How to Prepare for Coding Interviews at Top Tech Companies: A Comprehensive Guide
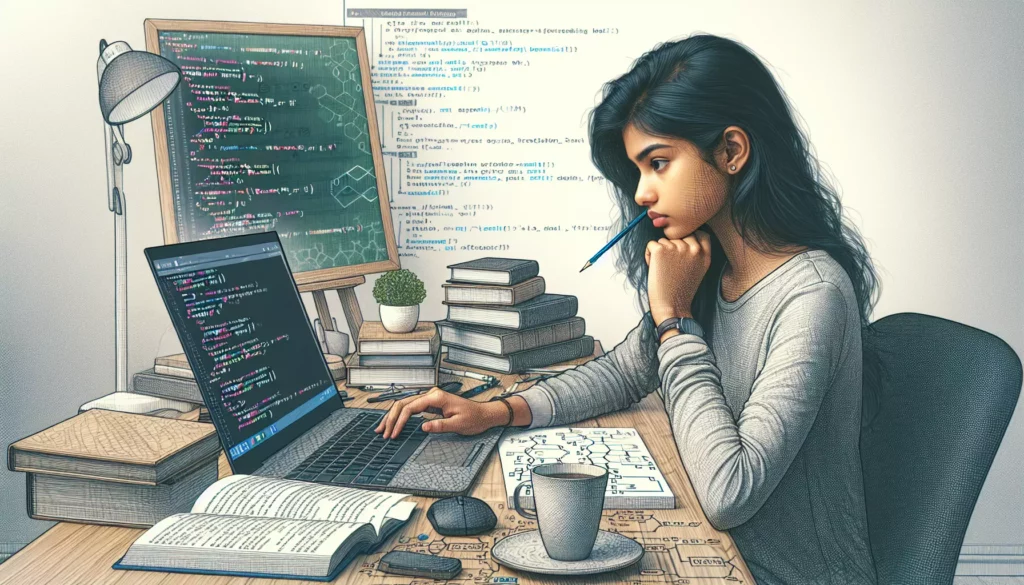
Landing a job at a top tech company like Google, Facebook, Amazon, Apple, or Microsoft (often collectively referred to as FAANG) is a dream for many software engineers. However, the road to securing a position at these tech giants is paved with challenging coding interviews that test not only your programming skills but also your problem-solving abilities and algorithmic thinking. In this comprehensive guide, we’ll walk you through the essential steps to prepare for coding interviews at top tech companies, ensuring you’re well-equipped to showcase your skills and land your dream job.
1. Understand the Interview Process
Before diving into preparation, it’s crucial to understand what to expect during the interview process at top tech companies. While the exact process may vary, most follow a similar structure:
- Initial Screening: Usually a phone or video call with a recruiter to discuss your background and interest in the role.
- Technical Phone Screen: A coding interview conducted over the phone or video call, often using a shared coding environment.
- On-site Interviews: Multiple rounds of face-to-face interviews, including coding challenges, system design discussions, and behavioral questions.
- Final Decision: The hiring committee reviews your performance and makes a decision.
Understanding this process will help you tailor your preparation to each stage and manage your expectations throughout the journey.
2. Master the Fundamentals of Data Structures and Algorithms
A solid grasp of data structures and algorithms is the foundation of success in coding interviews. Focus on mastering the following key areas:
Data Structures:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees and Graphs
- Hash Tables
- Heaps
Algorithms:
- Sorting and Searching
- Recursion and Dynamic Programming
- Greedy Algorithms
- Graph Algorithms (BFS, DFS, Dijkstra’s, etc.)
- Divide and Conquer
- Bit Manipulation
Make sure you not only understand these concepts theoretically but can also implement them efficiently in code. Practice writing clean, optimized solutions to problems involving these data structures and algorithms.
3. Practice Coding Problems Regularly
Consistent practice is key to improving your problem-solving skills and coding speed. Here are some strategies to make your practice effective:
- Use Online Platforms: Websites like LeetCode, HackerRank, and CodeSignal offer a wide range of coding problems similar to those asked in tech interviews.
