15 Beginner-Friendly Programming Projects to Kickstart Your Coding Journey
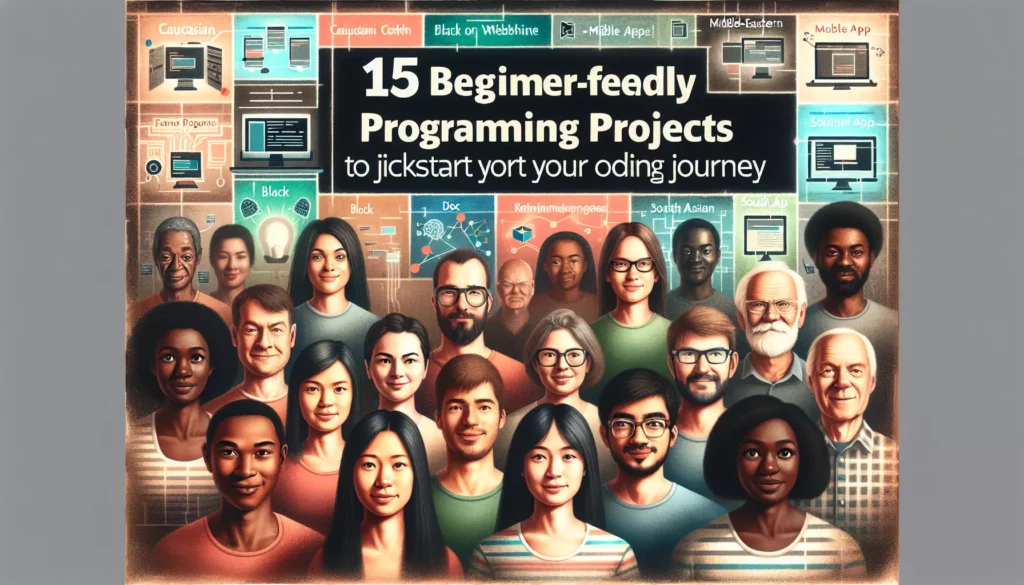
If you’re just starting your programming journey, one of the best ways to learn is by working on real projects. But finding the right project can be challenging – you want something that’s not too difficult, yet engaging enough to keep you motivated. That’s why we’ve compiled this list of 15 beginner-friendly programming projects that are perfect for newcomers to coding. These projects will help you apply your newfound skills, build your portfolio, and gain practical experience that will set you up for success in your programming career.
1. Calculator App
Creating a calculator app is a classic beginner project that helps you practice basic programming concepts like variables, conditional statements, and user input handling. You can start with a simple calculator that performs basic arithmetic operations and gradually add more complex features like scientific functions or a graphing calculator.
Key Skills You’ll Learn:
- Basic arithmetic operations
- User input handling
- Conditional statements
- Function creation and usage
Implementation Tips:
- Start with a command-line interface before moving to a graphical user interface (GUI)
- Use functions to organize your code for each operation
- Implement error handling for invalid inputs
2. To-Do List Application
A to-do list app is an excellent project for beginners as it introduces you to the concept of data structures and basic CRUD (Create, Read, Update, Delete) operations. You’ll learn how to store and manipulate data, which is a fundamental skill in programming.
Key Skills You’ll Learn:
- Working with arrays or lists
- Implementing CRUD operations
- Basic file I/O for data persistence
- User interface design (if creating a GUI version)
Implementation Tips:
- Start with a simple list that allows adding and viewing tasks
- Gradually add features like marking tasks as complete, deleting tasks, and setting due dates
- Consider using a text file to save tasks between program runs
3. Guess the Number Game
This simple game is an excellent way to practice working with random number generation, user input, and conditional statements. The computer generates a random number, and the player tries to guess it, receiving hints about whether their guess is too high or too low.
Key Skills You’ll Learn:
- Random number generation
- Loops and conditional statements
- User input validation
- Basic game logic
Implementation Tips:
- Start with a fixed range (e.g., 1-100) and later allow the user to set the range
- Add a counter to track the number of guesses
- Implement difficulty levels by limiting the number of allowed guesses
4. Simple Weather App
Creating a weather app introduces you to working with APIs (Application Programming Interfaces) and parsing JSON data. This project will teach you how to retrieve real-time data from external sources and display it to the user.
Key Skills You’ll Learn:
- Making HTTP requests
- Working with APIs
- Parsing JSON data
- Error handling for network requests
Implementation Tips:
- Use a free weather API like OpenWeatherMap
- Start by displaying basic information like temperature and conditions
- Gradually add features like forecasts and location search
5. Hangman Game
Implementing the classic Hangman game helps you practice working with strings, arrays, and loops. It’s also a great way to learn about ASCII art for displaying the hangman figure.
Key Skills You’ll Learn:
- String manipulation
- Array operations
- Game state management
- ASCII art (for displaying the hangman)
Implementation Tips:
- Start with a predefined list of words and later add the ability to fetch words from an API
- Implement a simple ASCII art display for the hangman
- Add difficulty levels by adjusting the number of allowed incorrect guesses
6. Rock Paper Scissors Game
This classic game is perfect for practicing conditional logic and random choice generation. It’s also a good introduction to creating simple AI opponents.
