Prompt Engineering for Coders: Unlocking the Power of AI-Assisted Programming
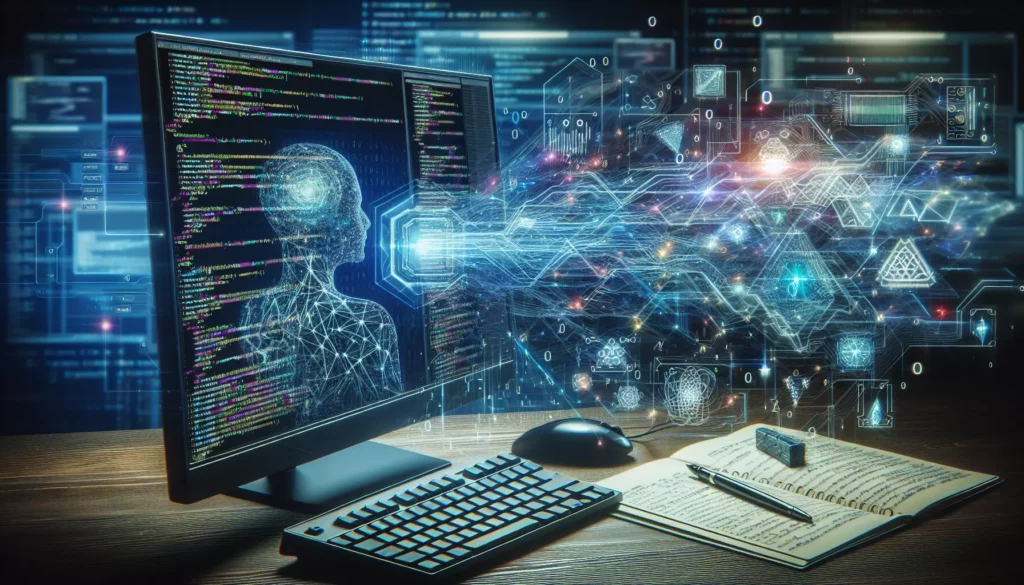
In the rapidly evolving landscape of software development, a new skill has emerged that bridges the gap between human creativity and artificial intelligence: prompt engineering. This powerful technique allows coders to harness the capabilities of large language models (LLMs) like GPT-3 and GPT-4 to enhance their productivity, solve complex problems, and even learn new programming concepts. In this comprehensive guide, we’ll explore how prompt engineering can revolutionize your coding journey, from beginner to advanced levels, and prepare you for the challenges of technical interviews at top tech companies.
What is Prompt Engineering?
Prompt engineering is the art and science of crafting effective inputs (prompts) for AI language models to generate desired outputs. For coders, this means learning how to communicate with AI assistants in a way that produces useful code snippets, explanations, or solutions to programming problems. By mastering prompt engineering, developers can leverage AI as a powerful tool in their coding arsenal.
Why Prompt Engineering Matters for Coders
- Accelerated learning: AI can explain complex concepts and provide tailored examples
- Increased productivity: Generate boilerplate code and solve routine tasks quickly
- Problem-solving assistance: Get help with debugging and algorithm optimization
- Interview preparation: Practice coding challenges with AI-generated problems and solutions
- Exposure to diverse coding styles and best practices
Getting Started with Prompt Engineering for Coding
To begin your journey in prompt engineering for coding, you’ll need access to an AI language model. While OpenAI’s GPT models are popular choices, there are also open-source alternatives and specialized coding assistants available. Here are some steps to get started:
- Choose an AI platform or tool (e.g., OpenAI’s ChatGPT, GitHub Copilot, or Replit’s Ghostwriter)
- Familiarize yourself with the platform’s interface and capabilities
- Start with simple prompts and gradually increase complexity
- Experiment with different prompt structures and styles
- Analyze the AI’s responses and refine your prompts accordingly
Basic Prompt Engineering Techniques for Coders
1. Be Specific and Contextual
When crafting prompts for coding tasks, provide as much context as possible. Specify the programming language, framework, or library you’re working with, and include any relevant constraints or requirements.
