Object-Oriented Programming: A Comprehensive Guide for Aspiring Developers
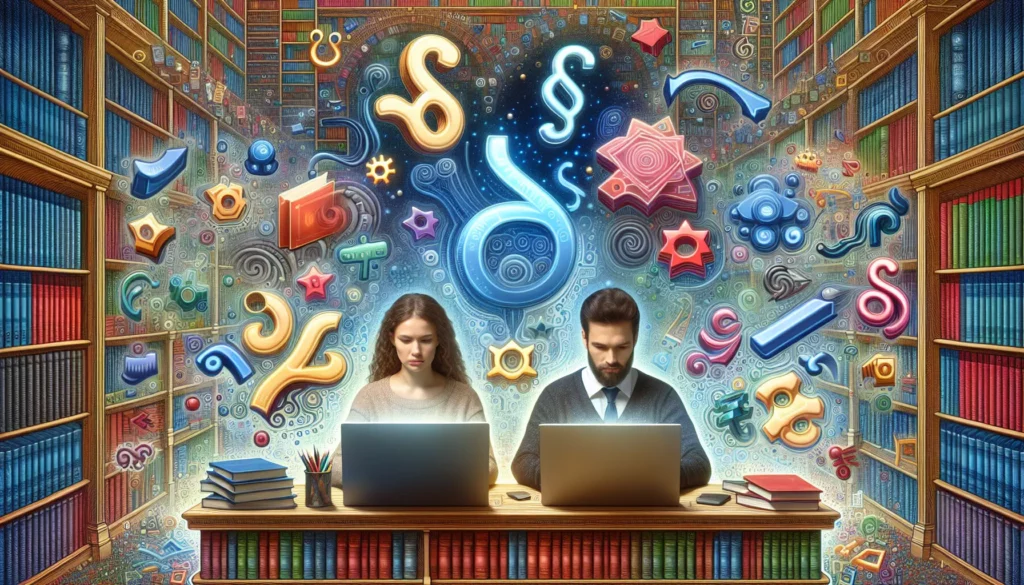
In the vast landscape of programming paradigms, Object-Oriented Programming (OOP) stands out as a cornerstone of modern software development. Whether you’re a beginner taking your first steps into the world of coding or an experienced developer looking to refine your skills, understanding OOP is crucial for your growth and success in the tech industry. In this comprehensive guide, we’ll dive deep into the concept of object-oriented programming, explore its importance, and see how it can elevate your coding prowess to new heights.
What is Object-Oriented Programming?
Object-Oriented Programming is a programming paradigm that organizes software design around data, or objects, rather than functions and logic. An object is a data field that has unique attributes and behavior. OOP focuses on the objects that developers want to manipulate rather than the logic required to manipulate them.
This approach to programming mimics a lot of real-world scenarios, making it easier for developers to create and understand complex systems. By breaking down a problem into smaller, manageable objects, OOP allows for more intuitive problem-solving and code organization.
The Four Pillars of OOP
To truly grasp the essence of object-oriented programming, it’s essential to understand its four fundamental principles:
- Encapsulation: This principle involves bundling the data and the methods that operate on that data within one unit, like a class. Encapsulation also means restricting direct access to some of an object’s components, which is a means of preventing accidental interference and misuse of the methods and data.
- Abstraction: Abstraction means hiding complex implementation details and showing only the necessary features of an object. It helps in reducing programming complexity and effort.
- Inheritance: This allows new classes to be based on existing classes, inheriting their attributes and methods. Inheritance promotes code reusability and establishes a relationship between different classes.
- Polymorphism: Polymorphism allows objects of different classes to be treated as objects of a common super class. It provides a way to perform a single action in different forms and allows you to invoke methods of a derived class through base class reference during runtime.
Why is Object-Oriented Programming Important?
Now that we’ve defined OOP and its core principles, let’s explore why it’s so crucial in modern software development:
1. Modularity for Easier Troubleshooting
Object-oriented programming is inherently modular. When working with objects, you can isolate areas of complexity, making it easier to test and debug your code. If an object is experiencing issues, you can focus on that specific object rather than sifting through an entire monolithic codebase.
