Top Programming Languages in 2023: A Comprehensive Guide for Aspiring Developers
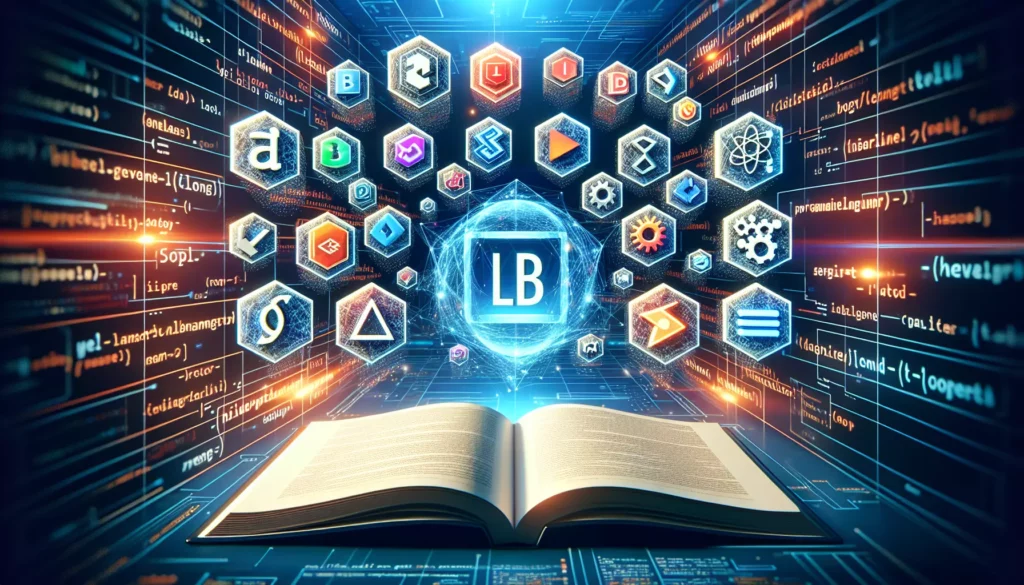
In the ever-evolving world of technology, staying up-to-date with the most in-demand programming languages is crucial for both aspiring and seasoned developers. As we navigate through 2023, the landscape of programming languages continues to shift, adapt, and expand to meet the needs of modern software development. In this comprehensive guide, we’ll explore the top programming languages that are dominating the tech industry this year, their key features, and why they’re essential for developers looking to advance their careers.
1. Python: The Versatile Powerhouse
Python continues to reign supreme as one of the most popular programming languages in 2023. Its versatility, readability, and extensive library support make it a go-to choice for developers across various domains.
Key Features:
- Easy to learn and read
- Extensive standard library and third-party packages
- Strong community support
- Ideal for web development, data science, AI, and machine learning
Python’s simplicity and power make it an excellent choice for beginners and experienced programmers alike. Here’s a simple example of a Python function that calculates the factorial of a number:
def factorial(n):
if n == 0 or n == 1:
return 1
else:
return n * factorial(n-1)
# Example usage
print(factorial(5)) # Output: 120
2. JavaScript: The Web Development Essential
JavaScript remains an indispensable language for web development in 2023. With the rise of full-stack development and the continued dominance of web applications, JavaScript’s importance cannot be overstated.
Key Features:
- Client-side and server-side scripting
- Extensive frameworks and libraries (React, Angular, Vue.js)
- Asynchronous programming capabilities
- Constantly evolving with new features and improvements
JavaScript’s ability to create interactive and dynamic web pages makes it a must-learn language for any aspiring web developer. Here’s a simple example of using JavaScript to change the content of an HTML element:
<!-- HTML -->
<p id="demo">Click the button to change this text.</p>
<button onclick="changeText()">Click me</button>
<!-- JavaScript -->
<script>
function changeText() {
document.getElementById("demo").innerHTML = "Text changed!";
}
</script>
3. Java: The Enterprise Standard
Java continues to be a cornerstone in enterprise software development. Its “write once, run anywhere” philosophy and robust ecosystem make it a top choice for large-scale applications.
