and how the pattern will fit in.
- Evaluate trade-offs: Each pattern has its pros and cons. Consider the implications on performance, complexity, and maintainability.
- Look for similarities: If your problem resembles a classic problem solved by a specific pattern, that pattern might be a good fit.
- Start simple: Don’t over-engineer. Sometimes, a simple solution is better than a complex pattern.
Anti-Patterns: What to Avoid
While design patterns represent best practices, anti-patterns represent common mistakes or bad practices. Some common anti-patterns include:
- God Object: A class that tries to do too much, violating the single responsibility principle.
- Spaghetti Code: Code with a complex and tangled control structure.
- Golden Hammer: Trying to solve every problem with a familiar tool or pattern, even when it’s not the best fit.
- Premature Optimization: Optimizing code before it’s necessary, often leading to more complex and harder-to-maintain code.
Design Patterns in Modern Software Development
While the core concepts of design patterns remain relevant, their application has evolved with modern software development practices:
Microservices Architecture
In microservices, patterns like API Gateway, Circuit Breaker, and Saga have become increasingly important for managing distributed systems.
Functional Programming
Some traditional OOP patterns are less relevant in functional programming, but new patterns have emerged, such as monads and functors.
Reactive Programming
Patterns like Observable and Reactor are crucial in reactive programming for managing asynchronous data streams.
Learning and Applying Design Patterns
To become proficient in using design patterns:
- Study the classics: Start with the patterns described in the Gang of Four book.
- Practice: Implement patterns in small projects to understand their nuances.
- Analyze existing code: Look for patterns in open-source projects and frameworks.
- Stay updated: Keep learning about new patterns emerging in modern software development.
- Discuss with peers: Share experiences and insights about pattern usage with other developers.
Design Patterns and Software Architecture
Design patterns play a crucial role in shaping software architecture. They help in creating modular, scalable, and maintainable systems. Some architectural patterns that incorporate multiple design patterns include:
- Model-View-Controller (MVC): Separates an application into three interconnected components.
- Model-View-ViewModel (MVVM): A variation of MVC often used in modern web and mobile applications.
- Layered Architecture: Organizes the application into layers with distinct responsibilities.
Design Patterns in Different Programming Languages
While the concepts of design patterns are language-agnostic, their implementation can vary across different programming languages:
Java
Java’s strong typing and OOP focus make it well-suited for classic design patterns. For example, the Singleton pattern in Java might look like this:
public class Singleton {
private static Singleton instance;
private Singleton() {}
public static synchronized Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
}
JavaScript
In JavaScript, patterns often leverage the language’s functional nature and prototypal inheritance. Here’s an example of the Module pattern:
const Module = (function() {
let privateVariable = 0;
function privateMethod() {
console.log("This is private");
}
return {
publicMethod: function() {
privateVariable++;
privateMethod();
},
getCount: function() {
return privateVariable;
}
};
})();
Module.publicMethod();
console.log(Module.getCount()); // Output: 1
Python
Python’s simplicity and dynamic typing can lead to more concise implementations of patterns. Here’s an example of the Decorator pattern:
def decorator(func):
def wrapper():
print("Something is happening before the function is called.")
func()
print("Something is happening after the function is called.")
return wrapper
@decorator
def say_hello():
print("Hello!")
say_hello()
# Output:
# Something is happening before the function is called.
# Hello!
# Something is happening after the function is called.
Design Patterns in Popular Frameworks
Many popular frameworks and libraries incorporate design patterns in their architecture:
- React: Uses the Observer pattern through its state management and the Composite pattern in its component structure.
- Angular: Heavily relies on the Dependency Injection pattern and implements the Observer pattern through RxJS.
- Spring Framework: Utilizes numerous patterns including Singleton, Factory, and Proxy.
Challenges in Using Design Patterns
While design patterns offer many benefits, they also come with challenges:
- Overuse: Applying patterns unnecessarily can lead to overly complex code.
- Misuse: Using a pattern in the wrong context can create more problems than it solves.
- Learning curve: Understanding when and how to apply patterns requires experience and practice.
- Performance considerations: Some patterns might introduce performance overhead if not implemented carefully.
The Future of Design Patterns
As software development continues to evolve, so do design patterns:
- AI and Machine Learning: New patterns are emerging to handle the unique challenges of AI systems.
- Cloud-native development: Patterns for distributed systems and containerization are becoming increasingly important.
- IoT and Edge Computing: These domains are driving the development of patterns for resource-constrained environments and real-time processing.
Conclusion
Design patterns are a fundamental tool in a software engineer’s toolkit. They provide proven solutions to common problems, enhance code reusability, and improve communication among developers. While mastering design patterns requires time and practice, the investment pays off in more robust, maintainable, and efficient software systems.
As you continue your journey in software development, remember that design patterns are guidelines, not strict rules. Always consider the specific context of your project and be prepared to adapt or combine patterns as needed. With a solid understanding of design patterns, you’ll be better equipped to tackle complex software challenges and create high-quality, scalable applications.
Keep learning, keep practicing, and don’t hesitate to explore beyond the classic patterns. The world of software design is constantly evolving, and staying curious will help you grow as a developer and contribute to the ongoing evolution of software engineering practices.