How to Study and Understand Algorithms Efficiently: A Comprehensive Guide
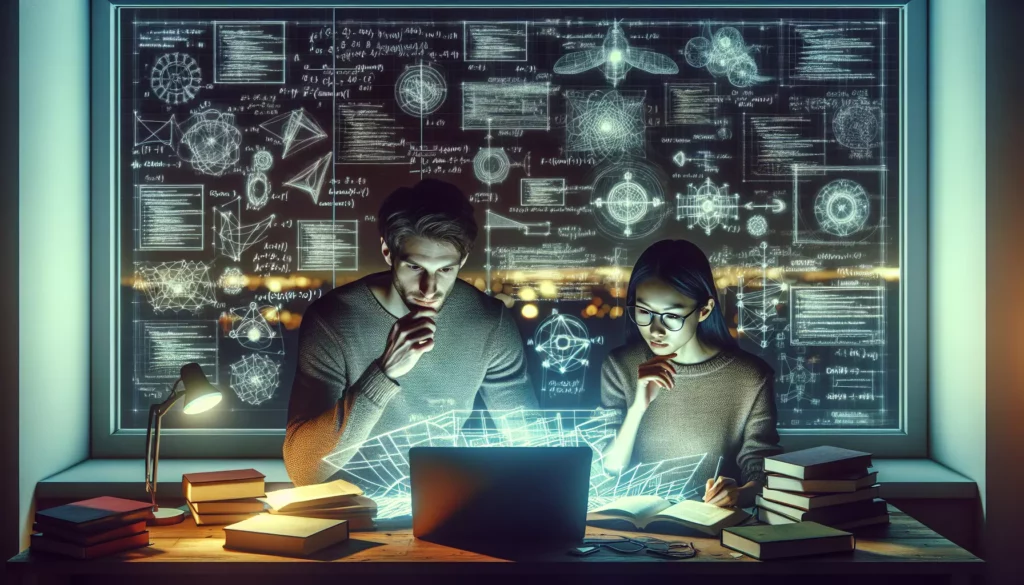
In the ever-evolving world of technology and computer science, algorithms play a crucial role in solving complex problems and optimizing processes. Whether you’re a beginner programmer or an experienced developer preparing for technical interviews at major tech companies, understanding algorithms is essential. This comprehensive guide will walk you through effective strategies to study and comprehend algorithms efficiently, helping you build a strong foundation in algorithmic thinking and problem-solving skills.
1. Start with the Basics
Before diving into complex algorithms, it’s crucial to have a solid understanding of fundamental concepts. These include:
- Data structures (arrays, linked lists, stacks, queues, trees, graphs)
- Time and space complexity analysis
- Basic programming constructs (loops, conditionals, functions)
- Recursion
Ensure you have a good grasp of these concepts, as they form the building blocks for more advanced algorithms.
2. Choose the Right Learning Resources
Selecting appropriate learning materials is crucial for efficient algorithm study. Consider the following resources:
2.1. Textbooks
Classic algorithm textbooks provide in-depth explanations and analysis. Some popular choices include:
- “Introduction to Algorithms” by Cormen, Leiserson, Rivest, and Stein
- “Algorithms” by Robert Sedgewick and Kevin Wayne
- “The Algorithm Design Manual” by Steven S. Skiena
2.2. Online Courses
Many universities and platforms offer excellent algorithm courses online, such as:
- Coursera’s “Algorithms Specialization” by Stanford University
- edX’s “Algorithms and Data Structures” by MIT
- Udacity’s “Intro to Algorithms”
2.3. Interactive Platforms
Platforms like AlgoCademy offer interactive coding tutorials and AI-powered assistance, making it easier to learn and practice algorithms in a hands-on environment.
2.4. Video Tutorials
YouTube channels and online video courses can provide visual explanations of complex algorithms. Some popular channels include:
- MIT OpenCourseWare
- mycodeschool
- Abdul Bari
3. Develop a Structured Learning Plan
Create a systematic approach to studying algorithms:
- Start with basic algorithms (sorting, searching)
- Move on to intermediate topics (dynamic programming, greedy algorithms)
- Progress to advanced algorithms (graph algorithms, string matching)
- Study specialized algorithms based on your interests or career goals
Set realistic goals and deadlines to keep yourself accountable and motivated throughout your learning journey.
4. Practice Implementation
Reading about algorithms is not enough; you need to implement them to truly understand their workings. Follow these steps:
- Choose a programming language you’re comfortable with
- Implement algorithms from scratch without relying on built-in functions
- Test your implementations with various input cases
- Optimize your code for better time and space complexity
Platforms like AlgoCademy provide interactive coding environments where you can practice implementing algorithms and receive immediate feedback.
5. Visualize Algorithms
Visual representations can significantly enhance your understanding of how algorithms work. Utilize the following techniques:
- Draw diagrams or flowcharts to represent algorithm steps
- Use online algorithm visualizers (e.g., VisuAlgo, Algorithm Visualizer)
- Create your own animations or simulations
Visualization helps you grasp the logic behind algorithms and identify potential optimization opportunities.
6. Analyze Time and Space Complexity
Understanding the efficiency of algorithms is crucial. For each algorithm you study:
- Analyze its time complexity (Big O notation)
- Evaluate its space complexity
- Compare it with alternative algorithms for the same problem
- Identify trade-offs between time and space efficiency
Practice analyzing complexity for various input sizes and scenarios to develop a intuitive understanding of algorithm performance.
7. Study Algorithm Design Techniques
Familiarize yourself with common algorithm design paradigms:
- Divide and Conquer
- Dynamic Programming
- Greedy Algorithms
- Backtracking
- Branch and Bound
Understanding these techniques will help you approach new problems and develop efficient solutions.
