How to Learn Python Programming Quickly: A Comprehensive Guide
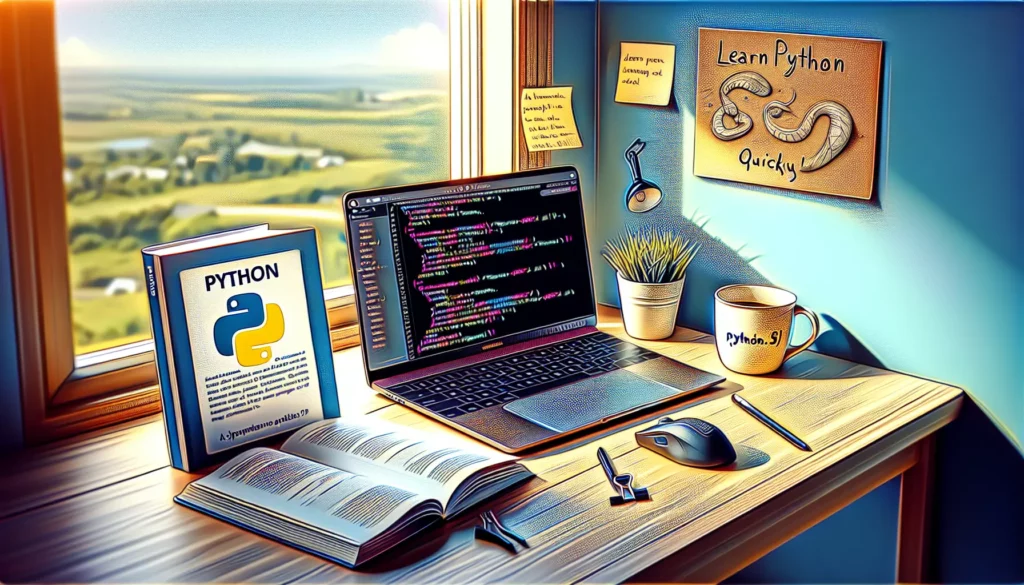
Python has become one of the most popular programming languages in the world, and for good reason. Its simplicity, versatility, and powerful libraries make it an excellent choice for beginners and experienced developers alike. Whether you’re looking to start a career in tech, enhance your data analysis skills, or simply explore the world of coding, learning Python quickly can be a game-changer. In this comprehensive guide, we’ll explore effective strategies and resources to help you master Python programming in record time.
1. Understanding the Importance of Python
Before diving into the learning process, it’s crucial to understand why Python is worth your time and effort:
- Versatility: Python is used in web development, data science, artificial intelligence, machine learning, automation, and more.
- Readability: Python’s clean and straightforward syntax makes it easier to learn and understand compared to many other programming languages.
- Community Support: A vast and active community means abundant resources, libraries, and frameworks are available.
- Career Opportunities: Python skills are in high demand across various industries, opening up numerous job prospects.
2. Setting Clear Goals and Creating a Learning Plan
To learn Python quickly, you need a structured approach:
- Define Your Objectives: Determine why you want to learn Python. Is it for web development, data analysis, or something else?
- Set Realistic Timeframes: Decide how much time you can dedicate daily or weekly to learning Python.
- Choose Learning Resources: Select a combination of online courses, books, and interactive platforms that suit your learning style.
- Create Milestones: Break down your learning journey into smaller, achievable goals to track your progress.
3. Start with the Basics
Begin your Python journey by mastering the fundamentals:
3.1. Python Syntax and Data Types
Familiarize yourself with Python’s basic syntax and common data types:
- Variables and naming conventions
- Strings, integers, floats, and booleans
- Lists, tuples, and dictionaries
- Basic operators and expressions
3.2. Control Flow
Learn how to control the flow of your programs:
- If-else statements
- For and while loops
- Break and continue statements
3.3. Functions and Modules
Understand how to create reusable code:
- Defining and calling functions
- Function arguments and return values
- Importing and using modules
4. Hands-On Practice: The Key to Rapid Learning
The fastest way to learn Python is through consistent practice. Here are some strategies to incorporate hands-on coding into your learning routine:
4.1. Code Every Day
Make it a habit to write Python code daily, even if it’s just for 30 minutes. Consistency is key to rapid improvement.
4.2. Work on Small Projects
Start with simple projects and gradually increase complexity. Some ideas include:
- A calculator program
- A to-do list application
- A simple game like rock-paper-scissors
- A basic web scraper
4.3. Solve Coding Challenges
Platforms like LeetCode, HackerRank, and CodeWars offer coding challenges that can sharpen your problem-solving skills and Python proficiency.
4.4. Contribute to Open Source Projects
Once you’ve gained some confidence, contributing to open-source projects can provide real-world coding experience and exposure to best practices.
5. Leverage Online Resources and Tools
Take advantage of the wealth of online resources available for learning Python:
5.1. Online Courses and Tutorials
- Coursera: Offers Python courses from top universities.
- edX: Provides free Python courses with optional paid certificates.
- Codecademy: Features interactive Python lessons with hands-on coding practice.
- FreeCodeCamp: Offers a comprehensive Python curriculum for free.
5.2. Interactive Learning Platforms
- AlgoCademy: Provides interactive coding tutorials and AI-powered assistance for learning Python and preparing for technical interviews.
- Repl.it: An online IDE that allows you to write and run Python code in your browser.
- Jupyter Notebooks: Great for data science and machine learning projects.
5.3. Python Documentation and Official Resources
The official Python documentation is an invaluable resource for understanding the language in-depth:
6. Join the Python Community
Engaging with the Python community can accelerate your learning process:
6.1. Online Forums and Communities
- Stack Overflow: A Q&A platform where you can ask Python-related questions and help others.
