11 Exciting Projects to Boost Your Programming Skills
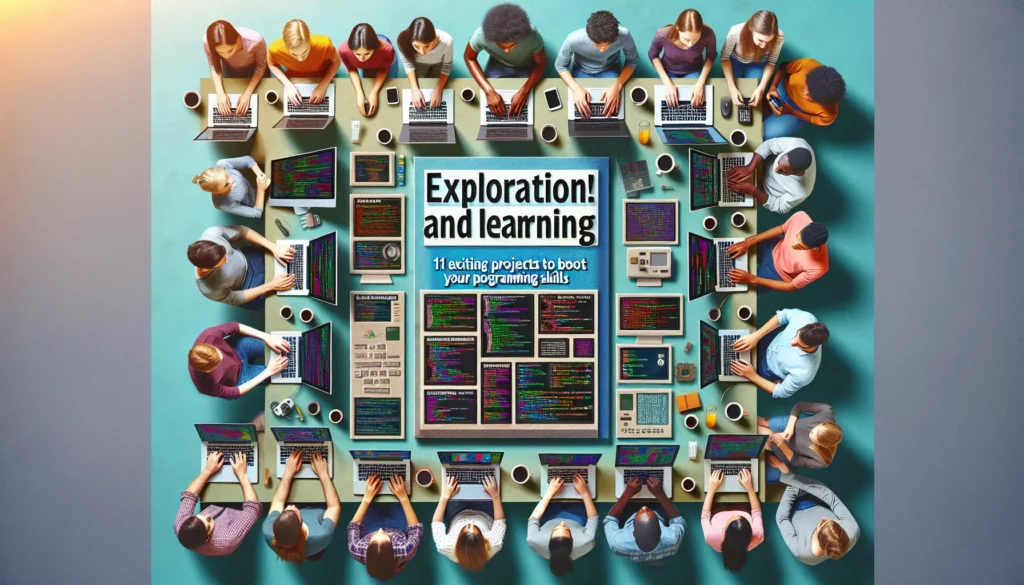
As an aspiring programmer or someone looking to enhance their coding abilities, one of the most effective ways to improve is by working on real-world projects. These hands-on experiences not only reinforce your theoretical knowledge but also help you develop problem-solving skills and familiarity with industry-standard tools and practices. In this comprehensive guide, we’ll explore 11 exciting projects that can significantly boost your programming skills, ranging from beginner-friendly tasks to more advanced undertakings.
1. Build a Personal Portfolio Website
Creating a personal portfolio website is an excellent starting point for beginners and a great way to showcase your skills to potential employers.
Key Learning Points:
- HTML, CSS, and JavaScript fundamentals
- Responsive web design
- Basic SEO principles
- Version control with Git
Project Steps:
- Design the layout of your website
- Create the HTML structure
- Style your website with CSS
- Add interactivity with JavaScript
- Make it responsive for different screen sizes
- Deploy your website using platforms like GitHub Pages or Netlify
This project will give you a solid foundation in web development and help you understand how different technologies work together to create a functional website.
2. Develop a To-Do List Application
A to-do list app is a classic project that introduces you to the basics of CRUD (Create, Read, Update, Delete) operations and data persistence.
Key Learning Points:
- Frontend framework basics (e.g., React, Vue, or Angular)
- State management
- Local storage or backend integration
- User input handling and form validation
Project Steps:
- Set up your development environment
- Create the user interface for adding, viewing, and managing tasks
- Implement task creation and storage functionality
- Add features like task editing, deletion, and marking as complete
- Implement data persistence using local storage or a backend service
- Add user authentication (optional, for more advanced learners)
This project will help you understand how to manage application state and handle user interactions effectively.
3. Create a Weather App
Building a weather application introduces you to working with APIs and handling asynchronous operations in your code.
Key Learning Points:
- API integration
- Asynchronous JavaScript (Promises, async/await)
- Data parsing and manipulation
- Error handling
Project Steps:
- Sign up for a weather API service (e.g., OpenWeatherMap)
- Create a user interface for entering location and displaying weather information
- Implement API calls to fetch weather data
- Parse and display the received data
- Add features like geolocation and forecast visualization
- Implement error handling for API failures or invalid user input
This project will teach you how to work with external data sources and handle potential issues that may arise during API communication.
4. Develop a Simple Blog Platform
Creating a blog platform is an excellent way to learn about full-stack development, including both frontend and backend technologies.
Key Learning Points:
- Backend development (e.g., Node.js with Express, Django, or Ruby on Rails)
- Database management (e.g., MongoDB, PostgreSQL)
- User authentication and authorization
- RESTful API design
Project Steps:
- Set up your backend environment and choose a database
- Design and implement the database schema for blog posts and users
- Create API endpoints for CRUD operations on blog posts
- Implement user authentication and authorization
- Develop a frontend interface for creating, editing, and viewing blog posts
- Add features like comments, tags, and search functionality
This project will give you a comprehensive understanding of how different components of a web application work together, from the database to the user interface.
5. Build a Chat Application
Developing a real-time chat application introduces you to the world of websockets and real-time communication protocols.
