Big O Notation in Algorithm Analysis: A Comprehensive Guide
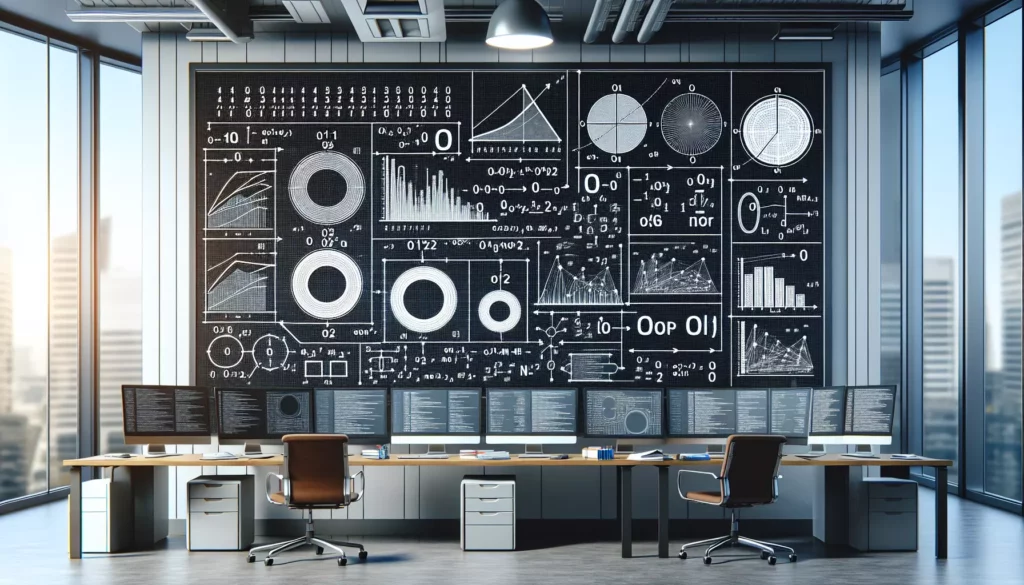
In the world of computer science and programming, efficiency is key. As software systems grow more complex and data sets become larger, the need for efficient algorithms becomes increasingly critical. This is where Big O notation comes into play. It’s a fundamental concept in algorithm analysis that helps developers understand and compare the performance of different algorithms. In this comprehensive guide, we’ll dive deep into Big O notation, exploring its importance, how it works, and how you can use it to become a better programmer.
What is Big O Notation?
Big O notation is a mathematical notation used in computer science to describe the performance or complexity of an algorithm. Specifically, it describes the worst-case scenario, or the maximum time an algorithm will take to complete as the input size grows. The “O” in Big O notation stands for “Order of”, which refers to the order of growth of the algorithm’s running time.
Big O notation allows us to express the runtime in terms of how quickly it grows relative to the input, as the input gets arbitrarily large. It’s not about the exact number of operations, but rather about how the number of operations grows as the input size increases.
Why is Big O Notation Important?
Understanding Big O notation is crucial for several reasons:
- Efficiency: It helps you write more efficient code by allowing you to compare different algorithms and choose the most suitable one for your specific use case.
- Scalability: It provides insight into how your algorithm will perform as the input size grows, which is crucial for building scalable applications.
- Optimization: By understanding the time and space complexity of your algorithms, you can identify bottlenecks and optimize your code more effectively.
- Interview Preparation: Big O notation is a common topic in technical interviews, especially for positions at major tech companies.
Common Big O Notations
Let’s explore some of the most common Big O notations, from fastest to slowest:
O(1) – Constant Time
An algorithm with O(1) complexity performs the same number of operations regardless of the input size. This is the most efficient Big O time complexity.
Example: Accessing an array element by its index.
function getFirstElement(arr) {
return arr[0];
}
O(log n) – Logarithmic Time
The number of operations grows logarithmically with the input size. This is very efficient, especially for large inputs.
Example: Binary search algorithm.
function binarySearch(arr, target) {
let left = 0;
let right = arr.length - 1;
while (left <= right) {
let mid = Math.floor((left + right) / 2);
if (arr[mid] === target) return mid;
if (arr[mid] < target) left = mid + 1;
else right = mid - 1;
}
return -1;
}
O(n) – Linear Time
The number of operations grows linearly with the input size. This is generally considered good performance.
Example: Linear search algorithm.
function linearSearch(arr, target) {
for (let i = 0; i < arr.length; i++) {
if (arr[i] === target) return i;
}
return -1;
}
O(n log n) – Linearithmic Time
This complexity is common in efficient sorting algorithms. It’s more efficient than O(n^2) but less efficient than O(n).
Example: Merge sort algorithm.
function mergeSort(arr) {
if (arr.length <= 1) return arr;
const mid = Math.floor(arr.length / 2);
const left = arr.slice(0, mid);
const right = arr.slice(mid);
return merge(mergeSort(left), mergeSort(right));
}
function merge(left, right) {
let result = [];
let leftIndex = 0;
let rightIndex = 0;
while (leftIndex < left.length && rightIndex < right.length) {
if (left[leftIndex] < right[rightIndex]) {
result.push(left[leftIndex]);
leftIndex++;
} else {
result.push(right[rightIndex]);
rightIndex++;
}
}
return result.concat(left.slice(leftIndex)).concat(right.slice(rightIndex));
}
O(n^2) – Quadratic Time
The number of operations grows quadratically with the input size. This is less efficient and can become problematic for large inputs.