- Follow a Structured Approach: Start with easy problems and gradually move to medium and hard difficulties. Aim to solve at least one problem daily.
- Time Yourself: Practice solving problems within a time limit to simulate interview conditions.
- Review Solutions: After solving a problem, compare your solution with others to learn different approaches and optimizations.
- Focus on Quality over Quantity: It’s better to thoroughly understand and master 100 problems than to rush through 500 without grasping the underlying concepts.
4. Learn to Communicate Your Thought Process
In coding interviews, how you approach a problem is often as important as solving it. Practice explaining your thought process out loud while coding. This skill, known as “thinking aloud,” helps interviewers understand your problem-solving approach. Here’s how to improve this skill:
- Clearly state the problem and ask clarifying questions.
- Discuss potential approaches before starting to code.
- Explain your reasoning for choosing a particular solution.
- Walk through your code step-by-step as you write it.
- Discuss the time and space complexity of your solution.
Consider recording yourself solving problems or practice with a friend to get comfortable with this process.
5. Study System Design and Scalability
For more senior positions, system design questions are crucial. These questions test your ability to design large-scale distributed systems. Key areas to focus on include:
- Scalability and Load Balancing
- Database Sharding
- Caching Mechanisms
- Microservices Architecture
- API Design
- Data Storage Solutions (SQL vs. NoSQL)
Resources like “Designing Data-Intensive Applications” by Martin Kleppmann and online courses on system design can be invaluable for this preparation.
6. Brush Up on Computer Science Fundamentals
While data structures and algorithms are the primary focus, don’t neglect other computer science fundamentals. Review these topics:
- Operating Systems Concepts
- Computer Networks
- Database Management Systems
- Object-Oriented Programming
- Design Patterns
- Concurrency and Multithreading
Having a well-rounded knowledge base will help you tackle a wider range of questions and demonstrate your depth of understanding.
7. Mock Interviews and Peer Programming
Simulating the interview environment is crucial for building confidence and identifying areas for improvement. Here’s how to incorporate mock interviews into your preparation:
- Use Interview Simulation Platforms: Websites like Pramp or interviewing.io offer free peer-to-peer mock interviews.
- Practice with Friends or Colleagues: Take turns interviewing each other and providing constructive feedback.
- Record Your Sessions: Review your performance to identify areas for improvement in your communication and problem-solving approach.
- Seek Diverse Feedback: Try to practice with people from different backgrounds and experience levels to get varied perspectives.
Remember, the goal is not just to solve the problem but to practice communicating effectively under pressure.
8. Develop a Problem-Solving Framework
Having a structured approach to problem-solving can help you tackle unfamiliar questions more effectively. Here’s a framework you can use:
- Understand the Problem: Clarify any ambiguities and confirm your understanding with the interviewer.
- Analyze the Constraints: Consider time and space complexity requirements.
- Brainstorm Solutions: Think of multiple approaches before settling on one.
- Design the Algorithm: Outline your approach before coding.
- Implement the Solution: Write clean, readable code.
- Test and Debug: Use example inputs and edge cases to verify your solution.
- Optimize: Discuss potential improvements or alternative solutions.
Practice applying this framework to various problems to make it second nature during interviews.
9. Learn to Handle Behavioral Questions
While technical skills are crucial, top tech companies also value soft skills and cultural fit. Prepare for behavioral questions that assess your teamwork, leadership, and problem-solving abilities. Common themes include:
- Handling conflicts with team members
- Overcoming challenging projects or deadlines
- Demonstrating leadership and initiative
- Discussing your biggest achievements and failures
Use the STAR method (Situation, Task, Action, Result) to structure your responses to these questions.