Key Features:
- Platform independence
- Strong typing and object-oriented programming
- Extensive libraries and frameworks
- Ideal for Android app development
Java’s stability and scalability make it a preferred language for building complex, enterprise-level applications. Here’s a simple Java program that demonstrates object-oriented programming:
public class Car {
private String brand;
private String model;
public Car(String brand, String model) {
this.brand = brand;
this.model = model;
}
public void startEngine() {
System.out.println(brand + " " + model + " engine started.");
}
public static void main(String[] args) {
Car myCar = new Car("Toyota", "Corolla");
myCar.startEngine(); // Output: Toyota Corolla engine started.
}
}
4. C++: The Performance Powerhouse
C++ remains a crucial language in 2023, especially in areas where performance is paramount, such as game development, system programming, and high-frequency trading.
Key Features:
- High performance and efficiency
- Low-level memory manipulation
- Object-oriented and generic programming support
- Extensive standard library
C++’s ability to provide close-to-hardware programming makes it indispensable for performance-critical applications. Here’s a simple C++ program demonstrating the use of classes and objects:
#include <iostream>
#include <string>
class Person {
private:
std::string name;
int age;
public:
Person(std::string n, int a) : name(n), age(a) {}
void introduce() {
std::cout << "My name is " << name << " and I'm " << age << " years old." << std::endl;
}
};
int main() {
Person person("Alice", 30);
person.introduce(); // Output: My name is Alice and I'm 30 years old.
return 0;
}
5. Go: The Modern Systems Programming Language
Go, developed by Google, has gained significant traction in recent years and continues to be a top language in 2023, especially in the realm of cloud computing and microservices.
Key Features:
- Simplicity and ease of use
- Built-in concurrency support
- Fast compilation and execution
- Strong standard library
Go’s simplicity and efficiency make it an excellent choice for building scalable web services and network programming. Here’s a simple Go program that demonstrates concurrent execution using goroutines:
package main
import (
"fmt"
"time"
)
func sayHello(name string) {
time.Sleep(time.Second)
fmt.Printf("Hello, %s!\n", name)
}
func main() {
go sayHello("Alice")
go sayHello("Bob")
time.Sleep(2 * time.Second)
}
6. TypeScript: The JavaScript Enhancer
TypeScript, a superset of JavaScript, has been gaining popularity and is considered one of the top programming languages in 2023, especially for large-scale JavaScript projects.
Key Features:
- Static typing
- Object-oriented programming features
- Improved tooling and IDE support
- Compatibility with existing JavaScript code
TypeScript’s ability to catch errors at compile-time and improve code maintainability makes it increasingly popular among developers. Here’s a simple TypeScript example demonstrating static typing:
interface Person {
name: string;
age: number;
}
function greet(person: Person): string {
return `Hello, ${person.name}! You are ${person.age} years old.`;
}
const john: Person = { name: "John", age: 30 };
console.log(greet(john)); // Output: Hello, John! You are 30 years old.
7. Rust: The Safe Systems Programming Language
Rust has been steadily climbing the ranks and is considered one of the most loved programming languages in 2023. It’s particularly valued for its focus on memory safety and concurrent programming.
Key Features:
- Memory safety without garbage collection
- Concurrency without data races
- Zero-cost abstractions
- Pattern matching and powerful type system
Rust’s ability to prevent common programming errors at compile-time makes it an excellent choice for systems programming. Here’s a simple Rust program demonstrating ownership and borrowing concepts:
fn main() {
let s1 = String::from("hello");
let len = calculate_length(&s1);
println!("The length of '{}' is {}.", s1, len);
}
fn calculate_length(s: &String) -> usize {
s.len()
}
8. Swift: The Apple Ecosystem Language
Swift, developed by Apple, continues to be a crucial language in 2023 for iOS, macOS, watchOS, and tvOS app development.