Key Skills You’ll Learn:
- Conditional statements
- Random choice generation
- Game logic implementation
- Basic AI concepts
Implementation Tips:
- Start with a command-line interface
- Implement a scoring system to track wins, losses, and ties
- Add different AI strategies (e.g., random, pattern-based) for increased difficulty
7. Password Generator
Creating a password generator is an excellent way to learn about string manipulation, random selection, and working with user preferences. This project also introduces you to basic security concepts.
Key Skills You’ll Learn:
- Random character generation
- String manipulation
- User input for customization
- Basic password security concepts
Implementation Tips:
- Allow users to specify password length and character types (uppercase, lowercase, numbers, symbols)
- Implement password strength checking
- Add an option to generate multiple passwords at once
8. Tic-Tac-Toe Game
Implementing Tic-Tac-Toe helps you practice working with 2D arrays or lists, game state management, and basic AI for the computer player. It’s also a great introduction to game development concepts.
Key Skills You’ll Learn:
- 2D array manipulation
- Game state checking (win conditions)
- Basic AI for computer player
- User interface design (if creating a GUI version)
Implementation Tips:
- Start with a command-line interface before moving to a graphical version
- Implement a simple AI that makes random valid moves
- Gradually improve the AI to make smarter moves (e.g., blocking the player from winning)
9. Simple Quiz Application
Building a quiz app helps you practice working with data structures, file I/O for storing questions and answers, and implementing scoring systems. It’s also a great way to learn about organizing and presenting information to users.
Key Skills You’ll Learn:
- Data structure design for questions and answers
- File I/O for loading quiz content
- Scoring system implementation
- User interface for presenting questions and collecting answers
Implementation Tips:
- Start with a small set of hardcoded questions before moving to file-based storage
- Implement different question types (multiple choice, true/false, short answer)
- Add features like timed quizzes or difficulty levels
10. URL Shortener
Creating a URL shortener introduces you to working with web technologies, databases, and unique ID generation. This project is an excellent stepping stone towards more complex web applications.
Key Skills You’ll Learn:
- Basic web server setup
- Database operations (storing and retrieving URLs)
- URL parsing and validation
- Generating unique short codes
Implementation Tips:
- Start with an in-memory storage before moving to a database
- Use a simple algorithm for generating short codes (e.g., random strings or incrementing IDs)
- Implement basic analytics (e.g., click counting)
11. Currency Converter
A currency converter app helps you practice working with APIs, handling real-time data, and performing calculations. It’s also a practical application that you might use in real life.
Key Skills You’ll Learn:
- API integration for exchange rates
- Numerical calculations and rounding
- User input validation for currency codes and amounts
- Caching exchange rates for improved performance
Implementation Tips:
- Use a free currency exchange rate API
- Start with a few major currencies before expanding to a comprehensive list
- Implement caching to reduce API calls and improve response times
12. Personal Portfolio Website
Creating a personal portfolio website is an excellent way to showcase your projects while learning about web development. This project introduces you to HTML, CSS, and potentially JavaScript for interactive elements.
Key Skills You’ll Learn:
- HTML structure and semantic markup
- CSS styling and layout
- Basic responsive design
- Version control with Git (for deploying your site)
Implementation Tips:
- Start with a simple single-page design
- Focus on clean, readable code and responsive layout
- Add interactive elements with JavaScript as you progress
13. Simple Note-Taking App
Building a note-taking app helps you practice data persistence, text editing features, and potentially cloud synchronization. It’s a great project for learning about local storage or simple database operations.
Key Skills You’ll Learn:
- Data persistence (local storage or database)
- Text editing and formatting
- Search functionality
- User authentication (for multi-user support)
Implementation Tips:
- Start with local storage before moving to a database solution
- Implement basic text formatting options
- Add features like note categorization and search functionality
14. Pomodoro Timer
Creating a Pomodoro timer introduces you to working with time-based events, audio notifications, and user settings. It’s a practical project that can help improve your productivity while you learn to code.
Key Skills You’ll Learn:
- Working with time and intervals
- Implementing audio notifications
- User settings and preferences
- Basic UI design for timers
Implementation Tips:
- Start with a simple timer that alternates between work and break periods
- Add customizable durations for work and break sessions
- Implement audio notifications and visual cues for session changes
15. Simple Drawing Application
Building a basic drawing app helps you learn about canvas manipulation, event handling, and creating user interfaces for creative tools. It’s an excellent introduction to graphics programming and user interaction.
Key Skills You’ll Learn:
- Working with HTML5 Canvas
- Handling mouse/touch events
- Implementing drawing tools (e.g., brush, eraser)
- Color selection and management
Implementation Tips:
- Start with basic line drawing functionality
- Add features like brush size adjustment and color selection
- Implement an eraser tool and the ability to clear the canvas
Conclusion
These 15 beginner-friendly programming projects offer a diverse range of challenges and learning opportunities. As you work through them, you’ll not only improve your coding skills but also gain valuable experience in problem-solving, project management, and creating user-friendly applications.
Remember, the key to learning programming is consistent practice and gradually increasing the complexity of your projects. Start with the projects that interest you the most, and don’t be afraid to modify or expand upon them as you gain confidence. Each project you complete will bring you one step closer to becoming a proficient programmer.
As you progress, consider exploring more advanced topics like data structures, algorithms, and software design patterns. Platforms like AlgoCademy can provide you with structured learning paths and interactive coding exercises to further enhance your skills and prepare you for technical interviews at top tech companies.
Happy coding, and may your programming journey be filled with exciting challenges and rewarding accomplishments!