10. Stay Updated with Industry Trends
Demonstrating knowledge of current trends in technology can set you apart in interviews. Stay informed about:
- Latest programming languages and frameworks
- Cloud computing technologies
- Machine learning and artificial intelligence advancements
- Cybersecurity practices
- Blockchain and cryptocurrency developments
Follow tech blogs, attend webinars, or participate in online tech communities to stay updated.
11. Optimize Your Resume and Online Presence
Before you even get to the interview stage, your resume and online presence play a crucial role in getting noticed by recruiters. Here are some tips:
- Tailor Your Resume: Highlight projects and experiences relevant to the position you’re applying for.
- Quantify Achievements: Use metrics to demonstrate the impact of your work.
- Showcase Personal Projects: Include links to your GitHub repositories or personal website.
- Optimize Your LinkedIn Profile: Ensure it’s up-to-date and includes keywords relevant to your target roles.
- Contribute to Open Source: Participation in open-source projects can demonstrate your coding skills and collaboration abilities.
12. Leverage Online Learning Resources
Take advantage of the wealth of online resources available for interview preparation. Some recommended platforms and materials include:
- Courses: Coursera, edX, and Udacity offer courses on algorithms and data structures.
- Books: “Cracking the Coding Interview” by Gayle Laakmann McDowell is a classic resource.
- YouTube Channels: Channels like “Back To Back SWE” and “Tech Dose” offer in-depth explanations of common interview problems.
- Coding Platforms: LeetCode, HackerRank, and CodeSignal provide practice problems and contests.
- Interview Experience Sharing: Websites like Glassdoor and Blind offer insights into company-specific interview processes.
13. Master the Art of Whiteboard Coding
Many on-site interviews involve coding on a whiteboard, which can be challenging if you’re not used to it. Here are some tips to improve your whiteboard coding skills:
- Practice writing code by hand or on a physical whiteboard.
- Focus on writing clean, readable code with proper indentation.
- Leave space between lines for potential additions or corrections.
- Use clear variable names and add comments to explain your logic.
- Practice erasing and rewriting parts of your code smoothly.
Remember, interviewers are more interested in your problem-solving process than perfect syntax on a whiteboard.
14. Develop Strategies for Handling Difficult Questions
You may encounter questions that you’re unsure how to solve. Here’s how to handle these situations:
- Stay Calm: Don’t panic if you don’t immediately know the answer.
- Break Down the Problem: Try to solve smaller parts of the problem if you can’t solve the whole thing.
- Think Aloud: Share your thoughts and partial ideas with the interviewer.
- Ask for Hints: It’s okay to ask for guidance if you’re stuck.
- Propose a Brute Force Solution: Even if it’s not optimal, it shows you can approach the problem.
Remember, interviewers often want to see how you think and approach challenges, not just whether you can solve every problem perfectly.
15. Prepare Questions for Your Interviewers
At the end of most interviews, you’ll have the opportunity to ask questions. This is your chance to demonstrate your interest in the company and role. Prepare thoughtful questions such as:
- What are the biggest challenges facing the team/department right now?
- Can you describe the team’s development process?
- How does the company support professional growth and learning?
- What do you enjoy most about working here?
- How does this role contribute to the company’s overall mission?
Asking insightful questions shows that you’re engaged and serious about the opportunity.
Conclusion
Preparing for coding interviews at top tech companies is a challenging but rewarding process. It requires dedication, consistent practice, and a strategic approach to learning and problem-solving. By following the steps outlined in this guide, you’ll be well-equipped to tackle even the most demanding technical interviews.
Remember, the key to success lies not just in memorizing solutions but in developing a deep understanding of fundamental concepts and honing your problem-solving skills. Embrace the learning process, stay persistent, and approach each interview as an opportunity to grow and showcase your abilities.
As you embark on this journey, keep in mind that interview preparation is a marathon, not a sprint. Set realistic goals, maintain a consistent study schedule, and don’t be discouraged by setbacks. With the right preparation and mindset, you’ll be well on your way to landing your dream job at a top tech company.
Good luck with your interview preparation, and may your hard work lead you to success in your tech career!