2. Reusability Through Inheritance
One of the most powerful features of OOP is the ability to reuse code through inheritance. By creating a new class that extends an existing one, you can leverage all the functionality of the parent class while adding or modifying behaviors specific to the child class. This not only saves time but also reduces the chances of introducing errors when implementing similar functionalities.
3. Flexibility Through Polymorphism
Polymorphism allows for flexibility in how you interact with objects of different types. This is particularly useful when you’re working with complex systems where objects may need to be processed differently based on their specific type, all while maintaining a common interface.
4. Effective Problem Modeling
OOP allows developers to model real-world problems more effectively. By thinking in terms of objects with properties and behaviors, you can create more intuitive and logical representations of complex systems. This approach often leads to cleaner, more maintainable code that’s easier for other developers to understand and work with.
5. Improved Software Maintainability
The modular nature of OOP, combined with encapsulation and abstraction, makes software easier to maintain over time. Changes can be made to specific objects without affecting the entire system, and new features can be added by creating new objects that interact with existing ones.
6. Enhanced Productivity and Scalability
OOP principles promote better organization of code, which can significantly boost productivity, especially in large-scale projects. As systems grow, the object-oriented approach allows for easier management of complexity and more efficient scaling of applications.
Practical Examples of OOP in Action
To better understand how OOP works in practice, let’s look at some examples using Python, a language known for its clear and readable syntax:
Example 1: Creating a Simple Class
Here’s a basic example of how to create a class in Python:
class Dog:
def __init__(self, name, age):
self.name = name
self.age = age
def bark(self):
print(f"{self.name} says Woof!")
# Creating an instance of the Dog class
my_dog = Dog("Buddy", 3)
my_dog.bark() # Output: Buddy says Woof!
In this example, we’ve created a Dog
class with attributes (name and age) and a method (bark). We then create an instance of this class and call its method.
Example 2: Inheritance
Let’s see how inheritance works by creating a subclass of our Dog
class:
class Labrador(Dog):
def __init__(self, name, age, color):
super().__init__(name, age)
self.color = color
def swim(self):
print(f"{self.name} is swimming. Labradors love water!")
my_lab = Labrador("Max", 2, "Chocolate")
my_lab.bark() # Output: Max says Woof!
my_lab.swim() # Output: Max is swimming. Labradors love water!
Here, the Labrador
class inherits from the Dog
class, adding a new attribute (color) and a new method (swim).
Example 3: Polymorphism
Polymorphism allows us to use a single interface with different underlying forms. Here’s an example:
class Cat:
def __init__(self, name, age):
self.name = name
self.age = age
def make_sound(self):
return "Meow!"
class Dog:
def __init__(self, name, age):
self.name = name
self.age = age
def make_sound(self):
return "Woof!"
def animal_sound(animal):
print(f"{animal.name} says {animal.make_sound()}")
cat = Cat("Whiskers", 4)
dog = Dog("Rover", 6)
animal_sound(cat) # Output: Whiskers says Meow!
animal_sound(dog) # Output: Rover says Woof!
In this example, we have two different classes (Cat
and Dog
) with a common method (make_sound
). The animal_sound
function can work with objects of either class, demonstrating polymorphism.
OOP in Different Programming Languages
While we’ve used Python in our examples, it’s worth noting that OOP is supported by many popular programming languages, each with its own syntax and peculiarities:
Java
Java is a class-based, object-oriented programming language designed to be portable and run on any platform. It’s known for its “write once, run anywhere” philosophy.
public class Dog {
private String name;
private int age;
public Dog(String name, int age) {
this.name = name;
this.age = age;
}
public void bark() {
System.out.println(name + " says Woof!");
}
}
// Usage
Dog myDog = new Dog("Buddy", 3);
myDog.bark(); // Output: Buddy says Woof!
C++
C++ is an extension of C that adds object-oriented features. It’s known for its performance and is widely used in system/application software, drivers, client-server applications, and embedded firmware.