Key Features:
- Fast and efficient performance
- Safe programming patterns
- Modern syntax and features
- Interoperability with Objective-C
Swift’s modern features and safety guarantees make it the preferred language for Apple platform development. Here’s a simple Swift example demonstrating optionals and optional binding:
func greet(name: String?) {
guard let unwrappedName = name else {
print("Hello, anonymous!")
return
}
print("Hello, \(unwrappedName)!")
}
greet(name: "Alice") // Output: Hello, Alice!
greet(name: nil) // Output: Hello, anonymous!
9. Kotlin: The Modern Java Alternative
Kotlin has been gaining popularity as a more concise and expressive alternative to Java, especially in Android app development.
Key Features:
- Interoperability with Java
- Concise and expressive syntax
- Null safety
- Coroutines for asynchronous programming
Kotlin’s modern features and Java interoperability make it an attractive choice for Android developers. Here’s a simple Kotlin program demonstrating null safety and string templates:
fun main() {
val name: String? = "Alice"
val greeting = "Hello, ${name ?: "anonymous"}!"
println(greeting) // Output: Hello, Alice!
}
10. R: The Statistical Computing Powerhouse
R continues to be a top language in 2023 for statistical computing, data analysis, and graphical visualization.
Key Features:
- Extensive statistical and graphical libraries
- Easy-to-use tools for data manipulation
- Strong community support in academia and industry
- Integration with other languages like C, C++, and Fortran
R’s specialized features for statistical computing make it indispensable in fields like data science and bioinformatics. Here’s a simple R script demonstrating basic data visualization:
# Create a vector of values
x <- c(1, 2, 3, 4, 5)
y <- c(2, 4, 6, 8, 10)
# Create a scatter plot
plot(x, y, main="Simple Scatter Plot", xlab="X axis", ylab="Y axis", pch=19, col="blue")
# Add a line of best fit
abline(lm(y ~ x), col="red")
Choosing the Right Language for Your Career
While these are the top programming languages in 2023, it’s important to remember that the best language for you depends on your career goals, interests, and the specific problems you want to solve. Here are some factors to consider when choosing a programming language to learn:
- Job Market Demand: Research which languages are most in-demand in your desired industry or location.
- Project Requirements: Consider the types of projects you want to work on and which languages are best suited for them.
- Learning Curve: Some languages are more beginner-friendly than others. Consider your current skill level and how much time you can dedicate to learning.
- Community and Resources: Languages with large, active communities often have more learning resources and third-party libraries available.
- Future Prospects: Consider the language’s growth trajectory and potential future applications.
The Importance of Algorithmic Thinking
Regardless of the programming language you choose to learn, developing strong algorithmic thinking skills is crucial for success in the tech industry. Platforms like AlgoCademy offer interactive coding tutorials and resources that focus on algorithmic problem-solving, which is essential for technical interviews at major tech companies.
By honing your algorithmic thinking skills, you’ll be better equipped to:
- Solve complex coding problems efficiently
- Optimize code for better performance
- Tackle technical interview questions with confidence
- Adapt to new programming languages and paradigms more easily
Conclusion
The programming language landscape in 2023 offers a diverse array of options for developers at all levels. Whether you’re just starting your coding journey or looking to expand your skill set, understanding these top programming languages can help guide your learning path and career decisions.
Remember that while mastering a specific language is important, the fundamental concepts of programming and problem-solving are universal. Focus on developing a strong foundation in computer science principles, and you’ll be well-equipped to adapt to the ever-changing world of technology.
As you embark on your journey to learn these top programming languages, consider leveraging resources like AlgoCademy to strengthen your algorithmic thinking and problem-solving skills. With dedication, practice, and the right resources, you’ll be well on your way to becoming a proficient programmer in 2023 and beyond.