Example prompt:
Write a Python function that takes a list of integers as input and returns the sum of all even numbers in the list. Use list comprehension for efficiency.
2. Use Role-Playing
Assign a specific role to the AI to guide its responses. This can be particularly useful when you need explanations or want to simulate a particular coding scenario.
Example prompt:
Act as an experienced Java developer. Explain the concept of dependency injection and provide a simple code example demonstrating its use in a Spring Boot application.
3. Break Down Complex Tasks
For more complex coding problems, break them down into smaller, manageable steps. This helps the AI provide more accurate and focused responses.
Example prompt:
I need to implement a binary search tree in C++. Let's approach this step-by-step:
1. Define the Node structure
2. Implement the insert function
3. Implement the search function
4. Implement the delete function
Please provide the code for step 1 and explain the structure.
4. Request Explanations and Comments
To enhance your learning experience, ask the AI to provide explanations and comments alongside the code it generates.
Example prompt:
Write a JavaScript function to implement the Fisher-Yates shuffle algorithm for an array. Include comments explaining each step of the algorithm and its time complexity.
Advanced Prompt Engineering Techniques for Coding
1. Chain of Thought Prompting
Encourage the AI to show its reasoning process when solving complex problems or optimizing algorithms. This technique can help you understand the thought process behind efficient solutions.
Example prompt:
Solve the "Longest Palindromic Substring" problem in Python. Walk me through your thought process, considering different approaches, their time and space complexities, and why you choose the final solution. Then, provide the optimized code with comments.
2. Few-Shot Learning
Provide examples of input-output pairs to guide the AI’s responses, especially when dealing with specific coding styles or patterns.
Example prompt:
I want to generate unit tests for a React component using Jest and React Testing Library. Here's an example of the format I'm looking for:
Input:
<Button onClick={handleClick} disabled={isLoading}>
{isLoading ? "Loading..." : "Click me"}
</Button>
Output:
import React from 'react';
import { render, screen, fireEvent } from '@testing-library/react';
import Button from './Button';
describe('Button component', () => {
test('renders button with correct text', () => {
render(<Button onClick={() => {}} disabled={false}>Click me</Button>);
expect(screen.getByText('Click me')).toBeInTheDocument();
});
test('calls onClick when clicked', () => {
const handleClick = jest.fn();
render(<Button onClick={handleClick} disabled={false}>Click me</Button>);
fireEvent.click(screen.getByText('Click me'));
expect(handleClick).toHaveBeenCalledTimes(1);
});
test('disables button when disabled prop is true', () => {
render(<Button onClick={() => {}} disabled={true}>Click me</Button>);
expect(screen.getByText('Click me')).toBeDisabled();
});
test('shows loading text when isLoading is true', () => {
render(<Button onClick={() => {}} disabled={false} isLoading={true}>Click me</Button>);
expect(screen.getByText('Loading...')).toBeInTheDocument();
});
});
Now, generate unit tests for the following React component:
<Dropdown
options={['Option 1', 'Option 2', 'Option 3']}
selectedOption={selectedOption}
onSelect={handleSelect}
placeholder="Select an option"
/>
3. Iterative Refinement
Use multiple prompts to refine and improve code generated by the AI. This technique is particularly useful for optimizing algorithms or refactoring complex functions.
Example prompt sequence:
1. Write a Python function to find the nth Fibonacci number using recursion.
2. Now, optimize the previous function using memoization to improve its time complexity.
3. Refactor the memoized version to use an iterative approach instead of recursion.
4. Finally, implement the same functionality using a generator function for memory efficiency when dealing with large n values.
4. Error Analysis and Debugging
Leverage the AI’s ability to analyze code and identify potential issues or bugs. This can be particularly helpful when preparing for technical interviews or debugging complex systems.