#include <iostream>
#include <string>
class Dog {
private:
std::string name;
int age;
public:
Dog(std::string name, int age) : name(name), age(age) {}
void bark() {
std::cout << name << " says Woof!" << std::endl;
}
};
// Usage
int main() {
Dog myDog("Buddy", 3);
myDog.bark(); // Output: Buddy says Woof!
return 0;
}
JavaScript
JavaScript is a prototype-based object-oriented language. While it doesn’t have classes in the traditional sense, it does support object-oriented programming patterns:
class Dog {
constructor(name, age) {
this.name = name;
this.age = age;
}
bark() {
console.log(`${this.name} says Woof!`);
}
}
// Usage
const myDog = new Dog("Buddy", 3);
myDog.bark(); // Output: Buddy says Woof!
OOP Best Practices
To make the most of object-oriented programming, consider these best practices:
- Keep it DRY (Don’t Repeat Yourself): Use inheritance and composition to reuse code and avoid repetition.
- Follow the Single Responsibility Principle: Each class should have one, and only one, reason to change.
- Use meaningful names: Choose clear, descriptive names for your classes, methods, and variables.
- Favor composition over inheritance: While inheritance is powerful, overuse can lead to inflexible designs. Consider using composition to create more flexible and maintainable code.
- Keep classes small and focused: Large classes with many responsibilities are harder to understand and maintain. Break them down into smaller, more focused classes.
- Use access modifiers: Properly use private, protected, and public access modifiers to control access to class members.
- Write clean, readable code: Follow coding standards and conventions to ensure your code is easy to read and understand.
Challenges in Object-Oriented Programming
While OOP offers many benefits, it’s not without its challenges:
- Steep learning curve: For beginners, understanding and implementing OOP concepts can be challenging.
- Overhead: OOP can introduce some performance overhead, especially in small programs.
- Overengineering: It’s easy to fall into the trap of creating overly complex class hierarchies.
- Tight coupling: If not designed carefully, OOP can lead to tightly coupled code that’s difficult to modify.
OOP in Modern Software Development
Object-oriented programming continues to be a fundamental paradigm in modern software development. It’s particularly well-suited for:
- Large-scale software systems: OOP’s modularity and reusability make it ideal for managing complex, large-scale projects.
- GUI Programming: Object-oriented design naturally lends itself to creating user interfaces, where each UI element can be an object.
- Game Development: Games often involve complex systems of interacting objects, making OOP a natural fit.
- Web Development: Many web frameworks and libraries are built around OOP principles.
Preparing for Technical Interviews with OOP
If you’re preparing for technical interviews, especially with major tech companies, having a solid grasp of OOP is crucial. Here are some tips:
- Practice implementing design patterns: Familiarize yourself with common OOP design patterns like Singleton, Factory, Observer, etc.
- Solve object-oriented design problems: Practice designing systems using OOP principles. For example, design a parking lot system or a library management system.
- Be ready to explain OOP concepts: Be prepared to explain encapsulation, abstraction, inheritance, and polymorphism, and provide examples of each.
- Understand OOP in your preferred language: Know how OOP is implemented in the programming language you’re most comfortable with.
- Practice refactoring: Take procedural code and refactor it into an object-oriented design.
Conclusion
Object-Oriented Programming is more than just a programming paradigm; it’s a powerful tool for creating scalable, maintainable, and efficient software systems. By organizing code into objects that model real-world entities or abstract concepts, OOP allows developers to create complex applications that are easier to understand, debug, and extend.
As you continue your journey in software development, whether you’re just starting out or preparing for technical interviews with top tech companies, mastering OOP will be invaluable. It will not only make you a more effective programmer but also give you a deeper understanding of software design and architecture.
Remember, like any skill, proficiency in OOP comes with practice. Start small, gradually tackle more complex problems, and don’t be afraid to refactor your code as you learn new concepts and best practices. With time and dedication, you’ll find that thinking in terms of objects becomes second nature, opening up new possibilities in your programming career.
Happy coding, and may your objects always be well-encapsulated!