- Reddit’s r/learnpython: A supportive community for Python learners.
- Python Discord: A chat platform where you can interact with other Python enthusiasts in real-time.
6.2. Local Meetups and Coding Groups
Look for Python meetups in your area using platforms like Meetup.com. Attending these events can provide networking opportunities and exposure to real-world Python applications.
7. Focus on Python Libraries and Frameworks
Once you’ve grasped the basics, explore popular Python libraries and frameworks relevant to your goals:
7.1. For Web Development
- Django: A high-level web framework for rapid development.
- Flask: A lightweight framework for building web applications.
7.2. For Data Science and Machine Learning
- NumPy: For numerical computing and array operations.
- Pandas: For data manipulation and analysis.
- Scikit-learn: For machine learning algorithms and tools.
- TensorFlow and PyTorch: For deep learning and neural networks.
7.3. For Automation and Scripting
- Requests: For making HTTP requests.
- Beautiful Soup: For web scraping and parsing HTML/XML.
- Selenium: For browser automation and testing.
8. Advanced Topics to Explore
As you progress, delve into more advanced Python concepts to deepen your understanding:
8.1. Object-Oriented Programming (OOP)
Master the principles of OOP in Python:
- Classes and objects
- Inheritance and polymorphism
- Encapsulation and abstraction
8.2. Decorators and Generators
Learn about these powerful Python features:
- Creating and using decorators
- Understanding generator functions and expressions
8.3. Asynchronous Programming
Explore asynchronous programming in Python:
- Asyncio library
- Coroutines and event loops
9. Best Practices for Rapid Python Learning
To maximize your learning efficiency, keep these best practices in mind:
9.1. Code Regularly
Consistency is key. Try to code for at least an hour every day, even if it’s just solving small problems or working on personal projects.
9.2. Read Other People’s Code
Analyzing well-written Python code can help you understand best practices and improve your own coding style. Browse popular open-source projects on GitHub to see how experienced developers structure their code.
9.3. Teach Others
Explaining Python concepts to others can reinforce your own understanding. Consider starting a blog, creating YouTube tutorials, or mentoring beginners in online communities.
9.4. Use Version Control
Learn to use Git and GitHub to manage your code. This skill is essential for collaboration and is highly valued in professional settings.
9.5. Write Clean and Readable Code
Focus on writing clean, well-documented code from the start. Follow the PEP 8 style guide for Python code:
def calculate_average(numbers):
"""
Calculate the average of a list of numbers.
Args:
numbers (list): A list of numbers.
Returns:
float: The average of the numbers.
"""
if not numbers:
return 0
return sum(numbers) / len(numbers)
# Example usage
my_numbers = [1, 2, 3, 4, 5]
average = calculate_average(my_numbers)
print(f"The average is: {average}")
10. Overcoming Common Challenges
As you learn Python quickly, you may encounter some challenges. Here’s how to overcome them:
10.1. Information Overload
Solution: Focus on one concept at a time. Don’t try to learn everything at once. Create a learning roadmap and stick to it.
10.2. Debugging Frustrations
Solution: Embrace debugging as a learning opportunity. Use print statements, debuggers, and error messages to understand what’s going wrong in your code.
10.3. Imposter Syndrome
Solution: Remember that everyone starts as a beginner. Celebrate your progress, no matter how small, and don’t compare yourself to others.
10.4. Lack of Project Ideas
Solution: Look for inspiration in your daily life or work. What problems can you solve with Python? Start with simple ideas and build upon them.
11. Preparing for Technical Interviews
If your goal is to land a Python-related job, prepare for technical interviews:
11.1. Data Structures and Algorithms
Focus on implementing common data structures and algorithms in Python:
- Lists, stacks, queues, and dictionaries
- Sorting and searching algorithms
- Tree and graph traversals
11.2. Time and Space Complexity
Understand Big O notation and be able to analyze the efficiency of your Python code.
11.3. Problem-Solving Skills
Practice solving coding challenges on platforms like LeetCode, HackerRank, or AlgoCademy. Focus on explaining your thought process as you solve problems.
11.4. System Design
For more advanced positions, learn about system design principles and how to architect scalable solutions using Python.
12. Continuing Your Python Journey
Learning Python quickly is just the beginning. To truly master the language and stay current in the field:
12.1. Stay Updated
Follow Python blogs, newsletters, and social media accounts to keep up with the latest developments in the Python ecosystem.
12.2. Attend Conferences and Workshops
Participate in Python conferences like PyCon to network with other developers and learn about cutting-edge Python applications.
12.3. Pursue Certifications
Consider obtaining Python certifications to validate your skills and boost your credibility in the job market.
12.4. Explore Specializations
Once you have a solid foundation, consider specializing in areas like data science, machine learning, or web development with Python.
Conclusion
Learning Python programming quickly is an achievable goal with the right approach and mindset. By following this comprehensive guide, you can accelerate your Python learning journey and build a strong foundation for your programming career. Remember that consistent practice, hands-on projects, and engagement with the Python community are key to rapid progress.
As you embark on this exciting journey, platforms like AlgoCademy can provide valuable support with their interactive tutorials, AI-powered assistance, and focus on practical coding skills. Whether you’re aiming to enhance your problem-solving abilities, prepare for technical interviews, or simply explore the world of coding, Python offers a wealth of opportunities for growth and innovation.
Start your Python adventure today, and you’ll be amazed at how quickly you can progress from a beginner to a proficient Python programmer. Happy coding!