8. Solve Problems Regularly
Consistent practice is key to mastering algorithms. Engage in problem-solving activities:
- Solve algorithm problems on platforms like LeetCode, HackerRank, or CodeForces
- Participate in coding competitions or hackathons
- Implement real-world projects that require algorithmic solutions
AlgoCademy offers a wide range of practice problems and challenges to help you hone your skills and prepare for technical interviews.
9. Understand the Problem-Solving Process
Develop a systematic approach to tackling algorithm problems:
- Understand the problem statement thoroughly
- Identify the input and expected output
- Consider edge cases and constraints
- Brainstorm potential solutions
- Choose the most efficient approach
- Implement the solution
- Test and debug
- Optimize if necessary
Following this process will help you approach problems methodically and improve your problem-solving skills.
10. Learn from Others
Collaboration and learning from peers can accelerate your understanding of algorithms:
- Join coding communities or study groups
- Participate in online forums (e.g., Stack Overflow, Reddit’s r/algorithms)
- Attend coding meetups or workshops
- Review and analyze other people’s code solutions
Engaging with the programming community exposes you to different perspectives and problem-solving approaches.
11. Teach Others
One of the most effective ways to solidify your understanding of algorithms is to teach them to others:
- Explain concepts to classmates or colleagues
- Write blog posts or create video tutorials
- Mentor junior developers
- Contribute to open-source algorithm libraries
Teaching forces you to break down complex concepts into simpler terms, reinforcing your own understanding in the process.
12. Focus on Understanding, Not Memorization
While it’s important to know common algorithms, focus on understanding the underlying principles rather than memorizing implementations:
- Analyze why certain algorithms work for specific problems
- Study the trade-offs between different algorithmic approaches
- Explore how to adapt algorithms to solve variations of a problem
- Practice deriving algorithms from first principles
This approach will help you develop the ability to create novel solutions when faced with unique problems.
13. Implement Data Structures from Scratch
To truly understand how algorithms interact with data structures:
- Implement basic data structures (linked lists, stacks, queues) from scratch
- Build more complex structures (trees, graphs, hash tables)
- Analyze the time and space complexity of operations on these structures
- Compare your implementations with standard library implementations
This exercise will give you a deeper appreciation for the intricacies of data manipulation in algorithms.
14. Study Algorithm History and Evolution
Understanding the historical context of algorithms can provide valuable insights:
- Learn about the origins of fundamental algorithms
- Study how algorithms have evolved over time
- Explore recent advancements in algorithmic research
- Understand the impact of hardware improvements on algorithm efficiency
This knowledge will help you appreciate the ongoing development in the field and inspire you to contribute to future advancements.
15. Apply Algorithms to Real-World Problems
To make your learning more relevant and engaging:
- Identify algorithmic problems in your daily life or work
- Implement solutions for real-world scenarios
- Analyze the performance of your solutions in practical settings
- Iterate and improve your implementations based on real-world constraints
This approach helps bridge the gap between theoretical knowledge and practical application.
16. Use Spaced Repetition
To retain your knowledge of algorithms over time:
- Review concepts and implementations periodically
- Use spaced repetition software (e.g., Anki) to create flashcards
- Revisit and solve previously encountered problems
- Gradually increase the time between review sessions as your understanding improves
This technique helps combat the forgetting curve and ensures long-term retention of algorithmic concepts.
17. Prepare for Technical Interviews
If your goal is to ace technical interviews at major tech companies:
- Focus on commonly asked algorithm questions
- Practice explaining your thought process while solving problems
- Simulate interview conditions (time constraints, whiteboarding)
- Study company-specific interview patterns and expectations
Platforms like AlgoCademy offer targeted preparation resources for technical interviews at FAANG and other top tech companies.
18. Explore Advanced Topics
Once you have a solid foundation, delve into more advanced algorithmic concepts:
- Approximation algorithms
- Randomized algorithms
- Parallel and distributed algorithms
- Machine learning algorithms
- Quantum algorithms
Studying these topics will broaden your understanding and keep you at the forefront of algorithmic developments.
19. Analyze Algorithm Correctness
Develop skills to prove the correctness of algorithms:
- Study formal methods for algorithm verification
- Practice writing mathematical proofs for algorithm correctness
- Analyze edge cases and boundary conditions
- Use induction and other proof techniques
This skill is crucial for developing robust and reliable algorithmic solutions.
20. Stay Updated with Research
Keep yourself informed about the latest developments in algorithm research:
- Read academic papers from top computer science conferences
- Follow algorithm researchers on social media
- Attend webinars or conferences on algorithmic advancements
- Experiment with implementing newly published algorithms
Staying current with research will inspire you and provide insights into cutting-edge algorithmic techniques.
Conclusion
Studying and understanding algorithms efficiently requires a multifaceted approach combining theoretical knowledge, practical implementation, and consistent practice. By following the strategies outlined in this guide, you can develop a strong foundation in algorithmic thinking and problem-solving skills. Remember that mastering algorithms is a continuous journey, and the key to success lies in persistence, curiosity, and a willingness to tackle challenging problems.
Platforms like AlgoCademy can significantly accelerate your learning process by providing interactive coding tutorials, AI-powered assistance, and a wealth of resources tailored for algorithm study and interview preparation. Whether you’re aiming to excel in your computer science courses, prepare for technical interviews at top tech companies, or simply enhance your programming skills, a structured and efficient approach to studying algorithms will set you on the path to success in the world of computer science and software development.