Key Learning Points:
- WebSocket technology
- Real-time data handling
- Scalability considerations
- User presence and status management
Project Steps:
- Set up a WebSocket server (e.g., using Socket.io with Node.js)
- Create a user interface for the chat application
- Implement real-time message sending and receiving
- Add features like user typing indicators and online status
- Implement chat rooms or private messaging
- Add message persistence using a database
This project will help you understand the principles of real-time communication and how to handle concurrent user interactions efficiently.
6. Develop an E-commerce Platform
Building an e-commerce platform is a complex project that covers various aspects of web development, from user authentication to payment processing.
Key Learning Points:
- Shopping cart functionality
- Payment gateway integration
- Inventory management
- Order processing and tracking
Project Steps:
- Design the database schema for products, users, and orders
- Create product listing and detail pages
- Implement a shopping cart system
- Add user authentication and account management
- Integrate a payment gateway (e.g., Stripe, PayPal)
- Implement order processing and inventory management
This project will give you insights into building complex, multi-faceted web applications and handling sensitive user data securely.
7. Create a URL Shortener
A URL shortener is a great project to learn about efficient data storage and retrieval, as well as handling high-volume requests.
Key Learning Points:
- Efficient database indexing
- URL redirection techniques
- Unique identifier generation
- Caching strategies
Project Steps:
- Design a database schema for storing long and short URLs
- Implement an algorithm for generating unique short codes
- Create an API endpoint for shortening URLs
- Implement URL redirection functionality
- Add features like custom short codes and expiration dates
- Implement analytics to track link clicks and usage
This project will teach you about optimizing database operations and handling URL redirections efficiently.
8. Develop a Simple Game
Creating a game, even a simple one, can help you improve your problem-solving skills and understand complex logic implementation.
Key Learning Points:
- Game logic and mechanics
- Graphics rendering (e.g., using HTML5 Canvas)
- User input handling
- Performance optimization
Project Steps:
- Choose a simple game concept (e.g., Tic-Tac-Toe, Snake, or Pong)
- Design the game’s user interface
- Implement the core game mechanics and rules
- Add graphics and animations
- Implement user controls and input handling
- Add scoring and game-over conditions
This project will challenge you to think about user experience and game design principles while improving your programming skills.
9. Build a RESTful API
Creating a RESTful API is crucial for understanding how modern web applications communicate and share data.
Key Learning Points:
- RESTful architecture principles
- API design best practices
- Authentication and authorization for APIs
- API documentation
Project Steps:
- Choose a domain for your API (e.g., a book library or movie database)
- Design the API endpoints and data models
- Implement CRUD operations for your resources
- Add authentication and authorization mechanisms
- Implement pagination and filtering for large datasets
- Create comprehensive API documentation
This project will give you a deep understanding of API design principles and how to create scalable, maintainable web services.
10. Develop a Chrome Extension
Building a Chrome extension allows you to extend browser functionality and learn about browser APIs and web security concepts.
Key Learning Points:
- Browser extension architecture
- Chrome extension APIs
- Cross-origin resource sharing (CORS)
- Web security best practices
Project Steps:
- Come up with an idea for a useful browser extension
- Create the extension’s manifest file
- Implement the extension’s functionality using HTML, CSS, and JavaScript
- Use Chrome extension APIs to interact with browser features
- Add options and settings for your extension
- Package and publish your extension to the Chrome Web Store
This project will introduce you to browser-specific development and help you understand how to create tools that enhance the web browsing experience.
11. Create a Data Visualization Dashboard
Building a data visualization dashboard helps you learn about processing and presenting large datasets in an intuitive manner.
Key Learning Points:
- Data processing and analysis
- Charting libraries (e.g., D3.js, Chart.js)
- Interactive visualizations
- Performance optimization for large datasets
Project Steps:
- Choose a dataset to visualize (e.g., COVID-19 statistics, stock market data)
- Process and clean the data as needed
- Design the layout of your dashboard
- Implement various chart types to represent different aspects of the data
- Add interactivity to the visualizations (e.g., filtering, zooming)
- Optimize the dashboard for performance with large datasets
This project will enhance your data handling skills and teach you how to present complex information in an accessible, visual format.
Conclusion
Embarking on these projects will significantly boost your programming skills across various domains of software development. Each project offers unique challenges and learning opportunities, helping you build a well-rounded skill set that is highly valued in the industry.
Remember, the key to improving your programming skills is consistent practice and pushing yourself to tackle new challenges. As you work through these projects, don’t hesitate to explore additional features or technologies that interest you. The more you experiment and learn, the more proficient you’ll become as a programmer.
Additionally, consider using platforms like AlgoCademy to supplement your project-based learning. AlgoCademy offers interactive coding tutorials, resources for learners, and tools to help you progress from beginner-level coding to preparing for technical interviews at major tech companies. With its focus on algorithmic thinking and problem-solving, AlgoCademy can provide valuable guidance as you work on these projects and beyond.
Remember that becoming a skilled programmer is a journey, not a destination. Each project you complete will bring new insights and areas for improvement. Embrace the learning process, stay curious, and keep coding!