Example: Bubble sort algorithm.
function bubbleSort(arr) {
const n = arr.length;
for (let i = 0; i < n; i++) {
for (let j = 0; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
// Swap elements
[arr[j], arr[j + 1]] = [arr[j + 1], arr[j]];
}
}
}
return arr;
}
O(2^n) – Exponential Time
The number of operations doubles with each addition to the input. This is very inefficient and is typically seen in recursive algorithms that solve a problem of size N by recursively solving two smaller problems of size N-1.
Example: Recursive calculation of Fibonacci numbers (naive approach).
function fibonacci(n) {
if (n <= 1) return n;
return fibonacci(n - 1) + fibonacci(n - 2);
}
How to Calculate Big O Notation
Calculating the Big O notation of an algorithm involves analyzing the code and determining how the number of operations grows as the input size increases. Here are some steps to follow:
- Identify the input: Determine what the algorithm’s input is and how it affects the number of operations.
- Count the operations: Look at each line of code and count how many operations it performs.
- Focus on dominant terms: As the input size grows, lower-order terms become less significant. Focus on the term that grows the fastest.
- Drop constants: In Big O notation, we drop constant factors. For example, O(2n) becomes O(n).
- Consider nested loops: Nested loops often lead to multiplication of terms. For example, a loop inside another loop might result in O(n^2) complexity.
Common Pitfalls in Big O Analysis
When analyzing algorithms using Big O notation, there are several common mistakes to avoid:
1. Forgetting about space complexity
Big O notation isn’t just about time complexity; it’s also used to describe space complexity. Always consider both when analyzing an algorithm.
2. Ignoring constant factors in practice
While we drop constants in Big O notation, in practice, an O(100n) algorithm might be slower than an O(n^2) algorithm for small inputs. Always consider the actual use case.
3. Assuming worst-case scenario always applies
Big O describes the worst-case scenario, but in practice, algorithms might perform better on average. Consider average-case analysis as well.
4. Overcomplicating the analysis
Sometimes, developers overthink the analysis. Remember, Big O is about the rate of growth, not the exact number of operations.
5. Not considering the input size
An O(n^2) algorithm might be fine for small inputs but problematic for large ones. Always consider the expected input size when choosing an algorithm.
Practical Applications of Big O Notation
Understanding Big O notation isn’t just an academic exercise; it has real-world applications in software development:
1. Algorithm Selection
When faced with a problem, understanding Big O helps you choose the most efficient algorithm. For example, if you need to search a sorted array, knowing that binary search (O(log n)) is more efficient than linear search (O(n)) can greatly improve your program’s performance.
2. Database Optimization
In database design, understanding the time complexity of different operations can help in creating efficient indexes and query structures.
3. API Design
When designing APIs, considering the Big O complexity of your endpoints can help ensure they remain responsive even as the amount of data grows.
4. System Scalability
Understanding how your algorithms scale is crucial when building systems that need to handle large amounts of data or traffic.
5. Code Optimization
Big O analysis can help identify bottlenecks in your code, guiding your optimization efforts to where they’ll have the most impact.
Big O Notation in Different Programming Paradigms
While the principles of Big O notation apply universally, how you analyze and optimize code can vary depending on the programming paradigm you’re working with:
Imperative Programming
In imperative programming, you’re often dealing directly with loops and conditional statements. Here, Big O analysis often involves counting loop iterations and considering how nested structures affect complexity.
Functional Programming
Functional programming emphasizes immutability and the use of higher-order functions. When analyzing functional code, you might need to consider the complexity of operations like map, reduce, or filter, and how they compose.
Object-Oriented Programming
In OOP, complexity can be hidden within method calls and object interactions. Analyzing OOP code often requires considering the complexity of method calls and how objects interact.
Big O Notation and Data Structures
Different data structures have different time complexities for various operations. Understanding these can help you choose the right data structure for your needs:
Arrays
- Access: O(1)
- Search: O(n)
- Insertion: O(n)
- Deletion: O(n)
Linked Lists
- Access: O(n)
- Search: O(n)
- Insertion: O(1)
- Deletion: O(1)
Hash Tables
- Search: O(1) average, O(n) worst case
- Insertion: O(1) average, O(n) worst case
- Deletion: O(1) average, O(n) worst case
Binary Search Trees
- Search: O(log n) average, O(n) worst case
- Insertion: O(log n) average, O(n) worst case
- Deletion: O(log n) average, O(n) worst case
Advanced Topics in Big O Notation
As you become more comfortable with Big O notation, you might encounter some more advanced concepts:
Amortized Analysis
Amortized analysis is a method of analyzing the time complexity of operations in a sequence, rather than individually. This is particularly useful for data structures like dynamic arrays, where occasional operations might be costly, but are infrequent enough that the average cost remains low.
Big Ω (Omega) and Big Θ (Theta) Notation
While Big O describes the upper bound of an algorithm’s running time, Big Ω describes the lower bound, and Big Θ describes both the upper and lower bounds. These can provide a more complete picture of an algorithm’s performance.
Multi-variable Big O Notation
Some algorithms have multiple input variables that affect their running time. In these cases, we might use notation like O(nm) to describe an algorithm’s complexity in terms of two variables, n and m.
Conclusion
Big O notation is a powerful tool in a programmer’s toolkit. It provides a standardized way to discuss and compare the efficiency of algorithms, helping developers make informed decisions about algorithm selection and optimization. By understanding Big O notation, you can write more efficient code, build more scalable systems, and excel in technical interviews.
Remember, while Big O notation is crucial, it’s not the only factor to consider when writing code. Readability, maintainability, and correctness are equally important. Strive for a balance between theoretical efficiency and practical concerns in your day-to-day coding.
As you continue your journey in software development, keep practicing Big O analysis on the algorithms you encounter and write. Over time, you’ll develop an intuition for algorithmic efficiency that will serve you well throughout your career.