Example prompt:
Analyze the following C++ code for potential bugs, inefficiencies, or improvements. Provide a detailed explanation of each issue found and suggest fixes:
#include <iostream>
#include <vector>
int findMaxSum(std::vector<int> &nums) {
int maxSum = 0;
int currentSum = 0;
for (int i = 0; i < nums.size(); i++) {
currentSum += nums[i];
if (currentSum > maxSum) {
maxSum = currentSum;
}
if (currentSum < 0) {
currentSum = 0;
}
}
return maxSum;
}
int main() {
std::vector<int> nums = {-2, 1, -3, 4, -1, 2, 1, -5, 4};
std::cout << "Max sum: " << findMaxSum(nums) << std::endl;
return 0;
}
Prompt Engineering for Technical Interview Preparation
As you progress in your coding journey, prompt engineering can be an invaluable tool for preparing for technical interviews, especially at top tech companies. Here are some strategies to leverage AI for interview preparation:
1. Generate Practice Problems
Use AI to create custom coding challenges that target specific algorithms or data structures you want to practice.
Example prompt:
Generate a leetcode-style coding problem that involves using a hash map and two-pointer technique. The problem should be of medium difficulty and relevant to real-world scenarios a software engineer might encounter at a FAANG company.
2. Simulate Interview Scenarios
Practice your problem-solving skills by simulating a technical interview with the AI acting as the interviewer.
Example prompt:
Act as a senior software engineer conducting a technical interview for a backend developer position at Google. Present a system design question related to building a distributed cache system. Ask follow-up questions based on my responses, and provide feedback on my approach.
3. Code Review and Optimization
Use the AI to review your solutions and suggest optimizations, helping you develop the critical thinking skills necessary for technical interviews.
Example prompt:
Review the following Python code for the "Merge K Sorted Lists" problem. Analyze its time and space complexity, suggest optimizations, and provide an improved solution if possible:
import heapq
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def mergeKLists(lists):
heap = []
for i, lst in enumerate(lists):
if lst:
heapq.heappush(heap, (lst.val, i, lst))
dummy = ListNode(0)
curr = dummy
while heap:
val, i, node = heapq.heappop(heap)
curr.next = ListNode(val)
curr = curr.next
if node.next:
heapq.heappush(heap, (node.next.val, i, node.next))
return dummy.next
4. Explore Different Approaches
Ask the AI to provide multiple solutions to a problem, helping you understand various approaches and trade-offs.
Example prompt:
Provide three different approaches to solve the "Longest Increasing Subsequence" problem in Java. For each approach, explain its algorithm, implement the solution, and analyze its time and space complexity. Finally, compare the trade-offs between the three approaches.
Best Practices for Prompt Engineering in Coding
To make the most of prompt engineering in your coding journey, keep these best practices in mind:
- Verify and understand the code: Always review and test the code generated by AI. Understanding how it works is crucial for learning and avoiding potential issues.
- Combine AI assistance with manual coding: Use AI as a tool to enhance your skills, not replace them. Practice writing code from scratch to build a strong foundation.
- Stay updated with AI capabilities: As language models evolve, their capabilities change. Keep exploring new features and techniques in prompt engineering.
- Use AI ethically: When using AI-generated code in projects, ensure you comply with licensing requirements and give appropriate attribution when necessary.
- Develop a prompt library: Create a collection of effective prompts for common coding tasks and interview preparation. Refine and expand this library over time.
- Practice prompt refinement: Continuously work on improving your prompts to get more accurate and useful responses from the AI.
- Collaborate and share knowledge: Discuss prompt engineering techniques with other developers to learn new approaches and share your insights.
Conclusion: Empowering Your Coding Journey with Prompt Engineering
Prompt engineering is a powerful skill that can significantly enhance your coding abilities, accelerate your learning, and prepare you for the challenges of technical interviews at top tech companies. By mastering the art of crafting effective prompts, you can unlock the full potential of AI language models as coding assistants, problem-solving partners, and learning aids.
As you continue to develop your prompt engineering skills, remember that the goal is not to replace your own coding abilities but to augment them. Use AI-generated code as a starting point for learning, inspiration, and problem-solving, but always strive to understand the underlying concepts and improve your own coding skills.
With practice and persistence, prompt engineering can become an invaluable tool in your coding toolkit, helping you tackle complex problems, explore new programming concepts, and ultimately become a more proficient and versatile software developer. Embrace this emerging skill, and let it propel you towards success in your coding journey and future technical interviews.